Fingerprint and demo library for ARM-STM32 made from the Arduino library for R503
Dependents: R503_fingerprint_HelloWorldV4
Fingerprint Class Reference
! Helper class to communicate with and keep state for fingerprint sensors More...
#include <Fingerprint.h>
Public Member Functions | |
Fingerprint (PinName serialTX, PinName serialRX, uint32_t password) | |
Instantiates sensor with Software Serial. | |
void | begin (uint32_t baud) |
Initializes serial interface and baud rate. | |
bool | verifyPassword (void) |
Verifies the sensors' access password (default password is 0x0000000). A good way to also check if the sensors is active and responding. | |
uint8_t | getParameters (void) |
Get the sensors parameters, fills in the member variables status_reg, system_id, capacity, security_level, device_addr, packet_len and baud_rate. | |
uint8_t | getImage (void) |
Ask the sensor to take an image of the finger pressed on surface. | |
uint8_t | image2Tz (uint8_t slot=1) |
Ask the sensor to convert image to feature template. | |
uint8_t | createModel (void) |
Ask the sensor to take two print feature template and create a model. | |
uint8_t | emptyDatabase (void) |
Ask the sensor to delete ALL models in memory. | |
uint8_t | storeModel (uint16_t id) |
Ask the sensor to store the calculated model for later matching. | |
uint8_t | loadModel (uint16_t id) |
Ask the sensor to load a fingerprint model from flash into buffer 1. | |
uint8_t | getModel (void) |
Ask the sensor to transfer 256-byte fingerprint template from the buffer to the UART. | |
uint8_t | deleteModel (uint16_t id) |
Ask the sensor to delete a model in memory. | |
uint8_t | fingerFastSearch (void) |
Ask the sensor to search the current slot 1 fingerprint features to match saved templates. The matching location is stored in fingerID and the matching confidence in confidence | |
uint8_t | fingerSearch (uint8_t slot=1) |
Ask the sensor to search the current slot fingerprint features to match saved templates. The matching location is stored in fingerID and the matching confidence in confidence | |
uint8_t | getTemplateCount (void) |
Ask the sensor for the number of templates stored in memory. The number is stored in templateCount on success. | |
uint8_t | setPassword (uint32_t password) |
Set the password on the sensor (future communication will require password verification so don't forget it!!!) | |
uint8_t | LEDcontrol (bool on) |
Control the built in LED. | |
uint8_t | LEDcontrol (uint8_t control, uint8_t speed, uint8_t coloridx, uint8_t count=0) |
Control the built in Aura LED (if exists). Check datasheet/manual for different colors and control codes available. | |
void | writeStructuredPacket (const Fingerprint_Packet &p) |
Helper function to process a packet and send it over UART to the sensor. | |
uint8_t | getStructuredPacket (Fingerprint_Packet *p, uint16_t timeout=DEFAULTTIMEOUT) |
Helper function to receive data over UART from the sensor and process it into a packet. | |
Data Fields | |
uint16_t | fingerID |
The matching location that is set by fingerFastSearch() | |
uint16_t | confidence |
The confidence of the fingerFastSearch() match, higher numbers are more confidents. | |
uint16_t | templateCount |
The number of stored templates in the sensor, set by getTemplateCount() | |
uint16_t | status_reg |
The status register (set by getParameters) | |
uint16_t | system_id |
The system identifier (set by getParameters) | |
uint16_t | capacity |
The fingerprint capacity (set by getParameters) | |
uint16_t | security_level |
The security level (set by getParameters) | |
uint32_t | device_addr |
The device address (set by getParameters) | |
uint16_t | packet_len |
The max packet length (set by getParameters) | |
uint16_t | baud_rate |
The UART baud rate (set by getParameters) |
Detailed Description
! Helper class to communicate with and keep state for fingerprint sensors
Definition at line 133 of file Fingerprint.h.
Constructor & Destructor Documentation
Fingerprint | ( | PinName | serialTX, |
PinName | serialRX, | ||
uint32_t | password | ||
) |
Instantiates sensor with Software Serial.
- Parameters:
-
ss Pointer to SoftwareSerial object password 32-bit integer password (default is 0)
< The status register (set by getParameters)
< The system identifier (set by getParameters)
< The fingerprint capacity (set by getParameters)
< The security level (set by getParameters)
< The device address (set by getParameters)
< The max packet length (set by getParameters)
< The UART baud rate (set by getParameters)
Definition at line 57 of file Fingerprint.cpp.
Member Function Documentation
void begin | ( | uint32_t | baudrate ) |
Initializes serial interface and baud rate.
- Parameters:
-
baudrate Sensor's UART baud rate (usually 57600, 9600 or 115200)
Definition at line 82 of file Fingerprint.cpp.
uint8_t createModel | ( | void | ) |
Ask the sensor to take two print feature template and create a model.
- Returns:
FINGERPRINT_OK
on success-
FINGERPRINT_PACKETRECIEVEERR
on communication error -
FINGERPRINT_ENROLLMISMATCH
on mismatch of fingerprints
Definition at line 176 of file Fingerprint.cpp.
uint8_t deleteModel | ( | uint16_t | location ) |
Ask the sensor to delete a model in memory.
- Parameters:
-
location The model location #
- Returns:
FINGERPRINT_OK
on success-
FINGERPRINT_BADLOCATION
if the location is invalid -
FINGERPRINT_FLASHERR
if the model couldn't be written to flash memory -
FINGERPRINT_PACKETRECIEVEERR
on communication error
Definition at line 229 of file Fingerprint.cpp.
uint8_t emptyDatabase | ( | void | ) |
Ask the sensor to delete ALL models in memory.
- Returns:
FINGERPRINT_OK
on success-
FINGERPRINT_BADLOCATION
if the location is invalid -
FINGERPRINT_FLASHERR
if the model couldn't be written to flash memory -
FINGERPRINT_PACKETRECIEVEERR
on communication error
Definition at line 243 of file Fingerprint.cpp.
uint8_t fingerFastSearch | ( | void | ) |
Ask the sensor to search the current slot 1 fingerprint features to match saved templates. The matching location is stored in fingerID and the matching confidence in confidence
- Returns:
FINGERPRINT_OK
on fingerprint match success-
FINGERPRINT_NOTFOUND
no match made -
FINGERPRINT_PACKETRECIEVEERR
on communication error
Definition at line 257 of file Fingerprint.cpp.
uint8_t fingerSearch | ( | uint8_t | slot = 1 ) |
Ask the sensor to search the current slot fingerprint features to match saved templates. The matching location is stored in fingerID and the matching confidence in confidence
- Parameters:
-
slot The slot to use for the print search, defaults to 1
- Returns:
FINGERPRINT_OK
on fingerprint match success-
FINGERPRINT_NOTFOUND
no match made -
FINGERPRINT_PACKETRECIEVEERR
on communication error
Definition at line 318 of file Fingerprint.cpp.
uint8_t getImage | ( | void | ) |
Ask the sensor to take an image of the finger pressed on surface.
- Returns:
FINGERPRINT_OK
on success-
FINGERPRINT_NOFINGER
if no finger detected -
FINGERPRINT_PACKETRECIEVEERR
on communication error -
FINGERPRINT_IMAGEFAIL
on imaging error
Definition at line 147 of file Fingerprint.cpp.
uint8_t getModel | ( | void | ) |
Ask the sensor to transfer 256-byte fingerprint template from the buffer to the UART.
- Returns:
FINGERPRINT_OK
on success-
FINGERPRINT_PACKETRECIEVEERR
on communication error
Definition at line 215 of file Fingerprint.cpp.
uint8_t getParameters | ( | void | ) |
Get the sensors parameters, fills in the member variables status_reg, system_id, capacity, security_level, device_addr, packet_len and baud_rate.
- Returns:
- True if password is correct
Definition at line 113 of file Fingerprint.cpp.
uint8_t getStructuredPacket | ( | Fingerprint_Packet * | packet, |
uint16_t | timeout = DEFAULTTIMEOUT |
||
) |
Helper function to receive data over UART from the sensor and process it into a packet.
- Parameters:
-
packet A structure containing the bytes received timeout how many milliseconds we're willing to wait
- Returns:
FINGERPRINT_OK
on success-
FINGERPRINT_TIMEOUT
orFINGERPRINT_BADPACKET
on failure
Definition at line 437 of file Fingerprint.cpp.
uint8_t getTemplateCount | ( | void | ) |
Ask the sensor for the number of templates stored in memory. The number is stored in templateCount on success.
- Returns:
FINGERPRINT_OK
on success-
FINGERPRINT_PACKETRECIEVEERR
on communication error
Definition at line 345 of file Fingerprint.cpp.
uint8_t image2Tz | ( | uint8_t | slot = 1 ) |
Ask the sensor to convert image to feature template.
- Parameters:
-
slot Location to place feature template (put one in 1 and another in 2 for verification to create model)
- Returns:
FINGERPRINT_OK
on success-
FINGERPRINT_IMAGEMESS
if image is too messy -
FINGERPRINT_PACKETRECIEVEERR
on communication error -
FINGERPRINT_FEATUREFAIL
on failure to identify fingerprint features -
FINGERPRINT_INVALIDIMAGE
on failure to identify fingerprint features
Definition at line 164 of file Fingerprint.cpp.
uint8_t LEDcontrol | ( | bool | on ) |
Control the built in LED.
- Parameters:
-
on True if you want LED on, False to turn LED off
- Returns:
FINGERPRINT_OK
on success
Definition at line 281 of file Fingerprint.cpp.
uint8_t LEDcontrol | ( | uint8_t | control, |
uint8_t | speed, | ||
uint8_t | coloridx, | ||
uint8_t | count = 0 |
||
) |
Control the built in Aura LED (if exists). Check datasheet/manual for different colors and control codes available.
- Parameters:
-
control The control code (e.g. breathing, full on) speed How fast to go through the breathing/blinking cycles coloridx What color to light the indicator count How many repeats of blinks/breathing cycles
- Returns:
FINGERPRINT_OK
on fingerprint match success-
FINGERPRINT_NOTFOUND
no match made -
FINGERPRINT_PACKETRECIEVEERR
on communication error
Definition at line 302 of file Fingerprint.cpp.
uint8_t loadModel | ( | uint16_t | location ) |
Ask the sensor to load a fingerprint model from flash into buffer 1.
- Parameters:
-
location The model location #
- Returns:
FINGERPRINT_OK
on success-
FINGERPRINT_BADLOCATION
if the location is invalid -
FINGERPRINT_PACKETRECIEVEERR
on communication error
Definition at line 203 of file Fingerprint.cpp.
uint8_t setPassword | ( | uint32_t | password ) |
Set the password on the sensor (future communication will require password verification so don't forget it!!!)
- Parameters:
-
password 32-bit password code
- Returns:
FINGERPRINT_OK
on success-
FINGERPRINT_PACKETRECIEVEERR
on communication error
Definition at line 364 of file Fingerprint.cpp.
uint8_t storeModel | ( | uint16_t | location ) |
Ask the sensor to store the calculated model for later matching.
- Parameters:
-
location The model location #
- Returns:
FINGERPRINT_OK
on success-
FINGERPRINT_BADLOCATION
if the location is invalid -
FINGERPRINT_FLASHERR
if the model couldn't be written to flash memory -
FINGERPRINT_PACKETRECIEVEERR
on communication error
Definition at line 190 of file Fingerprint.cpp.
bool verifyPassword | ( | void | ) |
Verifies the sensors' access password (default password is 0x0000000). A good way to also check if the sensors is active and responding.
- Returns:
- True if password is correct
Definition at line 93 of file Fingerprint.cpp.
void writeStructuredPacket | ( | const Fingerprint_Packet & | packet ) |
Helper function to process a packet and send it over UART to the sensor.
- Parameters:
-
packet A structure containing the bytes to transmit
Definition at line 377 of file Fingerprint.cpp.
Field Documentation
uint16_t baud_rate |
The UART baud rate (set by getParameters)
Definition at line 177 of file Fingerprint.h.
uint16_t capacity |
The fingerprint capacity (set by getParameters)
Definition at line 173 of file Fingerprint.h.
uint16_t confidence |
The confidence of the fingerFastSearch() match, higher numbers are more confidents.
Definition at line 167 of file Fingerprint.h.
uint32_t device_addr |
The device address (set by getParameters)
Definition at line 175 of file Fingerprint.h.
uint16_t fingerID |
The matching location that is set by fingerFastSearch()
Definition at line 164 of file Fingerprint.h.
uint16_t packet_len |
The max packet length (set by getParameters)
Definition at line 176 of file Fingerprint.h.
uint16_t security_level |
The security level (set by getParameters)
Definition at line 174 of file Fingerprint.h.
uint16_t status_reg |
The status register (set by getParameters)
Definition at line 171 of file Fingerprint.h.
uint16_t system_id |
The system identifier (set by getParameters)
Definition at line 172 of file Fingerprint.h.
uint16_t templateCount |
The number of stored templates in the sensor, set by getTemplateCount()
Definition at line 169 of file Fingerprint.h.
Generated on Thu Jul 14 2022 22:39:35 by
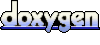