
Publish Grove Cayenne Spider Test
Dependencies: SX127x lorawan1v1
Fork of LoRaWAN-grove-cayenne by
vt100.h
00001 /* 00002 / _____) _ | | 00003 ( (____ _____ ____ _| |_ _____ ____| |__ 00004 \____ \| ___ | (_ _) ___ |/ ___) _ \ 00005 _____) ) ____| | | || |_| ____( (___| | | | 00006 (______/|_____)_|_|_| \__)_____)\____)_| |_| 00007 (C)2015 Semtech 00008 00009 Description: VT100 terminal support class 00010 00011 License: Revised BSD License, see LICENSE.TXT file include in the project 00012 00013 Maintainer: Miguel Luis and Gregory Cristian 00014 */ 00015 #ifndef __VT100_H__ 00016 #define __VT100_H__ 00017 00018 #ifndef STRING_STACK_LIMIT 00019 #define STRING_STACK_LIMIT 120 00020 #endif 00021 00022 #ifdef TARGET_MOTE_L152RC 00023 #define VT100_BAUD 115200 00024 #elif defined(TARGET_DISCO_L072CZ_LRWAN1) 00025 #define VT100_BAUD 38400 /* HSI? */ 00026 #elif defined(TARGET_NUCLEO_L152RE) 00027 #define VT100_BAUD 115200 00028 #elif defined(TARGET_NUCLEO_L073RZ) 00029 #define VT100_BAUD 115200 00030 #elif defined(TARGET_NRF52_DK) 00031 #define VT100_BAUD 115200 00032 #else 00033 #error find_usable_vt100_baudrate 00034 #endif 00035 00036 /** 00037 * Implements VT100 terminal commands support. 00038 * Implments also the same behaviour has RawSerial class. The only difference 00039 * is located in putc fucntion where writeable check is made befor sending the character. 00040 */ 00041 class VT100 : public SerialBase 00042 { 00043 public: 00044 enum TextAttributes 00045 { 00046 ATTR_OFF = 0, 00047 BOLD = 1, 00048 USCORE = 4, 00049 BLINK = 5, 00050 REVERSE = 7, 00051 BOLD_OFF = 21, 00052 USCORE_OFF = 24, 00053 BLINK_OFF = 25, 00054 REVERSE_OFF = 27, 00055 }; 00056 00057 enum Colors 00058 { 00059 BLACK = 0, 00060 RED = 1, 00061 GREEN = 2, 00062 BROWN = 3, 00063 BLUE = 4, 00064 MAGENTA = 5, 00065 CYAN = 6, 00066 WHITE = 7, 00067 }; 00068 00069 VT100( PinName tx, PinName rx ): SerialBase(tx, rx, VT100_BAUD) 00070 { 00071 // initializes terminal to "power-on" settings 00072 // ESC c 00073 this->printf( "\x1B\x63" ); 00074 } 00075 00076 void ClearScreen( uint8_t param ) 00077 { 00078 // ESC [ Ps J 00079 // 0 Clear screen from cursor down 00080 // 1 Clear screen from cursor up 00081 // 2 Clear entire screen 00082 this->printf( "\x1B[%dJ", param ); 00083 } 00084 00085 void ClearLine( uint8_t param ) 00086 { 00087 // ESC [ Ps K 00088 // 0 Erase from the active position to the end of the line, inclusive (default) 00089 // 1 Erase from the start of the screen to the active position, inclusive 00090 // 2 Erase all of the line, inclusive 00091 this->printf( "\x1B[%dK", param ); 00092 } 00093 00094 void SetAttribute( uint8_t attr ) 00095 { 00096 // ESC [ Ps;...;Ps m 00097 this->printf( "\x1B[%dm", attr ); 00098 } 00099 00100 void SetAttribute( uint8_t attr, uint8_t fgcolor, uint8_t bgcolor ) 00101 { 00102 // ESC [ Ps;...;Ps m 00103 this->printf( "\x1B[%d;%d;%dm", attr, fgcolor + 30, bgcolor + 40 ); 00104 } 00105 00106 void SetCursorMode( uint8_t visible ) 00107 { 00108 if( visible == true ) 00109 { 00110 // ESC [ ? 25 h 00111 this->printf( "\x1B[?25h" ); 00112 } 00113 else 00114 { 00115 // ESC [ ? 25 l 00116 this->printf( "\x1B[?25l" ); 00117 } 00118 } 00119 00120 void SetCursorPos( uint8_t line, uint8_t col ) 00121 { 00122 // ESC [ Pl ; Pc H 00123 this->printf( "\x1B[%d;%dH", line, col ); 00124 } 00125 00126 void PutStringAt( uint8_t line, uint8_t col, const char *s ) 00127 { 00128 this->SetCursorPos( line, col ); 00129 this->printf( "%s", s ); 00130 } 00131 00132 void PutCharAt( uint8_t line, uint8_t col, uint8_t c ) 00133 { 00134 this->SetCursorPos( line, col ); 00135 this->printf( "%c", c ); 00136 } 00137 00138 void PutHexAt( uint8_t line, uint8_t col, uint16_t n ) 00139 { 00140 this->SetCursorPos( line, col ); 00141 this->printf( "%X", n ); 00142 } 00143 00144 void PutBoxDrawingChar( uint8_t c ) 00145 { 00146 this->printf( "\x1B(0%c\x1b(B", c ); 00147 } 00148 00149 bool Readable( void ) 00150 { 00151 return this->readable( ); 00152 } 00153 00154 uint8_t GetChar( void ) 00155 { 00156 return this->getc( ); 00157 } 00158 00159 /* 00160 * RawSerial class implmentation copy. 00161 */ 00162 /** Read a char from the serial port 00163 * 00164 * @returns The char read from the serial port 00165 */ 00166 int getc( ) 00167 { 00168 return _base_getc( ); 00169 } 00170 00171 /** Write a char to the serial port 00172 * 00173 * @param c The char to write 00174 * 00175 * @returns The written char or -1 if an error occured 00176 */ 00177 int putc( int c ) 00178 { 00179 while( this->writeable( ) != 1 ); 00180 return _base_putc( c ); 00181 } 00182 00183 /** Write a string to the serial port 00184 * 00185 * @param str The string to write 00186 * 00187 * @returns 0 if the write succeeds, EOF for error 00188 */ 00189 int puts( const char *str ) 00190 { 00191 while( *str ) 00192 putc( *str++ ); 00193 return 0; 00194 } 00195 00196 // Experimental support for printf in RawSerial. No Stream inheritance 00197 // means we can't call printf() directly, so we use sprintf() instead. 00198 // We only call malloc() for the sprintf() buffer if the buffer 00199 // length is above a certain threshold, otherwise we use just the stack. 00200 int printf( const char *format, ... ) 00201 { 00202 std::va_list arg; 00203 va_start( arg, format ); 00204 int len = vsnprintf( NULL, 0, format, arg ); 00205 if( len < STRING_STACK_LIMIT ) 00206 { 00207 char temp[STRING_STACK_LIMIT]; 00208 vsprintf( temp, format, arg ); 00209 puts( temp ); 00210 } 00211 else 00212 { 00213 char *temp = new char[len + 1]; 00214 vsprintf( temp, format, arg ); 00215 puts( temp ); 00216 delete[] temp; 00217 } 00218 va_end( arg ); 00219 return len; 00220 } 00221 00222 private: 00223 00224 }; 00225 00226 #endif // __VT100_H__
Generated on Wed Jul 13 2022 22:22:27 by
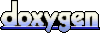