Sense keypresses from a 4x4 keypad A derivative ot he Hotboard_keypad library
Dependents: 26_Hotboards_MultiKey 26_Hotboards_EventKeypad
Fork of Hotboards_keypad by
Keypad Class Reference
Hotboards_keypad class. More...
#include <Hotboards_keypad.h>
Inherits Key.
Public Member Functions | |
Keypad (char *userKeymap, DigitalInOut *row, DigitalInOut *col, uint8_t numRows, uint8_t numCols) | |
Allows custom keymap, pin configuration, and keypad sizes. | |
char | getKey (void) |
Returns a single key only. | |
bool | getKeys (void) |
Populate the key list (check public key array). | |
KeyState | getState (void) |
Get the state of the first key on the list of active keys. | |
void | begin (char *userKeymap) |
Let the user define a keymap - assume the same row/column count as defined in constructor. | |
bool | isPressed (char keyChar) |
Return a true if the selected key is pressed. | |
void | setDebounceTime (uint) |
Set a new debounce time (1ms is the minimum value) | |
void | setHoldTime (uint) |
Set a new time to considered a key is in hold state. | |
void | addEventListener (void(*listener)(char)) |
Set a callback function to be called everytime an key change its status. | |
int | findInList (char keyChar) |
Search by character for a key in the list of active keys. | |
int | findInList (int keyCode) |
Search by code for a key in the list of active keys. | |
char | waitForKey (void) |
lock everything while waiting for a keypress. | |
bool | keyStateChanged (void) |
Return stateChanged element of the first key from the active list. | |
uint8_t | numKeys (void) |
The number of keys on the key list, key[LIST_MAX]. |
Detailed Description
Hotboards_keypad class.
Used to control general purpose leds
Example:
#include "mbed.h" #include "Hotboards_keypad.h" char keys[ 4 ][ 4 ] = { {'1','2','3','A'}, {'4','5','6','B'}, {'7','8','9','C'}, {'*','0','#','D'} }; DigitalInOut rowPins[ 4 ] = {PC_0, PC_1, PC_2, PC_3}; DigitalInOut colPins[ 4 ] = {PB_2, PB_1, PB_15, PB_14}; Keypad kpd( makeKeymap( keys ), rowPins, colPins, 4, 4 ); int main( void ) { while(1){ char key = keypad.getKey( ); if( key ){ // do something with key } } }
Definition at line 94 of file Hotboards_keypad.h.
Constructor & Destructor Documentation
Keypad | ( | char * | userKeymap, |
DigitalInOut * | row, | ||
DigitalInOut * | col, | ||
uint8_t | numRows, | ||
uint8_t | numCols | ||
) |
Allows custom keymap, pin configuration, and keypad sizes.
- Parameters:
-
userKeymap pointer to bidimentional array with key definitions row pointer to array with pins conected to rows col pointer to array with pins conected to columns numRows number of rows in use numCols number of columns in use
Example:
char keys[ 4 ][ 4 ] = { {'1','2','3','A'}, {'4','5','6','B'}, {'7','8','9','C'}, {'*','0','#','D'} }; DigitalInOut rowPins[ 4 ] = {PC_0, PC_1, PC_2, PC_3}; DigitalInOut colPins[ 4 ] = {PB_2, PB_1, PB_15, PB_14}; Keypad kpd( makeKeymap( keys ), rowPins, colPins, 4, 4 );
Definition at line 43 of file Hotboards_keypad.cpp.
Member Function Documentation
void addEventListener | ( | void(*)(char) | listener ) |
Set a callback function to be called everytime an key change its status.
- Parameters:
-
listener function to be called
Example:
kpd.addEventListener( keypadEvent );
Definition at line 255 of file Hotboards_keypad.cpp.
void begin | ( | char * | userKeymap ) |
Let the user define a keymap - assume the same row/column count as defined in constructor.
- Parameters:
-
userKeymap pointer to user keymap
Example:
// lets assume user wnats to change the keymap
kpd.begin( makeKeymap( NewKeys )),
Definition at line 61 of file Hotboards_keypad.cpp.
int findInList | ( | char | keyChar ) |
Search by character for a key in the list of active keys.
- Returns:
- Returns -1 if not found or the index into the list of active keys.
Example:
// kpd.getKeys function needs to be called first int index = findInList( '7' ); if( kpd.key[ index ].stateChanged ){ // the key change, so do something =) }
Definition at line 202 of file Hotboards_keypad.cpp.
int findInList | ( | int | keyCode ) |
Search by code for a key in the list of active keys.
- Returns:
- Returns -1 if not found or the index into the list of active keys.
Example:
// kpd.getKeys function needs to be called first int index = findInList( 10 ); if( kpd.key[ index ].stateChanged ){ // the key change, so do something =) }
Definition at line 213 of file Hotboards_keypad.cpp.
char getKey | ( | void | ) |
Returns a single key only.
Retained for backwards compatibility.
- Returns:
- key pressed (user defined key)
Example:
char key = keypad.getKey( ); if( key ){ // do something with key }
Definition at line 66 of file Hotboards_keypad.cpp.
bool getKeys | ( | void | ) |
Populate the key list (check public key array).
- Returns:
- true if user pressed any key(s)
Example:
if( kpd.getKeys( ) ){ // you need to poll the array kpd.key[] for( int i=0 ; i<LIST_MAX ; i++ ){ if( kpd.key[ i ].stateChanged ){ chat = kpd.key[ i ].kchar; } }
Definition at line 78 of file Hotboards_keypad.cpp.
KeyState getState | ( | void | ) |
Get the state of the first key on the list of active keys.
- Returns:
- key[0].kstate
Example:
if(kpd.getState() == PRESSED ){ // do something }
Definition at line 230 of file Hotboards_keypad.cpp.
bool isPressed | ( | char | keyChar ) |
Return a true if the selected key is pressed.
Is neccesary to call getKeys function first
- Returns:
- key pressed (user defined key)
Example:
if( kpd.getKeys( ) ){ if( kpd.isPressed( '2' ) ){ // key '2' have been press } }
Definition at line 190 of file Hotboards_keypad.cpp.
bool keyStateChanged | ( | void | ) |
Return stateChanged element of the first key from the active list.
- Returns:
- key[0].stateChanged
Example:
if( kpd.keyStateChanged() ){ // do something }
Definition at line 236 of file Hotboards_keypad.cpp.
uint8_t numKeys | ( | void | ) |
The number of keys on the key list, key[LIST_MAX].
- Returns:
- number of keys
Example:
int keyNum = numKeys();
Definition at line 242 of file Hotboards_keypad.cpp.
void setDebounceTime | ( | uint | debounce ) |
Set a new debounce time (1ms is the minimum value)
- Parameters:
-
debounce time in milliseconds
Example:
// change default 10ms debounce time to 20ms
kpd.setDebounceTime( 20 );
Definition at line 247 of file Hotboards_keypad.cpp.
void setHoldTime | ( | uint | hold ) |
Set a new time to considered a key is in hold state.
- Parameters:
-
hold hold time in milliseconds
Example:
// change default 500ms hold time to 250ms
kpd.setHoldTime( 250 );
Definition at line 251 of file Hotboards_keypad.cpp.
char waitForKey | ( | void | ) |
lock everything while waiting for a keypress.
- Returns:
- key pressed
Example:
char key = kpd.waitForKey();
Definition at line 223 of file Hotboards_keypad.cpp.
Generated on Fri Jul 15 2022 01:02:16 by
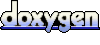