
A rouge-like rpg, heavily inspired on the binding of isaac. Running on a FRDM-K64F Mbed board. C++.
Dependencies: mbed MotionSensor
Title.h
00001 #ifndef TITLE_H 00002 #define TITLE_H 00003 00004 #include "Player.h" 00005 #include "N5110.h" 00006 #include "Gamepad.h" 00007 /**Title Class 00008 @author Steven Mahasin 00009 @brief Handles all Title Screen Interractions 00010 @date May 2019 00011 */ 00012 class Title 00013 { 00014 private: 00015 // Member Variables 00016 /** 00017 * @brief a counter that increments per loop, used to detect delays 00018 */ 00019 int _title_count; 00020 /** 00021 * @brief a delay period for the joystick to update another right or left input 00022 */ 00023 int _cursor_timer; 00024 /** 00025 * @brief an integer that stores which option the cursor is on 00026 * @note 0 = Start, 1 = Options, 2 = Credits, 3 = Tutorial 00027 */ 00028 int _title_option; 00029 00030 // Methods 00031 /** 00032 * @brief This function is used to draw the title screen along with the animations 00033 * @param lcd @details the screen the title screen is drawn on 00034 */ 00035 void draw_title_screen(N5110 &lcd); 00036 /** 00037 * @brief This function is used to read the gamepad buttons and joystick, interracting with the member variable _title_option 00038 * @param gamepad @details the gamepad the input is being read from 00039 */ 00040 void title_options_joystick(Gamepad &gamepad); 00041 00042 /** 00043 * @brief This function is called when the user chooses the title option "Option" 00044 * @param lcd @details the screen the Options screen is drawn on 00045 * @param gamepad @details the gamepad used to read the potentiometer 00046 * @param player @details the player that is being used as a demo for the options screen 00047 * @param global_contrast @details the global contrast that is being varried to be set for the whole game 00048 */ 00049 void title_option_option(N5110 &lcd, Gamepad &gamepad, Player &player, float &global_contrast); 00050 /** 00051 * @brief This function is called when the user chooses the title option "Credit" 00052 * @param lcd @details the screen the credits is being displayed on 00053 * @param gamepad @details the gamepad is used to read when the user decides to exit the credit page (button A) 00054 */ 00055 void title_option_credit(N5110 &lcd, Gamepad &gamepad); 00056 /** 00057 * @brief This function is called when the user chooses the title option "Tutorial" 00058 * @param lcd @details the screen tutotrial is being displayed on 00059 * @param gamepad @details the gamepad is used to read when the user decides to go to the next tutorial page (button A) 00060 */ 00061 void title_option_tutorial(N5110 &lcd, Gamepad &gamepad); 00062 00063 /** 00064 * @brief This function is displays tutorial page 0 00065 * @param lcd @details the screen the credits is being displayed on 00066 */ 00067 void print_tutorial_page_0(N5110 &lcd); 00068 /** 00069 * @brief This function is displays tutorial page 1 00070 * @param lcd @details the screen the credits is being displayed on 00071 */ 00072 void print_tutorial_page_1(N5110 &lcd); 00073 /** 00074 * @brief This function is displays tutorial page 2 00075 * @param lcd @details the screen the credits is being displayed on 00076 */ 00077 void print_tutorial_page_2(N5110 &lcd); 00078 00079 public: 00080 /** Constructor */ 00081 Title(); 00082 00083 // Accessor 00084 /** @brief to get the seed for rand() 00085 * @returns _title_count 00086 */ 00087 int get_seed(); 00088 00089 // Functions 00090 /** @brief This function runs the whole Title screen, it contains the title loop and calls for the rest of the methods 00091 * @param lcd @details the screen the Title screen is being displayed on 00092 * @param gamepad @details the input the Title screen processes 00093 * @param global_contrast @details the variable that is varied by the Title screen to chance screen contrast 00094 */ 00095 void main(N5110 &lcd, Gamepad &gamepad, float &global_contrast); 00096 }; 00097 00098 #endif
Generated on Tue Jul 19 2022 23:32:07 by
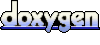