
TI's CC3100 websocket camera demo with Arducam mini ov5642 and freertos. Should work with other M3's. Work in progress test demo.
This module implements the HTTP digest access authentication routines. More...
Functions | |
static UINT32 | GetRandomUint () |
Simple random generator To improve randomness the initial seed has to be dynamic. | |
static void | Generate32BytesRandomString (UINT8 *str) |
This function will generate random 16 bytes to be used for Nonce and opaque strings. | |
void | HttpAuth_Init (struct HttpBlob username, struct HttpBlob password) |
Initialize the authentication module, so that it accepts the specified username and password This function should be called during server initialization in order to set initial user credentials This function may then be called at any time during the operation of the server in order to set different user credentials. | |
void | HttpAuth_ResponseAuthenticate (struct HttpRequest *pRequest, struct HttpBlob *pWWWAuthenticate) |
Builds and returns the WWW-Authenticate response header. | |
static UINT16 | HttpAuth_VerifyHeaderNameValue (struct HttpBlob *location, char *nameToken, UINT8 tokenlenLen, char *value, UINT8 valuelen, char **outValue) |
Find/verify name value pair in the input blob. | |
void | HttpAuth_RequestAuthenticate (struct HttpRequest *pRequest, struct HttpBlob authorization) |
Check the authentication header in a request, and either authorize the request or deny it If the authorization succeeds, then HTTP_REQUEST_FLAG_AUTHENTICATED is added to the request flags. | |
Variables | |
static struct HttpAuthState | g_authState |
The global state for digest-access authentication. | |
char | HTTP_AUTHENTICATE_RESPONSE_REALM [] = "WWW-Authenticate: Digest realm=\"" |
Header strings to be used for response headers. | |
char | HTTP_AUTHENTICATE_REALM [] = "realm" |
Authenticate header tokens. | |
UINT16 | HTTP_AUTHENTICATE_RESPONSE_HEADER_LENGTH |
The length of the response authentication header The two 32 numbers represent nonce and opaque strings and the -5 is to compensate for the sizeof() calls. |
Detailed Description
This module implements the HTTP digest access authentication routines.
Note this module is only compiled if HTTP_CORE_ENABLE_AUTH is defined in HttpConfig.h
When a "not authorized" response is sent to the client, the WWW-Authenticate header is built using HttpAuth_ResponseAuthenticate() This in turn generates new nonce and opaque values which will be used for authentication. Note that since only a single nonce is kept, only one client may ever be authenticated simultaneously. When another request with Authorization header is received, it is verified using HttpAuth_RequestAuthenticate() If all authentication tests pass, then the appropriate flag is set in the request to indicate that.
Function Documentation
static void Generate32BytesRandomString | ( | UINT8 * | str ) | [static] |
This function will generate random 16 bytes to be used for Nonce and opaque strings.
Definition at line 117 of file HttpAuth.cpp.
static UINT32 GetRandomUint | ( | ) | [static] |
Simple random generator To improve randomness the initial seed has to be dynamic.
Definition at line 90 of file HttpAuth.cpp.
void HttpAuth_Init | ( | struct HttpBlob | username, |
struct HttpBlob | password | ||
) |
Initialize the authentication module, so that it accepts the specified username and password This function should be called during server initialization in order to set initial user credentials This function may then be called at any time during the operation of the server in order to set different user credentials.
- Parameters:
-
username The authorized user's username password The authorized user's password
Definition at line 131 of file HttpAuth.cpp.
void HttpAuth_RequestAuthenticate | ( | struct HttpRequest * | pRequest, |
struct HttpBlob | authorization | ||
) |
Check the authentication header in a request, and either authorize the request or deny it If the authorization succeeds, then HTTP_REQUEST_FLAG_AUTHENTICATED is added to the request flags.
- Parameters:
-
pRequest All data about the request to authorize authorization The full string of the Authorization header
Definition at line 234 of file HttpAuth.cpp.
void HttpAuth_ResponseAuthenticate | ( | struct HttpRequest * | pRequest, |
struct HttpBlob * | pWWWAuthenticate | ||
) |
Builds and returns the WWW-Authenticate response header.
This implies generating a new nonce, etc. Notes about return value: Upon entry, pWWWAuthenticate should point to the place in the packet-send buffer where the header needs to be generated, and also specify the maximum amount of bytes available for the header at that place Upon return, pWWWAuthenticate points to the same location, but specifies the actual length of the header. If the returned length is 0, this means that there was not enough room in the buffer for the header. In such a case, the core may try again with a larger buffer
- Parameters:
-
pRequest All data about the request [in,out] pWWWAuthenticate On entry specifies the memory location to build the header at, and the maximum size. On return, specifies the same location and the actual size of the header line
Definition at line 148 of file HttpAuth.cpp.
static UINT16 HttpAuth_VerifyHeaderNameValue | ( | struct HttpBlob * | location, |
char * | nameToken, | ||
UINT8 | tokenlenLen, | ||
char * | value, | ||
UINT8 | valuelen, | ||
char ** | outValue | ||
) | [static] |
Find/verify name value pair in the input blob.
After return the location is stays in the same place since the order of the name value pairs in not constant Returns: if header not found return 0 if value token is NULL - return pointer to start of value length of value till the " delimiter if value token is not NULL - return 1 if values match
Definition at line 186 of file HttpAuth.cpp.
Variable Documentation
struct HttpAuthState g_authState [static] |
The global state for digest-access authentication.
Definition at line 44 of file HttpAuth.cpp.
char HTTP_AUTHENTICATE_REALM[] = "realm" |
Authenticate header tokens.
Definition at line 60 of file HttpAuth.cpp.
sizeof(HTTP_AUTHENTICATE_RESPONSE_REALM) + sizeof(HTTP_AUTHENTICATE_RESPONSE_NONCE) + sizeof(HTTP_AUTHENTICATE_RESPONSE_OPAQUE) + sizeof(HTTP_AUTHENTICATE_RESPONSE_EOH) + sizeof(HTTP_AUTH_REALM) + DIGEST_AUTHENTICATION_BUFFER_SIZE + DIGEST_AUTHENTICATION_BUFFER_SIZE - 5
The length of the response authentication header The two 32 numbers represent nonce and opaque strings and the -5 is to compensate for the sizeof() calls.
Definition at line 77 of file HttpAuth.cpp.
char HTTP_AUTHENTICATE_RESPONSE_REALM[] = "WWW-Authenticate: Digest realm=\"" |
Header strings to be used for response headers.
Definition at line 54 of file HttpAuth.cpp.
Generated on Wed Jul 13 2022 15:58:46 by
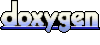