Fingerprint and demo library for ARM-STM32 made from the Arduino library for R503
Dependents: R503_fingerprint_HelloWorldV4
Fingerprint.h
00001 // STM32 adaptation by Christian Dupaty 03/2021 00002 00003 #ifndef FINGERPRINT_H 00004 #define FINGERPRINT_H 00005 00006 /*! 00007 * @file Fingerprint.h 00008 */ 00009 #include "mbed.h" 00010 #include <string.h> 00011 00012 typedef unsigned char uint8_t ; 00013 //typedef unsigned int uint16_t; 00014 //typedef unsigned long uint32_t; 00015 00016 #define FINGERPRINT_OK 0x00 //!< Command execution is complete 00017 #define FINGERPRINT_PACKETRECIEVEERR 0x01 //!< Error when receiving data package 00018 #define FINGERPRINT_NOFINGER 0x02 //!< No finger on the sensor 00019 #define FINGERPRINT_IMAGEFAIL 0x03 //!< Failed to enroll the finger 00020 #define FINGERPRINT_IMAGEMESS \ 00021 0x06 //!< Failed to generate character file due to overly disorderly 00022 //!< fingerprint image 00023 #define FINGERPRINT_FEATUREFAIL \ 00024 0x07 //!< Failed to generate character file due to the lack of character point 00025 //!< or small fingerprint image 00026 #define FINGERPRINT_NOMATCH 0x08 //!< Finger doesn't match 00027 #define FINGERPRINT_NOTFOUND 0x09 //!< Failed to find matching finger 00028 #define FINGERPRINT_ENROLLMISMATCH \ 00029 0x0A //!< Failed to combine the character files 00030 #define FINGERPRINT_BADLOCATION \ 00031 0x0B //!< Addressed PageID is beyond the finger library 00032 #define FINGERPRINT_DBRANGEFAIL \ 00033 0x0C //!< Error when reading template from library or invalid template 00034 #define FINGERPRINT_UPLOADFEATUREFAIL 0x0D //!< Error when uploading template 00035 #define FINGERPRINT_PACKETRESPONSEFAIL \ 00036 0x0E //!< Module failed to receive the following data packages 00037 #define FINGERPRINT_UPLOADFAIL 0x0F //!< Error when uploading image 00038 #define FINGERPRINT_DELETEFAIL 0x10 //!< Failed to delete the template 00039 #define FINGERPRINT_DBCLEARFAIL 0x11 //!< Failed to clear finger library 00040 #define FINGERPRINT_PASSFAIL \ 00041 0x13 //!< Find whether the fingerprint passed or failed 00042 #define FINGERPRINT_INVALIDIMAGE \ 00043 0x15 //!< Failed to generate image because of lac of valid primary image 00044 #define FINGERPRINT_FLASHERR 0x18 //!< Error when writing flash 00045 #define FINGERPRINT_INVALIDREG 0x1A //!< Invalid register number 00046 #define FINGERPRINT_ADDRCODE 0x20 //!< Address code 00047 #define FINGERPRINT_PASSVERIFY 0x21 //!< Verify the fingerprint passed 00048 #define FINGERPRINT_STARTCODE \ 00049 0xEF01 //!< Fixed falue of EF01H; High byte transferred first 00050 00051 #define FINGERPRINT_COMMANDPACKET 0x1 //!< Command packet 00052 #define FINGERPRINT_DATAPACKET \ 00053 0x2 //!< Data packet, must follow command packet or acknowledge packet 00054 #define FINGERPRINT_ACKPACKET 0x7 //!< Acknowledge packet 00055 #define FINGERPRINT_ENDDATAPACKET 0x8 //!< End of data packet 00056 00057 #define FINGERPRINT_TIMEOUT 0xFF //!< Timeout was reached 00058 #define FINGERPRINT_BADPACKET 0xFE //!< Bad packet was sent 00059 00060 #define FINGERPRINT_GETIMAGE 0x01 //!< Collect finger image 00061 #define FINGERPRINT_IMAGE2TZ 0x02 //!< Generate character file from image 00062 #define FINGERPRINT_SEARCH 0x04 //!< Search for fingerprint in slot 00063 #define FINGERPRINT_REGMODEL \ 00064 0x05 //!< Combine character files and generate template 00065 #define FINGERPRINT_STORE 0x06 //!< Store template 00066 #define FINGERPRINT_LOAD 0x07 //!< Read/load template 00067 #define FINGERPRINT_UPLOAD 0x08 //!< Upload template 00068 #define FINGERPRINT_DELETE 0x0C //!< Delete templates 00069 #define FINGERPRINT_EMPTY 0x0D //!< Empty library 00070 #define FINGERPRINT_READSYSPARAM 0x0F //!< Read system parameters 00071 #define FINGERPRINT_SETPASSWORD 0x12 //!< Sets passwords 00072 #define FINGERPRINT_VERIFYPASSWORD 0x13 //!< Verifies the password 00073 #define FINGERPRINT_HISPEEDSEARCH \ 00074 0x1B //!< Asks the sensor to search for a matching fingerprint template to the 00075 //!< last model generated 00076 #define FINGERPRINT_TEMPLATECOUNT 0x1D //!< Read finger template numbers 00077 #define FINGERPRINT_AURALEDCONFIG 0x35 //!< Aura LED control 00078 #define FINGERPRINT_LEDON 0x50 //!< Turn on the onboard LED 00079 #define FINGERPRINT_LEDOFF 0x51 //!< Turn off the onboard LED 00080 00081 #define FINGERPRINT_LED_BREATHING 0x01 //!< Breathing light 00082 #define FINGERPRINT_LED_FLASHING 0x02 //!< Flashing light 00083 #define FINGERPRINT_LED_ON 0x03 //!< Always on 00084 #define FINGERPRINT_LED_OFF 0x04 //!< Always off 00085 #define FINGERPRINT_LED_GRADUAL_ON 0x05 //!< Gradually on 00086 #define FINGERPRINT_LED_GRADUAL_OFF 0x06 //!< Gradually off 00087 #define FINGERPRINT_LED_RED 0x01 //!< Red LED 00088 #define FINGERPRINT_LED_BLUE 0x02 //!< Blue LED 00089 #define FINGERPRINT_LED_PURPLE 0x03 //!< Purple LED 00090 00091 ///////////////////////////////////////////////// 00092 // For debug uncomment this line 00093 #define FINGERPRINT_DEBUG 00094 ///////////////////////////////////////////////// 00095 00096 #define DEFAULTTIMEOUT 1000 //!< UART reading timeout in milliseconds 00097 00098 ///! Helper class to craft UART packets 00099 struct Fingerprint_Packet 00100 { 00101 /**************************************************************************/ 00102 /*! 00103 @brief Create a new UART-borne packet 00104 @param type Command, data, ack type packet 00105 @param length Size of payload 00106 @param data Pointer to bytes of size length we will memcopy into the 00107 internal buffer 00108 */ 00109 /**************************************************************************/ 00110 00111 Fingerprint_Packet(uint8_t type, uint16_t length, uint8_t *data) 00112 { 00113 this->start_code = FINGERPRINT_STARTCODE; 00114 this->type = type; 00115 this->length = length; 00116 address[0] = 0xFF; 00117 address[1] = 0xFF; 00118 address[2] = 0xFF; 00119 address[3] = 0xFF; 00120 if (length < 64) 00121 memcpy(this->data, data, length); 00122 else 00123 memcpy(this->data, data, 64); 00124 } 00125 uint16_t start_code; ///< "Wakeup" code for packet detection 00126 uint8_t address[4]; ///< 32-bit Fingerprint sensor address 00127 uint8_t type; ///< Type of packet 00128 uint16_t length; ///< Length of packet 00129 uint8_t data[64]; ///< The raw buffer for packet payload 00130 }; 00131 00132 ///! Helper class to communicate with and keep state for fingerprint sensors 00133 class Fingerprint { 00134 public: 00135 00136 Fingerprint(PinName serialTX, PinName serialRX, uint32_t password ); 00137 00138 void begin(uint32_t baud); 00139 00140 bool verifyPassword(void); 00141 uint8_t getParameters(void); 00142 00143 uint8_t getImage(void); 00144 uint8_t image2Tz(uint8_t slot = 1); 00145 uint8_t createModel(void); 00146 00147 uint8_t emptyDatabase(void); 00148 uint8_t storeModel(uint16_t id); 00149 uint8_t loadModel(uint16_t id); 00150 uint8_t getModel(void); 00151 uint8_t deleteModel(uint16_t id); 00152 uint8_t fingerFastSearch(void); 00153 uint8_t fingerSearch(uint8_t slot = 1); 00154 uint8_t getTemplateCount(void); 00155 uint8_t setPassword(uint32_t password); 00156 uint8_t LEDcontrol(bool on); 00157 uint8_t LEDcontrol(uint8_t control, uint8_t speed, uint8_t coloridx, uint8_t count = 0); 00158 00159 void writeStructuredPacket(const Fingerprint_Packet &p); 00160 uint8_t getStructuredPacket(Fingerprint_Packet *p, 00161 uint16_t timeout = DEFAULTTIMEOUT); 00162 00163 /// The matching location that is set by fingerFastSearch() 00164 uint16_t fingerID; 00165 /// The confidence of the fingerFastSearch() match, higher numbers are more 00166 /// confidents 00167 uint16_t confidence; 00168 /// The number of stored templates in the sensor, set by getTemplateCount() 00169 uint16_t templateCount; 00170 00171 uint16_t status_reg; ///< The status register (set by getParameters) 00172 uint16_t system_id; ///< The system identifier (set by getParameters) 00173 uint16_t capacity; ///< The fingerprint capacity (set by getParameters) 00174 uint16_t security_level; ///< The security level (set by getParameters) 00175 uint32_t device_addr; ///< The device address (set by getParameters) 00176 uint16_t packet_len; ///< The max packet length (set by getParameters) 00177 uint16_t baud_rate; ///< The UART baud rate (set by getParameters) 00178 00179 private: 00180 uint8_t checkPassword(void); 00181 uint32_t thePassword; 00182 uint32_t theAddress; 00183 uint8_t recvPacket[20]; 00184 uint8_t *pe; // pointeur ecriture buffer reception UART 00185 uint8_t *pl; // pointeur lecture buffer reception UART 00186 uint8_t buffUART[50]; // tampon reception UART 00187 void receiveUART(void); // recoit et stocke data dans buffUART 00188 uint8_t readUARTbuff(void); // retourne data de buffUART 00189 00190 protected: 00191 RawSerial R503Serial; 00192 }; 00193 00194 #endif
Generated on Thu Jul 14 2022 22:39:35 by
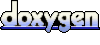