
X-CUBE-SPN1-20150128 example source code for one motor compiled under mbed. Tested OK on Nucleo F401. l6474.cpp is modified from original with defines in l6474_target_config.h to select the original behaviour (motor de-energised when halted), or new mode to continue powering with a (reduced) current in the coils (braking/position hold capability). On F401 avoid using mbed's InterruptIn on pins 10-15 (any port). Beware of other conflicts! L0 & F0 are included but untested.
stm32f0xx_hal_msp.cpp
00001 /** 00002 ****************************************************************************** 00003 * @file Multi/Examples/MotionControl/IHM01A1_ExampleFor1Motor/Src/stm32f0xx_hal_msp.c 00004 * @author IPC Rennes 00005 * @version V1.5.0 00006 * @date November 12, 2014 00007 * @brief HAL MSP module. 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 #ifdef TARGET_STM32F0 00038 /* Includes ------------------------------------------------------------------*/ 00039 #include "mbed.h" 00040 #include "ihm01a1.h" 00041 00042 00043 /** @defgroup MSP_module 00044 * @brief HAL MSP module. 00045 * @{ 00046 */ 00047 00048 /* Private typedef -----------------------------------------------------------*/ 00049 /* Private define ------------------------------------------------------------*/ 00050 /* Private macro -------------------------------------------------------------*/ 00051 /* Private variables ---------------------------------------------------------*/ 00052 00053 /* Private function prototypes -----------------------------------------------*/ 00054 extern void BSP_MotorControl_StepClockHandler(uint8_t deviceId); 00055 extern void BSP_MotorControl_FlagInterruptHandler(void); 00056 /* Private functions ---------------------------------------------------------*/ 00057 00058 /** @defgroup HAL_MSP_Private_Functions 00059 * @{ 00060 */ 00061 00062 /** 00063 * @brief SPI MSP Initialization 00064 * This function configures the hardware resources used in this example: 00065 * - Peripheral's clock enable 00066 * - Peripheral's GPIO Configuration 00067 * @param[in] hspi SPI handle pointer 00068 * @retval None 00069 */ 00070 void HAL_SPI_MspInit(SPI_HandleTypeDef *hspi) 00071 { 00072 GPIO_InitTypeDef GPIO_InitStruct; 00073 00074 if(hspi->Instance == SPIx) 00075 { 00076 /*##-1- Enable peripherals and GPIO Clocks #################################*/ 00077 /* Enable GPIO TX/RX clock */ 00078 SPIx_SCK_GPIO_CLK_ENABLE(); 00079 SPIx_MISO_GPIO_CLK_ENABLE(); 00080 SPIx_MOSI_GPIO_CLK_ENABLE(); 00081 /* Enable SPI clock */ 00082 SPIx_CLK_ENABLE(); 00083 00084 /*##-2- Configure peripheral GPIO ##########################################*/ 00085 /* SPI SCK GPIO pin configuration */ 00086 GPIO_InitStruct.Pin = SPIx_SCK_PIN; 00087 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00088 GPIO_InitStruct.Pull = GPIO_NOPULL; 00089 GPIO_InitStruct.Speed = GPIO_SPEED_MEDIUM; 00090 GPIO_InitStruct.Alternate = SPIx_SCK_AF; 00091 00092 HAL_GPIO_Init(SPIx_SCK_GPIO_PORT, &GPIO_InitStruct); 00093 00094 /* SPI MISO GPIO pin configuration */ 00095 GPIO_InitStruct.Pin = SPIx_MISO_PIN; 00096 GPIO_InitStruct.Alternate = SPIx_MISO_AF; 00097 00098 HAL_GPIO_Init(SPIx_MISO_GPIO_PORT, &GPIO_InitStruct); 00099 00100 /* SPI MOSI GPIO pin configuration */ 00101 GPIO_InitStruct.Pin = SPIx_MOSI_PIN; 00102 GPIO_InitStruct.Alternate = SPIx_MOSI_AF; 00103 00104 HAL_GPIO_Init(SPIx_MOSI_GPIO_PORT, &GPIO_InitStruct); 00105 } 00106 } 00107 00108 /** 00109 * @brief SPI MSP De-Initialization 00110 * This function frees the hardware resources used in this example: 00111 * - Disable the Peripheral's clock 00112 * - Revert GPIO configuration to its default state 00113 * @param[in] hspi SPI handle pointer 00114 * @retval None 00115 */ 00116 void HAL_SPI_MspDeInit(SPI_HandleTypeDef *hspi) 00117 { 00118 if(hspi->Instance == SPIx) 00119 { 00120 /*##-1- Reset peripherals ##################################################*/ 00121 SPIx_FORCE_RESET(); 00122 SPIx_RELEASE_RESET(); 00123 00124 /*##-2- Disable peripherals and GPIO Clocks ################################*/ 00125 /* Configure SPI SCK as alternate function */ 00126 HAL_GPIO_DeInit(SPIx_SCK_GPIO_PORT, SPIx_SCK_PIN); 00127 /* Configure SPI MISO as alternate function */ 00128 HAL_GPIO_DeInit(SPIx_MISO_GPIO_PORT, SPIx_MISO_PIN); 00129 /* Configure SPI MOSI as alternate function */ 00130 HAL_GPIO_DeInit(SPIx_MOSI_GPIO_PORT, SPIx_MOSI_PIN); 00131 } 00132 } 00133 00134 /** 00135 * @brief PWM MSP Initialization 00136 * @param[in] htim_pwm PWM handle pointer 00137 * @retval None 00138 */ 00139 void HAL_TIM_PWM_MspInit(TIM_HandleTypeDef* htim_pwm) 00140 { 00141 GPIO_InitTypeDef GPIO_InitStruct; 00142 if(htim_pwm->Instance == BSP_MOTOR_CONTROL_BOARD_TIMER_PWM1) 00143 { 00144 /* Peripheral clock enable */ 00145 __BSP_MOTOR_CONTROL_BOARD_TIMER_PWM1_CLCK_ENABLE(); 00146 00147 /* GPIO configuration */ 00148 GPIO_InitStruct.Pin = BSP_MOTOR_CONTROL_BOARD_PWM_1_PIN; 00149 GPIO_InitStruct.Mode = GPIO_MODE_AF_PP; 00150 GPIO_InitStruct.Pull = GPIO_NOPULL; 00151 GPIO_InitStruct.Speed = GPIO_SPEED_LOW; 00152 GPIO_InitStruct.Alternate = BSP_MOTOR_CONTROL_BOARD_AFx_TIMx_PWM1; 00153 HAL_GPIO_Init(BSP_MOTOR_CONTROL_BOARD_PWM_1_PORT, &GPIO_InitStruct); 00154 00155 /* Set Interrupt Group Priority of Timer Interrupt*/ 00156 HAL_NVIC_SetPriority(BSP_MOTOR_CONTROL_BOARD_PWM1_IRQn, 4, 0); 00157 00158 /* Enable the timer global Interrupt */ 00159 HAL_NVIC_EnableIRQ(BSP_MOTOR_CONTROL_BOARD_PWM1_IRQn); 00160 } 00161 else if(htim_pwm->Instance == BSP_MOTOR_CONTROL_BOARD_TIMER_PWM2) 00162 { 00163 /* Peripheral clock enable */ 00164 __BSP_MOTOR_CONTROL_BOARD_TIMER_PWM2_CLCK_ENABLE(); 00165 00166 /* GPIO configuration */ 00167 GPIO_InitStruct.Pin = BSP_MOTOR_CONTROL_BOARD_PWM_2_PIN; 00168 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00169 GPIO_InitStruct.Pull = GPIO_NOPULL; 00170 GPIO_InitStruct.Speed = GPIO_SPEED_LOW; 00171 HAL_GPIO_Init(BSP_MOTOR_CONTROL_BOARD_PWM_2_PORT, &GPIO_InitStruct); 00172 00173 /* Set Interrupt Group Priority of Timer Interrupt*/ 00174 HAL_NVIC_SetPriority(BSP_MOTOR_CONTROL_BOARD_PWM2_IRQn, 3, 0); 00175 00176 /* Enable the timer global Interrupt */ 00177 HAL_NVIC_EnableIRQ(BSP_MOTOR_CONTROL_BOARD_PWM2_IRQn); 00178 00179 } 00180 else if(htim_pwm->Instance == BSP_MOTOR_CONTROL_BOARD_TIMER_PWM3) 00181 { 00182 /* Peripheral clock enable */ 00183 __BSP_MOTOR_CONTROL_BOARD_TIMER_PWM3_CLCK_ENABLE(); 00184 00185 /* GPIO configuration */ 00186 GPIO_InitStruct.Pin = BSP_MOTOR_CONTROL_BOARD_PWM_3_PIN; 00187 GPIO_InitStruct.Mode = GPIO_MODE_OUTPUT_PP; 00188 GPIO_InitStruct.Pull = GPIO_NOPULL; 00189 GPIO_InitStruct.Speed = GPIO_SPEED_LOW; 00190 HAL_GPIO_Init(BSP_MOTOR_CONTROL_BOARD_PWM_3_PORT, &GPIO_InitStruct); 00191 00192 /* Set Interrupt Group Priority of Timer Interrupt*/ 00193 HAL_NVIC_SetPriority(BSP_MOTOR_CONTROL_BOARD_PWM3_IRQn, 3, 0); 00194 00195 /* Enable the timer global Interrupt */ 00196 HAL_NVIC_EnableIRQ(BSP_MOTOR_CONTROL_BOARD_PWM3_IRQn); 00197 } 00198 } 00199 00200 /** 00201 * @brief PWM MSP De-Initialization 00202 * @param[in] htim_pwm PWM handle pointer 00203 * @retval None 00204 */ 00205 void HAL_TIM_PWM_MspDeInit(TIM_HandleTypeDef* htim_pwm) 00206 { 00207 if(htim_pwm->Instance == BSP_MOTOR_CONTROL_BOARD_TIMER_PWM1) 00208 { 00209 /* Peripheral clock disable */ 00210 __BSP_MOTOR_CONTROL_BOARD_TIMER_PWM1_CLCK_DISABLE(); 00211 00212 /* GPIO Deconfiguration */ 00213 HAL_GPIO_DeInit(BSP_MOTOR_CONTROL_BOARD_PWM_1_PORT, BSP_MOTOR_CONTROL_BOARD_PWM_1_PIN); 00214 00215 } 00216 else if(htim_pwm->Instance == BSP_MOTOR_CONTROL_BOARD_TIMER_PWM2) 00217 { 00218 /* Peripheral clock disable */ 00219 __BSP_MOTOR_CONTROL_BOARD_TIMER_PWM2_CLCK_DISABLE(); 00220 00221 /* GPIO Deconfiguration */ 00222 HAL_GPIO_DeInit(BSP_MOTOR_CONTROL_BOARD_PWM_2_PORT, BSP_MOTOR_CONTROL_BOARD_PWM_2_PIN); 00223 00224 } 00225 else if(htim_pwm->Instance == BSP_MOTOR_CONTROL_BOARD_TIMER_PWM3) 00226 { 00227 /* Peripheral clock disable */ 00228 __BSP_MOTOR_CONTROL_BOARD_TIMER_PWM3_CLCK_DISABLE(); 00229 00230 /* GPIO Deconfiguration */ 00231 HAL_GPIO_DeInit(BSP_MOTOR_CONTROL_BOARD_PWM_3_PORT, BSP_MOTOR_CONTROL_BOARD_PWM_3_PIN); 00232 } 00233 } 00234 00235 /** 00236 * @brief PWM Callback 00237 * @param[in] htim PWM handle pointer 00238 * @retval None 00239 */ 00240 void HAL_TIM_PWM_PulseFinishedCallback(TIM_HandleTypeDef *htim) 00241 { 00242 if ((htim->Instance == BSP_MOTOR_CONTROL_BOARD_TIMER_PWM1)&& (htim->Channel == BSP_MOTOR_CONTROL_BOARD_HAL_ACT_CHAN_TIMER_PWM1)) 00243 { 00244 if (BSP_MotorControl_GetDeviceState(0) != INACTIVE) 00245 { 00246 BSP_MotorControl_StepClockHandler(0); 00247 } 00248 } 00249 if ((htim->Instance == BSP_MOTOR_CONTROL_BOARD_TIMER_PWM2)&& (htim->Channel == BSP_MOTOR_CONTROL_BOARD_HAL_ACT_CHAN_TIMER_PWM2)) 00250 { 00251 HAL_GPIO_TogglePin(BSP_MOTOR_CONTROL_BOARD_PWM_2_PORT, BSP_MOTOR_CONTROL_BOARD_PWM_2_PIN); 00252 if ((BSP_MotorControl_GetDeviceState(1) != INACTIVE)&& 00253 (HAL_GPIO_ReadPin(BSP_MOTOR_CONTROL_BOARD_PWM_2_PORT, BSP_MOTOR_CONTROL_BOARD_PWM_2_PIN) == GPIO_PIN_SET)) 00254 { 00255 BSP_MotorControl_StepClockHandler(1); 00256 } 00257 } 00258 if ((htim->Instance == BSP_MOTOR_CONTROL_BOARD_TIMER_PWM3)&& (htim->Channel == BSP_MOTOR_CONTROL_BOARD_HAL_ACT_CHAN_TIMER_PWM3)) 00259 { 00260 HAL_GPIO_TogglePin(BSP_MOTOR_CONTROL_BOARD_PWM_3_PORT, BSP_MOTOR_CONTROL_BOARD_PWM_3_PIN); 00261 if ((BSP_MotorControl_GetDeviceState(2) != INACTIVE)&& 00262 (HAL_GPIO_ReadPin(BSP_MOTOR_CONTROL_BOARD_PWM_3_PORT, BSP_MOTOR_CONTROL_BOARD_PWM_3_PIN) == GPIO_PIN_SET)) 00263 { 00264 BSP_MotorControl_StepClockHandler(2); 00265 } 00266 } 00267 } 00268 00269 /** 00270 * @brief External Line Callback 00271 * @param[in] GPIO_Pin pin number 00272 * @retval None 00273 */ 00274 void HAL_GPIO_EXTI_Callback(uint16_t GPIO_Pin) 00275 { 00276 if (GPIO_Pin == BSP_MOTOR_CONTROL_BOARD_FLAG_PIN) 00277 { 00278 BSP_MotorControl_FlagInterruptHandler(); 00279 } 00280 } 00281 /** 00282 * @} 00283 */ 00284 00285 /** 00286 * @} 00287 */ 00288 #endif 00289 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 22:53:31 by
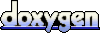