RTOS enabled i2c-driver based on the official i2c-C-api.
Fork of mbed-RtosI2cDriver by
I2CDriverTest01.h
00001 // A high prio thread reads at a rate of 1kHz from a SRF08 ultrasonic ranger 00002 // data packets of different size, whereas the lower prio main thread measures the CPU time left. 00003 00004 #include "mbed.h" 00005 #include "rtos.h" 00006 #include "I2CMasterRtos.h" 00007 #include "stdint.h" 00008 00009 volatile int g_disco=0; 00010 volatile int g_len=2; 00011 volatile int g_freq=100000; 00012 00013 I2CMasterRtos i2c(p28, p27); 00014 const uint32_t adr = 0x70<<1; 00015 00016 void highPrioCallBck(void const *args) 00017 { 00018 i2c.frequency(g_freq); 00019 // read back srf08 echo times (1+16 words) 00020 const char reg= 0x02; 00021 char result[64]; 00022 uint32_t t1=us_ticker_read(); 00023 i2c.read(adr, reg, result, g_len); 00024 uint32_t dt=us_ticker_read()-t1; 00025 uint16_t dst=((static_cast<uint16_t>(result[0])<<8)|static_cast<uint16_t>(result[1])); 00026 if(--g_disco>0) printf("dst=%4dcm dt=%4dus\n",dst,dt); 00027 } 00028 00029 int doit() 00030 { 00031 RtosTimer highPrioTicker(highPrioCallBck, osTimerPeriodic, (void *)0); 00032 00033 Thread::wait(500); 00034 // trigger srf08 ranging 00035 const char regNcmd[2]= {0x00,0x54}; 00036 i2c.write(adr, regNcmd, 2); 00037 osDelay(200); 00038 00039 highPrioTicker.start(1); 00040 00041 #if defined(TARGET_LPC1768) 00042 const int nTest=7; 00043 const int freq[nTest]= {1e5, 1e5, 1e5, 4e5, 4e5, 4e5, 4e5}; 00044 const int len[nTest]= {1, 4, 6, 1, 6, 12, 25}; 00045 #elif defined(TARGET_LPC11U24) 00046 const int nTest=5; 00047 const int freq[nTest]= {1e5, 1e5, 4e5, 4e5, 4e5 }; 00048 const int len[nTest]= {1, 6, 1, 6, 23}; 00049 #endif 00050 for(int i=0; i<nTest; ++i) { 00051 g_freq = freq[i]; 00052 g_len = len[i]; 00053 printf("f=%d l=%d\n",g_freq,g_len); 00054 Thread::wait(500); 00055 const uint32_t dt=1e6; 00056 uint32_t tStart = us_ticker_read(); 00057 uint32_t tLast = tStart; 00058 uint32_t tAct = tStart; 00059 uint32_t tMe=0; 00060 do { 00061 tAct=us_ticker_read(); 00062 #if defined(TARGET_LPC1768) 00063 if(tAct==tLast+1)++tMe; 00064 #elif defined(TARGET_LPC11U24) 00065 uint32_t delta = tAct-tLast; 00066 if(delta<=2)tMe+=delta; // on the 11U24 this loop takes a bit longer than 1µs (ISR ~3µs, task switch ~8µs) 00067 #endif 00068 tLast=tAct; 00069 } while(tAct-tStart<dt); 00070 printf("dc=%5.2f \n", 100.0*(float)tMe/dt); 00071 g_disco=5; 00072 while(g_disco>0); 00073 } 00074 return 0; 00075 }
Generated on Wed Jul 13 2022 17:20:05 by
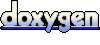