mbed-os for GR-LYCHEE
Dependents: mbed-os-example-blinky-gr-lychee GR-Boads_Camera_sample GR-Boards_Audio_Recoder GR-Boads_Camera_DisplayApp ... more
project.py
00001 """ The CLI entry point for exporting projects from the mbed tools to any of the 00002 supported IDEs or project structures. 00003 """ 00004 import sys 00005 from os.path import join, abspath, dirname, exists, basename 00006 ROOT = abspath(join(dirname(__file__), "..")) 00007 sys.path.insert(0, ROOT) 00008 00009 from shutil import move, rmtree 00010 from argparse import ArgumentParser 00011 from os.path import normpath, realpath 00012 00013 from tools.paths import EXPORT_DIR, MBED_HAL, MBED_LIBRARIES, MBED_TARGETS_PATH 00014 from tools.settings import BUILD_DIR 00015 from tools.export import EXPORTERS, mcu_ide_matrix 00016 from tools.tests import TESTS, TEST_MAP 00017 from tools.tests import test_known, test_name_known, Test 00018 from tools.targets import TARGET_NAMES 00019 from tools.utils import argparse_filestring_type, argparse_profile_filestring_type, argparse_many, args_error 00020 from tools.utils import argparse_force_lowercase_type 00021 from tools.utils import argparse_force_uppercase_type 00022 from tools.utils import print_large_string 00023 from tools.project_api import export_project, get_exporter_toolchain 00024 from tools.options import extract_profile, list_profiles 00025 00026 def setup_project (ide, target, program=None, source_dir=None, build=None, export_path=None): 00027 """Generate a name, if not provided, and find dependencies 00028 00029 Positional arguments: 00030 ide - IDE or project structure that will soon be exported to 00031 target - MCU that the project will build for 00032 00033 Keyword arguments: 00034 program - the index of a test program 00035 source_dir - the directory, or directories that contain all of the sources 00036 build - a directory that will contain the result of the export 00037 """ 00038 # Some libraries have extra macros (called by exporter symbols) to we need 00039 # to pass them to maintain compilation macros integrity between compiled 00040 # library and header files we might use with it 00041 if source_dir: 00042 # --source is used to generate IDE files to toolchain directly 00043 # in the source tree and doesn't generate zip file 00044 project_dir = export_path or source_dir[0] 00045 if program: 00046 project_name = TESTS[program] 00047 else: 00048 project_name = basename(normpath(realpath(source_dir[0]))) 00049 src_paths = source_dir 00050 lib_paths = None 00051 else: 00052 test = Test(program) 00053 if not build: 00054 # Substitute the mbed library builds with their sources 00055 if MBED_LIBRARIES in test.dependencies: 00056 test.dependencies.remove(MBED_LIBRARIES) 00057 test.dependencies.append(MBED_HAL) 00058 test.dependencies.append(MBED_TARGETS_PATH) 00059 00060 00061 src_paths = [test.source_dir] 00062 lib_paths = test.dependencies 00063 project_name = "_".join([test.id, ide, target]) 00064 project_dir = join(EXPORT_DIR, project_name) 00065 00066 return project_dir, project_name, src_paths, lib_paths 00067 00068 00069 def export (target, ide, build=None, src=None, macros=None, project_id=None, 00070 zip_proj=False, build_profile=None, export_path=None, silent=False): 00071 """Do an export of a project. 00072 00073 Positional arguments: 00074 target - MCU that the project will compile for 00075 ide - the IDE or project structure to export to 00076 00077 Keyword arguments: 00078 build - to use the compiled mbed libraries or not 00079 src - directory or directories that contain the source to export 00080 macros - extra macros to add to the project 00081 project_id - the name of the project 00082 clean - start from a clean state before exporting 00083 zip_proj - create a zip file or not 00084 00085 Returns an object of type Exporter (tools/exports/exporters.py) 00086 """ 00087 project_dir, name, src, lib = setup_project(ide, target, program=project_id, 00088 source_dir=src, build=build, export_path=export_path) 00089 00090 zip_name = name+".zip" if zip_proj else None 00091 00092 return export_project(src, project_dir, target, ide, name=name, 00093 macros=macros, libraries_paths=lib, zip_proj=zip_name, 00094 build_profile=build_profile, silent=silent) 00095 00096 00097 def main (): 00098 """Entry point""" 00099 # Parse Options 00100 parser = ArgumentParser() 00101 00102 targetnames = TARGET_NAMES 00103 targetnames.sort() 00104 toolchainlist = EXPORTERS.keys() 00105 toolchainlist.sort() 00106 00107 parser.add_argument("-m", "--mcu", 00108 metavar="MCU", 00109 type=argparse_force_uppercase_type(targetnames, "MCU"), 00110 help="generate project for the given MCU ({})".format( 00111 ', '.join(targetnames))) 00112 00113 parser.add_argument("-i", 00114 dest="ide", 00115 type=argparse_force_lowercase_type( 00116 toolchainlist, "toolchain"), 00117 help="The target IDE: %s"% str(toolchainlist)) 00118 00119 parser.add_argument("-c", "--clean", 00120 action="store_true", 00121 default=False, 00122 help="clean the export directory") 00123 00124 group = parser.add_mutually_exclusive_group(required=False) 00125 group.add_argument( 00126 "-p", 00127 type=test_known, 00128 dest="program", 00129 help="The index of the desired test program: [0-%s]"% (len(TESTS)-1)) 00130 00131 group.add_argument("-n", 00132 type=test_name_known, 00133 dest="program", 00134 help="The name of the desired test program") 00135 00136 parser.add_argument("-b", 00137 dest="build", 00138 default=False, 00139 action="store_true", 00140 help="use the mbed library build, instead of the sources") 00141 00142 group.add_argument("-L", "--list-tests", 00143 action="store_true", 00144 dest="list_tests", 00145 default=False, 00146 help="list available programs in order and exit") 00147 00148 group.add_argument("-S", "--list-matrix", 00149 action="store_true", 00150 dest="supported_ides", 00151 default=False, 00152 help="displays supported matrix of MCUs and IDEs") 00153 00154 parser.add_argument("-E", 00155 action="store_true", 00156 dest="supported_ides_html", 00157 default=False, 00158 help="writes tools/export/README.md") 00159 00160 parser.add_argument("--source", 00161 action="append", 00162 type=argparse_filestring_type, 00163 dest="source_dir", 00164 default=[], 00165 help="The source (input) directory") 00166 00167 parser.add_argument("-D", 00168 action="append", 00169 dest="macros", 00170 help="Add a macro definition") 00171 00172 parser.add_argument("--profile", dest="profile", action="append", 00173 type=argparse_profile_filestring_type, 00174 help="Build profile to use. Can be either path to json" \ 00175 "file or one of the default one ({})".format(", ".join(list_profiles())), 00176 default=[]) 00177 00178 parser.add_argument("--update-packs", 00179 dest="update_packs", 00180 action="store_true", 00181 default=False) 00182 00183 options = parser.parse_args() 00184 00185 # Print available tests in order and exit 00186 if options.list_tests is True: 00187 print '\n'.join([str(test) for test in sorted(TEST_MAP.values())]) 00188 sys.exit() 00189 00190 # Only prints matrix of supported IDEs 00191 if options.supported_ides: 00192 print_large_string(mcu_ide_matrix()) 00193 exit(0) 00194 00195 # Only prints matrix of supported IDEs 00196 if options.supported_ides_html: 00197 html = mcu_ide_matrix(verbose_html=True) 00198 try: 00199 with open("./export/README.md", "w") as readme: 00200 readme.write("Exporter IDE/Platform Support\n") 00201 readme.write("-----------------------------------\n") 00202 readme.write("\n") 00203 readme.write(html) 00204 except IOError as exc: 00205 print "I/O error({0}): {1}".format(exc.errno, exc.strerror) 00206 except: 00207 print "Unexpected error:", sys.exc_info()[0] 00208 raise 00209 exit(0) 00210 00211 if options.update_packs: 00212 from tools.arm_pack_manager import Cache 00213 cache = Cache(True, True) 00214 cache.cache_descriptors() 00215 00216 # Target 00217 if not options.mcu: 00218 args_error(parser, "argument -m/--mcu is required") 00219 00220 # Toolchain 00221 if not options.ide: 00222 args_error(parser, "argument -i is required") 00223 00224 # Clean Export Directory 00225 if options.clean: 00226 if exists(EXPORT_DIR): 00227 rmtree(EXPORT_DIR) 00228 00229 for mcu in options.mcu: 00230 zip_proj = not bool(options.source_dir) 00231 00232 if (options.program is None) and (not options.source_dir): 00233 args_error(parser, "one of -p, -n, or --source is required") 00234 # Export to selected toolchain 00235 exporter, toolchain_name = get_exporter_toolchain(options.ide) 00236 if options.mcu not in exporter.TARGETS: 00237 args_error(parser, "%s not supported by %s"%(options.mcu,options.ide)) 00238 profile = extract_profile(parser, options, toolchain_name, fallback="debug") 00239 if options.clean: 00240 rmtree(BUILD_DIR) 00241 export(options.mcu, options.ide, build=options.build, 00242 src=options.source_dir, macros=options.macros, 00243 project_id=options.program, zip_proj=zip_proj, 00244 build_profile=profile) 00245 00246 00247 if __name__ == "__main__": 00248 main()
Generated on Tue Jul 12 2022 11:02:31 by
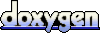