
KL25Z RGB LED fade demo using the HSI2RGBW_PWM libary
Dependencies: HSI2RGBW_PWM mbed
Fork of KL25Z_HSI2RGBW_PWM by
main.cpp
00001 /* 00002 *************************************************** 00003 * Code ported from http://saikoled.com * 00004 * Licensed under GPL3 * 00005 * Created 14 February 2013 * 00006 * Original MCU board : Arduino Leonardo * 00007 * Original CPU : ATmega32u4 * 00008 * Copyright 2013, Brian Neltner * 00009 *************************************************** 00010 00011 Sources 00012 ------- 00013 https://github.com/saikoLED/MyKi/blob/master/myki_16_bit_random_fade/myki_16_bit_random_fade.ino 00014 https://github.com/saikoLED/MyKi/blob/master/myki_16_bit_fade/myki_16_bit_fade.ino 00015 Above files are combined into this file using defines. 00016 00017 http://blog.saikoled.com/post/44677718712/how-to-convert-from-hsi-to-rgb-white 00018 00019 Other demos : Psychadelic demo 00020 DO NOT TRY THIS IF YOU ARE EPILEPTIC! THESE FREQUENCIES ARE EVEN WORSE THAN MOST STROBING LIGHTS! 00021 http://blog.saikoled.com/post/45760195354/generating-vivid-geometric-hallucinations-using-flicker 00022 00023 This software implements 4x 16-bit PWM with a filtered fading algorithm 00024 in HSI color space and HSI -> RGBW conversion to allow for better 00025 pastel color. 00026 */ 00027 00028 #include "mbed.h" 00029 #include "hsi2rgbw_pwm.h" 00030 00031 #define RANDOM_FADE // Disable this define to set Normal fade. 00032 00033 // Constants 00034 #define steptime 1 // Color transition time in ms. 00035 #define maxsaturation 1.0 00036 00037 #ifdef RANDOM_FADE 00038 #define propgain 0.0005 // "Small Constant" 00039 #define minsaturation 0.9 00040 #else 00041 #define hue_increment 0.01 00042 #endif 00043 00044 Serial pc(USBTX, USBRX); 00045 00046 // HSI to RGBW conversion with direct output to PWM channels 00047 hsi2rgbw_pwm led(LED_RED, LED_GREEN, LED_BLUE); 00048 00049 struct HSI { 00050 float h; 00051 float s; 00052 float i; 00053 #ifdef RANDOM_FADE 00054 float htarget; 00055 float starget; 00056 #endif 00057 } color; 00058 00059 void updatehue() { 00060 #ifdef RANDOM_FADE 00061 color.htarget += ((rand()%360)-180)*.1; 00062 color.htarget = fmod(color.htarget, 360); 00063 color.h += propgain*(color.htarget-color.h); 00064 color.h = fmod(color.h, 360); 00065 #else 00066 color.h = color.h + hue_increment; 00067 if(color.h > 360) 00068 color.h = 0; 00069 #endif 00070 } 00071 00072 void updatesaturation() { 00073 #ifdef RANDOM_FADE 00074 color.starget += ((rand()%10000)-5000)/0.00001; 00075 if (color.starget > maxsaturation) color.starget = maxsaturation; 00076 else if (color.starget < minsaturation) color.starget = minsaturation; 00077 color.s += propgain*(color.starget-color.s); 00078 if (color.s > maxsaturation) color.s = maxsaturation; 00079 else if (color.s < minsaturation) color.s = minsaturation; 00080 #else 00081 color.s = maxsaturation; 00082 #endif 00083 } 00084 00085 void primary_colors() { 00086 printf("briefly show RED - GREEN - BLUE using hsi2rgbw.\r\n"); 00087 color.s = 1; 00088 color.i = 1; 00089 for (color.h = 0 ; color.h < 360 ; color.h += 120) 00090 { 00091 led.hsi2rgbw(color.h, color.s, color.i); 00092 wait(1); 00093 } 00094 } 00095 00096 void cycle_rgbw() { 00097 float rgbw[4] = {0,0,0,0}; 00098 color.s = 1; 00099 color.i = 1; 00100 printf("briefly show RED - GREEN - BLUE using direct PWM output.\r\n"); 00101 rgbw[0] = 1.0f; 00102 led.pwm(rgbw); 00103 wait(1); 00104 rgbw[0] = 0.0f; 00105 rgbw[1] = 1.0f; 00106 led.pwm(rgbw); 00107 wait(1); 00108 rgbw[1] = 0.0f; 00109 rgbw[2] = 1.0f; 00110 led.pwm(rgbw); 00111 wait(1); 00112 } 00113 00114 void sendcolor() { 00115 while (color.h >=360) color.h = color.h - 360; 00116 while (color.h < 0) color.h = color.h + 360; 00117 if (color.i > 1) color.i = 1; 00118 if (color.i < 0) color.i = 0; 00119 if (color.s > 1) color.s = 1; 00120 if (color.s < 0) color.s = 0; 00121 // Fix ranges (somewhat redundantly). 00122 led.hsi2rgbw(color.h, color.s, color.i); 00123 } 00124 00125 void setup() { 00126 led.invertpwm(1); //KL25Z RGB LED uses common anode. 00127 color.h = 0; 00128 color.s = maxsaturation; 00129 color.i = 0; 00130 #ifdef RANDOM_FADE 00131 color.htarget = 0; 00132 color.starget = maxsaturation; 00133 #endif 00134 00135 // Initial color = off, hue of red fully saturated. 00136 while (color.i < 1) { 00137 sendcolor(); 00138 color.i = color.i + 0.001; // Increase Intensity 00139 updatehue(); 00140 updatesaturation(); 00141 wait_ms(steptime); 00142 } 00143 } 00144 00145 int main() { 00146 pc.baud (115200); 00147 printf("HSI to RGB random fade.\r\n"); 00148 printf("Initialize.\r\n"); 00149 00150 setup(); 00151 primary_colors(); 00152 cycle_rgbw(); 00153 printf("Run.\r\n"); 00154 while(1) { 00155 sendcolor(); 00156 updatehue(); 00157 updatesaturation(); 00158 wait_ms(steptime); 00159 } 00160 }
Generated on Thu Jul 14 2022 05:29:26 by
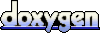