This is a device driver of AK8963, which is 3-axis magnetometer manufactured by AKM.
Dependents: SimpleSample combination combination
ak8963.h
00001 #ifndef AK8963_H 00002 #define AK8963_H 00003 00004 #include "mbed.h" 00005 00006 /** 00007 * This is a device driver of AK8963. 00008 * 00009 * @note AK8963 is a 3-axis magnetometer (magnetic sensor) device manufactured by AKM. 00010 * 00011 * Example: 00012 * @code 00013 * #include "mbed.h" 00014 * #include "ak8963.h" 00015 * 00016 * #define I2C_SPEED_100KHZ 100000 00017 * #define I2C_SPEED_400KHZ 400000 00018 * 00019 * int main() { 00020 * // Creates an instance of I2C 00021 * I2C connection(I2C_SDA0, I2C_SCL0); 00022 * connection.frequency(I2C_SPEED_100KHZ); 00023 * 00024 * // Creates an instance of AK8963 00025 * AK8963 ak8963(&connection, AK8963::SLAVE_ADDR_1); 00026 * 00027 * // Checks connectivity 00028 * if(ak8963.checkConnection() != AK8963::SUCCESS) { 00029 * // Failed to check device connection. 00030 * // - error handling here - 00031 * } 00032 * 00033 * // Puts the device into continuous measurement mode. 00034 * if(ak8963.setOperationMode(AK8963::MODE_CONTINUOUS_1) != AK8963::SUCCESS) { 00035 * // Failed to set the device into continuous measurement mode. 00036 * // - error handling here - 00037 * } 00038 * 00039 * while(true) { 00040 * // checks DRDY 00041 * if (statusAK8963 == AK8963::DATA_READY) { 00042 * AK8963::MagneticVector mag; 00043 * ak8963.getMagneticVector(&mag); 00044 * // You may use serial output to see data. 00045 * // serial.printf("%d,%5.1f,%5.1f,%5.1f\n", 00046 * // mag.isOverflow, 00047 * // mag.mx, mag.my, mag.mz); 00048 * statusAK8963 = AK8963::NOT_DATA_READY; 00049 * } else if (statusAK8963 == AK8963::NOT_DATA_READY) { 00050 * // Nothing to do. 00051 * } else { 00052 * // - error handling here - 00053 * } 00054 * } 00055 * } 00056 * @endcode 00057 */ 00058 class AK8963 00059 { 00060 public: 00061 /** 00062 * Possible slave address of AK8963. Selected by CAD1 and CAD0 pins. 00063 */ 00064 typedef enum { 00065 SLAVE_ADDR_1 = 0x0c, /**< CAD0 = Low, CAD1 = Low */ 00066 SLAVE_ADDR_2 = 0x0d, /**< CAD0 = High, CAD1 = Low */ 00067 SLAVE_ADDR_3 = 0x0e, /**< CAD0 = Low, CAD1 = High */ 00068 SLAVE_ADDR_4 = 0x0f, /**< CAD0 = High, CAD1 = High */ 00069 } SlaveAddress; 00070 00071 /** 00072 * Available opration modes in AK8963. 00073 */ 00074 typedef enum { 00075 MODE_POWER_DOWN = 0x00, /**< Power-down mode */ 00076 MODE_SINGLE_MEASUREMENT = 0x01, /**< Single measurement mode */ 00077 MODE_CONTINUOUS_1 = 0x02, /**< Continuous measurement mode 1, 8 Hz */ 00078 MODE_CONTINUOUS_2 = 0x06, /**< Continuous measurement mode 2, 100 Hz */ 00079 } OperationMode; 00080 00081 /** 00082 * Status of function. 00083 */ 00084 typedef enum { 00085 SUCCESS, /**< The function processed successfully. */ 00086 ERROR_I2C_READ, /**< Error related to I2C read. */ 00087 ERROR_I2C_WRITE, /**< Error related to I2C write. */ 00088 ERROR, /**< General Error */ 00089 DATA_READY, /**< Data ready */ 00090 NOT_DATA_READY, /**< Data ready is not asserted. */ 00091 } Status; 00092 00093 /** 00094 * Structure to hold a magnetic vector. 00095 */ 00096 typedef struct { 00097 float mx; /**< x component */ 00098 float my; /**< y component */ 00099 float mz; /**< z component */ 00100 bool isOverflow; /**< Indicating magnetic sensor overflow */ 00101 } MagneticVector; 00102 00103 /** 00104 * Constructor. 00105 * 00106 * @param conn instance of I2C 00107 * @param addr slave address of the device 00108 */ 00109 AK8963(I2C *conn, SlaveAddress addr); 00110 00111 /** 00112 * Check the connection. 00113 * 00114 * @note Connection check is performed by reading a register which has a fixed value and verify it. 00115 * 00116 * @return Returns SUCCESS when succeeded, otherwise returns another code. 00117 */ 00118 Status checkConnection(); 00119 00120 /** 00121 * Sets device operation mode. 00122 * 00123 * @param mode device opration mode 00124 * 00125 * @return Returns SUCCESS when succeeded, otherwise returns another code. 00126 */ 00127 Status setOperationMode(OperationMode mode); 00128 00129 /** 00130 * Check if data is ready, i.e. measurement is finished. 00131 * 00132 * @return Returns DATA_READY if data is ready or NOT_DATA_READY if data is not ready. If error happens, returns another code. 00133 */ 00134 Status isDataReady(); 00135 00136 /** 00137 * Gets magnetic vector from the device. 00138 * 00139 * @param vec Pointer to a instance of MagneticVector 00140 * 00141 * @return Returns SUCCESS when succeeded, otherwise returns another code. 00142 */ 00143 Status getMagneticVector(MagneticVector *vec); 00144 00145 private: 00146 /** 00147 * Sensitivity adjustment data. 00148 */ 00149 typedef struct { 00150 unsigned char x; /**< sensitivity adjustment value for x */ 00151 unsigned char y; /**< sensitivity adjustment value for y */ 00152 unsigned char z; /**< sensitivity adjustment value for z */ 00153 } SensitivityAdjustment; 00154 00155 /** 00156 * Holds sensitivity adjustment values 00157 */ 00158 SensitivityAdjustment sensitivityAdjustment; 00159 00160 /** 00161 * Holds a pointer to an I2C object. 00162 */ 00163 I2C *connection; 00164 00165 /** 00166 * Holds the slave address of AK8963. 00167 */ 00168 SlaveAddress slaveAddress; 00169 00170 /** 00171 * Writes data to the device. 00172 * @param registerAddress register address to be written 00173 * @param data data to be written 00174 * @param length of the data 00175 * @return Returns SUCCESS when succeeded, otherwise returns another. 00176 */ 00177 Status write(char registerAddress, const char *data, int length); 00178 00179 /** 00180 * Reads data from device. 00181 * @param registerAddress register address to be read 00182 * @param buf buffer to store the read data 00183 * @param length bytes to be read 00184 * @return Returns SUCCESS when succeeded, otherwise returns another. 00185 */ 00186 Status read(char registerAddress, char *buf, int length); 00187 00188 /** 00189 * Gets magnetic data, from the register ST1 (0x02) to ST2 (0x09), from the device. 00190 * 00191 * @param buf buffer to store the data read from the device. 00192 * 00193 * @return Returns SUCCESS when succeeded, otherwise returns another. 00194 */ 00195 Status getData(char *buf); 00196 00197 /** 00198 * Gets sensitivity adjustment values from the register ASAX, ASAY, and ASAZ. 00199 * 00200 * @param buf buffer to store sensitivity adjustment data 00201 * 00202 * @return SUCCESS when succeeded, otherwise returns another. 00203 */ 00204 Status getSensitivityAdjustment(SensitivityAdjustment *sns); 00205 00206 }; 00207 00208 #endif
Generated on Wed Jul 13 2022 16:32:57 by
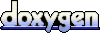