Library for multi-pin RGB leds that encapsulates three PwmOut objects.
MultipinRGB Class Reference
MutlipinRGB Library. More...
#include <MultipinRGB.h>
Public Member Functions | |
MultipinRGB (PinName red, PinName green, PinName blue, LedLogic_e activeState=ActiveLow) | |
Constructor. | |
~MultipinRGB () | |
Destructor. | |
void | writeLed (const Led_e led, const float dc) |
Sets duty cycle for led. | |
void | writeLeds (const float r, const float g, const float b) |
Sets duty cycle for all three leds. | |
float | readLed (const Led_e led) |
Reads duty cycle for led. | |
void | readLeds (float &r, float &g, float &b) |
Reads duty cycle for all three leds. | |
void | toggleLed (const Led_e led) |
Toggles led from off to on, or on to off. | |
void | setPeriod (const float p) |
Sets pwm period for all three leds. |
Detailed Description
MutlipinRGB Library.
Library for multi-pin RGB leds that encapsulates three PwmOut objects and provides access to the floating point read/write mbr fxs of the PwmOut objects.
Duty cycles should always be written as 0.0F for off, and 1.0F as 100% on, regardless of led active state.
Duty cycles are reported in the same manner.
#include "mbed.h" #include "MultipinRGB.h" int main () { MultipinRGB leds(LED1, LED2, LED3); float redDutyCycle(0.5F), grnDutyCycle(0.0F), bluDutyCycle(0.0F), temp; while(1) { leds.writeLeds(redDutyCycle, grnDutyCycle, bluDutyCycle); printf("RGB Duty Cycles = %3.1f, %3.1f, %3.1f\r\n", redDutyCycle, grnDutyCycle, bluDutyCycle); //shift r->g->b->r temp = bluDutyCycle; bluDutyCycle = grnDutyCycle; grnDutyCycle = redDutyCycle; redDutyCycle = temp; wait(0.25); } }
Definition at line 70 of file MultipinRGB.h.
Constructor & Destructor Documentation
MultipinRGB | ( | PinName | red, |
PinName | green, | ||
PinName | blue, | ||
LedLogic_e | activeState = ActiveLow |
||
) |
Constructor.
- Parameters:
-
[in] red - Pin that red led is connected to. [in] green - Pin that green led is connected to. [in] blue - Pin that blue led is connected to. [in] activeState - Active state of all three leds.
Definition at line 30 of file MultipinRGB.cpp.
~MultipinRGB | ( | ) |
Destructor.
Definition at line 52 of file MultipinRGB.cpp.
Member Function Documentation
float readLed | ( | const Led_e | led ) |
Reads duty cycle for led.
On Entry:
- Parameters:
-
[in] led - Led to update
On Exit:
- Parameters:
-
[out] none
- Returns:
- Current duty cycle for led, 0.0 to 1.0
Definition at line 107 of file MultipinRGB.cpp.
void readLeds | ( | float & | r, |
float & | g, | ||
float & | b | ||
) |
Reads duty cycle for all three leds.
On Entry:
- Parameters:
-
[in] r - float reference for red led duty cycle. [in] g - float reference for green led duty cycle. [in] b - float reference for blue led duty cycle.
On Exit:
- Parameters:
-
[out] r - Current duty cycle for led, 0.0 to 1.0 [out] g - Current duty cycle for led, 0.0 to 1.0 [out] b - Current duty cycle for led, 0.0 to 1.0
- Returns:
- none
Definition at line 140 of file MultipinRGB.cpp.
void setPeriod | ( | const float | p ) |
Sets pwm period for all three leds.
On Entry:
- Parameters:
-
[in] p - PWM period in seconds
On Exit:
- Parameters:
-
[out] none
- Returns:
- none
Definition at line 216 of file MultipinRGB.cpp.
void toggleLed | ( | const Led_e | led ) |
Toggles led from off to on, or on to off.
Duty Cycle will be 0% or 100% after this call.
On Entry:
- Parameters:
-
[in] led - Led to toggle
On Exit:
- Parameters:
-
[out] none
- Returns:
- none
Definition at line 158 of file MultipinRGB.cpp.
void writeLed | ( | const Led_e | led, |
const float | dc | ||
) |
Sets duty cycle for led.
On Entry:
- Parameters:
-
[in] led - Led to update [in] dc - Duty cycle for led, 0.0 to 1.0
On Exit:
- Parameters:
-
[out] none
- Returns:
- none
Definition at line 59 of file MultipinRGB.cpp.
void writeLeds | ( | const float | r, |
const float | g, | ||
const float | b | ||
) |
Sets duty cycle for all three leds.
On Entry:
- Parameters:
-
[in] r - Duty cycle for led, 0.0 to 1.0 [in] g - Duty cycle for led, 0.0 to 1.0 [in] b - Duty cycle for led, 0.0 to 1.0
On Exit:
- Parameters:
-
[out] none
- Returns:
- none
Definition at line 89 of file MultipinRGB.cpp.
Generated on Sun Jul 17 2022 02:57:35 by
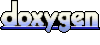