Some useful stuff
Dependents: FtEncoder FtControlSet
Buffer< T, N > Class Template Reference
A template for a simple buffer class holding at max N elements of type T. More...
#include <Buffer.h>
Inherited by BufferOnHeap< T, N >, and BufferOnStack< T, N >.
Public Member Functions | |
bool | push (const T &element) |
If used as queue or stack, use this function to insert new element to the buffer. | |
void | pushCircular (const T &element) |
If used as ring buffer, use this function to insert new elements to the Buffer. | |
const T & | popLatest () |
Pop the latest element from buffer. Returns a default instance of type T if empty. | |
const T & | popOldest () |
Pop the oldest element from buffer. Returns a default instance of type T if empty. | |
bool | empty () const |
Returns true if buffer is empty. | |
bool | full () const |
Returns true if buffer is full. | |
uint32_t | size () const |
Retuns number of currently stored elements. | |
uint32_t | maxSize () const |
returns maximum number of storable elements | |
void | clear () |
Clear the Buffer. | |
const T & | operator[] (uint32_t idx) |
Read only access operator: Element with index 0 is the oldest and the one with index size()-1 the latest. | |
Buffer & | operator= (const Buffer &buf) |
assignment operator ... | |
virtual | ~Buffer () |
virtual destructor | |
Protected Member Functions | |
Buffer () | |
Guess what: a constructor! But this class is kind of abstract, so it has been declared protected to remove it from the public interface. | |
Protected Attributes | |
T * | elements |
The buffer for the stored elements, initialized in the concrete specializations. |
Detailed Description
template<typename T, uint32_t N>
class Buffer< T, N >
A template for a simple buffer class holding at max N elements of type T.
Can be used as stack, queue or ring buffer This is kind of an abstract base class. There are heap and a stack based based concrete specializations.
Definition at line 11 of file Buffer.h.
Constructor & Destructor Documentation
Buffer | ( | ) | [protected] |
Member Function Documentation
uint32_t maxSize | ( | ) | const |
const T& operator[] | ( | uint32_t | idx ) |
const T& popLatest | ( | ) |
const T& popOldest | ( | ) |
bool push | ( | const T & | element ) |
void pushCircular | ( | const T & | element ) |
uint32_t size | ( | ) | const |
Field Documentation
Generated on Wed Jul 20 2022 14:56:27 by
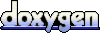