BLE SENSORS TAG BMP180
Dependents: LinkNode_BMP180 LinkNode_BMP180_derron
Fork of BMP180 by
BMP180 Class Reference
Bosch BMP180 Digital Pressure Sensor. More...
#include <BMP180.h>
Public Types | |
enum | oversampling_t { ULTRA_LOW_POWER = 0, STANDARD = 1, HIGH_RESOLUTION = 2, ULTRA_HIGH_RESOLUTION = 3 } |
Oversampling ratio. More... | |
Public Member Functions | |
BMP180 (PinName sda, PinName scl) | |
BMP180 constructor. | |
BMP180 (I2C *i2c) | |
BMP180 constructor. | |
~BMP180 () | |
BMP180 destructor. | |
int | init (void) |
Initialize BMP180. | |
int | reset (void) |
Reset BMP180. | |
int | checkId (void) |
Check ID. | |
int | startPressure (BMP180::oversampling_t oss) |
Start pressure conversion. | |
int | getPressure (int *pressure) |
Get pressure reading. | |
int | startTemperature (void) |
Start temperature conversion. | |
int | getTemperature (float *tempC) |
Get temperature reading. | |
int | getTemperature (int16_t *tempCx10) |
Get temperature reading. |
Detailed Description
Bosch BMP180 Digital Pressure Sensor.
#include <stdio.h> #include "mbed.h" #include "BMP180.h" I2C i2c(I2C_SDA, I2C_SCL); BMP180 bmp180(&i2c); int main(void) { while(1) { if (bmp180.init() != 0) { printf("Error communicating with BMP180\n"); } else { printf("Initialized BMP180\n"); break; } wait(1); } while(1) { bmp180.startTemperature(); wait_ms(5); // Wait for conversion to complete float temp; if(bmp180.getTemperature(&temp) != 0) { printf("Error getting temperature\n"); continue; } bmp180.startPressure(BMP180::ULTRA_LOW_POWER); wait_ms(10); // Wait for conversion to complete int pressure; if(bmp180.getPressure(&pressure) != 0) { printf("Error getting pressure\n"); continue; } printf("Pressure = %d Pa Temperature = %f C\n", pressure, temp); wait(1); } }
Definition at line 85 of file BMP180.h.
Member Enumeration Documentation
enum oversampling_t |
Oversampling ratio.
Dictates how many pressure samples to take. Conversion time varies depending on the number of samples taken. Refer to data sheet for timing specifications.
Constructor & Destructor Documentation
BMP180 | ( | PinName | sda, |
PinName | scl | ||
) |
BMP180 constructor.
- Parameters:
-
sda mbed pin to use for SDA line of I2C interface. scl mbed pin to use for SCL line of I2C interface.
Definition at line 52 of file BMP180.cpp.
BMP180 | ( | I2C * | i2c ) |
~BMP180 | ( | ) |
BMP180 destructor.
Definition at line 68 of file BMP180.cpp.
Member Function Documentation
int checkId | ( | void | ) |
Check ID.
Checks the device ID, should be 0x55 on reset.
- Returns:
- 0 if no errors, -1 if error.
Definition at line 121 of file BMP180.cpp.
int getPressure | ( | int * | pressure ) |
Get pressure reading.
Calculates the pressure using the data calibration data and formula. Pressure is reported in Pascals.
- Note:
- This function should be called after calling startPressure(). Refer to the data sheet for the timing requirements. Calling this function too soon can result in oversampling.
- Parameters:
-
pressure Pointer to store pressure reading.
- Returns:
- 0 if no errors, -1 if error.
Definition at line 159 of file BMP180.cpp.
int getTemperature | ( | int16_t * | tempCx10 ) |
Get temperature reading.
Calculates the temperature using the data calibration data and formula. Temperature is reported in 1/10ths degrees Celcius.
- Note:
- This function should be called after calling startTemperature(). Refer to the data sheet for the timing requirements. Calling this function too soon can result in oversampling.
- Parameters:
-
tempCx10 Pointer to store temperature reading.
- Returns:
- 0 if no errors, -1 if error.
Definition at line 244 of file BMP180.cpp.
int getTemperature | ( | float * | tempC ) |
Get temperature reading.
Calculates the temperature using the data calibration data and formula. Temperature is reported in degrees Celcius.
- Note:
- This function should be called after calling startTemperature(). Refer to the data sheet for the timing requirements. Calling this function too soon can result in oversampling.
- Parameters:
-
tempC Pointer to store temperature reading.
- Returns:
- 0 if no errors, -1 if error.
Definition at line 217 of file BMP180.cpp.
int init | ( | void | ) |
Initialize BMP180.
Gets the device ID and saves the calibration values.
- Returns:
- 0 if no errors, -1 if error.
Definition at line 76 of file BMP180.cpp.
int reset | ( | void | ) |
Reset BMP180.
Performs a soft reset of the device. Same sequence as power on reset.
- Returns:
- 0 if no errors, -1 if error.
Definition at line 103 of file BMP180.cpp.
int startPressure | ( | BMP180::oversampling_t | oss ) |
Start pressure conversion.
Initiates the pressure conversion sequence. Refer to data sheet for timing specifications.
- Parameters:
-
oss Number of samples to take.
- Returns:
- 0 if no errors, -1 if error.
Definition at line 143 of file BMP180.cpp.
int startTemperature | ( | void | ) |
Start temperature conversion.
Initiates the temperature conversion sequence. Refer to data sheet for timing specifications.
- Returns:
- 0 if no errors, -1 if error.
Definition at line 205 of file BMP180.cpp.
Generated on Thu Jul 21 2022 06:17:42 by
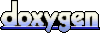