mbed library sources: Modified to operate FRDM-KL25Z at 48MHz from internal 32kHz oscillator (nothing else changed).
Fork of mbed-src by
Serial Class Reference
A serial port (UART) for communication with other serial devices. More...
#include <Serial.h>
Inherits mbed::Stream.
Public Member Functions | |
Serial (PinName tx, PinName rx, const char *name=NULL) | |
Create a Serial port, connected to the specified transmit and receive pins. | |
void | baud (int baudrate) |
Set the baud rate of the serial port. | |
void | format (int bits=8, Parity parity=Serial::None, int stop_bits=1) |
Set the transmission format used by the Serial port. | |
int | readable () |
Determine if there is a character available to read. | |
int | writeable () |
Determine if there is space available to write a character. | |
pFunctionPointer_t | attach (void(*fptr)(void), IrqType type=RxIrq) |
Attach a function to call whenever a serial interrupt is generated. | |
pFunctionPointer_t | add_handler (void(*fptr)(void), IrqType type=RxIrq) |
Add a function to be called when a serial interrupt is generated at the end of the call chain. | |
pFunctionPointer_t | add_handler_front (void(*fptr)(void), IrqType type=RxIrq) |
Add a function to be called when a serial interrupt is generated at the beginning of the call chain. | |
template<typename T > | |
pFunctionPointer_t | attach (T *tptr, void(T::*mptr)(void), IrqType type=RxIrq) |
Attach a member function to call whenever a serial interrupt is generated. | |
template<typename T > | |
pFunctionPointer_t | add_handler (T *tptr, void(T::*mptr)(void), IrqType type=RxIrq) |
Add a function to be called when a serial interrupt is generated at the end of the call chain. | |
template<typename T > | |
pFunctionPointer_t | add_handler_front (T *tptr, void(T::*mptr)(void), IrqType type=RxIrq) |
Add a function to be called when a serial interrupt is generated at the beginning of the call chain. | |
bool | remove_handler (pFunctionPointer_t pf, IrqType type=RxIrq) |
Remove a function from the list of functions to be called when a serial interrupt is generated. | |
void | send_break () |
Generate a break condition on the serial line. |
Detailed Description
A serial port (UART) for communication with other serial devices.
Can be used for Full Duplex communication, or Simplex by specifying one pin as NC (Not Connected)
Example:
// Print "Hello World" to the PC #include "mbed.h" Serial pc(USBTX, USBRX); int main() { pc.printf("Hello World\n"); }
Definition at line 48 of file Serial.h.
Constructor & Destructor Documentation
Serial | ( | PinName | tx, |
PinName | rx, | ||
const char * | name = NULL |
||
) |
Create a Serial port, connected to the specified transmit and receive pins.
- Parameters:
-
tx Transmit pin rx Receive pin
- Note:
- Either tx or rx may be specified as NC if unused
Definition at line 23 of file Serial.cpp.
Member Function Documentation
pFunctionPointer_t add_handler | ( | T * | tptr, |
void(T::*)(void) | mptr, | ||
IrqType | type = RxIrq |
||
) |
Add a function to be called when a serial interrupt is generated at the end of the call chain.
- Parameters:
-
tptr pointer to the object to call the member function on mptr pointer to the member function to be called type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty)
- Returns:
- The function object created for 'fptr'
pFunctionPointer_t add_handler | ( | void(*)(void) | fptr, |
IrqType | type = RxIrq |
||
) |
Add a function to be called when a serial interrupt is generated at the end of the call chain.
- Parameters:
-
fptr the function to add type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty)
- Returns:
- The function object created for 'fptr'
pFunctionPointer_t add_handler_front | ( | T * | tptr, |
void(T::*)(void) | mptr, | ||
IrqType | type = RxIrq |
||
) |
Add a function to be called when a serial interrupt is generated at the beginning of the call chain.
- Parameters:
-
tptr pointer to the object to call the member function on mptr pointer to the member function to be called type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty)
- Returns:
- The function object created for 'fptr'
pFunctionPointer_t add_handler_front | ( | void(*)(void) | fptr, |
IrqType | type = RxIrq |
||
) |
Add a function to be called when a serial interrupt is generated at the beginning of the call chain.
- Parameters:
-
fptr the function to add type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty)
- Returns:
- The function object created for 'fptr'
pFunctionPointer_t attach | ( | T * | tptr, |
void(T::*)(void) | mptr, | ||
IrqType | type = RxIrq |
||
) |
Attach a member function to call whenever a serial interrupt is generated.
- Parameters:
-
tptr pointer to the object to call the member function on mptr pointer to the member function to be called type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty) The function object created for 'tptr' and 'mptr'
pFunctionPointer_t attach | ( | void(*)(void) | fptr, |
IrqType | type = RxIrq |
||
) |
Attach a function to call whenever a serial interrupt is generated.
- Parameters:
-
fptr A pointer to a void function, or 0 to set as none type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty)
- Returns:
- The function object created for 'fptr'
Definition at line 47 of file Serial.cpp.
void baud | ( | int | baudrate ) |
Set the baud rate of the serial port.
- Parameters:
-
baudrate The baudrate of the serial port (default = 9600).
Definition at line 29 of file Serial.cpp.
void format | ( | int | bits = 8 , |
Parity | parity = Serial::None , |
||
int | stop_bits = 1 |
||
) |
Set the transmission format used by the Serial port.
- Parameters:
-
bits The number of bits in a word (5-8; default = 8) parity The parity used (Serial::None, Serial::Odd, Serial::Even, Serial::Forced1, Serial::Forced0; default = Serial::None) stop The number of stop bits (1 or 2; default = 1)
Definition at line 34 of file Serial.cpp.
int readable | ( | ) |
Determine if there is a character available to read.
- Returns:
- 1 if there is a character available to read, 0 otherwise
Definition at line 38 of file Serial.cpp.
bool remove_handler | ( | pFunctionPointer_t | pf, |
IrqType | type = RxIrq |
||
) |
Remove a function from the list of functions to be called when a serial interrupt is generated.
- Parameters:
-
pf the function object to remove type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty)
- Returns:
- true if the function was found and removed, false otherwise
Definition at line 67 of file Serial.cpp.
void send_break | ( | ) |
Generate a break condition on the serial line.
Definition at line 88 of file Serial.cpp.
int writeable | ( | ) |
Determine if there is space available to write a character.
- Returns:
- 1 if there is space to write a character, 0 otherwise
Definition at line 43 of file Serial.cpp.
Generated on Wed Jul 13 2022 19:29:55 by
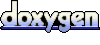