Library for multi-pin RGB leds that encapsulates three PwmOut objects.
Embed:
(wiki syntax)
Show/hide line numbers
MultipinRGB.cpp
00001 /****************************************************************************** 00002 * MIT License 00003 * 00004 * Copyright (c) 2017 Justin J. Jordan 00005 * 00006 * Permission is hereby granted, free of charge, to any person obtaining a copy 00007 * of this software and associated documentation files (the "Software"), to deal 00008 * in the Software without restriction, including without limitation the rights 00009 * to use, copy, modify, merge, publish, distribute, sublicense, and/or sell 00010 * copies of the Software, and to permit persons to whom the Software is 00011 * furnished to do so, subject to the following conditions: 00012 00013 * The above copyright notice and this permission notice shall be included in all 00014 * copies or substantial portions of the Software. 00015 00016 * THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00017 * IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00018 * FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL THE 00019 * AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00020 * LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING FROM, 00021 * OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER DEALINGS IN THE 00022 * SOFTWARE. 00023 ******************************************************************************/ 00024 00025 00026 #include "MultipinRGB.h" 00027 00028 00029 /*****************************************************************************/ 00030 MultipinRGB::MultipinRGB(PinName red, PinName green, PinName blue, LedLogic_e activeState): 00031 m_red(red), m_green(green), m_blue(blue), m_ledActiveState(activeState) 00032 { 00033 //turn off all leds, use default period 00034 if(m_ledActiveState == MultipinRGB::ActiveLow) 00035 { 00036 m_red.write(1.0F); 00037 m_green.write(1.0F); 00038 m_blue.write(1.0F); 00039 } 00040 else 00041 { 00042 m_red.write(0.0F); 00043 m_green.write(0.0F); 00044 m_blue.write(0.0F); 00045 } 00046 00047 00048 } 00049 00050 00051 /*****************************************************************************/ 00052 MultipinRGB::~MultipinRGB() 00053 { 00054 //empty block 00055 } 00056 00057 00058 /*****************************************************************************/ 00059 void MultipinRGB::writeLed(const Led_e led, const float dc) 00060 { 00061 switch(led) 00062 { 00063 case(Red): 00064 //if 00065 (m_ledActiveState == MultipinRGB::ActiveLow) ? 00066 m_red.write(1.0F - dc) : m_red.write(dc); 00067 break; 00068 00069 case(Green): 00070 //if 00071 (m_ledActiveState == MultipinRGB::ActiveLow) ? 00072 m_green.write(1.0F - dc) : m_green.write(dc); 00073 break; 00074 00075 case(Blue): 00076 //if 00077 (m_ledActiveState == MultipinRGB::ActiveLow) ? 00078 m_blue.write(1.0F - dc) : m_blue.write(dc); 00079 break; 00080 00081 default: 00082 mbed_die(); 00083 break; 00084 }; 00085 } 00086 00087 00088 /*****************************************************************************/ 00089 void MultipinRGB::writeLeds(const float r, const float g, const float b) 00090 { 00091 if(m_ledActiveState == MultipinRGB::ActiveLow) 00092 { 00093 m_red.write(1.0F - r); 00094 m_green.write(1.0F - g); 00095 m_blue.write(1.0F - b); 00096 } 00097 else 00098 { 00099 m_red.write(r); 00100 m_green.write(g); 00101 m_blue.write(b); 00102 } 00103 } 00104 00105 00106 /*****************************************************************************/ 00107 float MultipinRGB::readLed(const Led_e led) 00108 { 00109 float rtnVal; 00110 switch(led) 00111 { 00112 case(Red): 00113 //if 00114 (m_ledActiveState == MultipinRGB::ActiveLow) ? 00115 (rtnVal = (1.0F - m_red.read())) : (rtnVal = m_red.read()); 00116 break; 00117 00118 case(Green): 00119 //if 00120 (m_ledActiveState == MultipinRGB::ActiveLow) ? 00121 (rtnVal = (1.0F - m_green.read())) : (rtnVal = m_green.read()); 00122 break; 00123 00124 case(Blue): 00125 //if 00126 (m_ledActiveState == MultipinRGB::ActiveLow) ? 00127 (rtnVal = (1.0F - m_blue.read())) : (rtnVal = m_blue.read()); 00128 break; 00129 00130 default: 00131 mbed_die(); 00132 break; 00133 }; 00134 00135 return rtnVal; 00136 } 00137 00138 00139 /*****************************************************************************/ 00140 void MultipinRGB::readLeds(float& r, float& g, float& b) 00141 { 00142 if(m_ledActiveState == MultipinRGB::ActiveLow) 00143 { 00144 r = (1.0F - m_red.read()); 00145 g = (1.0F - m_green.read()); 00146 b = (1.0F - m_blue.read()); 00147 } 00148 else 00149 { 00150 r = m_red.read(); 00151 g = m_green.read(); 00152 b = m_blue.read(); 00153 } 00154 } 00155 00156 00157 /*****************************************************************************/ 00158 void MultipinRGB::toggleLed(const Led_e led) 00159 { 00160 float currentDc, newDc; 00161 00162 switch(led) 00163 { 00164 case(Red): 00165 currentDc = m_red.read(); 00166 break; 00167 00168 case(Green): 00169 currentDc = m_green.read(); 00170 break; 00171 00172 case(Blue): 00173 currentDc = m_blue.read(); 00174 break; 00175 00176 default: 00177 mbed_die(); 00178 break; 00179 }; 00180 00181 if(currentDc >= 1.0F) 00182 { 00183 newDc = 0.0F; 00184 } 00185 else if(currentDc <= 0.0F) 00186 { 00187 newDc = 1.0F; 00188 } 00189 else 00190 { 00191 newDc = (m_ledActiveState == MultipinRGB::ActiveLow) ? 1.0F : 0.0F; 00192 } 00193 00194 switch(led) 00195 { 00196 case(Red): 00197 m_red.write(newDc); 00198 break; 00199 00200 case(Green): 00201 m_green.write(newDc); 00202 break; 00203 00204 case(Blue): 00205 m_blue.write(newDc); 00206 break; 00207 00208 default: 00209 mbed_die(); 00210 break; 00211 }; 00212 } 00213 00214 00215 /*****************************************************************************/ 00216 void MultipinRGB::setPeriod(const float p) 00217 { 00218 m_red.period(p); 00219 m_green.period(p); 00220 m_blue.period(p); 00221 }
Generated on Sun Jul 17 2022 02:57:35 by
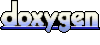