
A rouge-like rpg, heavily inspired on the binding of isaac. Running on a FRDM-K64F Mbed board. C++.
Dependencies: mbed MotionSensor
Title.cpp
00001 #include "Title.h" 00002 00003 Title::Title() 00004 { 00005 _title_count = 0; 00006 _cursor_timer = 20; 00007 _title_option = 0; 00008 } 00009 00010 int Title::get_seed() 00011 { 00012 return _title_count; 00013 } 00014 00015 void Title::main(N5110 &lcd, Gamepad &gamepad, float &global_contrast) 00016 { 00017 Player player(5, 36); 00018 while(1){ // Title Screen Loop 00019 _title_option = 0; 00020 while(!gamepad.check_event(Gamepad::A_PRESSED)){ 00021 lcd.clear(); 00022 draw_title_screen(lcd); 00023 lcd.refresh(); 00024 title_options_joystick(gamepad); 00025 _title_count++; 00026 wait_ms(1000/40); // 1s/framerate 00027 } 00028 while(gamepad.check_event(Gamepad::A_PRESSED)){} 00029 00030 if(_title_option == 0) { break; // Start game, exit title loop 00031 } else if (_title_option == 1) { title_option_option(lcd, gamepad, player, global_contrast); 00032 } else if (_title_option == 2) { title_option_credit(lcd, gamepad); 00033 } else if (_title_option == 3) { title_option_tutorial(lcd, gamepad); 00034 } 00035 } 00036 player.~Player(); 00037 } 00038 00039 void Title::draw_title_screen(N5110 &lcd) 00040 { 00041 lcd.drawSprite(11, 4, 15, 44, (char *)title_name_0); 00042 lcd.drawSpriteTransparent(19, 14, 17, 53, (char *)title_name_1); 00043 lcd.drawCircle(79, 7, 10, FILL_BLACK); 00044 lcd.drawCircle(81, 5, 8, FILL_WHITE); 00045 lcd.drawSprite(56, 6, 11, 5, (char *)star_sprite[abs(((_title_count/20) % 7) - 3)]); 00046 lcd.drawSprite(12, 34, 8, 8, (char *)button_A_sprite); 00047 lcd.drawSprite(22, 37, 3, 2, (char *)arrow_left_sprite); 00048 lcd.drawSprite(59, 37, 3, 2, (char *)arrow_right_sprite); 00049 lcd.drawSprite(69, 31, 12, 6, (char *)sprite_player[(_title_count/40) % 4][(_title_count/10) % 4]); 00050 lcd.drawSprite(26, 35, 9, 32, (char *)title_options_sprite[_title_option]); 00051 } 00052 00053 void Title::title_options_joystick(Gamepad &gamepad) 00054 { 00055 if ((gamepad.get_direction() == 3) && (_cursor_timer > 20)) { // Detect Joystick going right 00056 _cursor_timer = 0; 00057 if (_title_option >= 3) { 00058 _title_option = 0; 00059 } else { 00060 _title_option++; 00061 } 00062 } else if ((gamepad.get_direction() == 7) && (_cursor_timer > 20)) { // Detect Joystick going left 00063 _cursor_timer = 0; 00064 if (_title_option <= 0) { 00065 _title_option = 3; 00066 } else { 00067 _title_option--; 00068 } 00069 } 00070 _cursor_timer++; 00071 } 00072 00073 void Title::title_option_option(N5110 &lcd, Gamepad &gamepad, Player &player, float &global_contrast) 00074 { 00075 while(!gamepad.check_event(Gamepad::A_PRESSED)) { 00076 global_contrast = gamepad.read_pot(); 00077 lcd.setContrast(global_contrast); 00078 lcd.clear(); 00079 lcd.printString("Set contrast", 0, 0); 00080 lcd.printString("using the", 0, 1); 00081 lcd.printString("potentiometer", 0, 2); 00082 player.draw(lcd); 00083 player.draw_bullets(lcd, player.get_pos_y() + 2); 00084 lcd.refresh(); 00085 player.move(1, 0, (char *)level_map[0][0], (bool *)sprite_transparent_player); // Adding animation of walking 00086 player.undo_move_x(true); // Keeping the player in place 00087 player.buttons(false, true, false, false); // Instructing player to shoot right 00088 player.delete_out_of_bounds_bullets((char *)level_map[0][0], (bool *)sprite_transparent_player); // Move the bullets and delete those out of the screen 00089 wait_ms(1000/40); 00090 } 00091 wait(0.05); 00092 while(gamepad.check_event(Gamepad::A_PRESSED)) {} 00093 } 00094 00095 void Title::title_option_credit(N5110 &lcd, Gamepad &gamepad) 00096 { 00097 lcd.clear(); 00098 lcd.printString("Made by:", 0, 0); 00099 lcd.printString("Steven Mahasin", 0, 1); 00100 lcd.printString("201192939", 0, 2); 00101 lcd.refresh(); 00102 wait(0.05); 00103 while(!gamepad.check_event(Gamepad::A_PRESSED)) { 00104 } 00105 wait(0.05); 00106 while(gamepad.check_event(Gamepad::A_PRESSED)) { 00107 } 00108 } 00109 00110 void Title::title_option_tutorial(N5110 &lcd, Gamepad &gamepad) 00111 { 00112 print_tutorial_page_0(lcd); // print page 0 and wait for button toggle 00113 wait(0.05); 00114 while(!gamepad.check_event(Gamepad::A_PRESSED)) {} 00115 wait(0.05); 00116 while(gamepad.check_event(Gamepad::A_PRESSED)) {} 00117 print_tutorial_page_1(lcd); // print page 1 and wait for button toggle 00118 wait(0.05); 00119 while(!gamepad.check_event(Gamepad::A_PRESSED)) {} 00120 wait(0.05); 00121 while(gamepad.check_event(Gamepad::A_PRESSED)) {} 00122 print_tutorial_page_2(lcd); // print page 2 and wait for button toggle 00123 wait(0.05); 00124 while(!gamepad.check_event(Gamepad::A_PRESSED)) {} 00125 wait(0.05); 00126 while(gamepad.check_event(Gamepad::A_PRESSED)) {} 00127 } 00128 00129 void Title::print_tutorial_page_0(N5110 &lcd) 00130 { 00131 lcd.clear(); 00132 lcd.printString("Use the joypad", 0, 0); 00133 lcd.printString("to move the", 0, 1); 00134 lcd.printString("player", 0, 2); 00135 lcd.printString("Use buttons", 0, 3); 00136 lcd.printString("ABXY to shoot", 0, 4); 00137 lcd.printString("directionally", 0, 5); 00138 lcd.refresh(); 00139 } 00140 00141 void Title::print_tutorial_page_1(N5110 &lcd) 00142 { 00143 lcd.clear(); 00144 lcd.printString("Hold L to", 0, 0); 00145 lcd.printString("show player", 0, 1); 00146 lcd.printString("health", 0, 2); 00147 lcd.printString("Hold R to", 0, 3); 00148 lcd.printString("view explored", 0, 4); 00149 lcd.printString("minimap", 0, 5); 00150 lcd.refresh(); 00151 } 00152 00153 void Title::print_tutorial_page_2(N5110 &lcd) 00154 { 00155 lcd.clear(); 00156 lcd.printString("Press Start", 0, 0); 00157 lcd.printString("to pause", 0, 1); 00158 lcd.printString("the game", 0, 2); 00159 lcd.refresh(); 00160 }
Generated on Tue Jul 19 2022 23:32:07 by
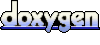