Sound recorder and playback device
Dependents: Seeed_Grove_Recorder_Example
ISD1820P.h
00001 #ifndef _ISD1820P_H_ 00002 #define _ISD1820P_H_ 00003 00004 #include "mbed.h" 00005 00006 /** ISD1820P class. 00007 * Used to control recording and playblack of sounds using this component: http://www.seeedstudio.com/depot/Grove-Recorder-p-1825.html 00008 */ 00009 class ISD1820P { 00010 public: 00011 /** 00012 * Constructor. Initializes the maximum record/playback sound to defaultMaxSoundTime. 00013 * 00014 * @param recordPin The pin that controls recording. 00015 * @param playPin The pin that controls playback. 00016 */ 00017 ISD1820P(PinName recordPin, PinName playPin); 00018 00019 /** 00020 * Starts a new recording. 00021 * 00022 * @param time Controls how long to record. 00023 */ 00024 void startRecording(float time); 00025 00026 /** 00027 * Starts a new recording with a length of the maximum time. 00028 */ 00029 void startRecordingMaxTime(); 00030 00031 /** 00032 * Stops the current recording. 00033 */ 00034 void stopRecording(); 00035 00036 /** 00037 * Starts playback of the previous recording. 00038 * 00039 * @param time Controls how long to play the last recording. 00040 */ 00041 void startPlaying(float time); 00042 00043 00044 /** 00045 * Starts playback of the previous recording up to the maximum time. 00046 */ 00047 void startPlayingMaxTime(); 00048 00049 /** 00050 * Stops the current playback 00051 */ 00052 void stopPlaying(); 00053 00054 /** 00055 * Set the default max recording and playback time. The default is 10 seconds. 00056 * 00057 * @param maxTime The maximum time in seconds to record and playback sounds. 00058 */ 00059 void setMaxSoundTime(float maxTime); 00060 00061 00062 private: 00063 /** 00064 * Default sound recording/playback is 10 seconds 00065 */ 00066 const static float defaultMaxSoundTime = 10.0f; 00067 00068 float maxSoundTime; 00069 00070 DigitalOut record; 00071 DigitalOut play; 00072 00073 Timeout recordTimeout; 00074 Timeout playTimeout; 00075 }; 00076 00077 #endif
Generated on Wed Jul 13 2022 00:04:16 by
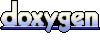