
Port of TI's CC3100 Websock camera demo. Using FreeRTOS, mbedTLS, also parts of Arducam for cams ov5642 and 0v2640. Can also use MT9D111. Work in progress. Be warned some parts maybe a bit flacky. This is for Seeed Arch max only, for an M3, see the demo for CM3 using the 0v5642 aducam mini.
Socket
Functions | |
int16_t | sl_Socket (int16_t Domain, int16_t Type, int16_t Protocol) |
create an endpoint for communication | |
int16_t | sl_Close (int16_t sd) |
gracefully close socket | |
int16_t | sl_Accept (int16_t sd, SlSockAddr_t *addr, SlSocklen_t *addrlen) |
Accept a connection on a socket. | |
int16_t | sl_Bind (int16_t sd, const SlSockAddr_t *addr, int16_t addrlen) |
assign a name to a socket | |
int16_t | sl_Listen (int16_t sd, int16_t backlog) |
listen for connections on a socket | |
int16_t | sl_Connect (int16_t sd, const SlSockAddr_t *addr, int16_t addrlen) |
Initiate a connection on a socket. | |
int16_t | sl_Select (int16_t nfds, SlFdSet_t *readsds, SlFdSet_t *writesds, SlFdSet_t *exceptsds, SlTimeval_t *timeout) |
Monitor socket activity. | |
void | SL_FD_SET (int16_t fd, SlFdSet_t *fdset) |
Select's SlFdSet_t SET function. | |
void | SL_FD_CLR (int16_t fd, SlFdSet_t *fdset) |
Select's SlFdSet_t CLR function. | |
int16_t | SL_FD_ISSET (int16_t fd, SlFdSet_t *fdset) |
Select's SlFdSet_t ISSET function. | |
void | SL_FD_ZERO (SlFdSet_t *fdset) |
Select's SlFdSet_t ZERO function. | |
int16_t | sl_SetSockOpt (int16_t sd, int16_t level, int16_t optname, const void *optval, SlSocklen_t optlen) |
set socket options | |
int16_t | sl_GetSockOpt (int16_t sd, int16_t level, int16_t optname, void *optval, SlSocklen_t *optlen) |
Get socket options. | |
int16_t | sl_Recv (int16_t sd, void *buf, int16_t Len, int16_t flags) |
read data from TCP socket | |
int16_t | sl_RecvFrom (int16_t sd, void *buf, int16_t Len, int16_t flags, SlSockAddr_t *from, SlSocklen_t *fromlen) |
read data from socket | |
int16_t | sl_Send (int16_t sd, const void *buf, int16_t Len, int16_t flags) |
write data to TCP socket | |
int16_t | sl_SendTo (int16_t sd, const void *buf, int16_t Len, int16_t flags, const SlSockAddr_t *to, SlSocklen_t tolen) |
write data to socket | |
uint32_t | sl_Htonl (uint32_t val) |
Reorder the bytes of a 32-bit unsigned value. | |
uint16_t | sl_Htons (uint16_t val) |
Reorder the bytes of a 16-bit unsigned value. |
Function Documentation
int16_t sl_Accept | ( | int16_t | sd, |
SlSockAddr_t * | addr, | ||
SlSocklen_t * | addrlen | ||
) | [inherited] |
Accept a connection on a socket.
This function is used with connection-based socket types (SOCK_STREAM). It extracts the first connection request on the queue of pending connections, creates a new connected socket, and returns a new file descriptor referring to that socket. The newly created socket is not in the listening state. The original socket sd is unaffected by this call. The argument sd is a socket that has been created with sl_Socket(), bound to a local address with sl_Bind(), and is listening for connections after a sl_Listen(). The argument addr is a pointer to a sockaddr structure. This structure is filled in with the address of the peer socket, as known to the communications layer. The exact format of the address returned addr is determined by the socket's address family. The addrlen argument is a value-result argument: it should initially contain the size of the structure pointed to by addr, on return it will contain the actual length (in bytes) of the address returned.
- Parameters:
-
[in] sd socket descriptor (handle) [out] addr the argument addr is a pointer to a sockaddr structure. This structure is filled in with the address of the peer socket, as known to the communications layer. The exact format of the address returned addr is determined by the socket's address
sockaddr:
- code for the address format. On this version only AF_INET is supported.
- socket address, the length depends on the code format[out] addrlen the addrlen argument is a value-result argument: it should initially contain the size of the structure pointed to by addr
- Returns:
- On success, a socket handle. On a non-blocking accept a possible negative value is SL_EAGAIN. On failure, negative value. SL_POOL_IS_EMPTY may be return in case there are no resources in the system In this case try again later or increase MAX_CONCURRENT_ACTIONS
- See also:
- sl_Socket sl_Bind sl_Listen
- Note:
- belongs to server_side
- Warning:
Definition at line 606 of file cc3100_socket.cpp.
int16_t sl_Bind | ( | int16_t | sd, |
const SlSockAddr_t * | addr, | ||
int16_t | addrlen | ||
) | [inherited] |
assign a name to a socket
This function gives the socket the local address addr. addr is addrlen bytes long. Traditionally, this is called When a socket is created with socket, it exists in a name space (address family) but has no name assigned. It is necessary to assign a local address before a SOCK_STREAM socket may receive connections.
- Parameters:
-
[in] sd socket descriptor (handle) [in] addr specifies the destination addrs
sockaddr:
- code for the address format. On this version only AF_INET is supported.
- socket address, the length depends on the code format[in] addrlen contains the size of the structure pointed to by addr
- Returns:
- On success, zero is returned. On error, a negative error code is returned.
- See also:
- sl_Socket sl_Accept sl_Listen
- Note:
- belongs to basic_api
- Warning:
Definition at line 239 of file cc3100_socket.cpp.
int16_t sl_Close | ( | int16_t | sd ) | [inherited] |
gracefully close socket
This function causes the system to release resources allocated to a socket.
In case of TCP, the connection is terminated.
- Parameters:
-
[in] sd socket handle (received in sl_Socket)
- Returns:
- On success, zero is returned. On error, a negative number is returned.
- See also:
- sl_Socket
- Note:
- belongs to ext_api
- Warning:
Definition at line 218 of file cc3100_socket.cpp.
int16_t sl_Connect | ( | int16_t | sd, |
const SlSockAddr_t * | addr, | ||
int16_t | addrlen | ||
) | [inherited] |
Initiate a connection on a socket.
Function connects the socket referred to by the socket descriptor sd, to the address specified by addr. The addrlen argument specifies the size of addr. The format of the address in addr is determined by the address space of the socket. If it is of type SOCK_DGRAM, this call specifies the peer with which the socket is to be associated; this address is that to which datagrams are to be sent, and the only address from which datagrams are to be received. If the socket is of type SOCK_STREAM, this call attempts to make a connection to another socket. The other socket is specified by address, which is an address in the communications space of the socket.
- Parameters:
-
[in] sd socket descriptor (handle) [in] addr specifies the destination addr
sockaddr:
- code for the address format. On this version only AF_INET is supported.
- socket address, the length depends on the code format[in] addrlen contains the size of the structure pointed to by addr
- Returns:
- On success, a socket handle. On a non-blocking connect a possible negative value is SL_EALREADY. On failure, negative value. SL_POOL_IS_EMPTY may be return in case there are no resources in the system In this case try again later or increase MAX_CONCURRENT_ACTIONS
- See also:
- sl_Socket
- Note:
- belongs to client_side
- Warning:
Definition at line 439 of file cc3100_socket.cpp.
void SL_FD_CLR | ( | int16_t | fd, |
SlFdSet_t * | fdset | ||
) | [inherited] |
Select's SlFdSet_t CLR function.
Clears current socket descriptor on SlFdSet_t container
Definition at line 930 of file cc3100_socket.cpp.
int16_t SL_FD_ISSET | ( | int16_t | fd, |
SlFdSet_t * | fdset | ||
) | [inherited] |
Select's SlFdSet_t ISSET function.
Checks if current socket descriptor is set (TRUE/FALSE)
- Returns:
- Returns TRUE if set, FALSE if unset
Definition at line 937 of file cc3100_socket.cpp.
void SL_FD_SET | ( | int16_t | fd, |
SlFdSet_t * | fdset | ||
) | [inherited] |
Select's SlFdSet_t SET function.
Sets current socket descriptor on SlFdSet_t container
Definition at line 923 of file cc3100_socket.cpp.
void SL_FD_ZERO | ( | SlFdSet_t * | fdset ) | [inherited] |
Select's SlFdSet_t ZERO function.
Clears all socket descriptors from SlFdSet_t
Definition at line 947 of file cc3100_socket.cpp.
int16_t sl_GetSockOpt | ( | int16_t | sd, |
int16_t | level, | ||
int16_t | optname, | ||
void * | optval, | ||
SlSocklen_t * | optlen | ||
) | [inherited] |
Get socket options.
This function manipulate the options associated with a socket. Options may exist at multiple protocol levels; they are always present at the uppermost socket level.
When manipulating socket options the level at which the option resides and the name of the option must be specified. To manipulate options at the socket level, level is specified as SOL_SOCKET. To manipulate options at any other level the protocol number of the appropriate proto- col controlling the option is supplied. For example, to indicate that an option is to be interpreted by the TCP protocol, level should be set to the protocol number of TCP;
The parameters optval and optlen are used to access optval - ues for setsockopt(). For getsockopt() they identify a buffer in which the value for the requested option(s) are to be returned. For getsockopt(), optlen is a value-result parameter, initially containing the size of the buffer pointed to by option_value, and modified on return to indicate the actual size of the value returned. If no option value is to be supplied or returned, option_value may be NULL.
- Parameters:
-
[in] sd socket handle [in] level defines the protocol level for this option [in] optname defines the option name to interrogate [out] optval specifies a value for the option [out] optlen specifies the length of the option value
- Returns:
- On success, zero is returned. On error, a negative value is returned.
- See also:
- sl_SetSockOpt
- Note:
- See sl_SetSockOpt belongs to ext_api
- Warning:
Definition at line 808 of file cc3100_socket.cpp.
uint32_t sl_Htonl | ( | uint32_t | val ) | [inherited] |
Reorder the bytes of a 32-bit unsigned value.
This function is used to Reorder the bytes of a 32-bit unsigned value from processor order to network order.
- Parameters:
-
[in] var variable to reorder
- Returns:
- Return the reorder variable,
- See also:
- sl_SendTo sl_Bind sl_Connect sl_RecvFrom sl_Accept
- Note:
- belongs to send_api
- Warning:
Definition at line 683 of file cc3100_socket.cpp.
uint16_t sl_Htons | ( | uint16_t | val ) | [inherited] |
Reorder the bytes of a 16-bit unsigned value.
This function is used to Reorder the bytes of a 16-bit unsigned value from processor order to network order.
- Parameters:
-
[in] var variable to reorder
- Returns:
- Return the reorder variable,
- See also:
- sl_SendTo sl_Bind sl_Connect sl_RecvFrom sl_Accept
- Note:
- belongs to send_api
- Warning:
Definition at line 701 of file cc3100_socket.cpp.
int16_t sl_Listen | ( | int16_t | sd, |
int16_t | backlog | ||
) | [inherited] |
listen for connections on a socket
The willingness to accept incoming connections and a queue limit for incoming connections are specified with listen(), and then the connections are accepted with accept. The listen() call applies only to sockets of type SOCK_STREAM The backlog parameter defines the maximum length the queue of pending connections may grow to.
- Parameters:
-
[in] sd socket descriptor (handle) [in] backlog specifies the listen queue depth.
- Returns:
- On success, zero is returned. On error, a negative error code is returned.
- See also:
- sl_Socket sl_Accept sl_Bind
- Note:
- belongs to server_side
- Warning:
Definition at line 578 of file cc3100_socket.cpp.
int16_t sl_Recv | ( | int16_t | sd, |
void * | buf, | ||
int16_t | Len, | ||
int16_t | flags | ||
) | [inherited] |
read data from TCP socket
function receives a message from a connection-mode socket
- Parameters:
-
[in] sd socket handle [out] buf Points to the buffer where the message should be stored. [in] Len Specifies the length in bytes of the buffer pointed to by the buffer argument. Range: 1-16000 bytes [in] flags Specifies the type of message reception. On this version, this parameter is not supported.
- Returns:
- return the number of bytes received, or a negative value if an error occurred. using a non-blocking recv a possible negative value is SL_EAGAIN. SL_POOL_IS_EMPTY may be return in case there are no resources in the system In this case try again later or increase MAX_CONCURRENT_ACTIONS
- See also:
- sl_RecvFrom
- Note:
- belongs to recv_api
- Warning:
- Examples:
An example of receiving data using TCP socket: SlSockAddrIn_t Addr; SlSockAddrIn_t LocalAddr; int16_t AddrSize = sizeof(SlSockAddrIn_t); int16_t SockID, newSockID; int16_t Status; int8_t Buf[RECV_BUF_LEN]; LocalAddr.sin_family = SL_AF_INET; LocalAddr.sin_port = sl_Htons(5001); LocalAddr.sin_addr.s_addr = 0; Addr.sin_family = SL_AF_INET; Addr.sin_port = sl_Htons(5001); Addr.sin_addr.s_addr = sl_Htonl(SL_IPV4_VAL(10,1,1,200)); SockID = sl_Socket(SL_AF_INET,SL_SOCK_STREAM, 0); Status = sl_Bind(SockID, (SlSockAddr_t *)&LocalAddr, AddrSize); Status = sl_Listen(SockID, 0); newSockID = sl_Accept(SockID, (SlSockAddr_t*)&Addr, (SlSocklen_t*) &AddrSize); Status = sl_Recv(newSockID, Buf, 1460, 0);
Example code for Rx transceiver mode using a raw socket int8_t buffer[1536]; int16_t sd; uint16_t size; SlTransceiverRxOverHead_t *transHeader; sd = sl_Socket(SL_AF_RF,SL_SOCK_RAW,11); // channel 11 while(1) { size = sl_Recv(sd,buffer,1536,0); transHeader = (SlTransceiverRxOverHead_t *)buffer; printf("RSSI is %d frame type is 0x%x size %d\n",transHeader->rssi,buffer[sizeof(SlTransceiverRxOverHead_t)],size); }
Definition at line 729 of file cc3100_socket.cpp.
int16_t sl_RecvFrom | ( | int16_t | sd, |
void * | buf, | ||
int16_t | Len, | ||
int16_t | flags, | ||
SlSockAddr_t * | from, | ||
SlSocklen_t * | fromlen | ||
) | [inherited] |
read data from socket
function receives a message from a connection-mode or connectionless-mode socket
- Parameters:
-
[in] sd socket handle [out] buf Points to the buffer where the message should be stored. [in] Len Specifies the length in bytes of the buffer pointed to by the buffer argument. Range: 1-16000 bytes [in] flags Specifies the type of message reception. On this version, this parameter is not supported. [in] from pointer to an address structure indicating the source address.
sockaddr:
- code for the address format. On this version only AF_INET is supported.
- socket address, the length depends on the code format[in] fromlen source address structure size. This parameter MUST be set to the size of the structure pointed to by addr.
- Returns:
- return the number of bytes received, or a negative value if an error occurred. using a non-blocking recv a possible negative value is SL_EAGAIN. SL_RET_CODE_INVALID_INPUT (-2) will be returned if fromlen has incorrect length. SL_POOL_IS_EMPTY may be return in case there are no resources in the system In this case try again later or increase MAX_CONCURRENT_ACTIONS
- See also:
- sl_Recv
- Note:
- belongs to recv_api
- Warning:
- Example:
An example of receiving data: SlSockAddrIn_t Addr; SlSockAddrIn_t LocalAddr; int16_t AddrSize = sizeof(SlSockAddrIn_t); int16_t SockID; int16_t Status; int8_t Buf[RECV_BUF_LEN]; LocalAddr.sin_family = SL_AF_INET; LocalAddr.sin_port = sl_Htons(5001); LocalAddr.sin_addr.s_addr = 0; SockID = sl_Socket(SL_AF_INET,SL_SOCK_DGRAM, 0); Status = sl_Bind(SockID, (SlSockAddr_t *)&LocalAddr, AddrSize); Status = sl_RecvFrom(SockID, Buf, 1472, 0, (SlSockAddr_t *)&Addr, (SlSocklen_t*)&AddrSize);
Definition at line 363 of file cc3100_socket.cpp.
int16_t sl_Select | ( | int16_t | nfds, |
SlFdSet_t * | readsds, | ||
SlFdSet_t * | writesds, | ||
SlFdSet_t * | exceptsds, | ||
SlTimeval_t * | timeout | ||
) | [inherited] |
Monitor socket activity.
Select allow a program to monitor multiple file descriptors, waiting until one or more of the file descriptors become "ready" for some class of I/O operation
- Parameters:
-
[in] nfds the highest-numbered file descriptor in any of the three sets, plus 1. [out] readsds socket descriptors list for read monitoring and accept monitoring [out] writesds socket descriptors list for connect monitoring only, write monitoring is not supported, non blocking connect is supported [out] exceptsds socket descriptors list for exception monitoring, not supported. [in] timeout is an upper bound on the amount of time elapsed before select() returns. Null or above 0xffff seconds means infinity timeout. The minimum timeout is 10 milliseconds, less than 10 milliseconds will be set automatically to 10 milliseconds. Max microseconds supported is 0xfffc00.
- Returns:
- On success, select() returns the number of file descriptors contained in the three returned descriptor sets (that is, the total number of bits that are set in readfds, writefds, exceptfds) which may be zero if the timeout expires before anything interesting happens. On error, a negative value is returned. readsds - return the sockets on which Read request will return without delay with valid data. writesds - return the sockets on which Write request will return without delay. exceptsds - return the sockets closed recently. SL_POOL_IS_EMPTY may be return in case there are no resources in the system In this case try again later or increase MAX_CONCURRENT_ACTIONS
- See also:
- sl_Socket
- Note:
- If the timeout value set to less than 5ms it will automatically set to 5ms to prevent overload of the system belongs to basic_api
Only one sl_Select can be handled at a time. Calling this API while the same command is called from another thread, may result in one of the two scenarios: 1. The command will wait (internal) until the previous command finish, and then be executed. 2. There are not enough resources and SL_POOL_IS_EMPTY error will return. In this case, MAX_CONCURRENT_ACTIONS can be increased (result in memory increase) or try again later to issue the command.
- Warning:
Definition at line 854 of file cc3100_socket.cpp.
int16_t sl_Send | ( | int16_t | sd, |
const void * | buf, | ||
int16_t | Len, | ||
int16_t | flags | ||
) | [inherited] |
write data to TCP socket
This function is used to transmit a message to another socket. Returns immediately after sending data to device. In case of TCP failure an async event SL_SOCKET_TX_FAILED_EVENT is going to be received. In case of a RAW socket (transceiver mode), extra 4 bytes should be reserved at the end of the frame data buffer for WLAN FCS
- Parameters:
-
[in] sd socket handle [in] buf Points to a buffer containing the message to be sent [in] Len message size in bytes. Range: 1-1460 bytes [in] flags Specifies the type of message transmission. On this version, this parameter is not supported for TCP. For transceiver mode, the SL_RAW_RF_TX_PARAMS macro can be used to determine transmission parameters (channel,rate,tx_power,preamble)
- Returns:
- Return the number of bytes transmitted, or -1 if an error occurred
- See also:
- sl_SendTo
- Note:
- belongs to send_api
- Warning:
- Example:
An example of sending data: SlSockAddrIn_t Addr; int16_t AddrSize = sizeof(SlSockAddrIn_t); int16_t SockID; int16_t Status; int8_t Buf[SEND_BUF_LEN]; Addr.sin_family = SL_AF_INET; Addr.sin_port = sl_Htons(5001); Addr.sin_addr.s_addr = sl_Htonl(SL_IPV4_VAL(10,1,1,200)); SockID = sl_Socket(SL_AF_INET,SL_SOCK_STREAM, 0); Status = sl_Connect(SockID, (SlSockAddr_t *)&Addr, AddrSize); Status = sl_Send(SockID, Buf, 1460, 0 );
Definition at line 516 of file cc3100_socket.cpp.
int16_t sl_SendTo | ( | int16_t | sd, |
const void * | buf, | ||
int16_t | Len, | ||
int16_t | flags, | ||
const SlSockAddr_t * | to, | ||
SlSocklen_t | tolen | ||
) | [inherited] |
write data to socket
This function is used to transmit a message to another socket (connection less socket SOCK_DGRAM, SOCK_RAW). Returns immediately after sending data to device. In case of transmission failure an async event SL_SOCKET_TX_FAILED_EVENT is going to be received.
- Parameters:
-
[in] sd socket handle [in] buf Points to a buffer containing the message to be sent [in] Len message size in bytes. Range: 1-1460 bytes [in] flags Specifies the type of message transmission. On this version, this parameter is not supported [in] to pointer to an address structure indicating the destination address.
sockaddr:
- code for the address format. On this version only AF_INET is supported.
- socket address, the length depends on the code format[in] tolen destination address structure size
- Returns:
- Return the number of transmitted bytes, or -1 if an error occurred
- See also:
- sl_Send
- Note:
- belongs to send_api
- Warning:
- Example:
An example of sending data: SlSockAddrIn_t Addr; int16_t AddrSize = sizeof(SlSockAddrIn_t); int16_t SockID; int16_t Status; int8_t Buf[SEND_BUF_LEN]; Addr.sin_family = SL_AF_INET; Addr.sin_port = sl_Htons(5001); Addr.sin_addr.s_addr = sl_Htonl(SL_IPV4_VAL(10,1,1,200)); SockID = sl_Socket(SL_AF_INET,SL_SOCK_DGRAM, 0); Status = sl_SendTo(SockID, Buf, 1472, 0, (SlSockAddr_t *)&Addr, AddrSize);
Definition at line 286 of file cc3100_socket.cpp.
int16_t sl_SetSockOpt | ( | int16_t | sd, |
int16_t | level, | ||
int16_t | optname, | ||
const void * | optval, | ||
SlSocklen_t | optlen | ||
) | [inherited] |
set socket options
This function manipulate the options associated with a socket. Options may exist at multiple protocol levels; they are always present at the uppermost socket level.
When manipulating socket options the level at which the option resides and the name of the option must be specified. To manipulate options at the socket level, level is specified as SOL_SOCKET. To manipulate options at any other level the protocol number of the appropriate proto- col controlling the option is supplied. For example, to indicate that an option is to be interpreted by the TCP protocol, level should be set to the protocol number of TCP;
The parameters optval and optlen are used to access optval - ues for setsockopt(). For getsockopt() they identify a buffer in which the value for the requested option(s) are to be returned. For getsockopt(), optlen is a value-result parameter, initially containing the size of the buffer pointed to by option_value, and modified on return to indicate the actual size of the value returned. If no option value is to be supplied or returned, option_value may be NULL.
- Parameters:
-
[in] sd socket handle [in] level defines the protocol level for this option - SL_SOL_SOCKET Socket level configurations (L4, transport layer)
- SL_IPPROTO_IP IP level configurations (L3, network layer)
- SL_SOL_PHY_OPT Link level configurations (L2, link layer)
[in] optname defines the option name to interrogate - SL_SOL_SOCKET
- SL_SO_KEEPALIVE
Enable/Disable periodic keep alive. Keeps TCP connections active by enabling the periodic transmission of messages
Timeout is 5 minutes.
Default: Enabled
This options takes SlSockKeepalive_t struct as parameter - SL_SO_RCVTIMEO
Sets the timeout value that specifies the maximum amount of time an input function waits until it completes.
Default: No timeout
This options takes SlTimeval_t struct as parameter - SL_SO_RCVBUF
Sets tcp max recv window size.
This options takes SlSockWinsize_t struct as parameter - SL_SO_NONBLOCKING
Sets socket to non-blocking operation Impacts: connect, accept, send, sendto, recv and recvfrom.
Default: Blocking. This options takes SlSockNonblocking_t struct as parameter - SL_SO_SECMETHOD
Sets method to tcp secured socket (SL_SEC_SOCKET)
Default: SL_SO_SEC_METHOD_SSLv3_TLSV1_2
This options takes SlSockSecureMethod struct as parameter - SL_SO_SEC_MASK
Sets specific cipher to tcp secured socket (SL_SEC_SOCKET)
Default: "Best" cipher suitable to method
This options takes SlSockSecureMask struct as parameter - SL_SO_SECURE_FILES_CA_FILE_NAME
Map secured socket to CA file by name
This options takes uint8_t buffer as parameter - SL_SO_SECURE_FILES_PRIVATE_KEY_FILE_NAME
Map secured socket to private key by name
This options takes uint8_t buffer as parameter - SL_SO_SECURE_FILES_CERTIFICATE_FILE_NAME
Map secured socket to certificate file by name
This options takes uint8_t buffer as parameter - SL_SO_SECURE_FILES_DH_KEY_FILE_NAME
Map secured socket to Diffie Hellman file by name
This options takes uint8_t buffer as parameter - SL_SO_CHANGE_CHANNEL
Sets channel in transceiver mode. This options takes uint32_t as channel number parameter
- SL_SO_KEEPALIVE
- SL_IPPROTO_IP
- SL_IP_MULTICAST_TTL
Set the time-to-live value of outgoing multicast packets for this socket.
This options takes uint8_t as parameter - SL_IP_ADD_MEMBERSHIP
UDP socket, Join a multicast group.
This options takes SlSockIpMreq struct as parameter - SL_IP_DROP_MEMBERSHIP
UDP socket, Leave a multicast group
This options takes SlSockIpMreq struct as parameter - SL_IP_RAW_RX_NO_HEADER
Raw socket remove IP header from received data.
Default: data includes ip header
This options takes uint32_t as parameter - SL_IP_HDRINCL
RAW socket only, the IPv4 layer generates an IP header when sending a packet unless
the IP_HDRINCL socket option is enabled on the socket.
When it is enabled, the packet must contain an IP header.
Default: disabled, IPv4 header generated by Network Stack
This options takes uint32_t as parameter - SL_IP_RAW_IPV6_HDRINCL (inactive)
RAW socket only, the IPv6 layer generates an IP header when sending a packet unless
the IP_HDRINCL socket option is enabled on the socket. When it is enabled, the packet must contain an IP header
Default: disabled, IPv4 header generated by Network Stack
This options takes uint32_t as parameter
- SL_IP_MULTICAST_TTL
- SL_SOL_PHY_OPT
- SL_SO_PHY_RATE
RAW socket, set WLAN PHY transmit rate
The values are based on RateIndex_e
This options takes uint32_t as parameter - SL_SO_PHY_TX_POWER
RAW socket, set WLAN PHY TX power
Valid rage is 1-15
This options takes uint32_t as parameter - SL_SO_PHY_NUM_FRAMES_TO_TX
RAW socket, set number of frames to transmit in transceiver mode. Default: 1 packet This options takes uint32_t as parameter - SL_SO_PHY_PREAMBLE
RAW socket, set WLAN PHY preamble for Long/Short
This options takes uint32_t as parameter
- SL_SO_PHY_RATE
[in] optval specifies a value for the option [in] optlen specifies the length of the option value
- Returns:
- On success, zero is returned. On error, a negative value is returned.
- See also:
- sl_getsockopt
- Note:
- belongs to basic_api
- Warning:
- Examples:
- SL_SO_KEEPALIVE: (disable Keepalive)
SlSockKeepalive_t enableOption; enableOption.KeepaliveEnabled = 0; sl_SetSockOpt(SockID,SL_SOL_SOCKET,SL_SO_KEEPALIVE, (uint8_t *)&enableOption,sizeof(enableOption));
- SL_SO_RCVTIMEO:
struct SlTimeval_t timeVal; timeVal.tv_sec = 1; // Seconds timeVal.tv_usec = 0; // Microseconds. 10000 microseconds resolution sl_SetSockOpt(SockID,SL_SOL_SOCKET,SL_SO_RCVTIMEO, (uint8_t *)&timeVal, sizeof(timeVal)); // Enable receive timeout
- SL_SO_RCVBUF:
SlSockWinsize_t size; size.Winsize = 3000; // bytes sl_SetSockOpt(SockID,SL_SOL_SOCKET,SL_SO_RCVBUF, (uint8_t *)&size, sizeof(size));
- SL_SO_NONBLOCKING:
SlSockNonblocking_t enableOption; enableOption.NonblockingEnabled = 1; sl_SetSockOpt(SockID,SL_SOL_SOCKET,SL_SO_NONBLOCKING, (uint8_t *)&enableOption,sizeof(enableOption)); // Enable/disable nonblocking mode
- SL_SO_SECMETHOD:
SlSockSecureMethod method; method.secureMethod = SL_SO_SEC_METHOD_SSLV3; // security method we want to use SockID = sl_Socket(SL_AF_INET,SL_SOCK_STREAM, SL_SEC_SOCKET); sl_SetSockOpt(SockID, SL_SOL_SOCKET, SL_SO_SECMETHOD, (uint8_t *)&method, sizeof(method));
- SL_SO_SECURE_MASK:
SlSockSecureMask cipher; cipher.secureMask = SL_SEC_MASK_SSL_RSA_WITH_RC4_128_SHA; // cipher type SockID = sl_Socket(SL_AF_INET,SL_SOCK_STREAM, SL_SEC_SOCKET); sl_SetSockOpt(SockID, SL_SOL_SOCKET, SL_SO_SEC_MASK,(uint8_t *)&cipher, sizeof(cipher));
- SL_SO_SECURE_FILES_CA_FILE_NAME:
sl_SetSockOpt(SockID,SL_SOL_SOCKET,SL_SO_SECURE_FILES_CA_FILE_NAME,"exuifaxCaCert.der",strlen("exuifaxCaCert.der"));
- SL_SO_SECURE_FILES_PRIVATE_KEY_FILE_NAME:
sl_SetSockOpt(SockID,SL_SOL_SOCKET,SL_SO_SECURE_FILES_PRIVATE_KEY_FILE_NAME,"myPrivateKey.der",strlen("myPrivateKey.der"));
- SL_SO_SECURE_FILES_CERTIFICATE_FILE_NAME:
sl_SetSockOpt(SockID,SL_SOL_SOCKET,SL_SO_SECURE_FILES_CERTIFICATE_FILE_NAME,"myCertificate.der",strlen("myCertificate.der"));
- SL_SO_SECURE_FILES_DH_KEY_FILE_NAME:
sl_SetSockOpt(SockID,SL_SOL_SOCKET,SL_SO_SECURE_FILES_DH_KEY_FILE_NAME,"myDHinServerMode.der",strlen("myDHinServerMode.der"));
- SL_IP_MULTICAST_TTL:
uint8_t ttl = 20; sl_SetSockOpt(SockID, SL_IPPROTO_IP, SL_IP_MULTICAST_TTL, &ttl, sizeof(ttl));
- SL_IP_ADD_MEMBERSHIP:
SlSockIpMreq mreq; sl_SetSockOpt(SockID, SL_IPPROTO_IP, SL_IP_ADD_MEMBERSHIP, &mreq, sizeof(mreq));
- SL_IP_DROP_MEMBERSHIP:
SlSockIpMreq mreq; sl_SetSockOpt(SockID, SL_IPPROTO_IP, SL_IP_DROP_MEMBERSHIP, &mreq, sizeof(mreq));
- SL_SO_CHANGE_CHANNEL:
uint32_t newChannel = 6; // range is 1-13 sl_SetSockOpt(SockID, SL_SOL_SOCKET, SL_SO_CHANGE_CHANNEL, &newChannel, sizeof(newChannel));
- SL_IP_RAW_RX_NO_HEADER:
uint32_t header = 1; // remove ip header sl_SetSockOpt(SockID, SL_IPPROTO_IP, SL_IP_RAW_RX_NO_HEADER, &header, sizeof(header));
- SL_IP_HDRINCL:
uint32_t header = 1; sl_SetSockOpt(SockID, SL_IPPROTO_IP, SL_IP_HDRINCL, &header, sizeof(header));
- SL_IP_RAW_IPV6_HDRINCL:
uint32_t header = 1; sl_SetSockOpt(SockID, SL_IPPROTO_IP, SL_IP_RAW_IPV6_HDRINCL, &header, sizeof(header));
- SL_SO_PHY_RATE:
uint32_t rate = 6; // see wlan.h RateIndex_e for values sl_SetSockOpt(SockID, SL_SOL_PHY_OPT, SL_SO_PHY_RATE, &rate, sizeof(rate));
- SL_SO_PHY_TX_POWER:
uint32_t txpower = 1; // valid range is 1-15 sl_SetSockOpt(SockID, SL_SOL_PHY_OPT, SL_SO_PHY_TX_POWER, &txpower, sizeof(txpower));
- SL_SO_PHY_NUM_FRAMES_TO_TX:
uint32_t numframes = 1; sl_SetSockOpt(SockID, SL_SOL_PHY_OPT, SL_SO_PHY_NUM_FRAMES_TO_TX, &numframes, sizeof(numframes));
- SL_SO_PHY_PREAMBLE:
uint32_t preamble = 1; sl_SetSockOpt(SockID, SL_SOL_PHY_OPT, SL_SO_PHY_PREAMBLE, &preamble, sizeof(preamble));
Definition at line 773 of file cc3100_socket.cpp.
int16_t sl_Socket | ( | int16_t | Domain, |
int16_t | Type, | ||
int16_t | Protocol | ||
) | [inherited] |
create an endpoint for communication
The socket function creates a new socket of a certain socket type, identified by an integer number, and allocates system resources to it. This function is called by the application layer to obtain a socket handle.
- Parameters:
-
[in] domain specifies the protocol family of the created socket. For example: AF_INET for network protocol IPv4 AF_RF for starting transceiver mode. Notes: - sending and receiving any packet overriding 802.11 header
- for optimized power consumption the socket will be started in TX only mode until receive command is activated AF_INET6 for IPv6
[in] type specifies the communication semantic, one of: SOCK_STREAM (reliable stream-oriented service or Stream Sockets) SOCK_DGRAM (datagram service or Datagram Sockets) SOCK_RAW (raw protocols atop the network layer) when used with AF_RF: SOCK_DGRAM - L2 socket SOCK_RAW - L1 socket - bypass WLAN CCA (Clear Channel Assessment) [in] protocol specifies a particular transport to be used with the socket. The most common are IPPROTO_TCP, IPPROTO_SCTP, IPPROTO_UDP, IPPROTO_DCCP. The value 0 may be used to select a default protocol from the selected domain and type
- Returns:
- On success, socket handle that is used for consequent socket operations. A successful return code should be a positive number (int16) On error, a negative (int16) value will be returned specifying the error code. SL_EAFNOSUPPORT - illegal domain parameter SL_EPROTOTYPE - illegal type parameter SL_EACCES - permission denied SL_ENSOCK - exceeded maximal number of socket SL_ENOMEM - memory allocation error SL_EINVAL - error in socket configuration SL_EPROTONOSUPPORT - illegal protocol parameter SL_EOPNOTSUPP - illegal combination of protocol and type parameters
- See also:
- sl_Close
- Note:
- belongs to basic_api
- Warning:
Definition at line 185 of file cc3100_socket.cpp.
Generated on Tue Jul 12 2022 22:22:39 by
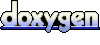