
4180 lab4
Dependencies: 4DGL-uLCD-SE DebounceIn SDFileSystem mbed-rtos mbed wave_player
Fork of WavePlayer_HelloWorld by
main.cpp
00001 00002 #include <mbed.h> 00003 #include <string> 00004 #include <list> 00005 #include "uLCD_4DGL.h" 00006 #include "DebounceIn.h" 00007 #include "wave_player.h" 00008 #include "SDFileSystem.h" 00009 #include "rtos.h" 00010 #include "stdio.h" 00011 #include "map_public.h" 00012 #include "globals.h" 00013 00014 DigitalOut led1(LED1); 00015 DigitalOut led2(LED2); 00016 DigitalOut led3(LED3); 00017 DigitalOut led4(LED4); 00018 00019 DebounceIn push5(p21); 00020 DebounceIn push4(p22); 00021 DebounceIn push3(p23); 00022 00023 DebounceIn push2(p24); 00024 DebounceIn push1(p25); 00025 00026 InterruptIn interrupt(p11); 00027 I2C i2c(p9, p10); 00028 00029 00030 00031 SDFileSystem sd(p5, p6, p7, p8, "sd"); //SD card 00032 00033 AnalogOut DACout(p18); 00034 uLCD_4DGL uLCD(p13,p14,p15); 00035 wave_player waver(&DACout); 00036 00037 Mutex stdio_mutex; 00038 Mutex lcd_mutex; 00039 00040 00041 volatile int check = 0; 00042 volatile int status = 0; 00043 volatile int global; 00044 volatile int point_bar = 4; 00045 volatile int songNum = 1; 00046 00047 00048 int score = 0; 00049 volatile int meter = 15; 00050 int scrollSpeed = 6; 00051 00052 int node1Pressed = 0; 00053 int node2Pressed = 0; 00054 int node3Pressed = 0; 00055 int node4Pressed = 0; 00056 00057 00058 struct gameNode { 00059 int yPos; 00060 int alive; 00061 gameNode *next; 00062 }; 00063 00064 gameNode* node1Root = NULL; 00065 gameNode* node2Root = NULL; 00066 gameNode* node3Root = NULL; 00067 gameNode* node4Root = NULL; 00068 00069 enum gameState{ 00070 begin, 00071 playing, 00072 over 00073 }; 00074 00075 00076 enum node{ 00077 node1, 00078 node2, 00079 node3, 00080 node4, 00081 none, 00082 }; 00083 00084 int nodeToDelete(none); 00085 00086 void deleteRoot (gameNode* &gNode, int nodeNum) { 00087 gameNode* temp = gNode; 00088 00089 switch(nodeNum) { 00090 case 1: 00091 //uLCD.line(4, gNode->yPos-scrollSpeed, 14, gNode->yPos+10-scrollSpeed, BACKGROUND_COLOR); 00092 //uLCD.line(4, gNode->yPos-scrollSpeed, 14, gNode->yPos-10-scrollSpeed, BACKGROUND_COLOR); 00093 if(gNode->yPos>100 && gNode->yPos<120) 00094 { 00095 uLCD.filled_rectangle(37, gNode->yPos-scrollSpeed, 59, gNode->yPos-scrollSpeed+5, YELLOW); 00096 } 00097 else 00098 { 00099 uLCD.filled_rectangle(37, gNode->yPos-scrollSpeed,59,gNode->yPos-scrollSpeed+5,0x1E01AF); 00100 } 00101 break; 00102 00103 case 2: 00104 if(gNode->yPos>100 && gNode->yPos<120) 00105 { 00106 uLCD.filled_rectangle(60, gNode->yPos-scrollSpeed, 82, gNode->yPos-scrollSpeed+5, YELLOW); 00107 } 00108 else 00109 { 00110 uLCD.filled_rectangle(60, gNode->yPos-scrollSpeed,82,gNode->yPos-scrollSpeed+5,0x1E01AF); 00111 } 00112 //uLCD.filled_rectangle(37,gNode->yPos-scrollSpeed,57,gNode->yPos-scrollSpeed+5,BACKGROUND_COLOR); 00113 //uLCD.line(47, gNode->yPos-scrollSpeed-10, 37, gNode->yPos-scrollSpeed, BACKGROUND_COLOR); 00114 //uLCD.line(47, gNode->yPos-scrollSpeed-10, 57, gNode->yPos-scrollSpeed, BACKGROUND_COLOR); 00115 break; 00116 00117 case 3: 00118 if(gNode->yPos>100 && gNode->yPos<120) 00119 { 00120 uLCD.filled_rectangle(83, gNode->yPos-scrollSpeed, 105, gNode->yPos-scrollSpeed+5, YELLOW); 00121 } 00122 else 00123 { 00124 uLCD.filled_rectangle(83, gNode->yPos-scrollSpeed,105,gNode->yPos-scrollSpeed+5,0x1E01AF); 00125 } 00126 //uLCD.filled_rectangle(70,gNode->yPos-scrollSpeed,90,gNode->yPos-scrollSpeed+5,BACKGROUND_COLOR); 00127 //uLCD.line(80, gNode->yPos-scrollSpeed+10, 70, gNode->yPos-scrollSpeed, BACKGROUND_COLOR); 00128 //uLCD.line(80, gNode->yPos-scrollSpeed+10, 90, gNode->yPos-scrollSpeed, BACKGROUND_COLOR); 00129 break; 00130 00131 case 4: 00132 if(gNode->yPos>100 && gNode->yPos<120) 00133 { 00134 uLCD.filled_rectangle(106, gNode->yPos-scrollSpeed, 128, gNode->yPos-scrollSpeed+5, YELLOW); 00135 } 00136 else 00137 { 00138 uLCD.filled_rectangle(106, gNode->yPos-scrollSpeed,128,gNode->yPos-scrollSpeed+5,0x1E01AF); 00139 } 00140 //uLCD.filled_rectangle(104,gNode->yPos-scrollSpeed,124,gNode->yPos-scrollSpeed+5,BACKGROUND_COLOR); 00141 //uLCD.line(124, gNode->yPos-scrollSpeed, 114, gNode->yPos-scrollSpeed+10, BACKGROUND_COLOR); 00142 //uLCD.line(124, gNode->yPos-scrollSpeed, 114, gNode->yPos-scrollSpeed-10, BACKGROUND_COLOR); 00143 break; 00144 } 00145 00146 gNode = gNode->next; 00147 delete temp; 00148 } 00149 00150 00151 00152 void addToEnd (gameNode* &root) { 00153 gameNode * temp = root; 00154 if (root == NULL) 00155 { 00156 root = new gameNode; 00157 root->yPos = 10; 00158 root->alive = 1; 00159 root->next = 0; 00160 } 00161 else { 00162 while (temp->next) 00163 { 00164 temp = temp->next; 00165 } 00166 00167 temp->next = new gameNode; 00168 temp = temp->next; 00169 temp->yPos = 10; 00170 temp->alive = 1; 00171 temp->next = 0; 00172 } 00173 } 00174 00175 00176 void drawnode1gameNode (int y) { 00177 if(y > 100 && y < 120) 00178 { 00179 uLCD.filled_rectangle(37,y-scrollSpeed, 59, y-scrollSpeed+5, YELLOW); 00180 } 00181 else { 00182 uLCD.filled_rectangle(37,y-scrollSpeed,59,y-scrollSpeed+5,0x1E01AF); 00183 } 00184 uLCD.filled_rectangle(37,y,59,y+5,WHITE); 00185 00186 } 00187 00188 void drawnode2gameNode (int y) { 00189 if(y > 100 && y < 120) 00190 { 00191 uLCD.filled_rectangle(60,y-scrollSpeed, 82, y-scrollSpeed+5, YELLOW); 00192 } 00193 else { 00194 uLCD.filled_rectangle(60,y-scrollSpeed,82,y-scrollSpeed+5,0x1E01AF); 00195 } 00196 uLCD.filled_rectangle(60,y,82,y+5,WHITE); 00197 00198 } 00199 00200 void drawnode3gameNode (int y) { 00201 if(y > 100 && y < 120) 00202 { 00203 uLCD.filled_rectangle(83,y-scrollSpeed, 105, y-scrollSpeed+5, YELLOW); 00204 } 00205 else { 00206 uLCD.filled_rectangle(83,y-scrollSpeed,105,y-scrollSpeed+5,0x1E01AF); 00207 } 00208 uLCD.filled_rectangle(83,y,105,y+5,WHITE); 00209 00210 } 00211 00212 void drawnode4gameNode (int y) { 00213 if(y > 100 && y < 120) 00214 { 00215 uLCD.filled_rectangle(106,y-scrollSpeed, 128, y-scrollSpeed+5, YELLOW); 00216 } 00217 else { 00218 uLCD.filled_rectangle(106,y-scrollSpeed,128,y-scrollSpeed+5,0x1E01AF); 00219 } 00220 uLCD.filled_rectangle(106,y,128,y+5,WHITE); 00221 } 00222 00223 00224 00225 void notify(const char* name, int state) { 00226 stdio_mutex.lock(); 00227 printf("%s: %d\n\r", name, state); 00228 stdio_mutex.unlock(); 00229 } 00230 00231 void test_thread(void const *args) { 00232 while (true) { 00233 notify((const char*)args, 0); Thread::wait(1000); 00234 notify((const char*)args, 1); Thread::wait(1000); 00235 } 00236 } 00237 00238 void thread1(void const *args) 00239 { 00240 00241 while(1) 00242 { 00243 if(status == 1) 00244 { 00245 00246 00247 //led1 = 1; 00248 status = 0; 00249 00250 if(songNum == 1) 00251 { 00252 FILE *wave_file; 00253 wave_file=fopen("/sd/TWICE_TT_converted.wav","r"); 00254 waver.play(wave_file); 00255 fclose(wave_file); 00256 meter = 0; 00257 } 00258 00259 else if(songNum == 2) 00260 { 00261 FILE *wave_file; 00262 wave_file=fopen("/sd/Star_Wars_converted.wav","r"); 00263 waver.play(wave_file); 00264 fclose(wave_file); 00265 meter = 0; 00266 } 00267 00268 else 00269 { 00270 FILE *wave_file; 00271 wave_file=fopen("/sd/Terra_converted.wav","r"); 00272 waver.play(wave_file); 00273 fclose(wave_file); 00274 meter = 0; 00275 } 00276 00277 //led2 = 1; 00278 } 00279 } 00280 00281 } 00282 00283 00284 00285 int main() 00286 { 00287 srand(time(NULL)); 00288 push1.mode(PullUp); 00289 push2.mode(PullUp); 00290 push3.mode(PullUp); 00291 push4.mode(PullUp); 00292 push5.mode(PullUp); 00293 00294 int setup = 1; 00295 00296 Thread t1(thread1); 00297 00298 uLCD.baudrate(3000000); 00299 00300 int gameOn=1; 00301 int ready; 00302 int node1Counter; 00303 int node2Counter; 00304 int node3Counter; 00305 int node4Counter; 00306 int deleted=0; 00307 int drawgameNode=1; 00308 Timer t; 00309 float time = 0.0; 00310 gameNode* temp; 00311 node1Counter = rand()%150+50; 00312 node2Counter = rand()%150+50; 00313 node3Counter = rand()%150+50; 00314 node4Counter = rand()%150+50; 00315 scrollSpeed = 6; 00316 00317 00318 00319 while(setup) 00320 { 00321 int GameStart = 0; 00322 int GameOver = 0; 00323 int old_pb = 0; 00324 00325 int new_pb1; 00326 int new_pb2; 00327 int new_pb3; 00328 00329 uLCD.locate(0,0); 00330 uLCD.printf("Rhythm Game"); 00331 uLCD.locate(0,2); 00332 uLCD.printf("Song Select"); 00333 uLCD.locate(3,4); 00334 uLCD.printf("TWICE_TT"); 00335 uLCD.locate(3,5); 00336 uLCD.printf("Star_Wars"); 00337 uLCD.locate(3,6); 00338 uLCD.printf("Terra"); 00339 uLCD.locate(0,4); 00340 uLCD.printf("->"); 00341 00342 int p_bar = 4; 00343 00344 00345 while(!GameOver) 00346 { 00347 new_pb1 = push1; 00348 new_pb2 = push2; 00349 new_pb3 = push3; 00350 00351 //Moving Down 00352 if((new_pb1 ==0)&&(old_pb==1)&&(p_bar!=4)) 00353 { 00354 if(p_bar==5) 00355 { 00356 p_bar = 4; 00357 uLCD.locate(0,p_bar); 00358 uLCD.printf("->"); 00359 uLCD.locate(0,5); 00360 uLCD.printf(" "); 00361 uLCD.locate(0,6); 00362 uLCD.printf(" "); 00363 } 00364 00365 else 00366 { 00367 p_bar = 5; 00368 uLCD.locate(0,p_bar); 00369 uLCD.printf("->"); 00370 uLCD.locate(0,4); 00371 uLCD.printf(" "); 00372 uLCD.locate(0,6); 00373 uLCD.printf(" "); 00374 } 00375 old_pb = new_pb1; 00376 } 00377 //Moving Up 00378 else if((new_pb3 ==0)&&(old_pb==1)&&(p_bar!=6)) 00379 { 00380 if(p_bar==4) 00381 { 00382 p_bar = 5; 00383 uLCD.locate(0,p_bar); 00384 uLCD.printf("->"); 00385 uLCD.locate(0,6); 00386 uLCD.printf(" "); 00387 uLCD.locate(0,4); 00388 uLCD.printf(" "); 00389 } 00390 00391 else 00392 { 00393 p_bar = 6; 00394 uLCD.locate(0,p_bar); 00395 uLCD.printf("->"); 00396 uLCD.locate(0,4); 00397 uLCD.printf(" "); 00398 uLCD.locate(0,5); 00399 uLCD.printf(" "); 00400 } 00401 old_pb = new_pb3; 00402 } 00403 00404 00405 else if((new_pb2 ==0)&&(old_pb==1)) 00406 { 00407 00408 00409 00410 uLCD.locate(0,8); 00411 uLCD.printf("song is selected"); 00412 wait(1); 00413 00414 uLCD.cls(); 00415 00416 if(p_bar == 4) 00417 { 00418 songNum = 1; 00419 status = 1; 00420 00421 uLCD.cls(); 00422 uLCD.locate(0,3); 00423 uLCD.printf("Start TT"); 00424 wait(.3); 00425 GameOver = 1; 00426 uLCD.cls(); 00427 } 00428 00429 else if(p_bar == 5) 00430 { 00431 songNum = 2; 00432 status = 1; 00433 00434 uLCD.cls(); 00435 uLCD.locate(0,3); 00436 uLCD.printf("Start StarWars"); 00437 wait(.3); 00438 GameOver = 1; 00439 uLCD.cls(); 00440 } 00441 00442 else 00443 { 00444 songNum = 3; 00445 status = 1; 00446 00447 uLCD.cls(); 00448 uLCD.locate(0,3); 00449 uLCD.printf("Start Terra"); 00450 wait(.3); 00451 GameOver = 1; 00452 uLCD.cls(); 00453 } 00454 00455 00456 00457 while(gameOn) 00458 { 00459 t.reset(); 00460 t.start(); 00461 drawgameNode = 1; 00462 00463 //map_init(); 00464 uLCD.filled_rectangle(31,0,36,128,0xD9431C); 00465 uLCD.filled_rectangle(37,0,128,120,0x1E01AF); 00466 uLCD.filled_rectangle(37,98,128,120,YELLOW); 00467 if(node1Counter <= 0) 00468 { 00469 addToEnd(node1Root); 00470 node1Counter = rand()%150+50; 00471 drawgameNode = 0; 00472 } 00473 node1Counter -= scrollSpeed; 00474 temp = node1Root;//node1Root = NULL 00475 while(temp!=NULL) 00476 { 00477 drawnode1gameNode(temp->yPos); 00478 temp->yPos += scrollSpeed; 00479 temp = temp->next; 00480 } 00481 if(node1Root != NULL) 00482 { 00483 if(node1Root->yPos >= 120 || nodeToDelete == node1) 00484 { 00485 //meter--; 00486 deleteRoot(node1Root, 1); 00487 deleted = 1; 00488 } 00489 } 00490 00491 if(node2Counter<=0&&drawgameNode) 00492 { 00493 addToEnd(node2Root); 00494 node2Counter = rand()%150+50; 00495 drawgameNode=0; 00496 } 00497 node2Counter -=scrollSpeed;//node2Counter = node2Counter - scrollSpeed 00498 temp = node2Root; 00499 while(temp != NULL) 00500 { 00501 drawnode2gameNode(temp->yPos); 00502 temp->yPos += scrollSpeed; 00503 temp = temp->next; 00504 } 00505 if(node2Root != NULL) 00506 { 00507 if(node2Root->yPos >= 120 || nodeToDelete == node2) 00508 { 00509 //meter--; 00510 deleteRoot(node2Root, 2); 00511 deleted = 1; 00512 } 00513 } 00514 00515 if(node3Counter<=0&&drawgameNode) 00516 { 00517 addToEnd(node3Root); 00518 node3Counter = rand()%150+50; 00519 drawgameNode=0; 00520 } 00521 node3Counter -=scrollSpeed; 00522 temp = node3Root; 00523 while(temp != NULL) 00524 { 00525 drawnode3gameNode(temp->yPos); 00526 temp->yPos += scrollSpeed; 00527 temp = temp->next; 00528 } 00529 00530 if(node3Root != NULL) 00531 { 00532 if(node3Root->yPos >= 120 || nodeToDelete == node3) 00533 { 00534 //meter--; 00535 deleteRoot(node3Root, 3); 00536 deleted = 1; 00537 } 00538 } 00539 00540 if(node4Counter<=0&&drawgameNode) 00541 { 00542 addToEnd(node4Root); 00543 node4Counter = rand()%150+50; 00544 drawgameNode=0; 00545 } 00546 node4Counter -=scrollSpeed; 00547 temp = node4Root; 00548 while(temp != NULL) 00549 { 00550 drawnode4gameNode(temp->yPos); 00551 temp->yPos += scrollSpeed; 00552 temp = temp->next; 00553 } 00554 if(node4Root != NULL) 00555 { 00556 if(node4Root->yPos >= 120 || nodeToDelete == node4) 00557 { 00558 //meter--; 00559 deleteRoot(node4Root, 4); 00560 deleted = 1; 00561 } 00562 } 00563 00564 //interrupt 00565 if(!push2) 00566 {//push button is a Pull-Up, so the initial condition is 1 flippled to 0 00567 node1Pressed = 1; 00568 if(node1Root) 00569 { 00570 if(node1Root->yPos > 90 && node1Root->yPos < 128) 00571 { 00572 score++; 00573 //meter+=2; 00574 } 00575 if(node1Root->yPos > 95) 00576 { 00577 nodeToDelete = node1; 00578 } 00579 } 00580 } 00581 if(!push3) 00582 { 00583 node2Pressed = 1; 00584 if(node2Root) 00585 { 00586 if(node2Root->yPos > 90 && node2Root->yPos < 128) 00587 { 00588 score++; 00589 //meter+=2; 00590 } 00591 if(node2Root->yPos > 95) 00592 { 00593 nodeToDelete = node2; 00594 } 00595 } 00596 } 00597 if(!push4) 00598 { 00599 node3Pressed = 1; 00600 if(node3Root) 00601 { 00602 if(node3Root->yPos > 90 && node3Root->yPos < 128) 00603 { 00604 score++; 00605 //meter+=2; 00606 } 00607 if(node3Root->yPos > 95) 00608 { 00609 nodeToDelete = node3; 00610 } 00611 } 00612 } 00613 if(!push5) 00614 { 00615 node4Pressed = 1; 00616 if(node4Root) 00617 { 00618 if(node4Root->yPos > 90 && node4Root->yPos < 128) 00619 { 00620 score++; 00621 //meter+=2; 00622 } 00623 if(node4Root->yPos > 95) 00624 { 00625 nodeToDelete = node4; 00626 } 00627 } 00628 } 00629 if(!push1) 00630 { 00631 status = 0; 00632 uLCD.cls(); 00633 break; 00634 } 00635 00636 00637 /////interrupt done 00638 t.stop(); 00639 time = .1-t.read(); 00640 if (time < 0) time = 0; 00641 uLCD.locate(0,0); 00642 uLCD.text_width(1); 00643 uLCD.printf("Score"); 00644 uLCD.locate(0,1); 00645 uLCD.printf("%04d",score); 00646 if(meter>15) meter=15; 00647 00648 if(meter==0) 00649 { 00650 while(node1Root) 00651 { 00652 deleteRoot(node1Root,1); 00653 } 00654 while(node2Root) 00655 { 00656 deleteRoot(node2Root,2); 00657 } 00658 while(node3Root) 00659 { 00660 deleteRoot(node3Root,3); 00661 } 00662 while(node4Root) 00663 { 00664 deleteRoot(node4Root,4); 00665 } 00666 uLCD.cls(); 00667 uLCD.locate(5,8); 00668 uLCD.text_mode(OPAQUE); 00669 uLCD.printf("GAME OVER"); 00670 uLCD.locate(2,9); 00671 uLCD.printf("your score: %d", score); 00672 meter=15; 00673 score=0; 00674 wait(2); 00675 break; 00676 } 00677 00678 00679 //uLCD.locate(0,2); 00680 //uLCD.set_font_size(4,4); 00681 //uLCD.printf("Life"); 00682 //uLCD.locate(0,3); 00683 //uLCD.printf("%2d",meter); 00684 00685 if (deleted) 00686 { 00687 nodeToDelete = none; 00688 deleted = 0; 00689 } 00690 00691 //wait(time); 00692 00693 //uLCD.cls(); 00694 old_pb = new_pb2; 00695 00696 }//GameOn over 00697 00698 00699 } 00700 else 00701 { 00702 uLCD.locate(0,9); 00703 //uLCD.printf("else else"); 00704 old_pb = new_pb1; 00705 } 00706 } 00707 00708 00709 } 00710 00711 }
Generated on Tue Jul 12 2022 16:35:26 by
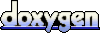