mbed library sources: Modified to operate FRDM-KL25Z at 48MHz from internal 32kHz oscillator (nothing else changed).
Fork of mbed-src by
Serial.h
00001 /* mbed Microcontroller Library 00002 * Copyright (c) 2006-2013 ARM Limited 00003 * 00004 * Licensed under the Apache License, Version 2.0 (the "License"); 00005 * you may not use this file except in compliance with the License. 00006 * You may obtain a copy of the License at 00007 * 00008 * http://www.apache.org/licenses/LICENSE-2.0 00009 * 00010 * Unless required by applicable law or agreed to in writing, software 00011 * distributed under the License is distributed on an "AS IS" BASIS, 00012 * WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. 00013 * See the License for the specific language governing permissions and 00014 * limitations under the License. 00015 */ 00016 #ifndef MBED_SERIAL_H 00017 #define MBED_SERIAL_H 00018 00019 #include "platform.h" 00020 00021 #if DEVICE_SERIAL 00022 00023 #include "Stream.h" 00024 #include "FunctionPointer.h" 00025 #include "serial_api.h" 00026 #include "CallChain.h" 00027 00028 namespace mbed { 00029 00030 /** A serial port (UART) for communication with other serial devices 00031 * 00032 * Can be used for Full Duplex communication, or Simplex by specifying 00033 * one pin as NC (Not Connected) 00034 * 00035 * Example: 00036 * @code 00037 * // Print "Hello World" to the PC 00038 * 00039 * #include "mbed.h" 00040 * 00041 * Serial pc(USBTX, USBRX); 00042 * 00043 * int main() { 00044 * pc.printf("Hello World\n"); 00045 * } 00046 * @endcode 00047 */ 00048 class Serial : public Stream { 00049 00050 public: 00051 /** Create a Serial port, connected to the specified transmit and receive pins 00052 * 00053 * @param tx Transmit pin 00054 * @param rx Receive pin 00055 * 00056 * @note 00057 * Either tx or rx may be specified as NC if unused 00058 */ 00059 Serial(PinName tx, PinName rx, const char *name=NULL); 00060 00061 /** Set the baud rate of the serial port 00062 * 00063 * @param baudrate The baudrate of the serial port (default = 9600). 00064 */ 00065 void baud(int baudrate); 00066 00067 enum Parity { 00068 None = 0, 00069 Odd, 00070 Even, 00071 Forced1, 00072 Forced0 00073 }; 00074 00075 enum IrqType { 00076 RxIrq = 0, 00077 TxIrq 00078 }; 00079 00080 /** Set the transmission format used by the Serial port 00081 * 00082 * @param bits The number of bits in a word (5-8; default = 8) 00083 * @param parity The parity used (Serial::None, Serial::Odd, Serial::Even, Serial::Forced1, Serial::Forced0; default = Serial::None) 00084 * @param stop The number of stop bits (1 or 2; default = 1) 00085 */ 00086 void format(int bits=8, Parity parity=Serial::None, int stop_bits=1); 00087 00088 /** Determine if there is a character available to read 00089 * 00090 * @returns 00091 * 1 if there is a character available to read, 00092 * 0 otherwise 00093 */ 00094 int readable(); 00095 00096 /** Determine if there is space available to write a character 00097 * 00098 * @returns 00099 * 1 if there is space to write a character, 00100 * 0 otherwise 00101 */ 00102 int writeable(); 00103 00104 /** Attach a function to call whenever a serial interrupt is generated 00105 * 00106 * @param fptr A pointer to a void function, or 0 to set as none 00107 * @param type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty) 00108 * 00109 * @returns 00110 * The function object created for 'fptr' 00111 */ 00112 pFunctionPointer_t attach(void (*fptr)(void), IrqType type=RxIrq); 00113 00114 /** Add a function to be called when a serial interrupt is generated at the end of the call chain 00115 * 00116 * @param fptr the function to add 00117 * @param type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty) 00118 * 00119 * @returns 00120 * The function object created for 'fptr' 00121 */ 00122 pFunctionPointer_t add_handler(void (*fptr)(void), IrqType type=RxIrq) { 00123 return add_handler_helper(fptr, type); 00124 } 00125 00126 /** Add a function to be called when a serial interrupt is generated at the beginning of the call chain 00127 * 00128 * @param fptr the function to add 00129 * @param type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty) 00130 * 00131 * @returns 00132 * The function object created for 'fptr' 00133 */ 00134 pFunctionPointer_t add_handler_front(void (*fptr)(void), IrqType type=RxIrq) { 00135 return add_handler_helper(fptr, type, true); 00136 } 00137 00138 /** Attach a member function to call whenever a serial interrupt is generated 00139 * 00140 * @param tptr pointer to the object to call the member function on 00141 * @param mptr pointer to the member function to be called 00142 * @param type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty) 00143 * 00144 * @param 00145 * The function object created for 'tptr' and 'mptr' 00146 */ 00147 template<typename T> 00148 pFunctionPointer_t attach(T* tptr, void (T::*mptr)(void), IrqType type=RxIrq) { 00149 if((mptr != NULL) && (tptr != NULL)) { 00150 _irq[type].clear(); 00151 pFunctionPointer_t pf = _irq[type].add(tptr, mptr); 00152 serial_irq_set(&_serial, (SerialIrq)type, 1); 00153 return pf; 00154 } 00155 else 00156 return NULL; 00157 } 00158 00159 /** Add a function to be called when a serial interrupt is generated at the end of the call chain 00160 * 00161 * @param tptr pointer to the object to call the member function on 00162 * @param mptr pointer to the member function to be called 00163 * @param type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty) 00164 * 00165 * @returns 00166 * The function object created for 'fptr' 00167 */ 00168 template<typename T> 00169 pFunctionPointer_t add_handler(T* tptr, void (T::*mptr)(void), IrqType type=RxIrq) { 00170 return add_handler_helper(tptr, mptr, type); 00171 } 00172 00173 /** Add a function to be called when a serial interrupt is generated at the beginning of the call chain 00174 * 00175 * @param tptr pointer to the object to call the member function on 00176 * @param mptr pointer to the member function to be called 00177 * @param type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty) 00178 * 00179 * @returns 00180 * The function object created for 'fptr' 00181 */ 00182 template<typename T> 00183 pFunctionPointer_t add_handler_front(T* tptr, void (T::*mptr)(void), IrqType type=RxIrq) { 00184 return add_handler_helper(tptr, mptr, type, true); 00185 } 00186 00187 /** Remove a function from the list of functions to be called when a serial interrupt is generated 00188 * 00189 * @param pf the function object to remove 00190 * @param type Which serial interrupt to attach the member function to (Seriall::RxIrq for receive, TxIrq for transmit buffer empty) 00191 * 00192 * @returns 00193 * true if the function was found and removed, false otherwise 00194 */ 00195 bool remove_handler(pFunctionPointer_t pf, IrqType type=RxIrq); 00196 00197 /** Generate a break condition on the serial line 00198 */ 00199 void send_break(); 00200 00201 static void _irq_handler(uint32_t id, SerialIrq irq_type); 00202 00203 protected: 00204 virtual int _getc(); 00205 virtual int _putc(int c); 00206 pFunctionPointer_t add_handler_helper(void (*function)(void), IrqType type, bool front=false); 00207 00208 template<typename T> 00209 pFunctionPointer_t add_handler_helper(T* tptr, void (T::*mptr)(void), IrqType type, bool front=false) { 00210 if ((mptr != NULL) && (tptr != NULL)) { 00211 pFunctionPointer_t pf = front ? _irq[type].add_front(tptr, mptr) : _irq[type].add(tptr, mptr); 00212 serial_irq_set(&_serial, (SerialIrq)type, 1); 00213 return pf; 00214 } 00215 else 00216 return NULL; 00217 } 00218 00219 serial_t _serial; 00220 CallChain _irq[2]; 00221 int _baud; 00222 }; 00223 00224 } // namespace mbed 00225 00226 #endif 00227 00228 #endif
Generated on Wed Jul 13 2022 19:29:54 by
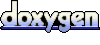