
X-CUBE-SPN1-20150128 example source code for one motor compiled under mbed. Tested OK on Nucleo F401. l6474.cpp is modified from original with defines in l6474_target_config.h to select the original behaviour (motor de-energised when halted), or new mode to continue powering with a (reduced) current in the coils (braking/position hold capability). On F401 avoid using mbed's InterruptIn on pins 10-15 (any port). Beware of other conflicts! L0 & F0 are included but untested.
main.cpp
00001 /** 00002 ****************************************************************************** 00003 * @file Multi/Examples/MotionControl/IHM01A1_ExampleFor1Motor/Src/main.c 00004 * @author IPC Rennes 00005 * @version V1.5.0 00006 * @date November 12, 2014 00007 * @brief This example shows how to use 1 IHM01A1 expansion board 00008 ****************************************************************************** 00009 * @attention 00010 * 00011 * <h2><center>© COPYRIGHT(c) 2014 STMicroelectronics</center></h2> 00012 * 00013 * Redistribution and use in source and binary forms, with or without modification, 00014 * are permitted provided that the following conditions are met: 00015 * 1. Redistributions of source code must retain the above copyright notice, 00016 * this list of conditions and the following disclaimer. 00017 * 2. Redistributions in binary form must reproduce the above copyright notice, 00018 * this list of conditions and the following disclaimer in the documentation 00019 * and/or other materials provided with the distribution. 00020 * 3. Neither the name of STMicroelectronics nor the names of its contributors 00021 * may be used to endorse or promote products derived from this software 00022 * without specific prior written permission. 00023 * 00024 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" 00025 * AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE 00026 * IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE 00027 * DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE 00028 * FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL 00029 * DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR 00030 * SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER 00031 * CAUSED AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, 00032 * OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00033 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00034 * 00035 ****************************************************************************** 00036 */ 00037 00038 /* Includes ------------------------------------------------------------------*/ 00039 #include "mbed.h" 00040 #include "ihm01a1.h" 00041 00042 /** @defgroup IHM01A1_Example_for_1_motor_device 00043 * @{ 00044 */ 00045 00046 /* Private typedef -----------------------------------------------------------*/ 00047 /* Private define ------------------------------------------------------------*/ 00048 /* Private macro -------------------------------------------------------------*/ 00049 /* Private variables ---------------------------------------------------------*/ 00050 static volatile uint16_t gLastError; 00051 00052 /* Private function prototypes -----------------------------------------------*/ 00053 static void MyFlagInterruptHandler(void); 00054 00055 /* Private functions ---------------------------------------------------------*/ 00056 00057 /** 00058 * @brief Main program 00059 * @param None 00060 * @retval None 00061 */ 00062 int main(void) 00063 { 00064 int32_t pos; 00065 uint16_t mySpeed; 00066 00067 /* STM32xx HAL library initialization */ 00068 // HAL_Init(); 00069 00070 /* Configure the system clock */ 00071 //mbed SystemClock_Config(); 00072 00073 //----- Init of the Motor control library 00074 /* Start the L6474 library to use 1 device */ 00075 /* The L6474 registers are set with the predefined values */ 00076 /* from file l6474_target_config.h*/ 00077 BSP_MotorControl_Init(BSP_MOTOR_CONTROL_BOARD_ID_L6474, 1); 00078 00079 /* Attach the function MyFlagInterruptHandler (defined below) to the flag interrupt */ 00080 BSP_MotorControl_AttachFlagInterrupt(MyFlagInterruptHandler); 00081 00082 /* Attach the function Error_Handler (defined below) to the error Handler*/ 00083 BSP_MotorControl_AttachErrorHandler(Error_Handler); 00084 00085 //----- Move of 16000 steps in the FW direction 00086 00087 /* Move device 0 of 16000 steps in the FORWARD direction*/ 00088 BSP_MotorControl_Move(0, FORWARD, 16000); 00089 00090 /* Wait for the motor of device 0 ends moving */ 00091 BSP_MotorControl_WaitWhileActive(0); 00092 00093 /* Wait for 2 seconds */ 00094 HAL_Delay(2000); 00095 00096 //----- Move of 16000 steps in the BW direction 00097 00098 /* Move device 0 of 16000 steps in the BACKWARD direction*/ 00099 BSP_MotorControl_Move(0, BACKWARD, 16000); 00100 00101 /* Wait for the motor of device 0 ends moving */ 00102 BSP_MotorControl_WaitWhileActive(0); 00103 00104 /* Set the current position of device 0 to be the Home position */ 00105 BSP_MotorControl_SetHome(0); 00106 00107 /* Wait for 2 seconds */ 00108 HAL_Delay(2000); 00109 00110 //----- Go to position -6400 00111 00112 /* Request device 0 to go to position -6400 */ 00113 BSP_MotorControl_GoTo(0,-6400); 00114 00115 /* Wait for the motor ends moving */ 00116 BSP_MotorControl_WaitWhileActive(0); 00117 00118 /* Get current position of device 0*/ 00119 pos = BSP_MotorControl_GetPosition(0); 00120 00121 if (pos != -6400) 00122 { 00123 Error_Handler(11); 00124 } 00125 00126 /* Set the current position of device 0 to be the Mark position */ 00127 BSP_MotorControl_SetMark(0); 00128 00129 /* Wait for 2 seconds */ 00130 HAL_Delay(2000); 00131 00132 //----- Go Home 00133 00134 /* Request device 0 to go to Home */ 00135 BSP_MotorControl_GoHome(0); 00136 BSP_MotorControl_WaitWhileActive(0); 00137 00138 /* Get current position of device 0 */ 00139 pos = BSP_MotorControl_GetPosition(0); 00140 00141 /* Wait for 2 seconds */ 00142 HAL_Delay(2000); 00143 00144 //----- Go to position 6400 00145 00146 /* Request device 0 to go to position 6400 */ 00147 BSP_MotorControl_GoTo(0,6400); 00148 00149 /* Wait for the motor of device 0 ends moving */ 00150 BSP_MotorControl_WaitWhileActive(0); 00151 00152 /* Get current position of device 0*/ 00153 pos = BSP_MotorControl_GetPosition(0); 00154 00155 /* Wait for 2 seconds */ 00156 HAL_Delay(2000); 00157 00158 //----- Go Mark which was set previously after go to -6400 00159 00160 /* Request device 0 to go to Mark position */ 00161 BSP_MotorControl_GoMark(0); 00162 00163 /* Wait for the motor of device 0 ends moving */ 00164 BSP_MotorControl_WaitWhileActive(0); 00165 00166 /* Get current position of device 0 */ 00167 pos = BSP_MotorControl_GetPosition(0); 00168 00169 /* Wait for 2 seconds */ 00170 HAL_Delay(2000); 00171 00172 //----- Run the motor BACKWARD 00173 00174 /* Request device 0 to run BACKWARD */ 00175 BSP_MotorControl_Run(0,BACKWARD); 00176 HAL_Delay(5000); 00177 00178 /* Get current speed of device 0 */ 00179 mySpeed = BSP_MotorControl_GetCurrentSpeed(0); 00180 00181 //----- Increase the speed while running 00182 00183 /* Increase speed of device 0 to 2400 step/s */ 00184 BSP_MotorControl_SetMaxSpeed(0,4800); 00185 HAL_Delay(9000); 00186 00187 /* Get current speed of device 0 */ 00188 mySpeed = BSP_MotorControl_GetCurrentSpeed(0); 00189 00190 //----- Decrease the speed while running 00191 00192 /* Decrease speed of device 0 to 1200 step/s */ 00193 BSP_MotorControl_SetMaxSpeed(0,1200); 00194 HAL_Delay(5000); 00195 00196 /* Get current speed */ 00197 mySpeed = BSP_MotorControl_GetCurrentSpeed(0); 00198 00199 //----- Increase acceleration while running 00200 00201 /* Increase acceleration of device 0 to 480 step/s^2 */ 00202 BSP_MotorControl_SetAcceleration(0,480); 00203 HAL_Delay(5000); 00204 00205 /* Increase speed of device 0 to 2400 step/s */ 00206 BSP_MotorControl_SetMaxSpeed(0,2400); 00207 HAL_Delay(5000); 00208 00209 /* Get current speed of device 0 */ 00210 mySpeed = BSP_MotorControl_GetCurrentSpeed(0); 00211 00212 if (mySpeed != 2400) 00213 { 00214 Error_Handler(10); 00215 } 00216 //----- Increase deceleration while running 00217 00218 /* Increase deceleration of device 0 to 480 step/s^2 */ 00219 BSP_MotorControl_SetDeceleration(0,480); 00220 HAL_Delay(5000); 00221 00222 /* Decrease speed of device 0 to 1200 step/s */ 00223 BSP_MotorControl_SetMaxSpeed(0,1200); 00224 HAL_Delay(5000); 00225 00226 /* Get current speed */ 00227 mySpeed = BSP_MotorControl_GetCurrentSpeed(0); 00228 00229 //----- Soft stopped required while running 00230 00231 /* Request soft stop of device 0 */ 00232 BSP_MotorControl_SoftStop(0); 00233 00234 /* Wait for the motor of device 0 ends moving */ 00235 BSP_MotorControl_WaitWhileActive(0); 00236 00237 /* Wait for 2 seconds */ 00238 HAL_Delay(2000); 00239 00240 //----- Run stopped by hardstop 00241 00242 /* Request device 0 to run in FORWARD direction */ 00243 BSP_MotorControl_Run(0,FORWARD); 00244 HAL_Delay(5000); 00245 00246 /* Request device 0 to immediatly stop */ 00247 BSP_MotorControl_HardStop(0); 00248 BSP_MotorControl_WaitWhileActive(0); 00249 00250 /* Wait for 2 seconds */ 00251 HAL_Delay(2000); 00252 00253 //----- GOTO stopped by softstop 00254 00255 /* Request device 0 to go to position 20000 */ 00256 BSP_MotorControl_GoTo(0,20000); 00257 HAL_Delay(5000); 00258 00259 /* Request device 0 to perform a soft stop */ 00260 BSP_MotorControl_SoftStop(0); 00261 BSP_MotorControl_WaitWhileActive(0); 00262 00263 /* Wait for 2 seconds */ 00264 HAL_Delay(2000); 00265 00266 //----- Read inexistent register to test MyFlagInterruptHandler 00267 00268 /* Try to read an inexistent register */ 00269 /* the flag interrupt should be raised */ 00270 /* and the MyFlagInterruptHandler function called */ 00271 BSP_MotorControl_CmdGetParam(0,0x1F); 00272 HAL_Delay(500); 00273 00274 //----- Change step mode to full step mode 00275 00276 /* Select full step mode for device 0 */ 00277 BSP_MotorControl_SelectStepMode(0,STEP_MODE_FULL); 00278 00279 /* Set speed and acceleration to be consistent with full step mode */ 00280 BSP_MotorControl_SetMaxSpeed(0,100); 00281 BSP_MotorControl_SetMinSpeed(0,50); 00282 BSP_MotorControl_SetAcceleration(0,10); 00283 BSP_MotorControl_SetDeceleration(0,10); 00284 00285 /* Request device 0 to go position 200 */ 00286 BSP_MotorControl_GoTo(0,200); 00287 00288 /* Wait for the motor of device 0 ends moving */ 00289 BSP_MotorControl_WaitWhileActive(0); 00290 00291 /* Get current position */ 00292 pos = BSP_MotorControl_GetPosition(0); 00293 00294 /* Wait for 2 seconds */ 00295 HAL_Delay(2000); 00296 00297 //----- Restore 1/16 microstepping mode 00298 00299 /* Reset device 0 to 1/16 microstepping mode */ 00300 BSP_MotorControl_SelectStepMode(0,STEP_MODE_1_16); //_FULL _HALF 1_16 00301 00302 /* Update speed, acceleration, deceleration for 1/16 microstepping mode*/ 00303 BSP_MotorControl_SetMaxSpeed(0,40000); 00304 BSP_MotorControl_SetMinSpeed(0,800); 00305 BSP_MotorControl_SetAcceleration(0,160); 00306 BSP_MotorControl_SetDeceleration(0,160); 00307 00308 /* Infinite loop */ 00309 while(1) 00310 { 00311 /* Request device 0 to go position -6400 */ 00312 BSP_MotorControl_GoTo(0,-6400); 00313 00314 /* Wait for the motor of device 0 ends moving */ 00315 BSP_MotorControl_WaitWhileActive(0); 00316 00317 /* Request device 0 to go position 6400 */ 00318 BSP_MotorControl_GoTo(0,6400); 00319 00320 /* Wait for the motor of device 0 ends moving */ 00321 BSP_MotorControl_WaitWhileActive(0); 00322 } 00323 } 00324 00325 /** 00326 * @brief This function is the User handler for the flag interrupt 00327 * @param None 00328 * @retval None 00329 */ 00330 void MyFlagInterruptHandler(void) 00331 { 00332 /* Get the value of the status register via the L6474 command GET_STATUS */ 00333 uint16_t statusRegister = BSP_MotorControl_CmdGetStatus(0); 00334 00335 /* Check HIZ flag: if set, power brigdes are disabled */ 00336 if ((statusRegister & L6474_STATUS_HIZ) == L6474_STATUS_HIZ) 00337 { 00338 // HIZ state 00339 // Action to be customized 00340 } 00341 00342 /* Check direction bit */ 00343 if ((statusRegister & L6474_STATUS_DIR) == L6474_STATUS_DIR) 00344 { 00345 // Forward direction is set 00346 // Action to be customized 00347 } 00348 else 00349 { 00350 // Backward direction is set 00351 // Action to be customized 00352 } 00353 00354 /* Check NOTPERF_CMD flag: if set, the command received by SPI can't be performed */ 00355 /* This often occures when a command is sent to the L6474 */ 00356 /* while it is in HIZ state */ 00357 if ((statusRegister & L6474_STATUS_NOTPERF_CMD) == L6474_STATUS_NOTPERF_CMD) 00358 { 00359 // Command received by SPI can't be performed 00360 // Action to be customized 00361 } 00362 00363 /* Check WRONG_CMD flag: if set, the command does not exist */ 00364 if ((statusRegister & L6474_STATUS_WRONG_CMD) == L6474_STATUS_WRONG_CMD) 00365 { 00366 //command received by SPI does not exist 00367 // Action to be customized 00368 } 00369 00370 /* Check UVLO flag: if not set, there is an undervoltage lock-out */ 00371 if ((statusRegister & L6474_STATUS_UVLO) == 0) 00372 { 00373 //undervoltage lock-out 00374 // Action to be customized 00375 } 00376 00377 /* Check TH_WRN flag: if not set, the thermal warning threshold is reached */ 00378 if ((statusRegister & L6474_STATUS_TH_WRN) == 0) 00379 { 00380 //thermal warning threshold is reached 00381 // Action to be customized 00382 } 00383 00384 /* Check TH_SHD flag: if not set, the thermal shut down threshold is reached */ 00385 if ((statusRegister & L6474_STATUS_TH_SD) == 0) 00386 { 00387 //thermal shut down threshold is reached 00388 // Action to be customized 00389 } 00390 00391 /* Check OCD flag: if not set, there is an overcurrent detection */ 00392 if ((statusRegister & L6474_STATUS_OCD) == 0) 00393 { 00394 //overcurrent detection 00395 // Action to be customized 00396 } 00397 00398 } 00399 00400 /** 00401 * @brief This function is executed in case of error occurrence. 00402 * @param error number of the error 00403 * @retval None 00404 */ 00405 void Error_Handler(uint16_t error) 00406 { 00407 /* Backup error number */ 00408 gLastError = error; 00409 00410 /* Infinite loop */ 00411 while(1) 00412 { 00413 } 00414 } 00415 00416 #ifdef USE_FULL_ASSERT 00417 00418 /** 00419 * @brief Reports the name of the source file and the source line number 00420 * where the assert_param error has occurred. 00421 * @param file: pointer to the source file name 00422 * @param line: assert_param error line source number 00423 * @retval None 00424 */ 00425 void assert_failed(uint8_t* file, uint32_t line) 00426 { 00427 /* User can add his own implementation to report the file name and line number, 00428 ex: printf("Wrong parameters value: file %s on line %d\r\n", file, line) */ 00429 00430 /* Infinite loop */ 00431 while (1) 00432 { 00433 } 00434 } 00435 #endif 00436 00437 /** 00438 * @} 00439 */ 00440 00441 00442 /************************ (C) COPYRIGHT STMicroelectronics *****END OF FILE****/
Generated on Tue Jul 12 2022 22:53:31 by
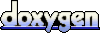