Final Commit
Embed:
(wiki syntax)
Show/hide line numbers
DebounceIn.h
00001 /* A digital input with switch debouncing 00002 * Copyright 2015, Takuo Watanabe 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 00017 #ifndef DEBOUNCEIN_H_ 00018 #define DEBOUNCEIN_H_ 00019 00020 #include "mbed.h" 00021 00022 /** A digital input with switch debouncing 00023 * 00024 * Example: 00025 * @code 00026 * #include "mbed.h" 00027 * #include "DebounceIn.h" 00028 * 00029 * int nrise = 0, nfall = 0; 00030 * void rise() { nrise++; } 00031 * void fall() { nfall++; } 00032 * 00033 * int main() { 00034 * DebounceIn button(p14); 00035 * button.rise(rise); 00036 * button.fall(fall); 00037 * while (true) { 00038 * printf("nrise=%d, nfall=%d\n", nrise, nfall); 00039 * wait(1); 00040 * } 00041 * } 00042 * @endcode 00043 */ 00044 class DebounceIn: public mbed::DigitalIn { 00045 public: 00046 /** Create a DebounceIn object connected to the specified pin 00047 * 00048 * @param pin DebounceIn pin to connect to 00049 * @param us Input sampling rate (default 1000 microseconds) 00050 */ 00051 DebounceIn(PinName pin, timestamp_t us = 1000); 00052 00053 virtual ~DebounceIn(); 00054 00055 /** Set the input sampling rate 00056 * 00057 * @param us Input sampling rate 00058 */ 00059 void set_sample_rate(timestamp_t us); 00060 00061 /** Read the input, represented as 0 or 1 (int) 00062 * 00063 * @returns An integer representing the state of the input pin 00064 */ 00065 int read(void); 00066 00067 #ifdef MBED_OPERATORS 00068 /** An operator shorthand for read() 00069 */ 00070 operator int(); 00071 #endif 00072 00073 /** Attach a function to call when a rising edge occurs on the input 00074 * 00075 * @param fptr pointer to a void function, or 0 to set as none 00076 */ 00077 void rise(void (*fptr)(void)); 00078 00079 /** Attach a member function to call when a rising edge occurs on the input 00080 * 00081 * @param tptr pointer to the object to call the member function on 00082 * @param mptr pointer to the member function to be called 00083 */ 00084 template<typename T> 00085 void rise(T* tptr, void (T::*mptr)(void)) { 00086 _rise.attach(tptr, mptr); 00087 } 00088 00089 /** Attach a function to call when a falling edge occurs on the input 00090 * 00091 * @param fptr pointer to a void function, or 0 to set as none 00092 */ 00093 void fall(void (*fptr)(void)); 00094 00095 /** Attach a member function to call when a falling edge occurs on the input 00096 * 00097 * @param tptr pointer to the object to call the member function on 00098 * @param mptr pointer to the member function to be called 00099 */ 00100 template<typename T> 00101 void fall(T* tptr, void (T::*mptr)(void)) { 00102 _fall.attach(tptr, mptr); 00103 } 00104 00105 private: 00106 Ticker _ticker; 00107 int _prev, _curr; 00108 FunctionPointer _rise, _fall; 00109 void sample(void); 00110 }; 00111 00112 #endif /* DEBOUNCEIN_H_ */
Generated on Mon Aug 1 2022 12:43:05 by
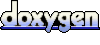