
Publish Grove Cayenne Spider Test
Dependencies: SX127x lorawan1v1
Fork of LoRaWAN-grove-cayenne by
SerialDisplay.cpp
00001 /* 00002 / _____) _ | | 00003 ( (____ _____ ____ _| |_ _____ ____| |__ 00004 \____ \| ___ | (_ _) ___ |/ ___) _ \ 00005 _____) ) ____| | | || |_| ____( (___| | | | 00006 (______/|_____)_|_|_| \__)_____)\____)_| |_| 00007 (C)2015 Semtech 00008 00009 Description: VT100 serial display management 00010 00011 License: Revised BSD License, see LICENSE.TXT file include in the project 00012 00013 Maintainer: Miguel Luis and Gregory Cristian 00014 */ 00015 #include "lorawan_board.h" 00016 00017 #include "SerialDisplay.h" 00018 #include "LoRaMacString.h" 00019 00020 #ifdef ENABLE_VT100 00021 VT100 vt( USBTX, USBRX ); 00022 00023 void SerialPrintCheckBox( bool activated, uint8_t color ) 00024 { 00025 if( activated == true ) 00026 { 00027 vt.SetAttribute( VT100::ATTR_OFF, color, color ); 00028 } 00029 else 00030 { 00031 vt.SetAttribute( VT100::ATTR_OFF ); 00032 } 00033 vt.printf( " " ); 00034 vt.SetAttribute( VT100::ATTR_OFF ); 00035 } 00036 00037 #ifdef LORAWAN_JOIN_EUI 00038 void SerialDisplayUpdateActivationMode( bool otaa ) 00039 { 00040 vt.SetCursorPos( 4, 17 ); // TODO 00041 SerialPrintCheckBox( otaa, VT100::WHITE ); 00042 } 00043 00044 void SerialDisplayUpdateEui( uint8_t line, const uint8_t *eui ) 00045 { 00046 vt.SetCursorPos( line, 27 ); 00047 for( uint8_t i = 0; i < 8; i++ ) 00048 { 00049 vt.printf( "%02x ", eui[i] ); 00050 } 00051 vt.SetCursorPos( line, 50 ); 00052 vt.printf( "]" ); 00053 } 00054 #endif /* LORAWAN_JOIN_EUI */ 00055 00056 void SerialDisplayUpdateKey( uint8_t line, const uint8_t *key ) 00057 { 00058 vt.SetCursorPos( line, 29 ); 00059 for( uint8_t i = 0; i < 16; i++ ) 00060 { 00061 vt.printf( "%02x ", key[i] ); 00062 } 00063 vt.SetCursorPos( line, 76 ); 00064 vt.printf( "]" ); 00065 } 00066 00067 void SerialDisplayUpdateNwkId( uint8_t id ) 00068 { 00069 vt.SetCursorPos( ROW_NwkId, 27 ); 00070 vt.printf( "%03d", id ); 00071 } 00072 00073 void SerialDisplayUpdateDevAddr( uint32_t addr ) 00074 { 00075 vt.SetCursorPos( ROW_DevAddr, 27 ); 00076 vt.printf( "%02X %02X %02X %02X", ( addr >> 24 ) & 0xFF, ( addr >> 16 ) & 0xFF, ( addr >> 8 ) & 0xFF, addr & 0xFF ); 00077 } 00078 00079 void SerialDisplayUpdateFrameType( bool confirmed ) 00080 { 00081 vt.SetCursorPos( ROW_FrameType, 17 ); 00082 SerialPrintCheckBox( confirmed, VT100::WHITE ); 00083 vt.SetCursorPos( ROW_FrameType, 32 ); 00084 SerialPrintCheckBox( !confirmed, VT100::WHITE ); 00085 } 00086 00087 void SerialDisplayUpdateAdr( bool adr ) 00088 { 00089 vt.SetCursorPos( ROW_ADR, 27 ); 00090 if( adr == true ) 00091 { 00092 vt.printf( " ON" ); 00093 } 00094 else 00095 { 00096 vt.printf( "OFF" ); 00097 } 00098 } 00099 00100 void SerialDisplayUpdateDutyCycle( bool dutyCycle ) 00101 { 00102 vt.SetCursorPos( ROW_DUTY, 27 ); 00103 if( dutyCycle == true ) 00104 { 00105 vt.printf( " ON" ); 00106 } 00107 else 00108 { 00109 vt.printf( "OFF" ); 00110 } 00111 } 00112 00113 void SerialDisplayUpdatePublicNetwork( bool network ) 00114 { 00115 vt.SetCursorPos( ROW_PUBLIC, 17 ); 00116 SerialPrintCheckBox( network, VT100::WHITE ); 00117 vt.SetCursorPos( ROW_PUBLIC, 30 ); 00118 SerialPrintCheckBox( !network, VT100::WHITE ); 00119 } 00120 00121 #ifdef LORAWAN_JOIN_EUI 00122 void SerialDisplayUpdateNetworkIsJoined( bool state ) 00123 { 00124 vt.SetCursorPos( ROW_JOINED, 17 ); 00125 SerialPrintCheckBox( !state, VT100::RED ); 00126 vt.SetCursorPos( ROW_JOINED, 30 ); 00127 SerialPrintCheckBox( state, VT100::GREEN ); 00128 } 00129 #endif /* LORAWAN_JOIN_EUI */ 00130 00131 /*void SerialDisplayUpdateLedState( uint8_t id, uint8_t state ) 00132 { 00133 switch( id ) 00134 { 00135 case 1: 00136 vt.SetCursorPos( 22, 17 ); 00137 SerialPrintCheckBox( state, VT100::RED ); 00138 break; 00139 case 2: 00140 vt.SetCursorPos( 22, 31 ); 00141 SerialPrintCheckBox( state, VT100::GREEN ); 00142 break; 00143 case 3: 00144 vt.SetCursorPos( 22, 45 ); 00145 SerialPrintCheckBox( state, VT100::BLUE ); 00146 break; 00147 } 00148 }*/ 00149 00150 void SerialDisplayUpdateData( uint8_t line, const uint8_t *buffer, uint8_t size ) 00151 { 00152 if( size != 0 ) 00153 { 00154 vt.SetCursorPos( line, 27 ); 00155 for( uint8_t i = 0; i < size; i++ ) 00156 { 00157 vt.printf( "%02X ", buffer[i] ); 00158 if( ( ( i + 1 ) % 16 ) == 0 ) 00159 { 00160 line++; 00161 vt.SetCursorPos( line, 27 ); 00162 } 00163 } 00164 for( uint8_t i = size; i < 64; i++ ) 00165 { 00166 vt.printf( "__ " ); 00167 if( ( ( i + 1 ) % 16 ) == 0 ) 00168 { 00169 line++; 00170 vt.SetCursorPos( line, 27 ); 00171 } 00172 } 00173 vt.SetCursorPos( line - 1, 74 ); 00174 vt.printf( "]" ); 00175 } 00176 else 00177 { 00178 vt.SetCursorPos( line, 27 ); 00179 for( uint8_t i = 0; i < 64; i++ ) 00180 { 00181 vt.printf( "__ " ); 00182 if( ( ( i + 1 ) % 16 ) == 0 ) 00183 { 00184 line++; 00185 vt.SetCursorPos( line, 27 ); 00186 } 00187 } 00188 vt.SetCursorPos( line - 1, 74 ); 00189 vt.printf( "]" ); 00190 } 00191 } 00192 00193 void SerialDisplayUpdateUplinkAcked( bool state ) 00194 { 00195 vt.SetCursorPos( ROW_UPLINK_ACKED, 36 ); 00196 SerialPrintCheckBox( state, VT100::GREEN ); 00197 } 00198 00199 //void SerialDisplayUpdateUplink( bool acked, uint8_t datarate, uint32_t counter, uint8_t port, uint8_t *buffer, uint8_t bufferSize, LoRaMacEventInfoStatus_t s) 00200 void SerialDisplayUplink(uint8_t fport, const uint8_t* buffer, uint8_t bufferSize ) 00201 { 00202 vt.SetCursorPos( ROW_DOWNLINK_RSSI, 32 ); 00203 vt.printf( " "); 00204 vt.SetCursorPos( ROW_DOWNLINK_SNR, 32 ); 00205 vt.printf( " "); 00206 00207 // Port 00208 vt.SetCursorPos( ROW_UPLINK_PORT, 34 ); 00209 vt.printf( "%3u", fport ); 00210 // Data 00211 SerialDisplayUpdateData( ROW_UPLINK_PAYLOAD, buffer, bufferSize ); 00212 } 00213 00214 void SerialDisplayMcpsConfirm( const McpsConfirm_t* mc) 00215 { 00216 char str[48]; 00217 static uint8_t lastStrLen = 0; 00218 // Acked 00219 SerialDisplayUpdateUplinkAcked(mc->AckReceived); 00220 LoRaMacEventInfoStatus_to_string(mc->Status, str); 00221 vt.printf("] %s", str); 00222 for (unsigned n = 0; n < lastStrLen; n++) 00223 vt.putc(' ' ); 00224 lastStrLen = strlen(str); 00225 // Datarate 00226 vt.SetCursorPos( ROW_UPLINK_DR, 33 ); 00227 vt.printf( "DR%u", mc->Datarate); 00228 // Counter 00229 vt.SetCursorPos( ROW_UPLINK_FCNT, 27 ); 00230 vt.printf( "%10" PRIu32, mc->UpLinkCounter); 00231 } 00232 00233 void SerialDisplayMcpsIndication() 00234 { 00235 } 00236 00237 void SerialDisplayUpdateDonwlinkRxData( bool state ) 00238 { 00239 vt.SetCursorPos( ROW_DOWNLINK_SNR, 4 ); 00240 SerialPrintCheckBox( state, VT100::GREEN ); 00241 } 00242 00243 void SerialDisplayMcpsIndication(const McpsIndication_t* mi) 00244 { 00245 int8_t Snr; 00246 00247 vt.SetCursorPos( ROW_DOWNLINK_RSSI, 32 ); 00248 vt.printf( "%5d", mi->Rssi ); 00249 00250 if (mi->Snr & 0x80) { // The SNR sign bit is 1 00251 // Invert and divide by 4 00252 Snr = ( ( ~mi->Snr + 1 ) & 0xFF ) >> 2; 00253 Snr = -Snr; 00254 } else { 00255 // Divide by 4 00256 Snr = ( mi->Snr & 0xFF ) >> 2; 00257 } 00258 vt.SetCursorPos( ROW_DOWNLINK_SNR, 32 ); 00259 vt.printf( "%5d", Snr ); 00260 00261 vt.SetCursorPos( ROW_DOWNLINK_FCNT, 27 ); 00262 vt.printf( "%10" PRIu32, mi->receivedFCntDown); 00263 00264 SerialDisplayUpdateDonwlinkRxData(mi->RxData); 00265 vt.SetCursorPos( ROW_DOWNLINK_FPORT, 34 ); 00266 if (mi->RxData) { 00267 vt.printf( "%3u", mi->Port); 00268 SerialDisplayUpdateData( ROW_DOWNLINK_PAYLOAD, mi->Buffer, mi->BufferSize ); 00269 } else { 00270 vt.printf(" "); 00271 SerialDisplayUpdateData( ROW_DOWNLINK_PAYLOAD, NULL, 0 ); 00272 } 00273 /*if (mcpsIndication->RxData) { 00274 LoRaMacDownlinkStatus.Port = mcpsIndication->Port; 00275 LoRaMacDownlinkStatus.Buffer = mcpsIndication->Buffer; 00276 LoRaMacDownlinkStatus.BufferSize = mcpsIndication->BufferSize; 00277 } else 00278 LoRaMacDownlinkStatus.Buffer = NULL;*/ 00279 } 00280 00281 #if 0 00282 void SerialDisplayUpdateDownlink( int16_t rssi, int8_t snr, uint32_t counter, uint8_t port, uint8_t *buffer, uint8_t bufferSize ) 00283 { 00284 // Rx data 00285 SerialDisplayUpdateDonwlinkRxData( buffer != NULL ); 00286 // RSSI 00287 vt.SetCursorPos( ROW_DOWNLINK_RSSI, 32 ); 00288 vt.printf( "%5d", rssi ); 00289 // SNR 00290 vt.SetCursorPos( ROW_DOWNLINK_SNR, 32 ); 00291 vt.printf( "%5d", snr ); 00292 // Counter 00293 vt.SetCursorPos( ROW_DOWNLINK_FCNT, 27 ); 00294 vt.printf( "%10" PRIu32, counter ); 00295 if( buffer != NULL ) 00296 { 00297 // Port 00298 vt.SetCursorPos( ROW_DOWNLINK_FPORT, 34 ); 00299 vt.printf( "%3u", port ); 00300 // Data 00301 SerialDisplayUpdateData( ROW_DOWNLINK_PAYLOAD, buffer, bufferSize ); 00302 } 00303 else 00304 { 00305 // Port 00306 vt.SetCursorPos( ROW_DOWNLINK_FPORT, 34 ); 00307 vt.printf( " " ); 00308 // Data 00309 SerialDisplayUpdateData( ROW_DOWNLINK_PAYLOAD, NULL, 0 ); 00310 } 00311 } 00312 #endif /* if 0 */ 00313 00314 void SerialDisplayDrawFirstLine( void ) 00315 { 00316 vt.PutBoxDrawingChar( 'l' ); 00317 for( int8_t i = 0; i <= 77; i++ ) 00318 { 00319 vt.PutBoxDrawingChar( 'q' ); 00320 } 00321 vt.PutBoxDrawingChar( 'k' ); 00322 vt.printf( "\r\n" ); 00323 } 00324 00325 void SerialDisplayDrawTitle( const char* title ) 00326 { 00327 vt.PutBoxDrawingChar( 'x' ); 00328 vt.printf( "%s", title ); 00329 vt.PutBoxDrawingChar( 'x' ); 00330 vt.printf( "\r\n" ); 00331 } 00332 void SerialDisplayDrawTopSeparator( void ) 00333 { 00334 vt.PutBoxDrawingChar( 't' ); 00335 for( int8_t i = 0; i <= 11; i++ ) 00336 { 00337 vt.PutBoxDrawingChar( 'q' ); 00338 } 00339 vt.PutBoxDrawingChar( 'w' ); 00340 for( int8_t i = 0; i <= 64; i++ ) 00341 { 00342 vt.PutBoxDrawingChar( 'q' ); 00343 } 00344 vt.PutBoxDrawingChar( 'u' ); 00345 vt.printf( "\r\n" ); 00346 } 00347 00348 void SerialDisplayDrawColSeparator( void ) 00349 { 00350 vt.PutBoxDrawingChar( 'x' ); 00351 for( int8_t i = 0; i <= 11; i++ ) 00352 { 00353 vt.PutBoxDrawingChar( ' ' ); 00354 } 00355 vt.PutBoxDrawingChar( 't' ); 00356 for( int8_t i = 0; i <= 64; i++ ) 00357 { 00358 vt.PutBoxDrawingChar( 'q' ); 00359 } 00360 vt.PutBoxDrawingChar( 'u' ); 00361 vt.printf( "\r\n" ); 00362 } 00363 00364 void SerialDisplayDrawSeparator( void ) 00365 { 00366 vt.PutBoxDrawingChar( 't' ); 00367 for( int8_t i = 0; i <= 11; i++ ) 00368 { 00369 vt.PutBoxDrawingChar( 'q' ); 00370 } 00371 vt.PutBoxDrawingChar( 'n' ); 00372 for( int8_t i = 0; i <= 64; i++ ) 00373 { 00374 vt.PutBoxDrawingChar( 'q' ); 00375 } 00376 vt.PutBoxDrawingChar( 'u' ); 00377 vt.printf( "\r\n" ); 00378 } 00379 00380 void SerialDisplayDrawLine( const char* firstCol, const char* secondCol ) 00381 { 00382 vt.PutBoxDrawingChar( 'x' ); 00383 vt.printf( "%s", firstCol ); 00384 vt.PutBoxDrawingChar( 'x' ); 00385 vt.printf( "%s", secondCol ); 00386 vt.PutBoxDrawingChar( 'x' ); 00387 vt.printf( "\r\n" ); 00388 } 00389 00390 void SerialDisplayDrawBottomLine( void ) 00391 { 00392 vt.PutBoxDrawingChar( 'm' ); 00393 for( int8_t i = 0; i <= 11; i++ ) 00394 { 00395 vt.PutBoxDrawingChar( 'q' ); 00396 } 00397 vt.PutBoxDrawingChar( 'v' ); 00398 for( int8_t i = 0; i <= 64; i++ ) 00399 { 00400 vt.PutBoxDrawingChar( 'q' ); 00401 } 00402 vt.PutBoxDrawingChar( 'j' ); 00403 vt.printf( "\r\n" ); 00404 } 00405 00406 void SerialDisplayInit( void ) 00407 { 00408 vt.ClearScreen( 2 ); 00409 vt.SetCursorMode( false ); 00410 vt.SetCursorPos( 0, 0 ); 00411 00412 // "+-----------------------------------------------------------------------------+" ); 00413 SerialDisplayDrawFirstLine( ); 00414 // "¦ LoRaWAN Demonstration Application ¦" ); 00415 SerialDisplayDrawTitle( " LoRaWAN Demonstration Application " ); 00416 // "+------------+----------------------------------------------------------------¦" ); 00417 SerialDisplayDrawTopSeparator( ); 00418 #ifdef LORAWAN_JOIN_EUI 00419 // "¦ Activation ¦ [ ]Over The Air ¦" ); 00420 SerialDisplayDrawLine( " Activation ", " [ ]Over The Air " ); 00421 // "¦ ¦ DevEui [__ __ __ __ __ __ __ __] ¦" ); 00422 SerialDisplayDrawLine( " ", " DevEui [__ __ __ __ __ __ __ __] " ); 00423 // "¦ ¦ JoinEui [__ __ __ __ __ __ __ __] ¦" ); 00424 SerialDisplayDrawLine( " ", " JoinEui [__ __ __ __ __ __ __ __] " ); 00425 // "¦ ¦ NwkKey [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __] ¦" ); 00426 SerialDisplayDrawLine( " ", " NwkKey [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __] " ); 00427 #ifdef OPTNEG 00428 SerialDisplayDrawLine( " ", " AppKey [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __] " ); 00429 #endif 00430 // "¦ +----------------------------------------------------------------¦" ); 00431 SerialDisplayDrawColSeparator( ); 00432 #endif /* LORAWAN_JOIN_EUI */ 00433 // "¦ ¦ [x]Personalisation ¦" ); 00434 SerialDisplayDrawLine( " ", " [ ]Personalisation " ); 00435 // "¦ ¦ NwkId [___] ¦" ); 00436 SerialDisplayDrawLine( " ", " NwkId [___] " ); 00437 // "¦ ¦ DevAddr [__ __ __ __] ¦" ); 00438 SerialDisplayDrawLine( " ", " DevAddr [__ __ __ __] " ); 00439 #ifdef OPTNEG 00440 SerialDisplayDrawLine( " ", " FNwkSIntKey [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __] "); 00441 SerialDisplayDrawLine( " ", " SNwkSIntKey [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __] "); 00442 SerialDisplayDrawLine( " ", " NwkSEncKey [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __] "); 00443 #else 00444 // "¦ ¦ NwkSKey [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __] ¦" ); 00445 SerialDisplayDrawLine( " ", " NwkSKey [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __] "); 00446 #endif 00447 // "¦ ¦ AppSKey [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __] ¦" ); 00448 SerialDisplayDrawLine( " ", " AppSKey [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __] "); 00449 // "+------------+----------------------------------------------------------------¦" ); 00450 SerialDisplayDrawSeparator( ); 00451 // "¦ MAC params ¦ [ ]Confirmed / [ ]Unconfirmed ¦" ); 00452 SerialDisplayDrawLine( " MAC params ", " [ ]Confirmed / [ ]Unconfirmed " ); 00453 // "¦ ¦ ADR [ ] ¦" ); 00454 SerialDisplayDrawLine( " ", " ADR [ ] " ); 00455 // "¦ ¦ Duty cycle[ ] ¦" ); 00456 SerialDisplayDrawLine( " ", " Duty cycle[ ] " ); 00457 // "+------------+----------------------------------------------------------------¦" ); 00458 SerialDisplayDrawSeparator( ); 00459 // "¦ Network ¦ [ ]Public / [ ]Private ¦" ); 00460 SerialDisplayDrawLine( " Network ", " [ ]Public / [ ]Private " ); 00461 #ifdef LORAWAN_JOIN_EUI 00462 // "¦ ¦ [ ]Joining / [ ]Joined ¦" ); 00463 SerialDisplayDrawLine( " ", " [ ]Joining / [ ]Joined " ); 00464 #endif /* LORAWAN_JOIN_EUI */ 00465 // "+------------+----------------------------------------------------------------¦" ); 00466 SerialDisplayDrawSeparator( ); 00467 // "¦ Uplink ¦ Acked [ ] ¦" ); 00468 SerialDisplayDrawLine( " Uplink ", " Acked [ ] " ); 00469 // "¦ ¦ Datarate [ ] ¦" ); 00470 SerialDisplayDrawLine( " ", " Datarate [ ] " ); 00471 // "¦ ¦ Counter [ ] ¦" ); 00472 SerialDisplayDrawLine( " ", " Counter [ ] " ); 00473 // "¦ ¦ Port [ ] ¦" ); 00474 SerialDisplayDrawLine( " ", " Port [ ] " ); 00475 // "¦ ¦ Data [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ ¦" ); 00476 SerialDisplayDrawLine( " ", " Data [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ " ); 00477 // "¦ ¦ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ ¦" ); 00478 SerialDisplayDrawLine( " ", " __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ " ); 00479 // "¦ ¦ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ ¦" ); 00480 SerialDisplayDrawLine( " ", " __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ " ); 00481 // "¦ ¦ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ ¦" ); 00482 SerialDisplayDrawLine( " ", " __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ " ); 00483 // "+------------+----------------------------------------------------------------¦" ); 00484 SerialDisplayDrawSeparator( ); 00485 // "¦ Downlink ¦ RSSI [ ] dBm ¦" ); 00486 SerialDisplayDrawLine( " Downlink ", " RSSI [ ] dBm " ); 00487 // "¦ [ ]Data ¦ SNR [ ] dB ¦" ); 00488 SerialDisplayDrawLine( " [ ]Data ", " SNR [ ] dB " ); 00489 // "¦ ¦ Counter [ ] ¦" ); 00490 // "¦ ¦ Counter [ ] ¦" ); 00491 SerialDisplayDrawLine( " ", " Counter [ ] " ); 00492 // "¦ ¦ Port [ ] ¦" ); 00493 SerialDisplayDrawLine( " ", " Port [ ] " ); 00494 // "¦ ¦ Data [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ ¦" ); 00495 SerialDisplayDrawLine( " ", " Data [__ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ " ); 00496 // "¦ ¦ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ ¦" ); 00497 SerialDisplayDrawLine( " ", " __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ " ); 00498 // "¦ ¦ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ ¦" ); 00499 SerialDisplayDrawLine( " ", " __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ " ); 00500 // "¦ ¦ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ ¦" ); 00501 SerialDisplayDrawLine( " ", " __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ __ " ); 00502 // "+------------+----------------------------------------------------------------+" ); 00503 SerialDisplayDrawBottomLine( ); 00504 vt.printf( "To refresh screen please hit 'r' key.\r\n" ); 00505 } 00506 00507 bool SerialDisplayReadable( void ) 00508 { 00509 return vt.Readable( ); 00510 } 00511 00512 uint8_t SerialDisplayGetChar( void ) 00513 { 00514 return vt.GetChar( ); 00515 } 00516 00517 #endif /* ENABLE_VT100 */ 00518
Generated on Wed Jul 13 2022 22:22:27 by
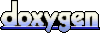