
Demo apps : receive a string from a client and respond with a different string, TCP/IP client
Dependencies: CC3000_Hostdriver mbed
doTCPIP.cpp
00001 /**************************************************************************** 00002 * 00003 * doTCPIP.cpp - CC3000 TCP/IP 00004 * Copyright (C) 2011 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions 00008 * are met: 00009 * 00010 * Redistributions of source code must retain the above copyright 00011 * notice, this list of conditions and the following disclaimer. 00012 * 00013 * Redistributions in binary form must reproduce the above copyright 00014 * notice, this list of conditions and the following disclaimer in the 00015 * documentation and/or other materials provided with the 00016 * distribution. 00017 * 00018 * Neither the name of Texas Instruments Incorporated nor the names of 00019 * its contributors may be used to endorse or promote products derived 00020 * from this software without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00023 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00024 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00025 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00026 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00027 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00028 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00029 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00030 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00031 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 *****************************************************************************/ 00035 00036 #include "doTCPIP.h" 00037 00038 volatile unsigned char newData; 00039 int server_running; 00040 unsigned char ForceFixedSSID; 00041 char runSmartConfig; // Flag indicating whether user requested to perform Smart Config 00042 volatile unsigned long ulCC3000Connected; 00043 unsigned char ConnectUsingSmartConfig; 00044 unsigned char myMAC[8]; 00045 userFS_t userFS; 00046 int do_mDNS = 0; 00047 00048 // Setup the functions to handle our CGI parameters 00049 tNetappIpconfigRetArgs ipinfo2; 00050 char requestBuffer[REQ_BUFFER_SIZE]; 00051 int LAN_Connected = 0; 00052 00053 unsigned char SmartConfigProfilestored = 0xff; 00054 00055 00056 /** \brief Flag indicating whether to print CC3000 Connection info */ 00057 static unsigned char obtainIpInfoFlag = FALSE; 00058 //Device name - used for Smart config in order to stop the Smart phone configuration process 00059 char DevServname[] = "CC3000"; 00060 volatile unsigned long SendmDNSAdvertisment; 00061 00062 /*int sendTCPIP(int port) 00063 { 00064 long temp, stat, skip, skipc; 00065 //socket descriptor 00066 long sock; 00067 //new TCP socket descriptor 00068 long newsock; 00069 //destination address 00070 sockaddr destAddr; 00071 //local address 00072 sockaddr LocalAddr; 00073 00074 // char buf2[40]; 00075 socklen_t addrlen; 00076 memset(&LocalAddr, 0, 8); 00077 LocalAddr.sa_family = AF_INET; 00078 LocalAddr.sa_data[0] = (port >> 8) & 0xff; 00079 LocalAddr.sa_data[1] = port & 0xff; 00080 memset (&LocalAddr.sa_data[2], 0, 4); 00081 sock = socket(AF_INET, SOCK_STREAM, IPPROTO_TCP); 00082 while(sock == -1) sock = socket(AF_INET, SOCK_DGRAM, IPPROTO_TCP); 00083 temp = bind(sock,&LocalAddr,sizeof(sockaddr)); 00084 temp = listen(sock, 1); 00085 addrlen = sizeof(destAddr); 00086 skip = 0; 00087 skipc = 0; 00088 while(1) 00089 { 00090 newsock = -2; 00091 printf("Server waiting for connection\n"); 00092 LED_D2_ON; 00093 while((newsock == -1) || (newsock == -2)) 00094 { 00095 newsock = accept(sock,&destAddr, &addrlen); 00096 if(do_mDNS) 00097 { 00098 printf("mDNS= 0x%08x\n", mdnsAdvertiser(1, DevServname, sizeof(DevServname))); 00099 do_mDNS = 0; 00100 } 00101 } 00102 printf("Connected\n"); 00103 // sss = seconds; 00104 //receive TCP data 00105 temp = 0; 00106 if(newsock >= 0) 00107 { 00108 recv(newsock, requestBuffer,20,0); 00109 printf("Input = %s\n", requestBuffer); 00110 while(1) 00111 { 00112 while(!newData) __wfi(); 00113 newData = 0; 00114 LED_D2_ON; 00115 stat = -2; 00116 //if(tSLInformation.usNumberOfFreeBuffers <= 2) 00117 //{ 00118 // printf("LOW= %d\n", tSLInformation.usNumberOfFreeBuffers); 00119 //} 00120 //Disable sys tick interrupts for debugging 00121 //SysTick->CTRL &= ~SysTick_CTRL_TICKINT_Msk; // *** Disable SysTick Timer Interrupt 00122 // stat = send(newsock,&axis6, sizeof(axis6), 0); 00123 // Disable sys tick interrupts for debugging 00124 //SysTick->CTRL |= SysTick_CTRL_TICKINT_Msk; // *** Enable SysTick Timer Interrupt 00125 LED_D2_OFF; 00126 if(stat != 96) 00127 { 00128 if(stat == -2) 00129 { 00130 skip++; 00131 skipc++; 00132 } 00133 //printf("status= %d\n", stat); 00134 if(stat == -1) break; 00135 } 00136 else 00137 { 00138 temp++; 00139 skipc = 0; 00140 } 00141 if(skipc > 150) 00142 { 00143 // printf("Zero Buffer Error Sent\n", temp, seconds - sss); 00144 return(-1); 00145 } 00146 } 00147 } else printf("bad socket= %d\n", newsock); 00148 closesocket(newsock); 00149 // printf("Done %d, time= %d, skipped= %d\n", temp, seconds - sss, skip); 00150 skip = 0; 00151 } 00152 }*/ 00153 00154 void sendPython(int port) 00155 { 00156 char python_msg[] = "Hello Python\n"; 00157 int stat; 00158 long sock; 00159 //new TCP socket descriptor 00160 long newsock; 00161 //destination address 00162 sockaddr destAddr; 00163 //local address 00164 sockaddr LocalAddr; 00165 socklen_t addrlen; 00166 memset(&LocalAddr, 0, 8); 00167 LocalAddr.sa_family = AF_INET; 00168 LocalAddr.sa_data[0] = (port >> 8) & 0xff; 00169 LocalAddr.sa_data[1] = port & 0xff; 00170 memset (&LocalAddr.sa_data[2], 0, 4); 00171 sock = socket(AF_INET, SOCK_STREAM, IPPROTO_TCP); 00172 while(sock == -1) sock = socket(AF_INET, SOCK_DGRAM, IPPROTO_TCP); 00173 bind(sock,&LocalAddr,sizeof(sockaddr)); 00174 listen(sock, 1); 00175 addrlen = sizeof(destAddr); 00176 while(1) 00177 { 00178 newsock = -2; 00179 printf("\nServer waiting for connection to Python\n"); 00180 LED_D2_ON; 00181 while((newsock == -1) || (newsock == -2)) 00182 { 00183 newsock = accept(sock,&destAddr, &addrlen); 00184 } 00185 printf("Connected\n"); 00186 //receive TCP data 00187 if(newsock >= 0) 00188 { 00189 stat = recv(newsock, requestBuffer,20,0); 00190 if(stat > 0) 00191 { 00192 printf("Receive Status= %d, Input = %s\n", stat, requestBuffer); 00193 stat = -2; 00194 stat = send(newsock, python_msg, strlen(python_msg), 0); 00195 printf("Send status= %d\n", stat); 00196 LED_D2_OFF; 00197 } 00198 else 00199 { 00200 printf("ERROR %d", stat); 00201 switch (stat) 00202 { 00203 case -1: 00204 { 00205 printf(": remote socket closed.\n"); 00206 break; 00207 } 00208 case -2: 00209 { 00210 printf(": no buffers available.\n"); 00211 break; 00212 } 00213 case -57: 00214 { 00215 printf(": timeout - no reply from remote.\n"); 00216 break; 00217 } 00218 default: 00219 printf("\n"); 00220 } 00221 } 00222 } else printf("bad socket= %d\n", newsock); 00223 closesocket(newsock); 00224 printf("Done, press any key to repeat\n"); 00225 getchar(); 00226 } 00227 } 00228 00229 int getTCPIP( char a1, char a2, char a3, char a4, int port) 00230 { 00231 long temp; 00232 //socket descriptor 00233 long sock; 00234 char msg1[] = "GET / HTTP/1.1\r\n\r\n\0"; 00235 //destination address 00236 sockaddr destAddr; 00237 //num of bytes received 00238 long numofbytes = 0; 00239 //data buffer 00240 char buff[256]; 00241 00242 memset(&destAddr, 0, 8); 00243 destAddr.sa_family = AF_INET; 00244 destAddr.sa_data[0] = (port >> 8) & 0xff; 00245 destAddr.sa_data[1] = port & 0xff; 00246 destAddr.sa_data[2] = a1; 00247 destAddr.sa_data[3] = a2; 00248 destAddr.sa_data[4] = a3; 00249 destAddr.sa_data[5] = a4; 00250 00251 //open socket 00252 sock = socket(AF_INET, SOCK_STREAM, IPPROTO_TCP); 00253 while(sock == -1) sock = socket(AF_INET, SOCK_DGRAM, IPPROTO_TCP); 00254 00255 //hci_unsolicited_event_handler(); 00256 00257 printf( "Client attempting connection\n"); 00258 if( connect(sock, &destAddr, sizeof(destAddr)) < 0) 00259 return(-1); 00260 00261 printf("Connected\n"); 00262 00263 //receive TCP data 00264 temp = 0; 00265 //hci_unsolicited_event_handler(); 00266 00267 send(sock, &msg1, strlen(msg1), 0); 00268 printf("Receiving Data\n"); 00269 00270 //printf("Input = %s\n", msg1); 00271 while(1) { 00272 numofbytes = recv(sock, &buff, 256,0); 00273 00274 //printf("R %d\n", numofbytes); 00275 newData = 1; 00276 if(numofbytes != -1) { 00277 closesocket(sock); 00278 printf("Done [%d]\n", temp); 00279 printf("Data: %s\n", buff); 00280 00281 //hci_unsolicited_event_handler(); 00282 00283 return(1); 00284 } 00285 temp++; 00286 } 00287 00288 return(0); // We'll never get here 00289 } 00290 00291 void initTCPIP(void) 00292 { 00293 int t; 00294 LAN_Connected = 0; 00295 // Start CC3000 State Machine 00296 resetCC3000StateMachine(); 00297 ulCC3000DHCP = 0; 00298 ulCC3000Connected = 0; 00299 // Initialize Board and CC3000 00300 initDriver(); 00301 printf("RunSmartConfig= %d\n", runSmartConfig); 00302 if(runSmartConfig == 1 ) 00303 { 00304 // Clear flag 00305 //ClearFTCflag(); 00306 unsetCC3000MachineState(CC3000_ASSOC); 00307 // Start the Smart Config Process 00308 StartSmartConfig(); 00309 runSmartConfig = 0; 00310 } 00311 // If connectivity is good, run the primary functionality 00312 while(1) 00313 { 00314 if(checkWiFiConnected()) break; 00315 wait(1); 00316 } 00317 printf("Connected\n"); 00318 if(!(currentCC3000State() & CC3000_SERVER_INIT)) 00319 { 00320 // If we're not blocked by accept or others, obtain the latest status 00321 netapp_ipconfig(&ipinfo2); // data is returned in the ipinfo2 structure 00322 } 00323 printf("\n*** Wi-Go board DHCP assigned IP Address = %d.%d.%d.%d\n", ipinfo2.aucIP[3], ipinfo2.aucIP[2], ipinfo2.aucIP[1], ipinfo2.aucIP[0]); 00324 LED_D3_ON; 00325 LAN_Connected = 1; 00326 t = mdnsAdvertiser(1, DevServname, sizeof(DevServname)); 00327 printf("mDNS Status= %x\n", t); 00328 } 00329 00330 void runTCPIPserver(void) 00331 { 00332 while(1) 00333 { 00334 LED_D3_OFF; 00335 LAN_Connected = 0; 00336 LED_D2_OFF; 00337 printf("\n\nStarting TCP/IP Server\n"); 00338 initTCPIP(); 00339 sendPython(TCPIP_PORT); 00340 // sendTCPIP(TCPIP_PORT); 00341 } 00342 } 00343 00344 /*void runTCPIPclient(int a1, int a2, int a3, int a4) 00345 { 00346 while(1) 00347 { 00348 LED_D3_OFF; 00349 LED_D2_OFF; 00350 printf("\n\nStarting TCP/IP Client connection\n"); 00351 initTCPIP(); 00352 getTCPIP( a1, a2, a3, a4, 80); // IP Address and Port of server 00353 } 00354 }*/ 00355 00356 void runTCPIPclient(int a1, int a2, int a3, int a4, int port) 00357 { 00358 int res; 00359 int i; 00360 00361 LED_D3_OFF; 00362 // LAN_Connected = 0; 00363 LED_D2_OFF; 00364 printf("\n\nStarting TCP/IP Client connection (3 attempts)\n"); 00365 // 00366 if ( LAN_Connected == 0) 00367 initTCPIP(); 00368 00369 i=0; 00370 while( i<3) { 00371 res=getTCPIP( a1, a2, a3, a4, port); // IP Address and Port of server 00372 if ( res != 1) { 00373 printf("[%d] Error connecting to: %d.%d.%d.%d:%d\n", ++i, a1, a2, a3, a4, port); 00374 } else { 00375 printf("Receiving Done. \n"); 00376 wait(1); 00377 return; 00378 } 00379 wait(3); 00380 } 00381 } 00382 00383 unsigned char checkWiFiConnected(void) 00384 { 00385 int t; 00386 if(!(currentCC3000State() & CC3000_ASSOC)) //try to associate with an Access Point 00387 { 00388 // Check whether Smart Config was run previously. If it was, we 00389 // use it to connect to an access point. Otherwise, we connect to the 00390 // default. 00391 if(((ConnectUsingSmartConfig==0)&&(SmartConfigProfilestored != SMART_CONFIG_SET)) || ForceFixedSSID) 00392 { 00393 // Smart Config not set, check whether we have an SSID 00394 // from the assoc terminal command. If not, use fixed SSID. 00395 printf("Attempting SSID Connection\n"); 00396 ConnectUsingSSID(SSID); 00397 } 00398 //unsolicicted_events_timer_init(); 00399 // Wait until connection is finished 00400 while ((ulCC3000DHCP == 0) || (ulCC3000Connected == 0)) 00401 { 00402 wait_ms(500); 00403 printf("waiting\n"); 00404 } 00405 } 00406 // Check if we are in a connected state. If so, set flags and LED 00407 if(ulCC3000Connected == 1) 00408 { 00409 if (obtainIpInfoFlag == FALSE) 00410 { 00411 obtainIpInfoFlag = TRUE; // Set flag so we don't constantly turn the LED on 00412 LED_D3_ON; 00413 } 00414 if (obtainIpInfoFlag == TRUE) 00415 { 00416 //If Smart Config was performed, we need to send complete notification to the configure (Smart Phone App) 00417 if (ConnectUsingSmartConfig==1) 00418 { 00419 ConnectUsingSmartConfig = 0; 00420 SmartConfigProfilestored = SMART_CONFIG_SET; 00421 } 00422 00423 } 00424 t = mdnsAdvertiser(1, DevServname, sizeof(DevServname)); 00425 printf("mDNS Status= %x\n", t); 00426 return TRUE; 00427 } 00428 return FALSE; 00429 } 00430 00431 void print_mac(void) 00432 { 00433 printf("\n\nWi-Go MAC address %02x:%02x:%02x:%02x:%02x:%02x\n\n", myMAC[0], myMAC[1], myMAC[2], myMAC[3], myMAC[4], myMAC[5]); 00434 } 00435 00436 void do_FTC(void) 00437 { 00438 printf("Running First Time Configuration\n"); 00439 server_running = 1; 00440 runSmartConfig = 1; 00441 initTCPIP(); 00442 RED_OFF; 00443 GREEN_OFF; 00444 BLUE_OFF; 00445 userFS.FTC = 1; 00446 nvmem_write( NVMEM_USER_FILE_1_FILEID, sizeof(userFS), 0, (unsigned char *) &userFS); 00447 runSmartConfig = 0; 00448 SmartConfigProfilestored = SMART_CONFIG_SET; 00449 wlan_stop(); 00450 printf("FTC finished\n"); 00451 } 00452 00453 00454 00455 00456
Generated on Wed Jul 13 2022 08:35:23 by
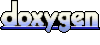