
Demo apps : receive a string from a client and respond with a different string, TCP/IP client
Dependencies: CC3000_Hostdriver mbed
doTCPIP.h
00001 /**************************************************************************** 00002 * 00003 * doTCPIP.h - CC3000 TCP/IP 00004 * Copyright (C) 2011 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions 00008 * are met: 00009 * 00010 * Redistributions of source code must retain the above copyright 00011 * notice, this list of conditions and the following disclaimer. 00012 * 00013 * Redistributions in binary form must reproduce the above copyright 00014 * notice, this list of conditions and the following disclaimer in the 00015 * documentation and/or other materials provided with the 00016 * distribution. 00017 * 00018 * Neither the name of Texas Instruments Incorporated nor the names of 00019 * its contributors may be used to endorse or promote products derived 00020 * from this software without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00023 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00024 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00025 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00026 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00027 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00028 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00029 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00030 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00031 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 *****************************************************************************/ 00035 00036 #ifndef DOTCPIP_H 00037 #define DOTCPIP_H 00038 00039 #include "cc3000.h" 00040 00041 /** TCP/IP Functions 00042 * 00043 */ 00044 #ifdef __cplusplus 00045 extern "C" { 00046 #endif 00047 00048 #ifndef FALSE 00049 #define FALSE 0 00050 #endif 00051 00052 #ifndef TRUE 00053 #define TRUE 1 00054 #endif 00055 00056 #define SMART_CONFIG_SET 0x55 00057 // SMTP Specific 00058 #define HTTP_PORT 80 00059 #define TCPIP_PORT 15000 00060 00061 #define USE_DHCP 1 00062 #define USE_STATIC_IP 2 00063 00064 #define NONE 0 00065 #define WEP 1 00066 #define WPA 2 00067 #define WPA2 3 00068 00069 // Modify the following settings as necessary for your Wi-Fi Network setup: 00070 #define IP_ALLOC_METHOD USE_DHCP // for DHCP assigned IP address 00071 //#define IP_ALLOC_METHOD USE_STATIC_IP // for static IP address 00072 00073 // Default SSID Settings 00074 //#define AP_KEY "thisthis" 00075 //#define AP_SECURITY WPA2 // WPA2 must be enabled for use with iPhone or Android phone hotspot! 00076 #define SSID "iot" 00077 #define AP_SECURITY NONE // no security but will connect quicker! 00078 #define STATIC_IP_OCT1 192 00079 #define STATIC_IP_OCT2 168 00080 #define STATIC_IP_OCT3 0 00081 #define STATIC_IP_OCT4 10 00082 00083 #define STATIC_GW_OCT4 1 // Static Gateway address = STATIC_IP_OCT1.STATIC_IP_OCT2.STATIC_IP_OCT3.STATIC_GW_OCT4 00084 00085 #define REQ_BUFFER_SIZE 400 00086 00087 // DynDNS server. From which to take public IP address. 00088 // dyndns: 91.198.22.70 00089 #define SRV_IP_OCT1 91 00090 #define SRV_IP_OCT2 198 00091 #define SRV_IP_OCT3 22 00092 #define SRV_IP_OCT4 70 00093 #define SRV_PORT 80 00094 00095 // Italian daytime server responding: 00096 // 29 AUG 2013 05:24:02 CEST 00097 // tempo.ien.it [193.204.114.105] 00098 #define TMSRV_IP_OCT1 193 00099 #define TMSRV_IP_OCT2 204 00100 #define TMSRV_IP_OCT3 114 00101 #define TMSRV_IP_OCT4 105 00102 #define TMSRV_PORT 13 00103 00104 typedef struct { 00105 unsigned char FTC; // First time config performed 00106 unsigned char PP_version[2]; // Patch Programmer version 00107 unsigned char SERV_PACK[2]; // Service Pack Version 00108 unsigned char DRV_VER[3]; // Driver Version 00109 unsigned char FW_VER[3]; // Firmware Version 00110 unsigned char validCIK; // CIK[] is valid (Client Interface Key) 00111 unsigned char CIK[40]; 00112 } userFS_t; 00113 00114 extern userFS_t userFS; 00115 00116 extern volatile unsigned long ulSmartConfigFinished,ulCC3000DHCP, OkToDoShutDown, ulCC3000DHCP_configured; 00117 extern int server_running; 00118 extern volatile unsigned char newData; 00119 extern unsigned char ForceFixedSSID; 00120 extern char runSmartConfig; 00121 extern char requestBuffer[]; 00122 extern unsigned char myMAC[8]; 00123 extern unsigned char SmartConfigProfilestored; 00124 extern int do_mDNS; 00125 extern int HsecondFlag; 00126 extern unsigned int seconds; 00127 extern volatile int ms5Flag; 00128 00129 extern void StartSmartConfig(void); 00130 00131 00132 //int sendTCPIP(int port); 00133 void sendPython(int port); 00134 void initTCPIP(void); 00135 void runTCPIPserver(void); 00136 int getTCPIP( char a1, char a2, char a3, char a4, int port); 00137 //void runTCPIPclient(int a1, int a2, int a3, int a4); 00138 void runTCPIPclient(int a1, int a2, int a3, int a4, int port); 00139 00140 /** Checks if WiFi is still connected. 00141 * @param None 00142 * @return TRUE if connected, FALSE if not 00143 * @note If not associated with an AP for 5 consecutive retries, it will reset the board. 00144 */ 00145 unsigned char checkWiFiConnected(void); 00146 00147 /** Print MAC address. 00148 * @param None 00149 * @return none 00150 */ 00151 void print_mac(void); 00152 00153 /** First time configuration. 00154 * @param None 00155 * @return none 00156 */ 00157 void do_FTC(void); 00158 00159 #ifdef __cplusplus 00160 } 00161 #endif // __cplusplus 00162 00163 #endif // DOTCPIP_H 00164
Generated on Wed Jul 13 2022 08:35:23 by
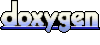