
Demo apps : receive a string from a client and respond with a different string, TCP/IP client
Dependencies: CC3000_Hostdriver mbed
cc3000.h
00001 /***************************************************************************** 00002 * 00003 * cc3000.h - CC3000 Function Definitions 00004 * Copyright (C) 2011 Texas Instruments Incorporated - http://www.ti.com/ 00005 * 00006 * Redistribution and use in source and binary forms, with or without 00007 * modification, are permitted provided that the following conditions 00008 * are met: 00009 * 00010 * Redistributions of source code must retain the above copyright 00011 * notice, this list of conditions and the following disclaimer. 00012 * 00013 * Redistributions in binary form must reproduce the above copyright 00014 * notice, this list of conditions and the following disclaimer in the 00015 * documentation and/or other materials provided with the 00016 * distribution. 00017 * 00018 * Neither the name of Texas Instruments Incorporated nor the names of 00019 * its contributors may be used to endorse or promote products derived 00020 * from this software without specific prior written permission. 00021 * 00022 * THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS 00023 * "AS IS" AND ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT 00024 * LIMITED TO, THE IMPLIED WARRANTIES OF MERCHANTABILITY AND FITNESS FOR 00025 * A PARTICULAR PURPOSE ARE DISCLAIMED. IN NO EVENT SHALL THE COPYRIGHT 00026 * OWNER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, INDIRECT, INCIDENTAL, 00027 * SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT NOT 00028 * LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, 00029 * DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY 00030 * THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT 00031 * (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE 00032 * OF THIS SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE. 00033 * 00034 *****************************************************************************/ 00035 00036 #ifndef CC3000_H 00037 #define CC3000_H 00038 00039 #include "wlan.h" 00040 00041 //***************************************************************************** 00042 // 00043 //! \addtogroup cc3000 00044 //! @{ 00045 // 00046 //***************************************************************************** 00047 00048 /** CC3000 Functions 00049 * 00050 */ 00051 #ifdef __cplusplus 00052 extern "C" { 00053 #endif 00054 00055 extern DigitalOut ledr; 00056 extern DigitalOut ledg; 00057 extern DigitalOut ledb; 00058 extern DigitalOut led1; 00059 extern DigitalOut led2; 00060 extern DigitalOut led3; 00061 00062 #define RED_OFF ledr = 1; 00063 #define RED_ON ledr = 0; 00064 #define RED_TOGGLE ledr = !ledr; 00065 #define GREEN_OFF ledg = 1; 00066 #define GREEN_ON ledg = 0; 00067 #define GREEN_TOGGLE ledg = !ledg; 00068 #define BLUE_OFF ledb = 1; 00069 #define BLUE_ON ledb = 0; 00070 #define BLUE_TOGGLE ledb = !ledb; 00071 #define LED_D1_OFF led1 = 1; 00072 #define LED_D1_ON led1 = 0; 00073 #define LED_D1_TOGGLE led1 = !led1; 00074 #define LED_D2_OFF led2 = 1; 00075 #define LED_D2_ON led2 = 0; 00076 #define LED_D2_TOGGLE led2 = !led2; 00077 #define LED_D3_OFF led3 = 1; 00078 #define LED_D3_ON led3 = 0; 00079 #define LED_D3_TOGGLE led3 = !led3; 00080 00081 #define SOCKET_INACTIVE_ERR -57 00082 #define NUM_STATES 6 00083 #define MAX_SSID_LEN 32 00084 #define FIRST_TIME_CONFIG_SET 0xAA 00085 #define NETAPP_IPCONFIG_MAC_OFFSET (20) 00086 #define CC3000_APP_BUFFER_SIZE (5) 00087 #define CC3000_RX_BUFFER_OVERHEAD_SIZE (20) 00088 00089 // CC3000 State Machine Definitions 00090 enum cc3000StateEnum 00091 { 00092 CC3000_UNINIT = 0x01, // CC3000 Driver Uninitialized 00093 CC3000_INIT = 0x02, // CC3000 Driver Initialized 00094 CC3000_ASSOC = 0x04, // CC3000 Associated to AP 00095 CC3000_IP_ALLOC = 0x08, // CC3000 has IP Address 00096 CC3000_SERVER_INIT = 0x10, // CC3000 Server Initialized 00097 CC3000_CLIENT_CONNECTED = 0x20 // CC3000 Client Connected to Server 00098 }; 00099 00100 00101 /** 00102 * Turn all LEDs Off 00103 * @param none 00104 * @return none 00105 */ 00106 void initLEDs(void); 00107 00108 /** Connect to an Access Point using the specified SSID. 00109 * @param ssidName is a string of the AP's SSID 00110 * @return none 00111 */ 00112 int ConnectUsingSSID(char * ssidName); 00113 00114 /** Handle asynchronous events from CC3000 device. 00115 * @param lEventType Event type 00116 * @param data 00117 * @param length 00118 * @return none 00119 */ 00120 void CC3000_UsynchCallback(long lEventType, char * data, unsigned char length); 00121 00122 /** Initialize a CC3000 device and triggers it to start operation. 00123 * @param none 00124 * @return none 00125 */ 00126 int initDriver(void); 00127 00128 /** Return the highest state which we're in. 00129 * @param None 00130 * @return none 00131 */ 00132 char highestCC3000State(void); 00133 00134 /** Return the current state bits. 00135 * @param None 00136 * @return none 00137 */ 00138 char currentCC3000State(void); 00139 00140 /** Sets a state from the state machine. 00141 * @param None 00142 * @return none 00143 */ 00144 void setCC3000MachineState(char stat); 00145 00146 /** Unsets a state from the state machine. 00147 * Also handles LEDs.\n 00148 * @param None 00149 * @return none 00150 */ 00151 void unsetCC3000MachineState(char stat); 00152 00153 /** Resets the State Machine. 00154 * @param None 00155 * @return none 00156 */ 00157 void resetCC3000StateMachine(void); 00158 00159 /** Obtains the CC3000 Connection Information from the CC3000. 00160 * @param None 00161 * @return none 00162 */ 00163 #ifndef CC3000_TINY_DRIVER 00164 tNetappIpconfigRetArgs * getCC3000Info(void); 00165 #endif 00166 00167 /** Trigger a smart configuration process on CC3000. 00168 * It exits upon completion of the process.\n 00169 * @param None 00170 * @return none 00171 */ 00172 void StartSmartConfig(void); 00173 00174 #ifdef __cplusplus 00175 } 00176 #endif // __cplusplus 00177 00178 //***************************************************************************** 00179 // 00180 // Close the Doxygen group. 00181 //! @} 00182 // 00183 //***************************************************************************** 00184 00185 #endif // CC3000_H 00186 00187
Generated on Wed Jul 13 2022 08:35:23 by
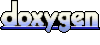