Eddystone test using modified DAL
Dependencies: BLE_API mbed-dev-bin nRF51822
Dependents: microbit-eddystone
Fork of microbit-dal by
MicroBitMultiButton.h
00001 /* 00002 The MIT License (MIT) 00003 00004 Copyright (c) 2016 British Broadcasting Corporation. 00005 This software is provided by Lancaster University by arrangement with the BBC. 00006 00007 Permission is hereby granted, free of charge, to any person obtaining a 00008 copy of this software and associated documentation files (the "Software"), 00009 to deal in the Software without restriction, including without limitation 00010 the rights to use, copy, modify, merge, publish, distribute, sublicense, 00011 and/or sell copies of the Software, and to permit persons to whom the 00012 Software is furnished to do so, subject to the following conditions: 00013 00014 The above copyright notice and this permission notice shall be included in 00015 all copies or substantial portions of the Software. 00016 00017 THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR 00018 IMPLIED, INCLUDING BUT NOT LIMITED TO THE WARRANTIES OF MERCHANTABILITY, 00019 FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL 00020 THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER 00021 LIABILITY, WHETHER IN AN ACTION OF CONTRACT, TORT OR OTHERWISE, ARISING 00022 FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER 00023 DEALINGS IN THE SOFTWARE. 00024 */ 00025 00026 #ifndef MICROBIT_MULTI_BUTTON_H 00027 #define MICROBIT_MULTI_BUTTON_H 00028 00029 #include "mbed.h" 00030 #include "MicroBitConfig.h" 00031 #include "MicroBitButton.h" 00032 #include "EventModel.h" 00033 00034 #define MICROBIT_MULTI_BUTTON_STATE_1 0x01 00035 #define MICROBIT_MULTI_BUTTON_STATE_2 0x02 00036 #define MICROBIT_MULTI_BUTTON_HOLD_TRIGGERED_1 0x04 00037 #define MICROBIT_MULTI_BUTTON_HOLD_TRIGGERED_2 0x08 00038 #define MICROBIT_MULTI_BUTTON_SUPRESSED_1 0X10 00039 #define MICROBIT_MULTI_BUTTON_SUPRESSED_2 0x20 00040 #define MICROBIT_MULTI_BUTTON_ATTACHED 0x40 00041 00042 /** 00043 * Class definition for MicroBitMultiButton. 00044 * 00045 * Represents a virtual button, capable of reacting to simultaneous presses of two 00046 * other buttons. 00047 */ 00048 class MicroBitMultiButton : public MicroBitComponent 00049 { 00050 uint16_t button1; // ID of the first button we're monitoring 00051 uint16_t button2; // ID of the second button we're monitoring 00052 MicroBitButtonEventConfiguration eventConfiguration; // Do we want to generate high level event (clicks), or defer this to another service. 00053 00054 /** 00055 * Retrieves the button id for the alternate button id given. 00056 * 00057 * @param b the id of the button whose state we would like to retrieve. 00058 * 00059 * @return the other sub button id. 00060 */ 00061 uint16_t otherSubButton(uint16_t b); 00062 00063 /** 00064 * Determines if the given button id is marked as pressed. 00065 * 00066 * @param button the id of the button whose state we would like to retrieve. 00067 * 00068 * @return 1 if pressed, 0 if not. 00069 */ 00070 int isSubButtonPressed(uint16_t button); 00071 00072 /** 00073 * Determines if the given button id is marked as held. 00074 * 00075 * @param button the id of the button whose state we would like to retrieve. 00076 * 00077 * @return 1 if held, 0 if not. 00078 */ 00079 int isSubButtonHeld(uint16_t button); 00080 00081 /** 00082 * Determines if the given button id is marked as supressed. 00083 * 00084 * @param button the id of the button whose state we would like to retrieve. 00085 * 00086 * @return 1 if supressed, 0 if not. 00087 */ 00088 int isSubButtonSupressed(uint16_t button); 00089 00090 /** 00091 * Configures the button pressed state for the given button id. 00092 * 00093 * @param button the id of the button whose state requires updating. 00094 * 00095 * @param value the value to set for this buttons state. (Transformed into a logical 0 or 1). 00096 */ 00097 void setButtonState(uint16_t button, int value); 00098 00099 /** 00100 * Configures the button held state for the given button id. 00101 * 00102 * @param button the id of the button whose state requires updating. 00103 * 00104 * @param value the value to set for this buttons state. (Transformed into a logical 0 or 1). 00105 */ 00106 void setHoldState(uint16_t button, int value); 00107 00108 /** 00109 * Configures the button suppressed state for the given button id. 00110 * 00111 * @param button the id of the button whose state requires updating. 00112 * 00113 * @param value the value to set for this buttons state. (Transformed into a logical 0 or 1). 00114 */ 00115 void setSupressedState(uint16_t button, int value); 00116 00117 public: 00118 00119 /** 00120 * Constructor. 00121 * 00122 * Create a representation of a virtual button, that generates events based upon the combination 00123 * of two given buttons. 00124 * 00125 * @param button1 the unique ID of the first button to watch. 00126 * 00127 * @param button2 the unique ID of the second button to watch. 00128 * 00129 * @param id the unique EventModel id of this MicroBitMultiButton instance. 00130 * 00131 * @code 00132 * multiButton(MICROBIT_ID_BUTTON_A, MICROBIT_ID_BUTTON_B, MICROBIT_ID_BUTTON_AB); 00133 * @endcode 00134 */ 00135 MicroBitMultiButton(uint16_t button1, uint16_t button2, uint16_t id); 00136 00137 /** 00138 * Tests if this MicroBitMultiButton instance is virtually pressed. 00139 * 00140 * @return 1 if both physical buttons are pressed simultaneously. 00141 * 00142 * @code 00143 * if(buttonAB.isPressed()) 00144 * display.scroll("Pressed!"); 00145 * @endcode 00146 */ 00147 int isPressed(); 00148 00149 /** 00150 * Changes the event configuration of this button to the given MicroBitButtonEventConfiguration. 00151 * All subsequent events generated by this button will then be informed by this configuration. 00152 * 00153 * @param config The new configuration for this button. Legal values are MICROBIT_BUTTON_ALL_EVENTS or MICROBIT_BUTTON_SIMPLE_EVENTS. 00154 * 00155 * @code 00156 * // Configure a button to generate all possible events. 00157 * buttonAB.setEventConfiguration(MICROBIT_BUTTON_ALL_EVENTS); 00158 * 00159 * // Configure a button to suppress MICROBIT_BUTTON_EVT_CLICK and MICROBIT_BUTTON_EVT_LONG_CLICK events. 00160 * buttonAB.setEventConfiguration(MICROBIT_BUTTON_SIMPLE_EVENTS); 00161 * @endcode 00162 */ 00163 void setEventConfiguration(MicroBitButtonEventConfiguration config); 00164 00165 private: 00166 00167 /** 00168 * A member function that is invoked when any event is detected from the two 00169 * button IDs this MicrobitMultiButton instance was constructed with. 00170 * 00171 * @param evt the event received from the default EventModel. 00172 */ 00173 void onButtonEvent(MicroBitEvent evt); 00174 }; 00175 00176 #endif
Generated on Tue Jul 12 2022 21:07:40 by
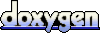