Library for EarthLCD ezLCD3xx line of products
Embed:
(wiki syntax)
Show/hide line numbers
ezLCDLib.cpp
00001 /** 00002 * 00003 * 00004 * 00005 * 00006 * 00007 * 00008 */ 00009 00010 00011 #include "mbed.h" 00012 #include "ezLCDLib.h" 00013 00014 void Tx_interrupt(); 00015 void Rx_interrupt(); 00016 void send_line(); 00017 void read_line(); 00018 00019 // Circular buffers for serial TX and RX data - used by interrupt routines 00020 const int buffer_size = 64; 00021 // might need to increase buffer size for high baud rates 00022 char tx_buffer[buffer_size]; 00023 char rx_buffer[buffer_size]; 00024 // Circular buffer pointers 00025 // volatile makes read-modify-write atomic 00026 volatile int tx_in=0; 00027 volatile int tx_out=0; 00028 volatile int rx_in=0; 00029 volatile int rx_out=0; 00030 00031 char buf[64]; 00032 00033 ezLCD3::ezLCD3(PinName tx, PinName rx) : Stream("ezLCD3") , _ser(tx, rx) 00034 { 00035 _ser.baud(115200); // default baud rate 00036 _ser.attach(this,&ezLCD3::Rx_interrupt, Serial::RxIrq); 00037 } 00038 00039 void ezLCD3::Rx_interrupt( void ) 00040 { 00041 // Loop just in case more than one character is in UART's receive FIFO buffer 00042 // Stop if buffer full 00043 while ((_ser.readable()) || (((rx_in + 1) % buffer_size) == rx_out)) { 00044 rx_buffer[rx_in] = _ser.getc(); 00045 rx_in = (rx_in + 1) % buffer_size; 00046 } 00047 return; 00048 } 00049 00050 /* itoa: convert n to characters in s */ 00051 void ezLCD3::itoa(int value, char *sp, int radix) 00052 { 00053 char tmp[16];// be careful with the length of the buffer 00054 char *tp = tmp; 00055 int i; 00056 unsigned v; 00057 int sign; 00058 00059 sign = (radix == 10 && value < 0); 00060 if (sign) v = -value; 00061 else v = (unsigned)value; 00062 00063 while (v || tp == tmp) { 00064 i = v % radix; 00065 v /= radix; // v/=radix uses less CPU clocks than v=v/radix does 00066 if (i < 10) 00067 *tp++ = i+'0'; 00068 else 00069 *tp++ = i + 'a' - 10; 00070 } 00071 00072 if (sign) 00073 *sp++ = '-'; 00074 while (tp > tmp) 00075 *sp++ = *--tp; 00076 } 00077 00078 bool ezLCD3::sync( void ) 00079 { 00080 rx_in=0; 00081 rx_out=0; 00082 _ser.putc('\r'); 00083 if(waitForCR()) 00084 return true; 00085 else 00086 return false; 00087 00088 } 00089 void ezLCD3::sendInt( int i ) 00090 { 00091 char value[5]= {0, 0, 0, 0, 0}; 00092 itoa(i, value, 10); 00093 if(value[0]) _ser.putc(value[0]); 00094 if(value[1]) _ser.putc(value[1]); 00095 if(value[2]) _ser.putc(value[2]); 00096 if(value[3]) _ser.putc(value[3]); 00097 if(value[4]) _ser.putc(value[4]); 00098 } 00099 00100 void ezLCD3::stripSpace(char* str) 00101 { 00102 char* i = str; 00103 char* j = str; 00104 while(*j != 0) { 00105 *i = *j++; 00106 if(*i != ' ') 00107 i++; 00108 } 00109 *i = 0; 00110 } 00111 00112 int ezLCD3::getInt( char *str ) 00113 { 00114 stripSpace(str); 00115 return atoi(str ); 00116 } 00117 00118 void ezLCD3::getString( char *str ) 00119 { 00120 char c=0; 00121 unsigned long timeOut=0; 00122 do { 00123 if(rx_in != rx_out) { 00124 c=rx_buffer[rx_out]; 00125 rx_out = (rx_out + 1) % buffer_size; 00126 *str++ = c; 00127 } 00128 timeOut++; 00129 } while(c!='\r' && timeOut != 0xfffffff); 00130 *str--; 00131 *str = 0; 00132 } 00133 00134 bool ezLCD3::waitForCR( void ) 00135 { 00136 char c=0; 00137 unsigned long timeOut=0; 00138 while(rx_in == rx_out) { 00139 if(timeOut++ > 0xffffff) return false; 00140 } 00141 c=rx_buffer[rx_out]; 00142 rx_out = (rx_out + 1) % buffer_size; 00143 if(c == '\r') { 00144 return true; 00145 } else { 00146 return false; 00147 } 00148 } 00149 00150 void ezLCD3::cls() 00151 { 00152 sendInt(Clr_Screen); 00153 _ser.putc('\r'); 00154 waitForCR(); 00155 } 00156 00157 void ezLCD3::cls(int bColor) 00158 { 00159 sendInt(Clr_Screen); 00160 _ser.putc(' '); 00161 sendInt(bColor); 00162 _ser.putc('\r'); 00163 waitForCR(); 00164 } 00165 00166 void ezLCD3::cls(int bColor, int fColor) 00167 { 00168 sendInt(Clr_Screen); 00169 _ser.putc(' '); 00170 sendInt(bColor); 00171 _ser.putc(' '); 00172 sendInt(fColor); 00173 _ser.putc('\r'); 00174 waitForCR(); 00175 } 00176 void ezLCD3::color(int fColor) 00177 { 00178 sendInt(Color); 00179 _ser.putc(' '); 00180 sendInt(fColor); 00181 _ser.putc('\r'); 00182 waitForCR(); 00183 } 00184 00185 void ezLCD3::xy(int x, int y) 00186 { 00187 sendInt(XY); 00188 _ser.putc(' '); 00189 sendInt(x); 00190 _ser.putc(' '); 00191 sendInt(y); 00192 _ser.putc('\r'); 00193 waitForCR(); 00194 } 00195 00196 void ezLCD3::plot( void ) 00197 { 00198 sendInt(Plot); 00199 _ser.putc('\r'); 00200 waitForCR(); 00201 } 00202 00203 void ezLCD3::plot( int x, int y ) 00204 { 00205 sendInt(Plot); 00206 _ser.putc(' '); 00207 sendInt(x); 00208 _ser.putc(' '); 00209 sendInt(y); 00210 _ser.putc('\r'); 00211 waitForCR(); 00212 } 00213 void ezLCD3::line(int x, int y ) 00214 { 00215 sendInt(Line); 00216 _ser.putc(' '); 00217 sendInt(x); 00218 _ser.putc(' '); 00219 sendInt(y); 00220 _ser.putc('\r'); 00221 waitForCR(); 00222 } 00223 void ezLCD3::lineType( int type ) 00224 { 00225 sendInt(Line_Type); 00226 _ser.putc(' '); 00227 sendInt(type); 00228 _ser.putc('\r'); 00229 waitForCR(); 00230 } 00231 void ezLCD3::lineWidth( int width ) 00232 { 00233 sendInt(Line_Width); 00234 _ser.putc(' '); 00235 sendInt(width); 00236 _ser.putc('\r'); 00237 waitForCR(); 00238 } 00239 void ezLCD3::circle(int radius, bool filled) 00240 { 00241 sendInt(Circle); 00242 _ser.putc(' '); 00243 sendInt(radius); 00244 if(filled) { 00245 _ser.putc(' '); 00246 _ser.putc('f'); 00247 } 00248 _ser.putc('\r'); 00249 waitForCR(); 00250 } 00251 00252 void ezLCD3::box(int width, int heigth, bool filled) 00253 { 00254 sendInt(Box); 00255 _ser.putc(' '); 00256 sendInt(width); 00257 _ser.putc(' '); 00258 sendInt(heigth); 00259 if(filled) { 00260 _ser.putc(' '); 00261 _ser.putc('f'); 00262 } 00263 _ser.putc('\r'); 00264 waitForCR(); 00265 } 00266 00267 void ezLCD3::light(int level) 00268 { 00269 sendInt( Light ); 00270 putc(' '); 00271 sendInt( level ); 00272 putc('\r'); 00273 waitForCR(); 00274 } 00275 00276 int ezLCD3::getXmax( void ) 00277 { 00278 sendInt(Xmax); 00279 _ser.putc('\r'); 00280 getString( buf ); 00281 return getInt( buf ); 00282 } 00283 int ezLCD3::getYmax( void ) 00284 { 00285 sendInt(Ymax); 00286 _ser.putc('\r'); 00287 getString( buf ); 00288 return getInt( buf ); 00289 } 00290 00291 void ezLCD3::sendString( char *str ) 00292 { 00293 unsigned char c; 00294 while( (c = *str++) ) 00295 _ser.putc(c); 00296 } 00297 00298 void ezLCD3::setStringID( int ID, char *str ) 00299 { 00300 sendInt(StringID); 00301 _ser.putc(' '); 00302 sendInt(ID); 00303 _ser.putc(' '); 00304 _ser.putc('\"'); 00305 sendString(str); 00306 _ser.putc('\"'); 00307 _ser.putc('\r'); 00308 waitForCR(); 00309 } 00310 00311 void ezLCD3::getStringID( int ID , char *str) 00312 { 00313 sendInt(StringID); 00314 _ser.putc(' '); 00315 sendInt(ID); 00316 _ser.putc('\r'); 00317 getString(str); 00318 } 00319 00320 void ezLCD3::print( char *str ) 00321 { 00322 sendInt(Print); 00323 _ser.putc(' '); 00324 _ser.putc('\"'); 00325 sendString(str); 00326 _ser.putc('\"'); 00327 _ser.putc('\r'); 00328 waitForCR(); 00329 } 00330 void ezLCD3::fontw( int ID, char *str) 00331 { 00332 sendInt(Fontw); 00333 _ser.putc(' '); 00334 sendInt(ID); 00335 _ser.putc(' '); 00336 _ser.putc('\"'); 00337 sendString(str); 00338 _ser.putc('\"'); 00339 _ser.putc('\r'); 00340 waitForCR(); 00341 } 00342 00343 void ezLCD3::fontw( int ID, int font) 00344 { 00345 sendInt(Fontw); 00346 _ser.putc(' '); 00347 sendInt(ID); 00348 _ser.putc(' '); 00349 sendInt(font); 00350 _ser.putc('\r'); 00351 waitForCR(); 00352 } 00353 00354 void ezLCD3::font( char *str) 00355 { 00356 sendInt(Font); 00357 _ser.putc(' '); 00358 sendString(str); 00359 _ser.putc('\r'); 00360 waitForCR(); 00361 } 00362 00363 void ezLCD3::fontO( bool dir ) 00364 { 00365 sendInt(Font_Orient); 00366 _ser.putc(' '); 00367 if(dir) 00368 _ser.putc('1'); 00369 else 00370 _ser.putc('0'); 00371 _ser.putc('\r'); 00372 waitForCR(); 00373 } 00374 00375 void ezLCD3::clipArea( int l, int t, int r, int b) 00376 { 00377 sendInt(ClipArea); 00378 _ser.putc(' '); 00379 sendInt(l); 00380 _ser.putc(' '); 00381 sendInt(t); 00382 _ser.putc(' '); 00383 sendInt(r); 00384 _ser.putc(' '); 00385 sendInt(b); 00386 _ser.putc(' '); 00387 _ser.putc('\r'); 00388 waitForCR(); 00389 } 00390 void ezLCD3::clipEnable( bool enable) 00391 { 00392 sendInt(ClipEnable); 00393 _ser.putc(' '); 00394 if(enable) 00395 _ser.putc('1'); 00396 else 00397 _ser.putc('0'); 00398 _ser.putc('\r'); 00399 00400 } 00401 void ezLCD3::theme(int ID, int EmbossDkColor, int EmbossLtColor, int TextColor0, int TextColor1, int TextColorDisabled, int Color0, int Color1, int ColorDisabled, int CommonBkColor, int Fontw) 00402 { 00403 sendInt(Widget_Theme); 00404 _ser.putc(' '); 00405 sendInt(ID); 00406 _ser.putc(' '); 00407 sendInt(EmbossDkColor); 00408 _ser.putc(' '); 00409 sendInt(EmbossLtColor); 00410 _ser.putc(' '); 00411 sendInt(TextColor0); 00412 _ser.putc(' '); 00413 sendInt(TextColor1); 00414 _ser.putc(' '); 00415 sendInt(TextColorDisabled); 00416 _ser.putc(' '); 00417 sendInt(Color0); 00418 _ser.putc(' '); 00419 sendInt(Color1); 00420 _ser.putc(' '); 00421 sendInt(ColorDisabled); 00422 _ser.putc(' '); 00423 sendInt(CommonBkColor); 00424 _ser.putc(' '); 00425 sendInt(Fontw); 00426 _ser.putc('\r'); 00427 waitForCR(); 00428 } 00429 void ezLCD3::groupBox( int ID, int x, int y, int w, int h, int options, int theme, int stringID) 00430 { 00431 sendInt(Set_Gbox); 00432 _ser.putc(' '); 00433 sendInt(ID); 00434 _ser.putc(' '); 00435 sendInt(x); 00436 _ser.putc(' '); 00437 sendInt(y); 00438 _ser.putc(' '); 00439 sendInt(w); 00440 _ser.putc(' '); 00441 sendInt(h); 00442 _ser.putc(' '); 00443 sendInt(options); 00444 _ser.putc(' '); 00445 sendInt(theme); 00446 _ser.putc(' '); 00447 sendInt(stringID); 00448 _ser.putc('\r'); 00449 waitForCR(); 00450 } 00451 00452 void ezLCD3::ameter( int ID, int x, int y, int w, int h, int options, int value, int min, int max, int theme, int stringID, int type) 00453 { 00454 sendInt(Set_AMeter); 00455 _ser.putc(' '); 00456 sendInt(ID); 00457 _ser.putc(' '); 00458 sendInt(x); 00459 _ser.putc(' '); 00460 sendInt(y); 00461 _ser.putc(' '); 00462 sendInt(w); 00463 _ser.putc(' '); 00464 sendInt(h); 00465 _ser.putc(' '); 00466 sendInt(options); 00467 _ser.putc(' '); 00468 sendInt(value); 00469 _ser.putc(' '); 00470 sendInt(min); 00471 _ser.putc(' '); 00472 sendInt(max); 00473 _ser.putc(' '); 00474 sendInt(theme); 00475 _ser.putc(' '); 00476 sendInt(stringID); 00477 _ser.putc(' '); 00478 sendInt(type); 00479 _ser.putc('\r'); 00480 waitForCR(); 00481 } 00482 00483 void ezLCD3::touchZone( int ID, int x, int y, int w, int h, bool option) 00484 { 00485 sendInt(Set_TouchZone); 00486 _ser.putc(' '); 00487 sendInt(ID); 00488 _ser.putc(' '); 00489 sendInt(x); 00490 _ser.putc(' '); 00491 sendInt(y); 00492 _ser.putc(' '); 00493 sendInt(w); 00494 _ser.putc(' '); 00495 sendInt(h); 00496 _ser.putc(' '); 00497 if(option) 00498 sendInt('1'); 00499 else 00500 sendInt('0'); 00501 _ser.putc('\r'); 00502 waitForCR(); 00503 } 00504 00505 void ezLCD3::button( int ID, int x, int y, int w, int h, int option, int align, int radius, int theme, int stringID ) 00506 { 00507 sendInt(Set_Button); 00508 _ser.putc(' '); 00509 sendInt(ID); 00510 _ser.putc(' '); 00511 sendInt(x); 00512 _ser.putc(' '); 00513 sendInt(y); 00514 _ser.putc(' '); 00515 sendInt(w); 00516 _ser.putc(' '); 00517 sendInt(h); 00518 _ser.putc(' '); 00519 sendInt(option); 00520 _ser.putc(' '); 00521 sendInt(align); 00522 _ser.putc(' '); 00523 sendInt(radius); 00524 _ser.putc(' '); 00525 sendInt(theme); 00526 _ser.putc(' '); 00527 sendInt(stringID); 00528 _ser.putc('\r'); 00529 waitForCR(); 00530 } 00531 void ezLCD3::image( char *str ) 00532 { 00533 sendInt(Picture); 00534 _ser.putc(' '); 00535 _ser.putc('\"'); 00536 sendString(str); 00537 _ser.putc('\"'); 00538 _ser.putc('\r'); 00539 waitForCR(); 00540 } 00541 00542 void ezLCD3::progressBar(int ID, int x, int y, int w, int h, int option, int value, int max, int theme, int stringID) 00543 { 00544 sendInt(Set_Progress); 00545 _ser.putc(' '); 00546 sendInt(ID); 00547 _ser.putc(' '); 00548 sendInt(x); 00549 _ser.putc(' '); 00550 sendInt(y); 00551 _ser.putc(' '); 00552 sendInt(w); 00553 _ser.putc(' '); 00554 sendInt(h); 00555 _ser.putc(' '); 00556 sendInt(option); 00557 _ser.putc(' '); 00558 sendInt(value); 00559 _ser.putc(' '); 00560 sendInt(max); 00561 _ser.putc(' '); 00562 sendInt(theme); 00563 _ser.putc(' '); 00564 sendInt(stringID); 00565 _ser.putc('\r'); 00566 waitForCR(); 00567 } 00568 00569 void ezLCD3::slider(int ID, int x, int y, int w, int h, int option, int range, int res, int value, int theme) 00570 { 00571 sendInt(Set_Slider); 00572 _ser.putc(' '); 00573 sendInt(ID); 00574 _ser.putc(' '); 00575 sendInt(x); 00576 _ser.putc(' '); 00577 sendInt(y); 00578 _ser.putc(' '); 00579 sendInt(w); 00580 _ser.putc(' '); 00581 sendInt(h); 00582 _ser.putc(' '); 00583 sendInt(option); 00584 _ser.putc(' '); 00585 sendInt(range); 00586 _ser.putc(' '); 00587 sendInt(res); 00588 _ser.putc(' '); 00589 sendInt(value); 00590 _ser.putc(' '); 00591 sendInt(theme); 00592 _ser.putc('\r'); 00593 waitForCR(); 00594 } 00595 00596 void ezLCD3::image( int x, int y, char *str ) 00597 { 00598 sendInt(Picture); 00599 _ser.putc(' '); 00600 sendInt(x); 00601 _ser.putc(' '); 00602 sendInt(y); 00603 _ser.putc(' '); 00604 _ser.putc('\"'); 00605 sendString(str); 00606 _ser.putc('\"'); 00607 _ser.putc('\r'); 00608 waitForCR(); 00609 } 00610 void ezLCD3::staticText( int ID, int x, int y, int w, int h, int option, int theme, int stringID) 00611 { 00612 sendInt(Set_StaticText); 00613 _ser.putc(' '); 00614 sendInt(ID); 00615 _ser.putc(' '); 00616 sendInt(x); 00617 _ser.putc(' '); 00618 sendInt(y); 00619 _ser.putc(' '); 00620 sendInt(w); 00621 _ser.putc(' '); 00622 sendInt(h); 00623 _ser.putc(' '); 00624 sendInt(option); 00625 _ser.putc(' '); 00626 sendInt(theme); 00627 _ser.putc(' '); 00628 sendInt(stringID); 00629 _ser.putc('\r'); 00630 waitForCR(); 00631 } 00632 00633 unsigned int ezLCD3::wstack( int type ) 00634 { 00635 sendInt( Wstack ); 00636 _ser.putc(' '); 00637 sendInt( type ); 00638 _ser.putc('\r'); 00639 getString( buf ); 00640 return getInt( buf ); 00641 } 00642 void ezLCD3::wvalue( int ID, int value) 00643 { 00644 sendInt( Widget_Values ); 00645 _ser.putc(' '); 00646 sendInt( ID ); 00647 _ser.putc(' '); 00648 sendInt( value ); 00649 _ser.putc('\r'); 00650 waitForCR(); 00651 } 00652 void ezLCD3::wstate( int ID, int option ) 00653 { 00654 sendInt( Widget_State ); 00655 _ser.putc(' '); 00656 sendInt( ID ); 00657 _ser.putc(' '); 00658 sendInt( option ); 00659 _ser.putc('\r'); 00660 waitForCR(); 00661 } 00662 00663 void ezLCD3::sendCommand(char *str) 00664 { 00665 sendString(str); 00666 _ser.putc('\r'); 00667 waitForCR(); 00668 } 00669 00670 int ezLCD3::_putc( int c) 00671 { 00672 sendInt(Print); 00673 _ser.putc(' '); 00674 _ser.putc('\"'); 00675 _ser.putc(c); 00676 _ser.putc('\"'); 00677 _ser.putc('\r'); 00678 waitForCR(); 00679 return (c); 00680 } 00681 00682 int ezLCD3::_getc(void) 00683 { 00684 char r = 0; 00685 return(r); 00686 } 00687 00688 int ezLCD3::putc ( int c ) 00689 { 00690 return(_ser.putc(c)); 00691 } 00692 00693 int ezLCD3::getc (void) 00694 { 00695 return(_ser.getc()); 00696 } 00697 00698 00699
Generated on Wed Jul 13 2022 05:25:49 by
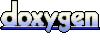