
Example code how to get temperature from the TMP102 temperature sensor
Embed:
(wiki syntax)
Show/hide line numbers
main.cpp
00001 /* 00002 00003 2645_I2C_TMP102_Library 00004 00005 Sample code from ELEC2645 Week 17 Lab 00006 00007 Demonstrates how to re-factor the TMP102 code into a library 00008 00009 (c) Craig A. Evans, University of Leeds, Feb 2016 00010 00011 */ 00012 00013 #include "mbed.h" 00014 // include the library header, ensure the library has been imported into the project 00015 #include "TMP102.h" 00016 00017 // Create TMP102 object 00018 TMP102 tmp102(I2C_SDA,I2C_SCL); 00019 // UART connection for PC 00020 Serial pc(USBTX,USBRX); 00021 00022 // K64F on-board LEDs 00023 DigitalOut r_led(LED_RED); 00024 DigitalOut g_led(LED_GREEN); 00025 DigitalOut b_led(LED_BLUE); 00026 // K64F on-board switches 00027 InterruptIn sw2(SW2); 00028 InterruptIn sw3(SW3); 00029 00030 // error function hangs flashing an LED 00031 void error(); 00032 // setup serial port 00033 void init_serial(); 00034 // set-up the on-board LEDs and switches 00035 void init_K64F(); 00036 00037 int main() 00038 { 00039 // initialise the board and serial port 00040 init_K64F(); 00041 init_serial(); 00042 // call the sensor init method using dot syntax 00043 tmp102.init(); 00044 00045 while (1) { 00046 00047 // read temperature and print over serial port 00048 float T = tmp102.get_temperature(); 00049 pc.printf("T = %f K\n",T); 00050 // small delay - 1s to match the update rate of the sensor (1 Hz) 00051 wait(1.0); 00052 00053 } 00054 00055 } 00056 00057 void init_serial() { 00058 // set to highest baud - ensure terminal software matches 00059 pc.baud(115200); 00060 } 00061 00062 void init_K64F() 00063 { 00064 // on-board LEDs are active-low, so set pin high to turn them off. 00065 r_led = 1; 00066 g_led = 1; 00067 b_led = 1; 00068 00069 // since the on-board switches have external pull-ups, we should disable the internal pull-down 00070 // resistors that are enabled by default using InterruptIn 00071 sw2.mode(PullNone); 00072 sw3.mode(PullNone); 00073 00074 }
Generated on Fri Jul 15 2022 04:05:10 by
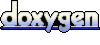