
Source code for a MIDI controller built from parts and designed to be extensible
Fork of USBMIDI_HelloWorld by
main.cpp
00001 // Hello World example for the USBMIDI library 00002 00003 #include "mbed.h" 00004 #include "USBMIDI.h" 00005 00006 void show_message(MIDIMessage msg) { 00007 switch (msg.type()) { 00008 case MIDIMessage::NoteOnType: 00009 printf("NoteOn key:%d, velocity: %d, channel: %d\n", msg.key(), msg.velocity(), msg.channel()); 00010 break; 00011 case MIDIMessage::NoteOffType: 00012 printf("NoteOff key:%d, velocity: %d, channel: %d\n", msg.key(), msg.velocity(), msg.channel()); 00013 break; 00014 case MIDIMessage::ControlChangeType: 00015 printf("ControlChange controller: %d, data: %d\n", msg.controller(), msg.value()); 00016 break; 00017 case MIDIMessage::PitchWheelType: 00018 printf("PitchWheel channel: %d, pitch: %d\n", msg.channel(), msg.pitch()); 00019 break; 00020 default: 00021 printf("Another message\n"); 00022 } 00023 } 00024 00025 static const int MIDI_MAX_VALUE = 16384; 00026 static const float MIDI_MAX_VALUE_F = 16384.0f; 00027 00028 00029 static const float TOLERANCE = 0.01f; 00030 static const int NOISE_FLOOR = 600; 00031 00032 static const float LOWER_TOLERANCE = 0.016f; 00033 static const float LOWER_TOLERANCE_BEGIN = 0.34f; 00034 00035 USBMIDI midi; 00036 AnalogIn pot1(p20); 00037 Serial pc(USBTX, USBRX); // tx, rx 00038 00039 void write_full_cc(int controlmsb, int controllsb, int channel, int value){ 00040 int lsb = value / 128; //value & 0x7F; 00041 int msb = value % 128; //value & 0x7F80 >> 7; 00042 midi.write(MIDIMessage::ControlChange(controlmsb, msb, channel)); 00043 midi.write(MIDIMessage::ControlChange(controllsb, lsb, channel)); 00044 } 00045 00046 #define SMOOTHING_AMOUNT 500 00047 00048 int main() { 00049 midi.attach(show_message); // call back for messages received 00050 00051 float last_value = 0.0f; 00052 while (1) { 00053 float counter=0.0f; 00054 for(int i=0;i<SMOOTHING_AMOUNT;i++){ 00055 wait_us(10); 00056 counter+=pot1; 00057 } 00058 float value = counter / SMOOTHING_AMOUNT; 00059 int midi_value = (int)(MIDI_MAX_VALUE_F * value); 00060 00061 //at low voltage noise takes over.. 00062 if(midi_value < NOISE_FLOOR){ 00063 value = 0.0f; 00064 midi_value = 0; 00065 } 00066 if(value - last_value > TOLERANCE || value - last_value < -TOLERANCE){ 00067 //as we approach noise floor, things get noisey.. 00068 if(value < LOWER_TOLERANCE_BEGIN && !(value - last_value > LOWER_TOLERANCE || value - last_value < -LOWER_TOLERANCE)){ 00069 continue; 00070 } 00071 pc.printf("sent: %f, %i\r\n", value, midi_value); 00072 last_value = value; 00073 write_full_cc(20, 52, 0, midi_value); 00074 } 00075 00076 } 00077 }
Generated on Fri Jul 15 2022 22:00:48 by
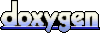