Library for driving the MMA8452 accelerometer over I2C
Dependents: MMA8452_Test MMA8452_Demo Dualing_Tanks IMU-Controlled_MP3_Player ... more
MMA8452 Class Reference
Wrapper for the MMA8452 I2C driven accelerometer. More...
#include <MMA8452.h>
Public Member Functions | |
MMA8452 (PinName sda, PinName scl, int frequency) | |
Create an accelerometer object connected to the specified I2C pins. | |
~MMA8452 () | |
Destructor. | |
int | activate () |
Puts the MMA8452 in active mode. | |
int | standby () |
Puts the MMA8452 in standby. | |
int | getDeviceID (char *dst) |
Read the device ID from the accelerometer (should be 0x2a) | |
int | getStatus (char *dst) |
Read the MMA8452 status register. | |
int | readXYZRaw (char *dst) |
Read the raw x, y, an z registers of the MMA8452 in one operation. | |
int | readXRaw (char *dst) |
Read the raw x register into the provided buffer. | |
int | readYRaw (char *dst) |
Read the raw y register into the provided buffer. | |
int | readZRaw (char *dst) |
Read the raw z register into the provided buffer. | |
int | readXYZCounts (int *x, int *y, int *z) |
Read the x, y, and z signed counts of the MMA8452 axes. | |
int | readXCount (int *x) |
Read the x axes signed count. | |
int | readYCount (int *y) |
Read the y axes signed count. | |
int | readZCount (int *z) |
Read the z axes signed count. | |
int | readXYZGravity (double *x, double *y, double *z) |
Read the x, y, and z accelerations measured in G. | |
int | readXGravity (double *x) |
Read the x gravity in G into the provided double pointer. | |
int | readYGravity (double *y) |
Read the y gravity in G into the provided double pointer. | |
int | readZGravity (double *z) |
Read the z gravity in G into the provided double pointer. | |
int | isXYZReady () |
Returns 1 if data has been internally sampled (is available) for all axes since last read, 0 otherwise. | |
int | isXReady () |
Returns 1 if data has been internally sampled (is available) for the x-axis since last read, 0 otherwise. | |
int | isYReady () |
Returns 1 if data has been internally sampled (is available) for the y-axis since last read, 0 otherwise. | |
int | isZReady () |
Returns 1 if data has been internally sampled (is available) for the z-axis since last read, 0 otherwise. | |
int | readRegister (char addr, char *dst) |
Reads a single byte from the specified MMA8452 register. | |
int | readRegister (char addr, char *dst, int nbytes) |
Reads n bytes from the specified MMA8452 register. | |
int | writeRegister (char addr, char data) |
Write to the specified MMA8452 register. | |
int | writeRegister (char addr, char *data, int nbytes) |
Write a data buffer to the specified MMA8452 register. |
Detailed Description
Wrapper for the MMA8452 I2C driven accelerometer.
Definition at line 114 of file MMA8452.h.
Constructor & Destructor Documentation
MMA8452 | ( | PinName | sda, |
PinName | scl, | ||
int | frequency | ||
) |
Create an accelerometer object connected to the specified I2C pins.
- Parameters:
-
sda I2C data port scl I2C clock port frequency
Definition at line 32 of file MMA8452.cpp.
~MMA8452 | ( | ) |
Destructor.
Definition at line 53 of file MMA8452.cpp.
Member Function Documentation
int activate | ( | ) |
Puts the MMA8452 in active mode.
- Returns:
- 0 on success, 1 on failure.
Definition at line 56 of file MMA8452.cpp.
int getDeviceID | ( | char * | dst ) |
Read the device ID from the accelerometer (should be 0x2a)
- Parameters:
-
dst pointer to store the ID
- Returns:
- 0 on success, 1 on failure.
Definition at line 155 of file MMA8452.cpp.
int getStatus | ( | char * | dst ) |
Read the MMA8452 status register.
- Parameters:
-
dst pointer to store the register value. @ return 0 on success, 1 on failure.
Definition at line 159 of file MMA8452.cpp.
int isXReady | ( | ) |
Returns 1 if data has been internally sampled (is available) for the x-axis since last read, 0 otherwise.
Definition at line 142 of file MMA8452.cpp.
int isXYZReady | ( | ) |
Returns 1 if data has been internally sampled (is available) for all axes since last read, 0 otherwise.
Definition at line 138 of file MMA8452.cpp.
int isYReady | ( | ) |
Returns 1 if data has been internally sampled (is available) for the y-axis since last read, 0 otherwise.
Definition at line 146 of file MMA8452.cpp.
int isZReady | ( | ) |
Returns 1 if data has been internally sampled (is available) for the z-axis since last read, 0 otherwise.
Definition at line 150 of file MMA8452.cpp.
int readRegister | ( | char | addr, |
char * | dst | ||
) |
Reads a single byte from the specified MMA8452 register.
- Parameters:
-
addr The internal register address. dst The destination buffer address.
- Returns:
- 1 on success, 0 on failure.
Definition at line 465 of file MMA8452.cpp.
int readRegister | ( | char | addr, |
char * | dst, | ||
int | nbytes | ||
) |
Reads n bytes from the specified MMA8452 register.
- Parameters:
-
addr The internal register address. dst The destination buffer address. nbytes The number of bytes to read.
- Returns:
- 1 on success, 0 on failure.
Definition at line 447 of file MMA8452.cpp.
int readXCount | ( | int * | x ) |
int readXGravity | ( | double * | x ) |
Read the x gravity in G into the provided double pointer.
- See also:
- readXYZGravity
Definition at line 320 of file MMA8452.cpp.
int readXRaw | ( | char * | dst ) |
Read the raw x register into the provided buffer.
- See also:
- readXYZRaw
Definition at line 196 of file MMA8452.cpp.
int readXYZCounts | ( | int * | x, |
int * | y, | ||
int * | z | ||
) |
Read the x, y, and z signed counts of the MMA8452 axes.
Count resolution is either 8 bits or 12 bits, and the range is either +-2G, +-4G, or +-8G depending on settings. The number of counts per G are 1024, 512, 256 for 2,4, and 8 G respectively at 12 bit resolution and 64, 32, 16 for 2, 4, and 8 G respectively at 8 bit resolution.
This function queries the MMA8452 and returns the signed counts for each axes.
- Parameters:
-
x Pointer to integer to store x count y Pointer to integer to store y count z Pointer to integer to store z count
- Returns:
- 0 on success, 1 on failure.
Definition at line 229 of file MMA8452.cpp.
int readXYZGravity | ( | double * | x, |
double * | y, | ||
double * | z | ||
) |
Read the x, y, and z accelerations measured in G.
The measurement resolution is controlled via setBitDepth which can be 8 or 12, and by setDynamicRange, which can be +-2G, +-4G, or +-8G.
- Parameters:
-
x A pointer to the double to store the x acceleration in. y A pointer to the double to store the y acceleration in. z A pointer to the double to store the z acceleration in.
- Returns:
- 0 on success, 1 on failure.
Definition at line 307 of file MMA8452.cpp.
int readXYZRaw | ( | char * | dst ) |
Read the raw x, y, an z registers of the MMA8452 in one operation.
All three registers are read sequentially and stored in the provided buffer. The stored values are signed 2's complement left-aligned 12 or 8 bit integers.
- Parameters:
-
dst The destination buffer. Note that this needs to be 3 bytes for BIT_DEPTH_8 and 6 bytes for BIT_DEPTH_12. It is upto the caller to ensure this.
- Returns:
- 0 for success, and 1 for failure
- See also:
- setBitDepth
Definition at line 185 of file MMA8452.cpp.
int readYCount | ( | int * | y ) |
int readYGravity | ( | double * | y ) |
Read the y gravity in G into the provided double pointer.
- See also:
- readXYZGravity
Definition at line 331 of file MMA8452.cpp.
int readYRaw | ( | char * | dst ) |
Read the raw y register into the provided buffer.
- See also:
- readXYZRaw
Definition at line 207 of file MMA8452.cpp.
int readZCount | ( | int * | z ) |
int readZGravity | ( | double * | z ) |
Read the z gravity in G into the provided double pointer.
- See also:
- readXYZGravity
Definition at line 342 of file MMA8452.cpp.
int readZRaw | ( | char * | dst ) |
Read the raw z register into the provided buffer.
- See also:
- readXYZRaw
Definition at line 218 of file MMA8452.cpp.
int standby | ( | ) |
Puts the MMA8452 in standby.
- Returns:
- 0 on success, 1 on failure.
Definition at line 62 of file MMA8452.cpp.
int writeRegister | ( | char | addr, |
char | data | ||
) |
Write to the specified MMA8452 register.
- Parameters:
-
addr The internal register address data Data byte to write
Definition at line 391 of file MMA8452.cpp.
int writeRegister | ( | char | addr, |
char * | data, | ||
int | nbytes | ||
) |
Write a data buffer to the specified MMA8452 register.
- Parameters:
-
addr The internal register address data Pointer to data buffer to write nbytes The length of the data buffer to write
Definition at line 421 of file MMA8452.cpp.
Generated on Tue Jul 12 2022 16:12:55 by
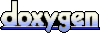