Fork of Adam Green's library with .cpp fix for current compiler
Dependents: MSCUsbHost mpod_nhk_english mpod_picasa_photoframe mpod_nhk_english_spxml ... more
Fork of FatFileSystem by
FATFileHandle.cpp
00001 /* mbed Microcontroller Library - FATFileHandle 00002 * Copyright (c) 2008, sford 00003 */ 00004 00005 #include "FATFileHandle.h" 00006 00007 #include <stdio.h> 00008 #include <stdlib.h> 00009 #include "ff.h" 00010 #include "FATFileSystem.h" 00011 00012 namespace mbed { 00013 00014 #if FFSDEBUG_ENABLED 00015 static const char *FR_ERRORS[] = { 00016 "FR_OK = 0", 00017 "FR_NOT_READY", 00018 "FR_NO_FILE", 00019 "FR_NO_PATH", 00020 "FR_INVALID_NAME", 00021 "FR_INVALID_DRIVE", 00022 "FR_DENIED", 00023 "FR_EXIST", 00024 "FR_RW_ERROR", 00025 "FR_WRITE_PROTECTED", 00026 "FR_NOT_ENABLED", 00027 "FR_NO_FILESYSTEM", 00028 "FR_INVALID_OBJECT", 00029 "FR_MKFS_ABORTED" 00030 }; 00031 #endif 00032 00033 FATFileHandle::FATFileHandle(FIL fh) { 00034 _fh = fh; 00035 } 00036 00037 int FATFileHandle::close() { 00038 FFSDEBUG("close\n"); 00039 int retval = f_close(&_fh); 00040 delete this; 00041 return retval; 00042 } 00043 00044 ssize_t FATFileHandle::write(const void* buffer, size_t length) { 00045 FFSDEBUG("write(%d)\n", length); 00046 UINT n; 00047 FRESULT res = f_write(&_fh, buffer, length, &n); 00048 if(res) { 00049 FFSDEBUG("f_write() failed (%d, %s)", res, FR_ERRORS[res]); 00050 return -1; 00051 } 00052 return n; 00053 } 00054 00055 ssize_t FATFileHandle::read(void* buffer, size_t length) { 00056 FFSDEBUG("read(%d)\n", length); 00057 UINT n; 00058 FRESULT res = f_read(&_fh, buffer, length, &n); 00059 if(res) { 00060 FFSDEBUG("f_read() failed (%d, %s)\n", res, FR_ERRORS[res]); 00061 return -1; 00062 } 00063 return n; 00064 } 00065 00066 int FATFileHandle::isatty() { 00067 return 0; 00068 } 00069 00070 off_t FATFileHandle::lseek(off_t position, int whence) { 00071 FFSDEBUG("lseek(%i,%i)\n",position,whence); 00072 if(whence == SEEK_END) { 00073 position += _fh.fsize; 00074 } else if(whence==SEEK_CUR) { 00075 position += _fh.fptr; 00076 } 00077 FRESULT res = f_lseek(&_fh, position); 00078 if(res) { 00079 FFSDEBUG("lseek failed (%d, %s)\n", res, FR_ERRORS[res]); 00080 return -1; 00081 } else { 00082 FFSDEBUG("lseek OK, returning %i\n", _fh.fptr); 00083 return _fh.fptr; 00084 } 00085 } 00086 00087 int FATFileHandle::fsync() { 00088 FFSDEBUG("fsync()\n"); 00089 FRESULT res = f_sync(&_fh); 00090 if (res) { 00091 FFSDEBUG("f_sync() failed (%d, %s)\n", res, FR_ERRORS[res]); 00092 return -1; 00093 } 00094 return 0; 00095 } 00096 00097 off_t FATFileHandle::flen() { 00098 FFSDEBUG("flen\n"); 00099 return _fh.fsize; 00100 } 00101 00102 } // namespace mbed
Generated on Tue Jul 12 2022 14:27:20 by
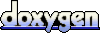