
Sample code on how to pass an optional function pointer to a library and set a single pin to either InterruptIn or DigitalOut.
DemoClass.h
00001 #ifndef DemoClass_H 00002 #define DemoClass_H 00003 00004 #include "mbed.h" 00005 00006 class DemoClass { 00007 public: 00008 /** 00009 * \brief Create an object connected to I2C bus, irq input or digital output and user-ISR. 00010 * \param sda SDA pin. 00011 * \param scl SCL pin. 00012 * \param irqsync Interrupt input when called with user-ISR pointer. 00013 * Digital output when called without user-ISR pointer. 00014 * \param fptr Pointer to user-ISR. 00015 * \return none 00016 */ 00017 00018 DemoClass(PinName sda, PinName scl, PinName irqsync = NC, void (*fptr)(void) = NULL); 00019 00020 /** 00021 * \brief Example status function. 00022 * \param none. 00023 * \return Always 1. 00024 */ 00025 bool Status(void); 00026 00027 private: 00028 I2C _i2c; 00029 FunctionPointer _fptr; 00030 void _sensorISR(void); 00031 }; 00032 00033 #endif
Generated on Wed Jul 13 2022 02:31:25 by
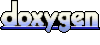