
Demo apps : receive a string from a client and respond with a different string, TCP/IP client
Dependencies: CC3000_Hostdriver mbed
main.cpp
00001 #include "mbed.h" 00002 #include "doTCPIP.h" 00003 00004 00005 // Serial USB port 00006 Serial pc(USBTX, USBRX); 00007 00008 //Wi-Go battery charger control 00009 DigitalOut PWR_EN1(PTB2); 00010 DigitalOut PWR_EN2(PTB3); 00011 00012 int main() 00013 { 00014 char c; 00015 // set current to 500mA since we're turning on the Wi-Fi 00016 PWR_EN1 = 0; 00017 PWR_EN2 = 1; 00018 00019 //Set baudrate to 115200 instead of the default 9600 00020 pc.baud (115200); 00021 00022 initLEDs(); 00023 Init_HostDriver(); 00024 runSmartConfig = 0; 00025 ulSmartConfigFinished = 0; 00026 // server_running = 1; 00027 newData = 0; 00028 socket_active_status = 0xFFFF; 00029 ForceFixedSSID = 0; 00030 00031 GREEN_ON; 00032 00033 // Trigger a WLAN device 00034 wlan_start(0); 00035 nvmem_read( NVMEM_USER_FILE_1_FILEID, sizeof(userFS), 0, (unsigned char *) &userFS); 00036 nvmem_get_mac_address(myMAC); 00037 printf("\x1B[2J"); //VT100 erase screen 00038 printf("\x1B[H"); //VT100 home 00039 printf("CC3000 Python demo.\n"); 00040 print_mac(); 00041 wlan_stop(); 00042 printf("FTC %i\n",userFS.FTC); 00043 printf("PP_version %i.%i\n",userFS.PP_version[0], userFS.PP_version[1]); 00044 printf("SERV_PACK %i.%i\n",userFS.SERV_PACK[0], userFS.SERV_PACK[1]); 00045 printf("DRV_VER %i.%i.%i\n",userFS.DRV_VER[0], userFS.DRV_VER[1], userFS.DRV_VER[2]); 00046 printf("FW_VER %i.%i.%i\n",userFS.FW_VER[0], userFS.FW_VER[1], userFS.FW_VER[2]); 00047 00048 printf("\n<0> Normal run. SmartConfig will\n start if no valid connection exists.\n"); 00049 printf("<1> Connect using fixed SSID : %s\n", SSID); 00050 printf("<2> TCP/IP client:\n Discover public IP address.\n Get time and date from a daytime server in Italy.\n"); 00051 printf("<9> SmartConfig.\n"); 00052 c = getchar(); 00053 switch (c) 00054 { 00055 case '0': 00056 ForceFixedSSID = 0; 00057 if(!userFS.FTC) 00058 { 00059 do_FTC(); 00060 wlan_stop(); 00061 printf("\nPress any key to run the socket demo...\n"); 00062 getchar(); 00063 } 00064 break; 00065 case '1': 00066 ForceFixedSSID = 1; 00067 break; 00068 case '2': 00069 SmartConfigProfilestored = SMART_CONFIG_SET; 00070 // Run TCP/IP Client connection demo. 00071 // Discover public IP address 00072 runTCPIPclient( SRV_IP_OCT1, SRV_IP_OCT2, SRV_IP_OCT3, SRV_IP_OCT4, SRV_PORT); 00073 wait(3); 00074 // Take date and time fomr daytime server (well know port 13) 00075 runTCPIPclient( TMSRV_IP_OCT1, TMSRV_IP_OCT2, TMSRV_IP_OCT3, TMSRV_IP_OCT4, TMSRV_PORT); 00076 printf("Finished - Press reset to restart.\n"); 00077 while(1); 00078 case '9': 00079 ForceFixedSSID = 0; 00080 // server_running = 1; 00081 runSmartConfig = 1; 00082 initTCPIP(); 00083 // server_running = 1; 00084 RED_OFF; 00085 GREEN_OFF; 00086 BLUE_OFF; 00087 printf("Press the reset button on your board........\n"); 00088 while(1) 00089 { 00090 GREEN_ON; 00091 wait_ms(500); 00092 GREEN_OFF; 00093 wait_ms(500); 00094 } 00095 default: 00096 printf("Wrong selection.\n"); 00097 printf("Reset the board and try again.\n"); 00098 } 00099 // server_running = 0; 00100 SmartConfigProfilestored = SMART_CONFIG_SET; 00101 RED_OFF; 00102 GREEN_OFF; 00103 BLUE_OFF; 00104 runTCPIPserver(); // Run TCP/IP Connection to host 00105 }
Generated on Wed Jul 13 2022 08:35:23 by
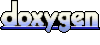