
4180 lab4
Dependencies: 4DGL-uLCD-SE DebounceIn SDFileSystem mbed-rtos mbed wave_player
Fork of WavePlayer_HelloWorld by
map_public.h
00001 #ifndef MAP_PUBLIC_H 00002 #define MAP_PUBLIC_H 00003 00004 /// The enum define the status of a grid on the map 00005 typedef enum { 00006 GRID_BACK, 00007 GRID_SIDELINE, 00008 GRID_NODELINE, 00009 } GRID_STATUS; 00010 00011 /// The structure to store the information of a grid 00012 typedef struct { 00013 int x; ///< The upper-left corner of the grid. It is the x coordinate on the screen. 00014 int y; ///< The upper-left corner of the grid. It is the y coordinate on the screen. 00015 GRID_STATUS status; ///< See enum GRID_STATUS 00016 } GRID; 00017 00018 /** Call map_init() once at the begining of your code 00019 @brief It initialize the map structure and draw the map. 00020 */ 00021 void map_init(void); 00022 00023 /** Remove the candy/big-candy from map 00024 @brief It could be called by Pacman when it eat the cookie. 00025 @param grid_x The horizontal position in the grid. 00026 @param grid_y The vertical position in the grid. 00027 @return 1:There is a candy be eaten. 0:The is no candy at the grid. 00028 */ 00029 //double map_eat_candy(int grid_x, int grid_y); 00030 00031 /** Get the information about the grid 00032 @param grid_x The horizontal position in the grid. 00033 @param grid_y The vertical position in the grid. 00034 @return The data structure of the grid. You could access the contents by using the_grid.x , the_grid.status ... etc. 00035 */ 00036 GRID map_get_grid_status(int grid_x, int grid_y); 00037 00038 /** Draw the grid 00039 @param grid_x The horizontal position in the grid. 00040 @param grid_y The vertical position in the grid. 00041 */ 00042 void map_draw_grid(unsigned grid_x, unsigned grid_y); 00043 00044 /** Get the number of remaining candy. 00045 @brief The game should be ended when there is no candy. 00046 @return The number of remaining cookie. 00047 */ 00048 //int map_remaining_candy(void); 00049 00050 #endif //MAP_H
Generated on Tue Jul 12 2022 16:35:26 by
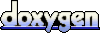