This is a device driver of AK8963, which is 3-axis magnetometer manufactured by AKM.
Dependents: SimpleSample combination combination
AK8963 Class Reference
This is a device driver of AK8963. More...
#include <ak8963.h>
Data Structures | |
struct | MagneticVector |
Structure to hold a magnetic vector. More... | |
Public Types | |
enum | SlaveAddress { SLAVE_ADDR_1 = 0x0c, SLAVE_ADDR_2 = 0x0d, SLAVE_ADDR_3 = 0x0e, SLAVE_ADDR_4 = 0x0f } |
Possible slave address of AK8963. More... | |
enum | OperationMode { MODE_POWER_DOWN = 0x00, MODE_SINGLE_MEASUREMENT = 0x01, MODE_CONTINUOUS_1 = 0x02, MODE_CONTINUOUS_2 = 0x06 } |
Available opration modes in AK8963. More... | |
enum | Status { SUCCESS, ERROR_I2C_READ, ERROR_I2C_WRITE, ERROR, DATA_READY, NOT_DATA_READY } |
Status of function. More... | |
Public Member Functions | |
AK8963 (I2C *conn, SlaveAddress addr) | |
Constructor. | |
Status | checkConnection () |
Check the connection. | |
Status | setOperationMode (OperationMode mode) |
Sets device operation mode. | |
Status | isDataReady () |
Check if data is ready, i.e. | |
Status | getMagneticVector (MagneticVector *vec) |
Gets magnetic vector from the device. |
Detailed Description
This is a device driver of AK8963.
- Note:
- AK8963 is a 3-axis magnetometer (magnetic sensor) device manufactured by AKM.
Example:
#include "mbed.h" #include "ak8963.h" #define I2C_SPEED_100KHZ 100000 #define I2C_SPEED_400KHZ 400000 int main() { // Creates an instance of I2C I2C connection(I2C_SDA0, I2C_SCL0); connection.frequency(I2C_SPEED_100KHZ); // Creates an instance of AK8963 AK8963 ak8963(&connection, AK8963::SLAVE_ADDR_1); // Checks connectivity if(ak8963.checkConnection() != AK8963::SUCCESS) { // Failed to check device connection. // - error handling here - } // Puts the device into continuous measurement mode. if(ak8963.setOperationMode(AK8963::MODE_CONTINUOUS_1) != AK8963::SUCCESS) { // Failed to set the device into continuous measurement mode. // - error handling here - } while(true) { // checks DRDY if (statusAK8963 == AK8963::DATA_READY) { AK8963::MagneticVector mag; ak8963.getMagneticVector(&mag); // You may use serial output to see data. // serial.printf("%d,%5.1f,%5.1f,%5.1f\n", // mag.isOverflow, // mag.mx, mag.my, mag.mz); statusAK8963 = AK8963::NOT_DATA_READY; } else if (statusAK8963 == AK8963::NOT_DATA_READY) { // Nothing to do. } else { // - error handling here - } } }
Definition at line 58 of file ak8963.h.
Member Enumeration Documentation
enum OperationMode |
enum SlaveAddress |
enum Status |
Constructor & Destructor Documentation
AK8963 | ( | I2C * | conn, |
SlaveAddress | addr | ||
) |
Constructor.
- Parameters:
-
conn instance of I2C addr slave address of the device
Definition at line 52 of file ak8963.cpp.
Member Function Documentation
AK8963::Status checkConnection | ( | ) |
Check the connection.
- Note:
- Connection check is performed by reading a register which has a fixed value and verify it.
- Returns:
- Returns SUCCESS when succeeded, otherwise returns another code.
Definition at line 58 of file ak8963.cpp.
AK8963::Status getMagneticVector | ( | MagneticVector * | vec ) |
Gets magnetic vector from the device.
- Parameters:
-
vec Pointer to a instance of MagneticVector
- Returns:
- Returns SUCCESS when succeeded, otherwise returns another code.
Definition at line 167 of file ak8963.cpp.
AK8963::Status isDataReady | ( | ) |
Check if data is ready, i.e.
measurement is finished.
- Returns:
- Returns DATA_READY if data is ready or NOT_DATA_READY if data is not ready. If error happens, returns another code.
Definition at line 110 of file ak8963.cpp.
AK8963::Status setOperationMode | ( | AK8963::OperationMode | mode ) |
Sets device operation mode.
- Parameters:
-
mode device opration mode
- Returns:
- Returns SUCCESS when succeeded, otherwise returns another code.
Definition at line 141 of file ak8963.cpp.
Generated on Wed Jul 13 2022 16:32:57 by
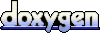