Library to simplify using capture pins on hardware.
TimerCapture Class Reference
A class that will start TIMER2 on the LPC1768 and configure it to use one of the two capture pins (p29 or p30). More...
#include <TimerCapture.h>
Public Member Functions | |
TimerCapture (PinName pCapturePin) | |
Configures registers to use the given pin as a capture pin. | |
uint32_t | getTime () |
Get the time captured by the capture pin's register. | |
Static Public Member Functions | |
static void | startTimer () |
Starts the TIMER2 timer, and configures it if it's not already configured. | |
static bool | isRunning () |
Checks if the TIMER2 timer is running. | |
static void | stopTimer () |
Stops the TIMER2 timer. | |
static void | resetTimer () |
Resets the TIMER2 timer. |
Detailed Description
A class that will start TIMER2 on the LPC1768 and configure it to use one of the two capture pins (p29 or p30).
Capture pins allow the hardware to "timestamp" when a pin goes high or low, depending on the configuration. For more information, consult the LPC1768 user manual.
Example
The following example will set up a capture pin, and will print out the timestamp from the capture register whenever a keypress is detected on the USB serial interface.
#include "mbed.h" #include "TimerCapture.h" Serial pc(USBTX, USBRX); TimerCapture * capture; void handleInput() { pc.getc(); // clear the interrupt handler. printf("Capture register reads: %d\r\n", capture->getTime()); } int main() { pc.printf("Timer Capture Program Starting\r\n"); TimerCapture::startTimer(); capture = new TimerCapture(p29); pc.attach(&handleInput); }
- Attention:
- Because this code operates directly on TIMER2, it has the potential to (a) mess with existing code or (b) cause errors when you instantiate too many objects. Make sure to read this documentation thoroughly, else you may find yourself staring at lights of death!
Definition at line 37 of file TimerCapture.h.
Constructor & Destructor Documentation
TimerCapture | ( | PinName | pCapturePin ) |
Configures registers to use the given pin as a capture pin.
Initializes an object that will configure and read from a capture pin. If the timer is not already running, it will get configured.
- Attention:
- There are only two pins that will act as capture pins for TIMER2. These are P0.4 (DIP p30) and P0.5(DIP p29).
- Warning:
- This will cause a runtime error if:
- pCapturePin is not set to an actual capture pin on the LPC1768.
- The specified capture pin is already in use (i.e. already configured).
- The timer hasn't yet been started (i.e. peripheral is off)
- Parameters:
-
pCapturePin The PinName intended to be used for the capture.
Definition at line 5 of file TimerCapture.cpp.
Member Function Documentation
uint32_t getTime | ( | ) |
Get the time captured by the capture pin's register.
- Returns:
- Time in miliseconds since the timer got started.
Definition at line 93 of file TimerCapture.cpp.
bool isRunning | ( | ) | [static] |
Checks if the TIMER2 timer is running.
- Returns:
- True if the timer is running, false otherwise.
Definition at line 81 of file TimerCapture.cpp.
void resetTimer | ( | ) | [static] |
Resets the TIMER2 timer.
- Attention:
- This will also clear both timer registers. You've been warned!
Definition at line 85 of file TimerCapture.cpp.
void startTimer | ( | ) | [static] |
Starts the TIMER2 timer, and configures it if it's not already configured.
Definition at line 64 of file TimerCapture.cpp.
void stopTimer | ( | ) | [static] |
Stops the TIMER2 timer.
Definition at line 75 of file TimerCapture.cpp.
Generated on Thu Jul 14 2022 05:37:43 by
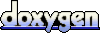