NetServices Stack source
Dependents: HelloWorld ServoInterfaceBoardExample1 4180_Lab4
Transmission Control Protocol for IP. More...
Go to the source code of this file.
Functions | |
static u16_t | tcp_new_port (void) |
A nastly hack featuring 'goto' statements that allocates a new TCP local port. | |
void | tcp_tmr (void) |
Called periodically to dispatch TCP timers. | |
static err_t | tcp_close_shutdown (struct tcp_pcb *pcb, u8_t rst_on_unacked_data) |
Closes the TX side of a connection held by the PCB. | |
err_t | tcp_close (struct tcp_pcb *pcb) |
Closes the connection held by the PCB. | |
err_t | tcp_shutdown (struct tcp_pcb *pcb, int shut_rx, int shut_tx) |
Causes all or part of a full-duplex connection of this PCB to be shut down. | |
void | tcp_abandon (struct tcp_pcb *pcb, int reset) |
Abandons a connection and optionally sends a RST to the remote host. | |
void | tcp_abort (struct tcp_pcb *pcb) |
Aborts the connection by sending a RST (reset) segment to the remote host. | |
err_t | tcp_bind (struct tcp_pcb *pcb, ip_addr_t *ipaddr, u16_t port) |
Binds the connection to a local portnumber and IP address. | |
static err_t | tcp_accept_null (void *arg, struct tcp_pcb *pcb, err_t err) |
Default accept callback if no accept callback is specified by the user. | |
struct tcp_pcb * | tcp_listen_with_backlog (struct tcp_pcb *pcb, u8_t backlog) |
Set the state of the connection to be LISTEN, which means that it is able to accept incoming connections. | |
u32_t | tcp_update_rcv_ann_wnd (struct tcp_pcb *pcb) |
Update the state that tracks the available window space to advertise. | |
void | tcp_recved (struct tcp_pcb *pcb, u16_t len) |
This function should be called by the application when it has processed the data. | |
err_t | tcp_connect (struct tcp_pcb *pcb, ip_addr_t *ipaddr, u16_t port, tcp_connected_fn connected) |
Connects to another host. | |
void | tcp_slowtmr (void) |
Called every 500 ms and implements the retransmission timer and the timer that removes PCBs that have been in TIME-WAIT for enough time. | |
void | tcp_fasttmr (void) |
Is called every TCP_FAST_INTERVAL (250 ms) and process data previously "refused" by upper layer (application) and sends delayed ACKs. | |
void | tcp_segs_free (struct tcp_seg *seg) |
Deallocates a list of TCP segments (tcp_seg structures). | |
void | tcp_seg_free (struct tcp_seg *seg) |
Frees a TCP segment (tcp_seg structure). | |
void | tcp_setprio (struct tcp_pcb *pcb, u8_t prio) |
Sets the priority of a connection. | |
struct tcp_seg * | tcp_seg_copy (struct tcp_seg *seg) |
Returns a copy of the given TCP segment. | |
err_t | tcp_recv_null (void *arg, struct tcp_pcb *pcb, struct pbuf *p, err_t err) |
Default receive callback that is called if the user didn't register a recv callback for the pcb. | |
static void | tcp_kill_prio (u8_t prio) |
Kills the oldest active connection that has lower priority than prio. | |
static void | tcp_kill_timewait (void) |
Kills the oldest connection that is in TIME_WAIT state. | |
struct tcp_pcb * | tcp_alloc (u8_t prio) |
Allocate a new tcp_pcb structure. | |
struct tcp_pcb * | tcp_new (void) |
Creates a new TCP protocol control block but doesn't place it on any of the TCP PCB lists. | |
void | tcp_arg (struct tcp_pcb *pcb, void *arg) |
Used to specify the argument that should be passed callback functions. | |
void | tcp_recv (struct tcp_pcb *pcb, tcp_recv_fn recv) |
Used to specify the function that should be called when a TCP connection receives data. | |
void | tcp_sent (struct tcp_pcb *pcb, tcp_sent_fn sent) |
Used to specify the function that should be called when TCP data has been successfully delivered to the remote host. | |
void | tcp_err (struct tcp_pcb *pcb, tcp_err_fn err) |
Used to specify the function that should be called when a fatal error has occured on the connection. | |
void | tcp_accept (struct tcp_pcb *pcb, tcp_accept_fn accept) |
Used for specifying the function that should be called when a LISTENing connection has been connected to another host. | |
void | tcp_poll (struct tcp_pcb *pcb, tcp_poll_fn poll, u8_t interval) |
Used to specify the function that should be called periodically from TCP. | |
void | tcp_pcb_purge (struct tcp_pcb *pcb) |
Purges a TCP PCB. | |
void | tcp_pcb_remove (struct tcp_pcb **pcblist, struct tcp_pcb *pcb) |
Purges the PCB and removes it from a PCB list. | |
u32_t | tcp_next_iss (void) |
Calculates a new initial sequence number for new connections. | |
u16_t | tcp_eff_send_mss (u16_t sendmss, ip_addr_t *addr) |
Calcluates the effective send mss that can be used for a specific IP address by using ip_route to determin the netif used to send to the address and calculating the minimum of TCP_MSS and that netif's mtu (if set). | |
void | tcp_debug_print (struct tcp_hdr *tcphdr) |
Print a tcp header for debugging purposes. | |
void | tcp_debug_print_state (enum tcp_state s) |
Print a tcp state for debugging purposes. | |
void | tcp_debug_print_flags (u8_t flags) |
Print tcp flags for debugging purposes. | |
void | tcp_debug_print_pcbs (void) |
Print all tcp_pcbs in every list for debugging purposes. | |
s16_t | tcp_pcbs_sane (void) |
Check state consistency of the tcp_pcb lists. | |
Variables | |
struct tcp_pcb * | tcp_bound_pcbs |
List of all TCP PCBs bound but not yet (connected || listening) | |
union tcp_listen_pcbs_t | tcp_listen_pcbs |
List of all TCP PCBs in LISTEN state. | |
struct tcp_pcb * | tcp_active_pcbs |
List of all TCP PCBs that are in a state in which they accept or send data. | |
struct tcp_pcb * | tcp_tw_pcbs |
List of all TCP PCBs in TIME-WAIT state. | |
struct tcp_pcb ** | tcp_pcb_lists [] |
An array with all (non-temporary) PCB lists, mainly used for smaller code size. | |
struct tcp_pcb * | tcp_tmp_pcb |
Only used for temporary storage. | |
static u8_t | tcp_timer |
Timer counter to handle calling slow-timer from tcp_tmr() |
Detailed Description
Transmission Control Protocol for IP.
This file contains common functions for the TCP implementation, such as functinos for manipulating the data structures and the TCP timer functions. TCP functions related to input and output is found in tcp_in.c and tcp_out.c respectively.
Definition in file tcp.c.
Function Documentation
void tcp_abandon | ( | struct tcp_pcb * | pcb, |
int | reset | ||
) |
Abandons a connection and optionally sends a RST to the remote host.
Deletes the local protocol control block. This is done when a connection is killed because of shortage of memory.
- Parameters:
-
pcb the tcp_pcb to abort reset boolean to indicate whether a reset should be sent
void tcp_abort | ( | struct tcp_pcb * | pcb ) |
Aborts the connection by sending a RST (reset) segment to the remote host.
The pcb is deallocated. This function never fails.
ATTENTION: When calling this from one of the TCP callbacks, make sure you always return ERR_ABRT (and never return ERR_ABRT otherwise or you will risk accessing deallocated memory or memory leaks!
- Parameters:
-
pcb the tcp pcb to abort
void tcp_accept | ( | struct tcp_pcb * | pcb, |
tcp_accept_fn | accept | ||
) |
Used for specifying the function that should be called when a LISTENing connection has been connected to another host.
- Parameters:
-
pcb tcp_pcb to set the accept callback accept callback function to call for this pcb when LISTENing connection has been connected to another host
static err_t tcp_accept_null | ( | void * | arg, |
struct tcp_pcb * | pcb, | ||
err_t | err | ||
) | [static] |
struct tcp_pcb* tcp_alloc | ( | u8_t | prio ) | [read] |
void tcp_arg | ( | struct tcp_pcb * | pcb, |
void * | arg | ||
) |
err_t tcp_bind | ( | struct tcp_pcb * | pcb, |
ip_addr_t * | ipaddr, | ||
u16_t | port | ||
) |
Binds the connection to a local portnumber and IP address.
If the IP address is not given (i.e., ipaddr == NULL), the IP address of the outgoing network interface is used instead.
- Parameters:
-
pcb the tcp_pcb to bind (no check is done whether this pcb is already bound!) ipaddr the local ip address to bind to (use IP_ADDR_ANY to bind to any local address port the local port to bind to
- Returns:
- ERR_USE if the port is already in use ERR_OK if bound
err_t tcp_close | ( | struct tcp_pcb * | pcb ) |
Closes the connection held by the PCB.
Listening pcbs are freed and may not be referenced any more. Connection pcbs are freed if not yet connected and may not be referenced any more. If a connection is established (at least SYN received or in a closing state), the connection is closed, and put in a closing state. The pcb is then automatically freed in tcp_slowtmr(). It is therefore unsafe to reference it (unless an error is returned).
- Parameters:
-
pcb the tcp_pcb to close
- Returns:
- ERR_OK if connection has been closed another err_t if closing failed and pcb is not freed
static err_t tcp_close_shutdown | ( | struct tcp_pcb * | pcb, |
u8_t | rst_on_unacked_data | ||
) | [static] |
Closes the TX side of a connection held by the PCB.
For tcp_close(), a RST is sent if the application didn't receive all data (tcp_recved() not called for all data passed to recv callback).
Listening pcbs are freed and may not be referenced any more. Connection pcbs are freed if not yet connected and may not be referenced any more. If a connection is established (at least SYN received or in a closing state), the connection is closed, and put in a closing state. The pcb is then automatically freed in tcp_slowtmr(). It is therefore unsafe to reference it.
- Parameters:
-
pcb the tcp_pcb to close
- Returns:
- ERR_OK if connection has been closed another err_t if closing failed and pcb is not freed
err_t tcp_connect | ( | struct tcp_pcb * | pcb, |
ip_addr_t * | ipaddr, | ||
u16_t | port, | ||
tcp_connected_fn | connected | ||
) |
Connects to another host.
The function given as the "connected" argument will be called when the connection has been established.
- Parameters:
-
pcb the tcp_pcb used to establish the connection ipaddr the remote ip address to connect to port the remote tcp port to connect to connected callback function to call when connected (or on error)
- Returns:
- ERR_VAL if invalid arguments are given ERR_OK if connect request has been sent other err_t values if connect request couldn't be sent
void tcp_debug_print | ( | struct tcp_hdr * | tcphdr ) |
void tcp_debug_print_flags | ( | u8_t | flags ) |
void tcp_debug_print_pcbs | ( | void | ) |
void tcp_debug_print_state | ( | enum tcp_state | s ) |
u16_t tcp_eff_send_mss | ( | u16_t | sendmss, |
ip_addr_t * | addr | ||
) |
void tcp_err | ( | struct tcp_pcb * | pcb, |
tcp_err_fn | err | ||
) |
void tcp_fasttmr | ( | void | ) |
static void tcp_kill_prio | ( | u8_t | prio ) | [static] |
static void tcp_kill_timewait | ( | void | ) | [static] |
Kills the oldest connection that is in TIME_WAIT state.
Called from tcp_alloc() if no more connections are available.
struct tcp_pcb* tcp_listen_with_backlog | ( | struct tcp_pcb * | pcb, |
u8_t | backlog | ||
) | [read] |
Set the state of the connection to be LISTEN, which means that it is able to accept incoming connections.
The protocol control block is reallocated in order to consume less memory. Setting the connection to LISTEN is an irreversible process.
- Parameters:
-
pcb the original tcp_pcb backlog the incoming connections queue limit
- Returns:
- tcp_pcb used for listening, consumes less memory.
- Note:
- The original tcp_pcb is freed. This function therefore has to be called like this: tpcb = tcp_listen(tpcb);
struct tcp_pcb* tcp_new | ( | void | ) | [read] |
Creates a new TCP protocol control block but doesn't place it on any of the TCP PCB lists.
The pcb is not put on any list until binding using tcp_bind().
static u16_t tcp_new_port | ( | void | ) | [static] |
u32_t tcp_next_iss | ( | void | ) |
void tcp_pcb_purge | ( | struct tcp_pcb * | pcb ) |
void tcp_pcb_remove | ( | struct tcp_pcb ** | pcblist, |
struct tcp_pcb * | pcb | ||
) |
s16_t tcp_pcbs_sane | ( | void | ) |
void tcp_poll | ( | struct tcp_pcb * | pcb, |
tcp_poll_fn | poll, | ||
u8_t | interval | ||
) |
void tcp_recv | ( | struct tcp_pcb * | pcb, |
tcp_recv_fn | recv | ||
) |
err_t tcp_recv_null | ( | void * | arg, |
struct tcp_pcb * | pcb, | ||
struct pbuf * | p, | ||
err_t | err | ||
) |
void tcp_recved | ( | struct tcp_pcb * | pcb, |
u16_t | len | ||
) |
This function should be called by the application when it has processed the data.
The purpose is to advertise a larger window when the data has been processed.
- Parameters:
-
pcb the tcp_pcb for which data is read len the amount of bytes that have been read by the application
struct tcp_seg* tcp_seg_copy | ( | struct tcp_seg * | seg ) | [read] |
void tcp_seg_free | ( | struct tcp_seg * | seg ) |
void tcp_segs_free | ( | struct tcp_seg * | seg ) |
void tcp_sent | ( | struct tcp_pcb * | pcb, |
tcp_sent_fn | sent | ||
) |
void tcp_setprio | ( | struct tcp_pcb * | pcb, |
u8_t | prio | ||
) |
err_t tcp_shutdown | ( | struct tcp_pcb * | pcb, |
int | shut_rx, | ||
int | shut_tx | ||
) |
Causes all or part of a full-duplex connection of this PCB to be shut down.
This doesn't deallocate the PCB!
- Parameters:
-
pcb PCB to shutdown shut_rx shut down receive side if this is != 0 shut_tx shut down send side if this is != 0
- Returns:
- ERR_OK if shutdown succeeded (or the PCB has already been shut down) another err_t on error.
void tcp_slowtmr | ( | void | ) |
void tcp_tmr | ( | void | ) |
u32_t tcp_update_rcv_ann_wnd | ( | struct tcp_pcb * | pcb ) |
Variable Documentation
struct tcp_pcb* tcp_active_pcbs |
struct tcp_pcb* tcp_bound_pcbs |
union tcp_listen_pcbs_t tcp_listen_pcbs |
struct tcp_pcb** tcp_pcb_lists[] |
{&tcp_listen_pcbs.pcbs, &tcp_bound_pcbs, &tcp_active_pcbs, &tcp_tw_pcbs}
An array with all (non-temporary) PCB lists, mainly used for smaller code size.
u8_t tcp_timer [static] |
struct tcp_pcb* tcp_tmp_pcb |
struct tcp_pcb* tcp_tw_pcbs |
Generated on Tue Jul 12 2022 11:52:59 by
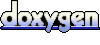