RTOS enabled i2c-driver based on the official i2c-C-api.
Fork of mbed-RtosI2cDriver by
I2CDriverTest02.h
00001 // exchange messages betwen the LPC1768's two i2c ports using high level reead/write commands 00002 // changing master and slave mode on the fly 00003 00004 #include "mbed.h" 00005 #include "rtos.h" 00006 #include "I2CMasterRtos.h" 00007 #include "I2CSlaveRtos.h" 00008 00009 const int freq = 400000; 00010 const int adr = 42<<1; 00011 const int len=34; 00012 const char mstMsg[len]="We are mbed, resistance is futile"; 00013 const char slvMsg[len]="Fine with me, let's get addicted "; 00014 00015 static void slvRxMsg(I2CSlaveRtos& slv) 00016 { 00017 char rxMsg[len]; 00018 memset(rxMsg,0,len); 00019 if ( slv.receive() == I2CSlave::WriteAddressed) { 00020 slv.read(rxMsg, len); 00021 printf("thread %X received message as i2c slave: '%s'\n",Thread::gettid(),rxMsg); 00022 } else 00023 printf("Ouch slv rx failure\n"); 00024 } 00025 00026 static void slvTxMsg(I2CSlaveRtos& slv) 00027 { 00028 if ( slv.receive() == I2CSlave::ReadAddressed) { 00029 slv.write(slvMsg, len); 00030 } else 00031 printf("Ouch slv tx failure\n"); 00032 } 00033 00034 static void mstTxMsg(I2CMasterRtos& mst) 00035 { 00036 mst.write(adr,mstMsg,len); 00037 } 00038 00039 static void mstRxMsg(I2CMasterRtos& mst) 00040 { 00041 char rxMsg[len]; 00042 memset(rxMsg,0,len); 00043 mst.read(adr,rxMsg,len); 00044 printf("thread %X received message as i2c master: '%s'\n",Thread::gettid(),rxMsg); 00045 } 00046 00047 static void channel1(void const *args) 00048 { 00049 I2CMasterRtos mst(p9,p10,freq); 00050 I2CSlaveRtos slv(p9,p10,freq,adr); 00051 while(1) { 00052 slvRxMsg(slv); 00053 slvTxMsg(slv); 00054 Thread::wait(100); 00055 mstTxMsg(mst); 00056 Thread::wait(100); 00057 mstRxMsg(mst); 00058 } 00059 } 00060 00061 void channel2(void const *args) 00062 { 00063 I2CMasterRtos mst(p28,p27,freq); 00064 I2CSlaveRtos slv(p28,p27,freq,adr); 00065 while(1) { 00066 Thread::wait(100); 00067 mstTxMsg(mst); 00068 Thread::wait(100); 00069 mstRxMsg(mst); 00070 slvRxMsg(slv); 00071 slvTxMsg(slv); 00072 } 00073 } 00074 00075 int doit() 00076 { 00077 Thread selftalk01(channel1,0,osPriorityAboveNormal); 00078 Thread selftalk02(channel2,0,osPriorityAboveNormal); 00079 00080 Thread::wait(10000); 00081 00082 return 0; 00083 } 00084
Generated on Wed Jul 13 2022 17:20:05 by
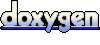