
A rouge-like rpg, heavily inspired on the binding of isaac. Running on a FRDM-K64F Mbed board. C++.
Dependencies: mbed MotionSensor
Health Class Reference
#include <Health.h>
Inherits Entity.
Public Member Functions | |
Health (float pos_x, float pos_y) | |
Constructor. | |
virtual void | move (float unused, float unused1, char *unused2, bool *unused3) |
just because entity has a pure virtual function move, the function is of no use in health as it does not move | |
virtual void | take_damage (int damage) |
reduce _hp by damage | |
virtual void | draw (N5110 &lcd) |
a virtual function of drawing the health onto the screen | |
void | undo_move_x (bool condition) |
a function to undo entity's movement in the x direction if condition is true | |
void | undo_move_y (bool condition) |
a function to undo entity's movement in the y direction if condition is true | |
void | update_prev_pos () |
updates the _prev_pos into _position | |
bool | entity_to_map_collision_test (float pos_x, float pos_y, char *two_d_map, bool *doorways) |
checks if the entity collides the map | |
void | set_position (float x, float y) |
mutates position of the entity to x and y | |
void | position_add_x (float change_x) |
adds change_x onto x-position of the entity | |
void | position_add_y (float change_y) |
adds change_y onto y-position of the entity | |
int | get_hp_drop_chance () |
gets the entity's chance to drop a health | |
int | get_hitbox_width () |
gets the entity's hitbox width | |
int | get_hitbox_height () |
gets the entity's hitbox height | |
int | get_face () |
gets the entity's face | |
int | get_sprite_width () |
gets the entity's sprite width | |
int | get_sprite_height () |
gets the entity's sprite height | |
int | get_offset_x () |
gets the entity's sprite x-offset | |
int | get_offset_y () |
gets the entity's sprite y-offset | |
int | get_pos_x () |
gets the entity's x-position | |
int | get_pos_y () |
gets the entity's y-position | |
int | get_prev_pos_x () |
gets the entity's previous x-position | |
int | get_prev_pos_y () |
gets the entity's previous y-position | |
int | get_attack () |
gets the entity's attack | |
int | get_hp () |
gets the entity's hp | |
float | get_velocity () |
gets the entity's velocity | |
Protected Attributes | |
Hitbox | _hitbox |
A struct containing hitbox data for the entity width and height. | |
SpriteSize | _sprite_size |
A struct containing sprite size data for the entity, to be used when drawing sprites on top of their hitboxes sprite width, sprite height, sprite offset x from hitbox, sprite offset y from hitbox. | |
Position | _position |
A struct containing the position of the entity, this position is the top-left corner of the hitbox. | |
Position | _prev_pos |
A struct containing the position of the entity one loop behind. | |
FrameCount | _frame |
A struct containing frame count, frame number and frame max. | |
int | _hp |
The health point of an entity. | |
int | _attack |
The damage the entity does if it attacks another entity. | |
int | _face |
The direction the entity is facing. | |
float | _velocity |
The speed the entity moves. | |
int | _hp_drop_chance |
The chance(out of 100) of dropping a heart when the entity is deleted. |
Detailed Description
Health Class.
- Date:
- May 2019
Definition at line 10 of file Health.h.
Constructor & Destructor Documentation
Health | ( | float | pos_x, |
float | pos_y | ||
) |
Constructor.
creates a heart that heals when picked up
- Parameters:
-
pos_x initialise _position.x pos_y initialise _position.y
Definition at line 3 of file Health.cpp.
Member Function Documentation
void draw | ( | N5110 & | lcd ) | [virtual] |
a virtual function of drawing the health onto the screen
- Parameters:
-
lcd the screen where the health is drawn on
Implements Entity.
Definition at line 25 of file Health.cpp.
bool entity_to_map_collision_test | ( | float | pos_x, |
float | pos_y, | ||
char * | two_d_map, | ||
bool * | doorways | ||
) | [inherited] |
checks if the entity collides the map
- Parameters:
-
pos_x entity's x-position pos_y entity's y-position two_d_map the 2d map array that dictates where there are walls or empty space doorways an array that dictates which side of the wall has a doorway
- Returns:
- true if entity collide with the map
Definition at line 21 of file Entity.cpp.
int get_attack | ( | ) | [inherited] |
int get_face | ( | ) | [inherited] |
int get_hitbox_height | ( | ) | [inherited] |
int get_hitbox_width | ( | ) | [inherited] |
int get_hp | ( | ) | [inherited] |
int get_hp_drop_chance | ( | ) | [inherited] |
gets the entity's chance to drop a health
- Returns:
- _hp_drop_chance
Definition at line 66 of file Entity.cpp.
int get_offset_x | ( | ) | [inherited] |
gets the entity's sprite x-offset
- Returns:
- _sprite_size.offset_x
Definition at line 90 of file Entity.cpp.
int get_offset_y | ( | ) | [inherited] |
gets the entity's sprite y-offset
- Returns:
- _sprite_size.offset_y
Definition at line 94 of file Entity.cpp.
int get_pos_x | ( | ) | [inherited] |
int get_pos_y | ( | ) | [inherited] |
int get_prev_pos_x | ( | ) | [inherited] |
gets the entity's previous x-position
- Returns:
- _prev_pos.x
Definition at line 106 of file Entity.cpp.
int get_prev_pos_y | ( | ) | [inherited] |
gets the entity's previous y-position
- Returns:
- _prev_pos.y
Definition at line 110 of file Entity.cpp.
int get_sprite_height | ( | ) | [inherited] |
gets the entity's sprite height
- Returns:
- _sprite_size.height
Definition at line 86 of file Entity.cpp.
int get_sprite_width | ( | ) | [inherited] |
float get_velocity | ( | ) | [inherited] |
void move | ( | float | unused, |
float | unused1, | ||
char * | unused2, | ||
bool * | unused3 | ||
) | [virtual] |
just because entity has a pure virtual function move, the function is of no use in health as it does not move
- Parameters:
-
unused not used unused1 not used unused2 not used unused3 not used
Implements Entity.
Definition at line 20 of file Health.cpp.
void position_add_x | ( | float | change_x ) | [inherited] |
adds change_x onto x-position of the entity
- Parameters:
-
change_x displacement x
Definition at line 56 of file Entity.cpp.
void position_add_y | ( | float | change_y ) | [inherited] |
adds change_y onto y-position of the entity
- Parameters:
-
change_y displacement y
Definition at line 60 of file Entity.cpp.
void set_position | ( | float | x, |
float | y | ||
) | [inherited] |
mutates position of the entity to x and y
- Parameters:
-
x x-coordinate value y y-coordinate value
Definition at line 50 of file Entity.cpp.
void take_damage | ( | int | damage ) | [virtual] |
reduce _hp by damage
- Parameters:
-
damage the amount of damage to be taken
Implements Entity.
Definition at line 34 of file Health.cpp.
void undo_move_x | ( | bool | condition ) | [inherited] |
a function to undo entity's movement in the x direction if condition is true
- Parameters:
-
condition a boolean statement
Definition at line 4 of file Entity.cpp.
void undo_move_y | ( | bool | condition ) | [inherited] |
a function to undo entity's movement in the y direction if condition is true
- Parameters:
-
condition a boolean statement
Definition at line 10 of file Entity.cpp.
void update_prev_pos | ( | ) | [inherited] |
updates the _prev_pos into _position
Definition at line 16 of file Entity.cpp.
Field Documentation
int _attack [protected, inherited] |
int _face [protected, inherited] |
FrameCount _frame [protected, inherited] |
Hitbox _hitbox [protected, inherited] |
int _hp [protected, inherited] |
int _hp_drop_chance [protected, inherited] |
Position _position [protected, inherited] |
Position _prev_pos [protected, inherited] |
SpriteSize _sprite_size [protected, inherited] |
Generated on Tue Jul 19 2022 23:32:07 by
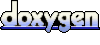