Class to communicate with ELV(R) MAX! wireless devices with RFM22B-Modules. Based on Library RF22. Initial version unable to send! Only receive! See http://mbed.org/users/charly/notebook/reading-a-max-wireless-window-sensor-with-rfm22-an/
Dependents: RF22_MAX_test_Send
RF22Max.h
00001 // RF22Max.h 00002 // 00003 // decode messages from ELV-MAX! wireless devices 00004 // based on RF22-Class (C) by Mike McCauley (mikem@open.com.au) for RFM22 transceiver-modules by HopeRF 00005 // 00006 00007 /// \version 1.00 Working class for Receive 00008 /// \author Karl Zweimueller http://mbed.org/users/charly/ 00009 00010 #ifndef RF22Max_h 00011 #define RF22Max_h 00012 00013 #include "mbed.h" 00014 #include <RF22.h> 00015 00016 // define DEBUG if you want to see bufer-output on pc (defined in main) 00017 #ifdef DEBUG 00018 extern Serial pc; 00019 #endif 00020 00021 #define lengthof(x) (sizeof(x) / sizeof(*x)) 00022 ///////////////////////////////////////////////////////////////////// 00023 /// \class RF22Max RF22Max.h <RF22Max.h> 00024 /// \brief receive (in future also send) packets from/to MAX! Devices by ELV (http://www.elv.de/max-funk-heizungsregler-system.html) 00025 /// 00026 /// This class provides basic functions for sending and receiving MAX!-messages, 00027 /// at the moment only receiving possible 00028 /// ueses recv() from RF22 00029 /// based on RF22-Class (C) by Mike McCauley (mikem@open.com.au) for RFM22 transceiver-modules by HopeRF 00030 /// returns decodes message and payload for further processing 00031 /// 00032 class RF22Max : public RF22 00033 { 00034 public: 00035 /// \brief a message from a max!-Device 00036 /// 00037 /// structure for a MAX!-Message 00038 /// includes decoded fields and the payload 00039 /// also has some specific field, which are only valid on some message-types 00040 /// 00041 /// Message-types: 00042 /// 00043 /// 0x00: "PairPing" 00044 /// 00045 /// 0x01: "PairPong"; 00046 /// 00047 /// 0x02: "Ack"; 00048 /// 00049 /// 0x03: "TimeInformation"; 00050 /// 00051 /// 0x10: "ConfigWeekProfile"; 00052 /// 00053 /// 0x11: "ConfigTemperatures"; 00054 /// 00055 /// 0x12: "ConfigValve"; 00056 /// 00057 /// 0x20: "AddLinkPartner"; 00058 /// 00059 /// 0x21: "RemoveLinkPartner"; 00060 /// 00061 /// 0x22: "SetGroupId"; 00062 /// 00063 /// 0x23: "RemoveGroupId"; 00064 /// 00065 /// 0x30: "ShutterContactState"; 00066 /// 00067 /// 0x40: "SetTemperature"; 00068 /// 00069 /// 0x42: "WallThermostatState"; 00070 /// 00071 /// 0x43: "SetComfortTemperature"; 00072 /// 00073 /// 0x44: "SetEcoTemperature"; 00074 /// 00075 /// 0x50: "PushButtonState"; 00076 /// 00077 /// 0x60: "ThermostatState"; 00078 /// 00079 /// 0x82: "SetDisplayActualTemperature"; 00080 /// 00081 /// 0xF1: "WakeUp"; 00082 /// 00083 /// 0xF0: "Reset"; 00084 /// 00085 00086 typedef struct { 00087 uint8_t len; ///< Message-length 00088 uint8_t cnt; ///< Message-counter 00089 uint8_t flags; ///< unknown 00090 uint8_t type; ///< Message-type 00091 char type_str[50]; ///< Message-Type in text 00092 uint32_t frm_adr; ///< Unique address of device From 00093 uint32_t to_adr; ///< Unique address of device To 00094 uint8_t groupid; ///< Groupid 00095 uint8_t payload[50]; ///< Data 00096 uint16_t crc; ///< CRC for the message 00097 char state[50]; ///< State of the device: open, closed, auto, eco,... 00098 char battery_state[50]; ///< Battery-state of the device : good, low 00099 } max_message; 00100 00101 /// Constructor 00102 /// Create an object for a RFM22-module connected to the controller via SPI, SlaveSelect and Interrupt-pin 00103 /// \param[in] slaveSelectPin SlaveSelectPin 00104 /// \param[in] mosi SPI 00105 /// \param[in] miso SPI 00106 /// \param[in] sclk SPI 00107 /// \param[in] interrupt Interrupt line for RFM22 00108 RF22Max(PinName slaveSelectPin, PinName mosi, PinName miso, PinName sclk, PinName interrupt); 00109 00110 /// Initialize the module for MAX!-messages 00111 /// sets frequency, datarate, ... 00112 /// \return true if everything was successful 00113 boolean init(); 00114 00115 /// start receiver and see if a valid MAX!-message is available and return it undecoded to the caller 00116 /// 00117 /// nonblocking 00118 /// 00119 /// do NOT check crc and DO dewithening 00120 /// \param[in] buf Location to copy the received message 00121 /// \param[in,out] len Pointer to available space in buf(copies maximum len bytes!). Set to the actual number of octets copied. 00122 /// \return true if a valid message was copied to buf 00123 boolean recv(uint8_t* buf, uint8_t* len); 00124 00125 /// start receiver and see if a valid MAX!-message is available and return the decoded message to the caller 00126 /// 00127 /// nonblocking 00128 /// 00129 /// check crc and do dewithening and do decoding of fields 00130 /// \param[in] message A pointer to a RF22Max::max_message 00131 /// \return true if a valid message was copied to buf 00132 boolean recv_max(RF22Max::max_message* message); 00133 00134 private: 00135 00136 #ifdef DEBUG 00137 /// show received buffer on serial pc 00138 void printHex(uint8_t *buf, size_t len, bool nl); 00139 #endif 00140 00141 00142 /// calc_crc_setup setup crc-calculation 00143 uint16_t calc_crc_step(uint8_t crcData, uint16_t crcReg); 00144 00145 public: 00146 /// calculate crc for the data in buf with len 00147 /// \param[in] buf message to calculate crc for 00148 /// \param[in,out] len of message 00149 uint16_t calc_crc(uint8_t *buf, size_t len); 00150 00151 00152 }; // end class RF22Max 00153 00154 #endif
Generated on Mon Jul 18 2022 04:16:30 by
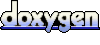