TEST
Dependents: ADXL345Test ADXL345Test1
Fork of TM1638 by
TM1638.h
00001 /* mbed TM1638 Library, for TM1638 LED controller 00002 * Copyright (c) 2015, v01: WH, Initial version 00003 * 2016, v02: WH, refactored display and keyboard defines 00004 * 2016, v03: WH, Added QYF-TM1638 and LKM1638, refactoring of writeData() 00005 * 00006 * Permission is hereby granted, free of charge, to any person obtaining a copy 00007 * of this software and associated documentation files (the "Software"), to deal 00008 * in the Software without restriction, including without limitation the rights 00009 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00010 * copies of the Software, and to permit persons to whom the Software is 00011 * furnished to do so, subject to the following conditions: 00012 * 00013 * The above copyright notice and this permission notice shall be included in 00014 * all copies or substantial portions of the Software. 00015 * 00016 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00017 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00018 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00019 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00020 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00021 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN 00022 * THE SOFTWARE. 00023 */ 00024 00025 #ifndef TM1638_H 00026 #define TM1638_H 00027 00028 // Select one of the testboards for TM1638 LED controller 00029 #include "TM1638_Config.h" 00030 00031 /** An interface for driving TM1638 LED controller 00032 * 00033 * @code 00034 * #include "mbed.h" 00035 * #include "TM1638.h" 00036 * 00037 * DisplayData_t size is 16 bytes (8 grids @ 10 segments) 00038 * TM1638::DisplayData_t all_str = {0xFF,0x3F, 0xFF,0x3F, 0xFF,0x3F, 0xFF,0x3F, 0xFF,0x3F, 0xFF,0x3F, 0xFF,0x3F, 0xFF,0x3F}; 00039 * 00040 * // KeyData_t size is 4 bytes 00041 * TM1638::KeyData_t keydata; 00042 * 00043 * // TM1638 declaration 00044 * TM1638 TM1638(p5,p6,p7, p8); 00045 * 00046 * int main() { 00047 * TM1638.cls(); 00048 * TM1638.writeData(all_str); 00049 * wait(1); 00050 * TM1638.setBrightness(TM1638_BRT0); 00051 * wait(1); 00052 * TM1638.setBrightness(TM1638_BRT3); 00053 * 00054 * while (1) { 00055 * // Check and read keydata 00056 * if (TM1638.getKeys(&keydata)) { 00057 * pc.printf("Keydata 0..3 = 0x%02x 0x%02x 0x%02x 0x%02x\r\n", keydata[0], keydata[1], keydata[2], keydata[3]); 00058 * 00059 * if (keydata[0] == 0x01) { //sw1 00060 * TM1638.cls(); 00061 * TM1638.writeData(all_str); 00062 * } 00063 * } 00064 * } 00065 * } 00066 * @endcode 00067 */ 00068 00069 00070 //TM1638 Display and Keymatrix data 00071 #define TM1638_MAX_NR_GRIDS 8 00072 #define TM1638_BYTES_PER_GRID 2 00073 //Significant bits Keymatrix data 00074 #define TM1638_KEY_MSK 0x77 00075 00076 //Memory size in bytes for Display and Keymatrix 00077 #define TM1638_DISPLAY_MEM (TM1638_MAX_NR_GRIDS * TM1638_BYTES_PER_GRID) 00078 #define TM1638_KEY_MEM 4 00079 00080 00081 //Reserved bits for commands 00082 #define TM1638_CMD_MSK 0xC0 00083 00084 //Data setting commands 00085 #define TM1638_DATA_SET_CMD 0x40 00086 #define TM1638_DATA_WR 0x00 00087 #define TM1638_KEY_RD 0x02 00088 #define TM1638_ADDR_INC 0x00 00089 #define TM1638_ADDR_FIXED 0x04 00090 #define TM1638_MODE_NORM 0x00 00091 #define TM1638_MODE_TEST 0x08 00092 00093 //Address setting commands 00094 #define TM1638_ADDR_SET_CMD 0xC0 00095 #define TM1638_ADDR_MSK 0x0F 00096 00097 //Display control commands 00098 #define TM1638_DSP_CTRL_CMD 0x80 00099 #define TM1638_BRT_MSK 0x07 00100 #define TM1638_BRT0 0x00 //Pulsewidth 1/16 00101 #define TM1638_BRT1 0x01 00102 #define TM1638_BRT2 0x02 00103 #define TM1638_BRT3 0x03 00104 #define TM1638_BRT4 0x04 00105 #define TM1638_BRT5 0x05 00106 #define TM1638_BRT6 0x06 00107 #define TM1638_BRT7 0x07 //Pulsewidth 14/16 00108 00109 #define TM1638_BRT_DEF TM1638_BRT3 00110 00111 #define TM1638_DSP_OFF 0x00 00112 #define TM1638_DSP_ON 0x08 00113 00114 00115 /** A class for driving TM1638 LED controller 00116 * 00117 * @brief Supports 8 Grids @ 10 Segments. 00118 * Also supports a scanned keyboard of upto 24 keys. 00119 * SPI bus interface device. 00120 */ 00121 class TM1638 { 00122 public: 00123 /** Datatype for display data */ 00124 typedef char DisplayData_t[TM1638_DISPLAY_MEM]; 00125 00126 /** Datatypes for keymatrix data */ 00127 typedef char KeyData_t[TM1638_KEY_MEM]; 00128 00129 /** Constructor for class for driving TM1638 LED controller 00130 * 00131 * @brief Supports 8 Grids @ 10 segments. 00132 * Also supports a scanned keyboard of upto 24 keys. 00133 * SPI bus interface device. 00134 * 00135 * @param PinName mosi, miso, sclk, cs SPI bus pins 00136 */ 00137 TM1638(PinName mosi, PinName miso, PinName sclk, PinName cs); 00138 00139 /** Clear the screen and locate to 0 00140 */ 00141 void cls(); 00142 00143 /** Write databyte to TM1638 00144 * @param char data byte written at given address 00145 * @param int address display memory location to write byte 00146 * @return none 00147 */ 00148 void writeData(char data, int address); 00149 00150 /** Write Display datablock to TM1638 00151 * @param DisplayData_t data Array of TM1638_DISPLAY_MEM (=16) bytes for displaydata 00152 * @param length number bytes to write (valid range 0..(TM1638_MAX_NR_GRIDS * TM1638_BYTES_PER_GRID) (=16), when starting at address 0) 00153 * @param int address display memory location to write bytes (default = 0) 00154 * @return none 00155 */ 00156 void writeData(DisplayData_t data, int length = (TM1638_MAX_NR_GRIDS * TM1638_BYTES_PER_GRID), int address = 0); 00157 00158 /** Read keydata block from TM1638 00159 * @param *keydata Ptr to Array of TM1638_KEY_MEM (=4) bytes for keydata 00160 * @return bool keypress True when at least one key was pressed 00161 * 00162 * Note: Due to the hardware configuration the TM1638 key matrix scanner will detect multiple keys pressed at same time, 00163 * but this may result in some spurious keys also being set in keypress data array. 00164 * It may be best to ignore all keys in those situations. That option is implemented in this method depending on #define setting. 00165 */ 00166 bool getKeys(KeyData_t *keydata); 00167 00168 /** Set Brightness 00169 * 00170 * @param char brightness (3 significant bits, valid range 0..7 (1/16 .. 14/16 dutycycle) 00171 * @return none 00172 */ 00173 void setBrightness(char brightness = TM1638_BRT_DEF); 00174 00175 /** Set the Display mode On/off 00176 * 00177 * @param bool display mode 00178 */ 00179 void setDisplay(bool on); 00180 00181 private: 00182 SPI _spi; 00183 DigitalOut _cs; 00184 char _display; 00185 char _bright; 00186 00187 /** Init the SPI interface and the controller 00188 * @param none 00189 * @return none 00190 */ 00191 void _init(); 00192 00193 /** Helper to reverse all command or databits. The TM1638 expects LSB first, whereas SPI is MSB first 00194 * @param char data 00195 * @return bitreversed data 00196 */ 00197 char _flip(char data); 00198 00199 /** Write command and parameter to TM1638 00200 * @param int cmd Command byte 00201 * &Param int data Parameters for command 00202 * @return none 00203 */ 00204 void _writeCmd(int cmd, int data); 00205 }; 00206 00207 00208 #if (LEDKEY8_TEST == 1) 00209 // Derived class for TM1638 used in LED&KEY display unit 00210 // 00211 00212 #include "Font_7Seg.h" 00213 00214 #define LEDKEY8_NR_GRIDS 8 00215 #define LEDKEY8_NR_DIGITS 8 00216 #define LEDKEY8_NR_UDC 8 00217 00218 //Access to 8 Switches 00219 #define LEDKEY8_SW1_IDX 0 00220 #define LEDKEY8_SW1_BIT 0x01 00221 #define LEDKEY8_SW2_IDX 1 00222 #define LEDKEY8_SW2_BIT 0x01 00223 #define LEDKEY8_SW3_IDX 2 00224 #define LEDKEY8_SW3_BIT 0x01 00225 #define LEDKEY8_SW4_IDX 3 00226 #define LEDKEY8_SW4_BIT 0x01 00227 00228 #define LEDKEY8_SW5_IDX 0 00229 #define LEDKEY8_SW5_BIT 0x10 00230 #define LEDKEY8_SW6_IDX 1 00231 #define LEDKEY8_SW6_BIT 0x10 00232 #define LEDKEY8_SW7_IDX 2 00233 #define LEDKEY8_SW7_BIT 0x10 00234 #define LEDKEY8_SW8_IDX 3 00235 #define LEDKEY8_SW8_BIT 0x10 00236 00237 /** Constructor for class for driving TM1638 controller as used in LEDKEY8 00238 * 00239 * @brief Supports 8 Digits of 7 Segments + DP + LED Icons, Also supports a scanned keyboard of 8 keys. 00240 * 00241 * @param PinName mosi, miso, sclk, cs SPI bus pins 00242 */ 00243 class TM1638_LEDKEY8 : public TM1638, public Stream { 00244 public: 00245 00246 /** Enums for Icons */ 00247 // Grid encoded in 8 MSBs, Icon pattern encoded in 16 LSBs 00248 enum Icon { 00249 LD1 = (1<<24) | S7_LD1, /**< LED1 */ 00250 LD2 = (2<<24) | S7_LD2, /**< LED2 */ 00251 LD3 = (3<<24) | S7_LD3, /**< LED3 */ 00252 LD4 = (4<<24) | S7_LD4, /**< LED4 */ 00253 LD5 = (5<<24) | S7_LD5, /**< LED5 */ 00254 LD6 = (6<<24) | S7_LD6, /**< LED6 */ 00255 LD7 = (7<<24) | S7_LD7, /**< LED7 */ 00256 LD8 = (8<<24) | S7_LD8, /**< LED8 */ 00257 00258 DP1 = (1<<24) | S7_DP1, /**< Decimal Point 1 */ 00259 DP2 = (2<<24) | S7_DP2, /**< Decimal Point 2 */ 00260 DP3 = (3<<24) | S7_DP3, /**< Decimal Point 3 */ 00261 DP4 = (4<<24) | S7_DP4, /**< Decimal Point 4 */ 00262 DP5 = (5<<24) | S7_DP5, /**< Decimal Point 5 */ 00263 DP6 = (6<<24) | S7_DP6, /**< Decimal Point 6 */ 00264 DP7 = (7<<24) | S7_DP7, /**< Decimal Point 7 */ 00265 DP8 = (8<<24) | S7_DP8 /**< Decimal Point 8 */ 00266 }; 00267 00268 typedef char UDCData_t[LEDKEY8_NR_UDC]; 00269 00270 /** Constructor for class for driving TM1638 LED controller as used in LEDKEY8 00271 * 00272 * @brief Supports 8 Digits of 7 Segments + DP + LED Icons. Also supports a scanned keyboard of 8 keys. 00273 * 00274 * @param PinName mosi, miso, sclk, cs SPI bus pins 00275 */ 00276 TM1638_LEDKEY8(PinName mosi, PinName miso, PinName sclk, PinName cs); 00277 00278 #if DOXYGEN_ONLY 00279 /** Write a character to the Display 00280 * 00281 * @param c The character to write to the display 00282 */ 00283 int putc(int c); 00284 00285 /** Write a formatted string to the Display 00286 * 00287 * @param format A printf-style format string, followed by the 00288 * variables to use in formatting the string. 00289 */ 00290 int printf(const char* format, ...); 00291 #endif 00292 00293 /** Locate cursor to a screen column 00294 * 00295 * @param column The horizontal position from the left, indexed from 0 00296 */ 00297 void locate(int column); 00298 00299 /** Clear the screen and locate to 0 00300 * @param bool clrAll Clear Icons also (default = false) 00301 */ 00302 void cls(bool clrAll = false); 00303 00304 /** Set Icon 00305 * 00306 * @param Icon icon Enums Icon has Grid position encoded in 8 MSBs, Icon pattern encoded in 16 LSBs 00307 * @return none 00308 */ 00309 void setIcon(Icon icon); 00310 00311 /** Clr Icon 00312 * 00313 * @param Icon icon Enums Icon has Grid position encoded in 8 MSBs, Icon pattern encoded in 16 LSBs 00314 * @return none 00315 */ 00316 void clrIcon(Icon icon); 00317 00318 /** Set User Defined Characters (UDC) 00319 * 00320 * @param unsigned char udc_idx The Index of the UDC (0..7) 00321 * @param int udc_data The bitpattern for the UDC (16 bits) 00322 */ 00323 void setUDC(unsigned char udc_idx, int udc_data); 00324 00325 00326 /** Number of screen columns 00327 * 00328 * @param none 00329 * @return columns 00330 */ 00331 int columns(); 00332 00333 /** Write databyte to TM1638 00334 * @param char data byte written at given address 00335 * @param int address display memory location to write byte 00336 * @return none 00337 */ 00338 void writeData(char data, int address){ 00339 TM1638::writeData(data, address); 00340 } 00341 00342 /** Write Display datablock to TM1638 00343 * @param DisplayData_t data Array of TM1638_DISPLAY_MEM (=16) bytes for displaydata 00344 * @param length number bytes to write (valid range 0..(LEDKEY8_NR_GRIDS * TM1638_BYTES_PER_GRID) (=16), when starting at address 0) 00345 * @param int address display memory location to write bytes (default = 0) 00346 * @return none 00347 */ 00348 void writeData(DisplayData_t data, int length = (LEDKEY8_NR_GRIDS * TM1638_BYTES_PER_GRID), int address = 0) { 00349 TM1638::writeData(data, length, address); 00350 } 00351 00352 protected: 00353 // Stream implementation functions 00354 virtual int _putc(int value); 00355 virtual int _getc(); 00356 00357 private: 00358 int _column; 00359 int _columns; 00360 00361 DisplayData_t _displaybuffer; 00362 UDCData_t _UDC_7S; 00363 }; 00364 #endif 00365 00366 00367 #if (QYF_TEST == 1) 00368 // Derived class for TM1638 used in QYF-TM1638 display unit 00369 // 00370 00371 #include "Font_7Seg.h" 00372 00373 #define QYF_NR_GRIDS 8 00374 #define QYF_NR_DIGITS 8 00375 #define QYF_NR_UDC 8 00376 00377 //Access to 16 Switches 00378 #define QYF_SW1_IDX 0 00379 #define QYF_SW1_BIT 0x04 00380 #define QYF_SW2_IDX 0 00381 #define QYF_SW2_BIT 0x40 00382 #define QYF_SW3_IDX 1 00383 #define QYF_SW3_BIT 0x04 00384 #define QYF_SW4_IDX 1 00385 #define QYF_SW4_BIT 0x40 00386 00387 #define QYF_SW5_IDX 2 00388 #define QYF_SW5_BIT 0x04 00389 #define QYF_SW6_IDX 2 00390 #define QYF_SW6_BIT 0x40 00391 #define QYF_SW7_IDX 3 00392 #define QYF_SW7_BIT 0x04 00393 #define QYF_SW8_IDX 3 00394 #define QYF_SW8_BIT 0x40 00395 00396 #define QYF_SW9_IDX 0 00397 #define QYF_SW9_BIT 0x02 00398 #define QYF_SW10_IDX 0 00399 #define QYF_SW10_BIT 0x20 00400 #define QYF_SW11_IDX 1 00401 #define QYF_SW11_BIT 0x02 00402 #define QYF_SW12_IDX 1 00403 #define QYF_SW12_BIT 0x20 00404 00405 #define QYF_SW13_IDX 2 00406 #define QYF_SW13_BIT 0x02 00407 #define QYF_SW14_IDX 2 00408 #define QYF_SW14_BIT 0x20 00409 #define QYF_SW15_IDX 3 00410 #define QYF_SW15_BIT 0x02 00411 #define QYF_SW16_IDX 3 00412 #define QYF_SW16_BIT 0x20 00413 00414 /** Constructor for class for driving TM1638 controller as used in QYF 00415 * 00416 * @brief Supports 8 Digits of 7 Segments + DP, Also supports a scanned keyboard of 16 keys. 00417 * 00418 * @param PinName mosi, miso, sclk, cs SPI bus pins 00419 */ 00420 class TM1638_QYF : public TM1638, public Stream { 00421 public: 00422 00423 /** Enums for Icons */ 00424 // Grid encoded in 8 MSBs, Icon pattern encoded in 16 LSBs 00425 enum Icon { 00426 DP1 = (8<<24) | S7_DP1, /**< Decimal Point 1 */ 00427 DP2 = (8<<24) | S7_DP2, /**< Decimal Point 2 */ 00428 DP3 = (8<<24) | S7_DP3, /**< Decimal Point 3 */ 00429 DP4 = (8<<24) | S7_DP4, /**< Decimal Point 4 */ 00430 DP5 = (8<<24) | S7_DP5, /**< Decimal Point 5 */ 00431 DP6 = (8<<24) | S7_DP6, /**< Decimal Point 6 */ 00432 DP7 = (8<<24) | S7_DP7, /**< Decimal Point 7 */ 00433 DP8 = (8<<24) | S7_DP8 /**< Decimal Point 8 */ 00434 }; 00435 00436 typedef char UDCData_t[QYF_NR_UDC]; 00437 00438 /** Constructor for class for driving TM1638 LED controller as used in QYF 00439 * 00440 * @brief Supports 8 Digits of 7 Segments + DP Icons. Also supports a scanned keyboard of 16 keys. 00441 * 00442 * @param PinName mosi, miso, sclk, cs SPI bus pins 00443 */ 00444 TM1638_QYF(PinName mosi, PinName miso, PinName sclk, PinName cs); 00445 00446 #if DOXYGEN_ONLY 00447 /** Write a character to the Display 00448 * 00449 * @param c The character to write to the display 00450 */ 00451 int putc(int c); 00452 00453 /** Write a formatted string to the Display 00454 * 00455 * @param format A printf-style format string, followed by the 00456 * variables to use in formatting the string. 00457 */ 00458 int printf(const char* format, ...); 00459 #endif 00460 00461 /** Locate cursor to a screen column 00462 * 00463 * @param column The horizontal position from the left, indexed from 0 00464 */ 00465 void locate(int column); 00466 00467 /** Clear the screen and locate to 0 00468 * @param bool clrAll Clear Icons also (default = false) 00469 */ 00470 void cls(bool clrAll = false); 00471 00472 /** Set Icon 00473 * 00474 * @param Icon icon Enums Icon has Grid position encoded in 8 MSBs, Icon pattern encoded in 16 LSBs 00475 * @return none 00476 */ 00477 void setIcon(Icon icon); 00478 00479 /** Clr Icon 00480 * 00481 * @param Icon icon Enums Icon has Grid position encoded in 8 MSBs, Icon pattern encoded in 16 LSBs 00482 * @return none 00483 */ 00484 void clrIcon(Icon icon); 00485 00486 /** Set User Defined Characters (UDC) 00487 * 00488 * @param unsigned char udc_idx The Index of the UDC (0..7) 00489 * @param int udc_data The bitpattern for the UDC (16 bits) 00490 */ 00491 void setUDC(unsigned char udc_idx, int udc_data); 00492 00493 00494 /** Number of screen columns 00495 * 00496 * @param none 00497 * @return columns 00498 */ 00499 int columns(); 00500 00501 /** Write databyte to TM1638 00502 * @param char data byte written at given address 00503 * @param int address display memory location to write byte 00504 * @return none 00505 */ 00506 void writeData(char data, int address){ 00507 TM1638::writeData(data, address); 00508 } 00509 00510 /** Write Display datablock to TM1638 00511 * @param DisplayData_t data Array of TM1638_DISPLAY_MEM (=16) bytes for displaydata 00512 * @param length number bytes to write (valid range 0..(QYF_NR_GRIDS * TM1638_BYTES_PER_GRID) (=16), when starting at address 0) 00513 * @param int address display memory location to write bytes (default = 0) 00514 * @return none 00515 */ 00516 void writeData(DisplayData_t data, int length = (QYF_NR_GRIDS * TM1638_BYTES_PER_GRID), int address = 0) { 00517 TM1638::writeData(data, length, address); 00518 } 00519 00520 protected: 00521 // Stream implementation functions 00522 virtual int _putc(int value); 00523 virtual int _getc(); 00524 00525 private: 00526 int _column; 00527 int _columns; 00528 00529 DisplayData_t _displaybuffer; 00530 UDCData_t _UDC_7S; 00531 }; 00532 #endif 00533 00534 #if (LKM1638_TEST == 1) 00535 // Derived class for TM1638 used in LKM1638 TM1638 display unit 00536 // 00537 00538 #include "Font_7Seg.h" 00539 00540 #define LKM1638_NR_GRIDS 8 00541 #define LKM1638_NR_DIGITS 8 00542 #define LKM1638_NR_UDC 8 00543 00544 //Access to 8 Switches 00545 #define LKM1638_SW1_IDX 0 00546 #define LKM1638_SW1_BIT 0x01 00547 #define LKM1638_SW2_IDX 1 00548 #define LKM1638_SW2_BIT 0x01 00549 #define LKM1638_SW3_IDX 2 00550 #define LKM1638_SW3_BIT 0x01 00551 #define LKM1638_SW4_IDX 3 00552 #define LKM1638_SW4_BIT 0x01 00553 00554 #define LKM1638_SW5_IDX 0 00555 #define LKM1638_SW5_BIT 0x10 00556 #define LKM1638_SW6_IDX 1 00557 #define LKM1638_SW6_BIT 0x10 00558 #define LKM1638_SW7_IDX 2 00559 #define LKM1638_SW7_BIT 0x10 00560 #define LKM1638_SW8_IDX 3 00561 #define LKM1638_SW8_BIT 0x10 00562 00563 /** Constructor for class for driving TM1638 controller as used in LKM1638 00564 * 00565 * @brief Supports 8 Digits of 7 Segments + DP, Also supports 8 Bi-color LEDs and a scanned keyboard of 8 keys. 00566 * 00567 * @param PinName mosi, miso, sclk, cs SPI bus pins 00568 */ 00569 class TM1638_LKM1638 : public TM1638, public Stream { 00570 public: 00571 00572 /** Enums for Icons */ 00573 // Grid encoded in 8 MSBs, Icon pattern encoded in 16 LSBs 00574 enum Icon { 00575 DP1 = (1<<24) | S7_DP1, /**< Decimal Point 1 */ 00576 DP2 = (2<<24) | S7_DP2, /**< Decimal Point 2 */ 00577 DP3 = (3<<24) | S7_DP3, /**< Decimal Point 3 */ 00578 DP4 = (4<<24) | S7_DP4, /**< Decimal Point 4 */ 00579 DP5 = (5<<24) | S7_DP5, /**< Decimal Point 5 */ 00580 DP6 = (6<<24) | S7_DP6, /**< Decimal Point 6 */ 00581 DP7 = (7<<24) | S7_DP7, /**< Decimal Point 7 */ 00582 DP8 = (8<<24) | S7_DP8, /**< Decimal Point 8 */ 00583 00584 GR1 = (1<<24) | S7_GR1, /**< Green LED 1 */ 00585 GR2 = (2<<24) | S7_GR2, /**< Green LED 2 */ 00586 GR3 = (3<<24) | S7_GR3, /**< Green LED 3 */ 00587 GR4 = (4<<24) | S7_GR4, /**< Green LED 4 */ 00588 GR5 = (5<<24) | S7_GR5, /**< Green LED 5 */ 00589 GR6 = (6<<24) | S7_GR6, /**< Green LED 6 */ 00590 GR7 = (7<<24) | S7_GR7, /**< Green LED 7 */ 00591 GR8 = (8<<24) | S7_GR8, /**< Green LED 8 */ 00592 00593 RD1 = (1<<24) | S7_RD1, /**< Red LED 1 */ 00594 RD2 = (2<<24) | S7_RD2, /**< Red LED 2 */ 00595 RD3 = (3<<24) | S7_RD3, /**< Red LED 3 */ 00596 RD4 = (4<<24) | S7_RD4, /**< Red LED 4 */ 00597 RD5 = (5<<24) | S7_RD5, /**< Red LED 5 */ 00598 RD6 = (6<<24) | S7_RD6, /**< Red LED 6 */ 00599 RD7 = (7<<24) | S7_RD7, /**< Red LED 7 */ 00600 RD8 = (8<<24) | S7_RD8, /**< Red LED 8 */ 00601 00602 YL1 = (1<<24) | S7_YL1, /**< Yellow LED 1 */ 00603 YL2 = (2<<24) | S7_YL2, /**< Yellow LED 2 */ 00604 YL3 = (3<<24) | S7_YL3, /**< Yellow LED 3 */ 00605 YL4 = (4<<24) | S7_YL4, /**< Yellow LED 4 */ 00606 YL5 = (5<<24) | S7_YL5, /**< Yellow LED 5 */ 00607 YL6 = (6<<24) | S7_YL6, /**< Yellow LED 6 */ 00608 YL7 = (7<<24) | S7_YL7, /**< Yellow LED 7 */ 00609 YL8 = (8<<24) | S7_YL8 /**< Yellow LED 8 */ 00610 }; 00611 00612 typedef char UDCData_t[LKM1638_NR_UDC]; 00613 00614 /** Constructor for class for driving TM1638 LED controller as used in LKM1638 00615 * 00616 * @brief Supports 8 Digits of 7 Segments + DP Icons. Also supports 8 Bi-Color LEDs and a scanned keyboard of 8 keys. 00617 * 00618 * @param PinName mosi, miso, sclk, cs SPI bus pins 00619 */ 00620 TM1638_LKM1638(PinName mosi, PinName miso, PinName sclk, PinName cs); 00621 00622 #if DOXYGEN_ONLY 00623 /** Write a character to the Display 00624 * 00625 * @param c The character to write to the display 00626 */ 00627 int putc(int c); 00628 00629 /** Write a formatted string to the Display 00630 * 00631 * @param format A printf-style format string, followed by the 00632 * variables to use in formatting the string. 00633 */ 00634 int printf(const char* format, ...); 00635 #endif 00636 00637 /** Locate cursor to a screen column 00638 * 00639 * @param column The horizontal position from the left, indexed from 0 00640 */ 00641 void locate(int column); 00642 00643 /** Clear the screen and locate to 0 00644 * @param bool clrAll Clear Icons also (default = false) 00645 */ 00646 void cls(bool clrAll = false); 00647 00648 /** Set Icon 00649 * 00650 * @param Icon icon Enums Icon has Grid position encoded in 8 MSBs, Icon pattern encoded in 16 LSBs 00651 * @return none 00652 */ 00653 void setIcon(Icon icon); 00654 00655 /** Clr Icon 00656 * 00657 * @param Icon icon Enums Icon has Grid position encoded in 8 MSBs, Icon pattern encoded in 16 LSBs 00658 * @return none 00659 */ 00660 void clrIcon(Icon icon); 00661 00662 /** Set User Defined Characters (UDC) 00663 * 00664 * @param unsigned char udc_idx The Index of the UDC (0..7) 00665 * @param int udc_data The bitpattern for the UDC (16 bits) 00666 */ 00667 void setUDC(unsigned char udc_idx, int udc_data); 00668 00669 00670 /** Number of screen columns 00671 * 00672 * @param none 00673 * @return columns 00674 */ 00675 int columns(); 00676 00677 /** Write databyte to TM1638 00678 * @param char data byte written at given address 00679 * @param int address display memory location to write byte 00680 * @return none 00681 */ 00682 void writeData(char data, int address){ 00683 TM1638::writeData(data, address); 00684 } 00685 00686 /** Write Display datablock to TM1638 00687 * @param DisplayData_t data Array of TM1638_DISPLAY_MEM (=16) bytes for displaydata 00688 * @param length number bytes to write (valid range 0..(LKM1638_NR_GRIDS * TM1638_BYTES_PER_GRID) (=16), when starting at address 0) 00689 * @param int address display memory location to write bytes (default = 0) 00690 * @return none 00691 */ 00692 void writeData(DisplayData_t data, int length = (LKM1638_NR_GRIDS * TM1638_BYTES_PER_GRID), int address = 0) { 00693 TM1638::writeData(data, length, address); 00694 } 00695 00696 protected: 00697 // Stream implementation functions 00698 virtual int _putc(int value); 00699 virtual int _getc(); 00700 00701 private: 00702 int _column; 00703 int _columns; 00704 00705 DisplayData_t _displaybuffer; 00706 UDCData_t _UDC_7S; 00707 }; 00708 #endif 00709 00710 #endif
Generated on Tue Jul 12 2022 18:47:06 by
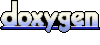