Library for EarthLCD ezLCD3xx line of products
ezLCD3 Class Reference
Interface to the EarthLCD ezLCD3xx line of smart displays Derived class from Serial so that you can conveniently printf(), putc(), etc to the display. More...
#include <ezLCDLib.h>
Public Types | |
enum | Commands { Command = 0 , Clr_Screen = 2, Ping = 3, zBeep = 4, Light = 5 , Font = 10, Fontw = 11, Font_Orient = 12, Line_Width = 13, Line_Type = 14, XY = 15, StringID = 16, Plot = 17, Line = 18, Box = 19, Circle = 20, Arc = 21, Pie = 22, Picture = 24, Print = 25, Beep_Freq = 26, Calibrate = 28, zReset = 29, Rec_Macro = 30, Play_Macro = 31, Stop_Macro = 32, Pause_Macro = 33, Loop_Macro = 34, Speed_Macro = 35 , Security = 40, Location = 41 , ClipEnable = 46, ClipArea = 47 , Format = 60 , Set_Button = 70, Set_CheckBox = 71, Set_Gbox = 72, Set_RadioButton = 73, Set_DMeter = 74, DMeter_Value = 75, Set_AMeter = 76, AMeter_Value = 77, AMeter_Color = 78, Set_TouchZone = 79, Set_Dial = 80, Set_Slider = 82, Set_Progress = 85, Progress_Value = 86, Set_StaticText = 87, StaticText_Value = 88, Choice = 89, Widget_Theme = 90, Widget_Values = 91, Widget_State = 92 , Xmax = 100, Ymax = 101, Wait = 102, Waitn = 103, Waitt = 104, Threshold = 105, Verbose = 106, Lecho = 107, Xtouch = 110, Ytouch = 111, Stouch = 112 } |
Numerical values for the EarthSEMPL commands. More... | |
Public Member Functions | |
ezLCD3 (PinName tx, PinName rx) | |
Create a new interface to a ezLCD3xx display. | |
void | sendInt (int i) |
Send a integer to the display. | |
int | getInt (char *str) |
Get a integer from the display. | |
void | getString (char *str) |
Get a string from the display. | |
bool | waitForCR (void) |
Waits for a CR from display. | |
void | cls (void) |
clear the screen to black | |
void | cls (int bColor) |
clear the screen with background color | |
void | cls (int bColor, int fColor) |
clear the screen with background color and drawing color | |
void | color (int color) |
Set drawing color. | |
void | xy (int x, int y) |
set x y position for drawing and text | |
void | plot (void) |
set pixel at current x y | |
void | plot (int x, int y) |
set pixel in current draw color at x y | |
void | line (int x, int y) |
draw line from current x y to new x y | |
void | lineType (int type) |
Set line drawing type. | |
void | lineWidth (int width) |
Set line drawing width. | |
void | circle (int radius, bool filled) |
Draw circle in current color at current x y. | |
void | box (int width, int heigth, bool filled) |
draw a box from current x y of width and heigth | |
void | light (int level) |
set backlight brightness | |
void | sendCommand (char *str) |
Send a command direct to display. | |
int | getXmax (void) |
Return Xmax of display. | |
int | getYmax (void) |
Return Ymax of display. | |
void | sendString (char *str) |
Send a string of data to display. | |
void | setStringID (int ID, char *str) |
Set stringID to display. | |
void | getStringID (int ID, char *str) |
Get stringID from Display. | |
void | print (char *str) |
print string at current x y | |
void | fontw (int id, char *str) |
Set widget font Fonts are located in the flash drive of the display font 0 and font 1 are builtin fonts and are much faster to draw in the /EZUSERS/FONTS and /EZSYS/FONTS directory use the ezLCD3xx font convert utilty to convert true type fonts. | |
void | theme (int ID, int EmbossDkColor, int EmbossLtColor, int TextColor0, int TextColor1, int TextColorDisabled, int Color0, int Color1, int ColorDisabled, int CommonBkColor, int Fontw) |
Sets color themes for widgets. | |
void | ameter (int ID, int x, int y, int w, int h, int options, int value, int min, int max, int theme, int stringID, int type) |
Analog Meter Widget. | |
void | touchZone (int ID, int x, int y, int w, int h, bool option) |
Touchzone Widget. | |
void | button (int ID, int x, int y, int w, int h, int option, int align, int radius, int theme, int stringID) |
Button Widget. | |
void | image (char *str) |
Display Bitmap Display Bitmap at current x y supports GIF BMP JPG images are located in the /EZUSERS/FONTS and /EZSYS/FONTS directory. | |
void | image (int x, int y, char *str) |
Display Bitmap Display Bitmap at specfied x y supports GIF BMP JPG images are located in the /EZUSERS/FONTS and /EZSYS/FONTS directory. | |
void | staticText (int ID, int x, int y, int w, int h, int option, int theme, int stringID) |
StaticBox Widget. | |
void | progressBar (int ID, int x, int y, int w, int h, int option, int value, int max, int theme, int stringID) |
ProgressBar Widget. | |
void | slider (int ID, int x, int y, int w, int h, int option, int range, int res, int value, int theme) |
Slider Widget. | |
void | wstate (int ID, int option) |
wstate | |
void | wvalue (int ID, int value) |
Wvalue set widget values. | |
unsigned int | wstack (int type) |
Wstack is a 32 level stack of widget events. | |
int | putc (int c) |
Send a character directly to the serial interface. | |
int | getc () |
Receive a character directly to the serial interface. |
Detailed Description
Interface to the EarthLCD ezLCD3xx line of smart displays Derived class from Serial so that you can conveniently printf(), putc(), etc to the display.
Example:
#include "mbed.h" #include "TSISensor.h" #include "ezLCDLib.h" #include "MMA8451Q.h" #define MMA8451_I2C_ADDRESS (0x1d<<1) enum { BLACK, GRAY, SILVER, WHITE, RED, MAROON, YELLOW, OLIVE, LIME, GREEN, AQUA, TEAL, BLUE, NAVY, FUCHSIA, PURPLE }; #define arLCD //InterruptIn ezLCD3Int( PTD7); // interrupt instance for touch //InterruptIn ezLCD3Int( PTA13); // interrupt instance for touch #ifdef arLCD InterruptIn ezLCD3Int( PTD4 ); // interrupt instance for touch DigitalOut _nrst(PTC7); ezLCD3 lcd(PTA2, PTA1); //rx,tx TSISensor tsi; AnalogIn adc1(PTB0); MMA8451Q acc(PTE25, PTE24, MMA8451_I2C_ADDRESS); #endif DigitalOut myled(LED_BLUE); volatile bool ezLCD3Touch = false; int color = BLACK; int temp; int touch=0; int adc1Count = 0; float adc1Average = 0; char ezID[20]; char ezVER[20]; char adcBuf[20]; void ezLCD3Isr( void ) { ezLCD3Touch = true; } int main() { wait(3); while(!lcd.sync()); ezLCD3Int.fall(&ezLCD3Isr); ezLCD3Touch = false; myled=1; lcd.cls(BLACK); lcd.theme(0, 111, 106, 0, 130, 0, 13, 12, 101, 100, 0); lcd.xy(0,0);//11 lcd.color(BLACK); //1 lcd.box(319,239, 1 );//2 lcd.color(155); lcd.xy(1,1); lcd.line(317,1); lcd.xy(1,1); lcd.line(1,237); lcd.color(WHITE); lcd.xy(2,237); lcd.line(317,237); lcd.line(317,2); lcd.color(WHITE);//3 lcd.xy(300,100);//4 lcd.xy(300,200);//6 lcd.light(50);//8 lcd.xy(40,10);//9 lcd.print("--==[\\[28m Hello \\[65mMBED\\[28m World \\[3m]==--");//10 lcd.xy(20,100);//11 lcd.color(YELLOW);//12 lcd.setStringID( 2,"Button1"); lcd.setStringID( 3,"Button2"); lcd.button(2,20,40,100,40,1,0,10,0,2); lcd.button(3,200,40,100,40,1,0,10,0,3); lcd.getStringID( 65,ezID); lcd.getStringID( 66,ezVER); lcd.print(ezID); lcd.color(PURPLE); lcd.xy(20,120);//11 lcd.print(ezVER); lcd.color(TEAL); lcd.xy(20,140); lcd.printf("MaxX %d MaxY %d", lcd.getXmax()+1, lcd.getYmax()+1); //13 14 lcd.light(5);//15 lcd.light(100);//16 lcd.image(20,165,"0.gif"); lcd.image(55,165,"1.gif"); lcd.image(90,165,"2.gif"); lcd.image(125,165,"3.gif"); lcd.color(WHITE);//17 lcd.setStringID(4, "meter"); lcd.setStringID(5, "%"); lcd.setStringID(6, "X"); lcd.setStringID(7, "Y"); lcd.setStringID(8, "Z"); lcd.staticText( 4, 200, 210, 100, 25, 8, 0, 4); lcd.progressBar( 5, 200, 180, 100, 25, 1, 0, 100, 0 , 5); lcd.progressBar( 6, 200, 90, 100, 25, 1, 0, 100, 0 , 6); lcd.progressBar( 7, 200, 120, 100, 25, 1, 0, 100, 0 , 7); lcd.progressBar( 8, 200, 150, 100, 25, 1, 0, 100, 0 , 8); while(1) { if(ezLCD3Touch) { ezLCD3Touch = false; touch=lcd.wstack(LIFO); myled = !myled; lcd.xy(10,210); lcd.color(BLACK); lcd.box(180,20,1); lcd.color(WHITE); switch( touch ) { case 240: lcd.print("Button1 Pressed"); break; case 214: lcd.print("Button1 Released"); break; case 224: lcd.print("Button1 Cancel"); break; case 340: lcd.print("Button2 Pressed"); break; case 314: lcd.print("Button2 Released"); break; case 324: lcd.print("Button2 Cancel"); break; default: lcd.print("default"); break; } } adc1Average += adc1.read(); adc1Count ++; if (adc1Count == 500) { adc1Count=0; sprintf(adcBuf, "%1.3f V",(adc1Average/500)*3.3); lcd.setStringID(4, adcBuf); lcd.wstate(4,REDRAW); adc1Average =0; } if(adc1Count ==250) { lcd.wvalue(5,abs( tsi.readPercentage()*100)); lcd.wvalue(6,abs(acc.getAccX())*100); lcd.wvalue(7,abs(acc.getAccY())*100); lcd.wvalue(8,abs(acc.getAccZ())*100); } } }
Definition at line 185 of file ezLCDLib.h.
Member Enumeration Documentation
enum Commands |
Numerical values for the EarthSEMPL commands.
- Enumerator:
Definition at line 529 of file ezLCDLib.h.
Constructor & Destructor Documentation
ezLCD3 | ( | PinName | tx, |
PinName | rx | ||
) |
Create a new interface to a ezLCD3xx display.
- Parameters:
-
tx -- mbed transmit pin rx -- mbed receive pin
Definition at line 33 of file ezLCDLib.cpp.
Member Function Documentation
void ameter | ( | int | ID, |
int | x, | ||
int | y, | ||
int | w, | ||
int | h, | ||
int | options, | ||
int | value, | ||
int | min, | ||
int | max, | ||
int | theme, | ||
int | stringID, | ||
int | type | ||
) |
Analog Meter Widget.
Definition at line 452 of file ezLCDLib.cpp.
void box | ( | int | width, |
int | heigth, | ||
bool | filled | ||
) |
draw a box from current x y of width and heigth
- Parameters:
-
[in] width [in] heigth [in] filled true for a filled box outline if false
Definition at line 252 of file ezLCDLib.cpp.
void button | ( | int | ID, |
int | x, | ||
int | y, | ||
int | w, | ||
int | h, | ||
int | option, | ||
int | align, | ||
int | radius, | ||
int | theme, | ||
int | stringID | ||
) |
Button Widget.
Definition at line 505 of file ezLCDLib.cpp.
void circle | ( | int | radius, |
bool | filled | ||
) |
Draw circle in current color at current x y.
- Parameters:
-
[in] radius diameter of circle [in] filled true for a filled box outline if false
Definition at line 239 of file ezLCDLib.cpp.
void cls | ( | int | bColor ) |
clear the screen with background color
- Parameters:
-
[in] bcolor Background color
Definition at line 157 of file ezLCDLib.cpp.
void cls | ( | void | ) |
clear the screen to black
Definition at line 150 of file ezLCDLib.cpp.
void cls | ( | int | bColor, |
int | fColor | ||
) |
clear the screen with background color and drawing color
- Parameters:
-
[in] bColor background color [in] fColor drawing color
Definition at line 166 of file ezLCDLib.cpp.
void color | ( | int | color ) |
Set drawing color.
- Parameters:
-
[in] color color to draw with
Definition at line 176 of file ezLCDLib.cpp.
void fontw | ( | int | id, |
char * | str | ||
) |
Set widget font Fonts are located in the flash drive of the display
font 0 and font 1 are builtin fonts and are much faster to draw
in the /EZUSERS/FONTS and /EZSYS/FONTS directory
use the ezLCD3xx font convert utilty to convert true type fonts.
- Parameters:
-
str font name
Definition at line 330 of file ezLCDLib.cpp.
int getc | ( | void | ) |
Receive a character directly to the serial interface.
- Returns:
- c The character received from the
Definition at line 693 of file ezLCDLib.cpp.
int getInt | ( | char * | str ) |
Get a integer from the display.
- Parameters:
-
[out] str string to put the data in
Definition at line 112 of file ezLCDLib.cpp.
void getString | ( | char * | str ) |
Get a string from the display.
- Parameters:
-
[out] str string to put the data in
Definition at line 118 of file ezLCDLib.cpp.
void getStringID | ( | int | ID, |
char * | str | ||
) |
Get stringID from Display.
- Parameters:
-
ID string ID to read str string to put data in
Example:
lcd.getstringID(66, ezVer); // get ezLCD Firmware version lcd.print(ezVer); // print version
Definition at line 311 of file ezLCDLib.cpp.
int getXmax | ( | void | ) |
int getYmax | ( | void | ) |
void image | ( | char * | str ) |
Display Bitmap Display Bitmap at current x y
supports GIF BMP JPG
images are located in the /EZUSERS/FONTS and /EZSYS/FONTS directory.
- Parameters:
-
str filename
Definition at line 531 of file ezLCDLib.cpp.
void image | ( | int | x, |
int | y, | ||
char * | str | ||
) |
Display Bitmap Display Bitmap at specfied x y
supports GIF BMP JPG
images are located in the /EZUSERS/FONTS and /EZSYS/FONTS directory.
- Parameters:
-
x x location to start bitmap y y location to start bitmap str filename
Definition at line 596 of file ezLCDLib.cpp.
void light | ( | int | level ) |
set backlight brightness
- Parameters:
-
[in] level is brightness 0=off 100=full in steps of 32
Definition at line 267 of file ezLCDLib.cpp.
void line | ( | int | x, |
int | y | ||
) |
draw line from current x y to new x y
- Parameters:
-
[in] x is the x coordinate [in] y is the y coordinate
Definition at line 213 of file ezLCDLib.cpp.
void lineType | ( | int | type ) |
Set line drawing type.
- Parameters:
-
[in] type Options: 0 = solid, 1= dotted (1 pixel spacing between dots), 2 = dashed (2 pixel spacing between dashes)
Definition at line 223 of file ezLCDLib.cpp.
void lineWidth | ( | int | width ) |
void plot | ( | void | ) |
set pixel at current x y
Definition at line 196 of file ezLCDLib.cpp.
void plot | ( | int | x, |
int | y | ||
) |
set pixel in current draw color at x y
- Parameters:
-
[in] x is the x coordinate [in] y is the y coordinate
Definition at line 203 of file ezLCDLib.cpp.
void print | ( | char * | str ) |
print string at current x y
- Parameters:
-
str string prints directly to display
Definition at line 320 of file ezLCDLib.cpp.
void progressBar | ( | int | ID, |
int | x, | ||
int | y, | ||
int | w, | ||
int | h, | ||
int | option, | ||
int | value, | ||
int | max, | ||
int | theme, | ||
int | stringID | ||
) |
ProgressBar Widget.
- Parameters:
-
ID x y w h option value max theme stringID
Definition at line 542 of file ezLCDLib.cpp.
int putc | ( | int | c ) |
Send a character directly to the serial interface.
- Parameters:
-
c The character to send to the
Definition at line 688 of file ezLCDLib.cpp.
void sendCommand | ( | char * | str ) |
Send a command direct to display.
- Parameters:
-
str command string
Example:
lcd.sendCommand( "cls" ); // clear display
Definition at line 663 of file ezLCDLib.cpp.
void sendInt | ( | int | i ) |
Send a integer to the display.
- Parameters:
-
[in] i integer to send
Definition at line 89 of file ezLCDLib.cpp.
void sendString | ( | char * | str ) |
Send a string of data to display.
Definition at line 291 of file ezLCDLib.cpp.
void setStringID | ( | int | ID, |
char * | str | ||
) |
Set stringID to display.
- Parameters:
-
ID string ID to write str string to write
Definition at line 298 of file ezLCDLib.cpp.
void slider | ( | int | ID, |
int | x, | ||
int | y, | ||
int | w, | ||
int | h, | ||
int | option, | ||
int | range, | ||
int | res, | ||
int | value, | ||
int | theme | ||
) |
Slider Widget.
- Parameters:
-
ID x y w h option range res value theme
Definition at line 569 of file ezLCDLib.cpp.
void staticText | ( | int | ID, |
int | x, | ||
int | y, | ||
int | w, | ||
int | h, | ||
int | option, | ||
int | theme, | ||
int | stringID | ||
) |
StaticBox Widget.
Definition at line 610 of file ezLCDLib.cpp.
void theme | ( | int | ID, |
int | EmbossDkColor, | ||
int | EmbossLtColor, | ||
int | TextColor0, | ||
int | TextColor1, | ||
int | TextColorDisabled, | ||
int | Color0, | ||
int | Color1, | ||
int | ColorDisabled, | ||
int | CommonBkColor, | ||
int | Fontw | ||
) |
Sets color themes for widgets.
Definition at line 401 of file ezLCDLib.cpp.
void touchZone | ( | int | ID, |
int | x, | ||
int | y, | ||
int | w, | ||
int | h, | ||
bool | option | ||
) |
Touchzone Widget.
Definition at line 483 of file ezLCDLib.cpp.
bool waitForCR | ( | void | ) |
Waits for a CR from display.
Definition at line 134 of file ezLCDLib.cpp.
unsigned int wstack | ( | int | type ) |
Wstack is a 32 level stack of widget events.
- Parameters:
-
type LIFO - Get the last widget event off the stack type FIFO - Get the first widget event off the stack type CLEAR - Clear stack
- Returns:
- widget id and even touch, release
Definition at line 633 of file ezLCDLib.cpp.
void wstate | ( | int | ID, |
int | option | ||
) |
wstate
Definition at line 652 of file ezLCDLib.cpp.
void wvalue | ( | int | ID, |
int | value | ||
) |
Wvalue set widget values.
- Parameters:
-
ID widget ID to update value widget value to update
Definition at line 642 of file ezLCDLib.cpp.
void xy | ( | int | x, |
int | y | ||
) |
set x y position for drawing and text
- Parameters:
-
[in] x is the x coordinate [in] y is the y coordinate
Definition at line 185 of file ezLCDLib.cpp.
Generated on Wed Jul 13 2022 05:25:49 by
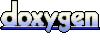