Final Commit
DebounceIn Class Reference
A digital input with switch debouncing. More...
#include <DebounceIn.h>
Public Member Functions | |
DebounceIn (PinName pin, timestamp_t us=1000) | |
Create a DebounceIn object connected to the specified pin. | |
void | set_sample_rate (timestamp_t us) |
Set the input sampling rate. | |
int | read (void) |
Read the input, represented as 0 or 1 (int) | |
operator int () | |
An operator shorthand for read() | |
void | rise (void(*fptr)(void)) |
Attach a function to call when a rising edge occurs on the input. | |
template<typename T > | |
void | rise (T *tptr, void(T::*mptr)(void)) |
Attach a member function to call when a rising edge occurs on the input. | |
void | fall (void(*fptr)(void)) |
Attach a function to call when a falling edge occurs on the input. | |
template<typename T > | |
void | fall (T *tptr, void(T::*mptr)(void)) |
Attach a member function to call when a falling edge occurs on the input. |
Detailed Description
A digital input with switch debouncing.
Example:
#include "mbed.h" #include "DebounceIn.h" int nrise = 0, nfall = 0; void rise() { nrise++; } void fall() { nfall++; } int main() { DebounceIn button(p14); button.rise(rise); button.fall(fall); while (true) { printf("nrise=%d, nfall=%d\n", nrise, nfall); wait(1); } }
Definition at line 44 of file DebounceIn.h.
Constructor & Destructor Documentation
DebounceIn | ( | PinName | pin, |
timestamp_t | us = 1000 |
||
) |
Create a DebounceIn object connected to the specified pin.
- Parameters:
-
pin DebounceIn pin to connect to us Input sampling rate (default 1000 microseconds)
Definition at line 19 of file DebounceIn.cpp.
Member Function Documentation
void fall | ( | void(*)(void) | fptr ) |
Attach a function to call when a falling edge occurs on the input.
- Parameters:
-
fptr pointer to a void function, or 0 to set as none
Definition at line 50 of file DebounceIn.cpp.
void fall | ( | T * | tptr, |
void(T::*)(void) | mptr | ||
) |
Attach a member function to call when a falling edge occurs on the input.
- Parameters:
-
tptr pointer to the object to call the member function on mptr pointer to the member function to be called
Definition at line 101 of file DebounceIn.h.
operator int | ( | ) |
An operator shorthand for read()
Definition at line 36 of file DebounceIn.cpp.
int read | ( | void | ) |
Read the input, represented as 0 or 1 (int)
- Returns:
- An integer representing the state of the input pin
Definition at line 29 of file DebounceIn.cpp.
void rise | ( | void(*)(void) | fptr ) |
Attach a function to call when a rising edge occurs on the input.
- Parameters:
-
fptr pointer to a void function, or 0 to set as none
Definition at line 46 of file DebounceIn.cpp.
void rise | ( | T * | tptr, |
void(T::*)(void) | mptr | ||
) |
Attach a member function to call when a rising edge occurs on the input.
- Parameters:
-
tptr pointer to the object to call the member function on mptr pointer to the member function to be called
Definition at line 85 of file DebounceIn.h.
void set_sample_rate | ( | timestamp_t | us ) |
Set the input sampling rate.
- Parameters:
-
us Input sampling rate
Definition at line 41 of file DebounceIn.cpp.
Generated on Mon Aug 1 2022 12:43:05 by
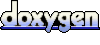