
A rouge-like rpg, heavily inspired on the binding of isaac. Running on a FRDM-K64F Mbed board. C++.
Dependencies: mbed MotionSensor
Entity.h
00001 #ifndef ENTITY_H 00002 #define ENTITY_H 00003 #include "sprites.h" 00004 #include "math.h" 00005 #include "N5110.h" 00006 00007 /**Entity Abstract Class 00008 @author Steven Mahasin 00009 @brief Creates an Entity which holds entity datas, functions(movements, damaged actions, collision test, etc), accessors and functions to be inherited by child classes 00010 @date May 2019 00011 */ 00012 class Entity 00013 { 00014 protected: 00015 struct Hitbox { 00016 int width; 00017 int height; 00018 }; 00019 struct SpriteSize { 00020 int width; 00021 int height; 00022 // Top-left corner of sprite is offset_x 00023 // to the right of top-left corner of hitbox 00024 int offset_x; 00025 // Top-left corner of sprite is offset_y 00026 // below of top-left corner of hitbox 00027 int offset_y; 00028 }; 00029 struct Position { 00030 float x; 00031 float y; 00032 }; 00033 struct FrameCount { 00034 int count; 00035 int number; 00036 int max; 00037 }; 00038 /** 00039 * @brief A struct containing hitbox data for the entity 00040 * @info width and height 00041 */ 00042 Hitbox _hitbox; 00043 /** 00044 * @brief A struct containing sprite size data for the entity, to be used when drawing sprites on top of their hitboxes 00045 * @info sprite width, sprite height, sprite offset x from hitbox, sprite offset y from hitbox 00046 */ 00047 SpriteSize _sprite_size; 00048 /** 00049 * @brief A struct containing the position of the entity, this position is the top-left corner of the hitbox 00050 */ 00051 Position _position; 00052 /** 00053 * @brief A struct containing the position of the entity one loop behind 00054 */ 00055 Position _prev_pos; 00056 /** 00057 * @brief A struct containing frame count, frame number and frame max. Used to animate entities 00058 */ 00059 FrameCount _frame; 00060 /** 00061 * @brief The health point of an entity 00062 */ 00063 int _hp; 00064 /** 00065 * @brief The damage the entity does if it attacks another entity 00066 */ 00067 int _attack; 00068 /** 00069 * @brief The direction the entity is facing 00070 */ 00071 int _face; 00072 /** 00073 * @brief The speed the entity moves 00074 */ 00075 float _velocity; 00076 /** 00077 * @brief The chance(out of 100) of dropping a heart when the entity is deleted. 00078 */ 00079 int _hp_drop_chance; 00080 00081 public: 00082 // Function 00083 /** 00084 * @brief a virtual function movement of the entity to be inherited 00085 * @param x_value @details either joystick x or player's x position 00086 * @param y_value @details either joystick y or player's y position 00087 * @param map @details the 2d map array that dictates where there are walls or empty space 00088 * @param doorways @details an array that dictates which side of the wall has a doorway 00089 */ 00090 virtual void move(float x_value, float y_value, char * map, bool * doorways) = 0; // movement control and miscellaneous updates 00091 /** 00092 * @brief a virtual function of the action when taking damage to be inherited 00093 * @param damage @details the amount of damage to be taken 00094 */ 00095 virtual void take_damage(int damage) = 0; 00096 /** 00097 * @brief a virtual function of drawing the entity to be inherited 00098 * @param lcd @details the screen where the entity is drawn on 00099 */ 00100 virtual void draw(N5110 &lcd) = 0; 00101 /** 00102 * @brief a function to undo entity's movement in the x direction if condition is true 00103 * @param condition @details a boolean statement 00104 */ 00105 void undo_move_x(bool condition); 00106 /** 00107 * @brief a function to undo entity's movement in the y direction if condition is true 00108 * @param condition @details a boolean statement 00109 */ 00110 void undo_move_y(bool condition); 00111 /** 00112 * @brief updates the _prev_pos into _position 00113 */ 00114 void update_prev_pos(); 00115 /** 00116 * @brief checks if the entity collides the map 00117 * @param pos_x @details entity's x-position 00118 * @param pos_y @details entity's y-position 00119 * @param two_d_map @details the 2d map array that dictates where there are walls or empty space 00120 * @param doorways @details an array that dictates which side of the wall has a doorway 00121 * @return true if entity collide with the map 00122 */ 00123 bool entity_to_map_collision_test(float pos_x, float pos_y, char * two_d_map, bool * doorways); 00124 00125 // Mutator 00126 /** 00127 * @brief mutates position of the entity to x and y 00128 * @param x @details x-coordinate value 00129 * @param y @details y-coordinate value 00130 */ 00131 void set_position(float x, float y); 00132 /** 00133 * @brief adds change_x onto x-position of the entity 00134 * @param change_x @details displacement x 00135 */ 00136 void position_add_x(float change_x); 00137 /** 00138 * @brief adds change_y onto y-position of the entity 00139 * @param change_y @details displacement y 00140 */ 00141 void position_add_y(float change_y); 00142 00143 // Accessors 00144 /** 00145 * @brief gets the entity's chance to drop a health 00146 * @return _hp_drop_chance 00147 */ 00148 int get_hp_drop_chance(); 00149 /** 00150 * @brief gets the entity's hitbox width 00151 * @return _hitbox.width 00152 */ 00153 int get_hitbox_width(); 00154 /** 00155 * @brief gets the entity's hitbox height 00156 * @return _hitbox.height 00157 */ 00158 int get_hitbox_height(); 00159 /** 00160 * @brief gets the entity's face 00161 * @return _face 00162 */ 00163 int get_face(); 00164 /** 00165 * @brief gets the entity's sprite width 00166 * @return _sprite_size.width 00167 */ 00168 int get_sprite_width(); 00169 /** 00170 * @brief gets the entity's sprite height 00171 * @return _sprite_size.height 00172 */ 00173 int get_sprite_height(); 00174 /** 00175 * @brief gets the entity's sprite x-offset 00176 * @return _sprite_size.offset_x 00177 */ 00178 int get_offset_x(); 00179 /** 00180 * @brief gets the entity's sprite y-offset 00181 * @return _sprite_size.offset_y 00182 */ 00183 int get_offset_y(); 00184 /** 00185 * @brief gets the entity's x-position 00186 * @return _position.x 00187 */ 00188 int get_pos_x(); 00189 /** 00190 * @brief gets the entity's y-position 00191 * @return _position.y 00192 */ 00193 int get_pos_y(); 00194 /** 00195 * @brief gets the entity's previous x-position 00196 * @return _prev_pos.x 00197 */ 00198 int get_prev_pos_x(); 00199 /** 00200 * @brief gets the entity's previous y-position 00201 * @return _prev_pos.y 00202 */ 00203 int get_prev_pos_y(); 00204 /** 00205 * @brief gets the entity's attack 00206 * @return _attack 00207 */ 00208 int get_attack(); 00209 /** 00210 * @brief gets the entity's hp 00211 * @return _hp 00212 */ 00213 int get_hp(); 00214 /** 00215 * @brief gets the entity's velocity 00216 * @return _velocity 00217 */ 00218 float get_velocity(); 00219 00220 }; 00221 00222 #endif
Generated on Tue Jul 19 2022 23:32:07 by
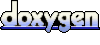