mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
x509_csr.h File Reference
X.509 certificate signing request parsing and writing. More...
Go to the source code of this file.
Data Structures | |
struct | _x509_csr |
Certificate Signing Request (CSR) structure. More... | |
struct | _x509write_csr |
Container for writing a CSR. More... | |
Functions | |
void | x509write_csr_init (x509write_csr *ctx) |
Initialize a CSR context. | |
int | x509write_csr_set_subject_name (x509write_csr *ctx, const char *subject_name) |
Set the subject name for a CSR Subject names should contain a comma-separated list of OID types and values: e.g. | |
void | x509write_csr_set_key (x509write_csr *ctx, pk_context *key) |
Set the key for a CSR (public key will be included, private key used to sign the CSR when writing it) | |
void | x509write_csr_set_md_alg (x509write_csr *ctx, md_type_t md_alg) |
Set the MD algorithm to use for the signature (e.g. | |
int | x509write_csr_set_key_usage (x509write_csr *ctx, unsigned char key_usage) |
Set the Key Usage Extension flags (e.g. | |
int | x509write_csr_set_ns_cert_type (x509write_csr *ctx, unsigned char ns_cert_type) |
Set the Netscape Cert Type flags (e.g. | |
int | x509write_csr_set_extension (x509write_csr *ctx, const char *oid, size_t oid_len, const unsigned char *val, size_t val_len) |
Generic function to add to or replace an extension in the CSR. | |
void | x509write_csr_free (x509write_csr *ctx) |
Free the contents of a CSR context. | |
int | x509write_csr_der (x509write_csr *ctx, unsigned char *buf, size_t size, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng) |
Write a CSR (Certificate Signing Request) to a DER structure Note: data is written at the end of the buffer! Use the return value to determine where you should start using the buffer. | |
int | x509write_csr_pem (x509write_csr *ctx, unsigned char *buf, size_t size, int(*f_rng)(void *, unsigned char *, size_t), void *p_rng) |
Write a CSR (Certificate Signing Request) to a PEM string. | |
Structures and functions for X.509 Certificate Signing Requests (CSR) | |
typedef struct _x509_csr | x509_csr |
Certificate Signing Request (CSR) structure. | |
typedef struct _x509write_csr | x509write_csr |
Container for writing a CSR. | |
int | x509_csr_parse_der (x509_csr *csr, const unsigned char *buf, size_t buflen) |
Load a Certificate Signing Request (CSR) in DER format. | |
int | x509_csr_parse (x509_csr *csr, const unsigned char *buf, size_t buflen) |
Load a Certificate Signing Request (CSR), DER or PEM format. | |
int | x509_csr_parse_file (x509_csr *csr, const char *path) |
Load a Certificate Signing Request (CSR) | |
int | x509_csr_info (char *buf, size_t size, const char *prefix, const x509_csr *csr) |
Returns an informational string about the CSR. | |
void | x509_csr_init (x509_csr *csr) |
Initialize a CSR. | |
void | x509_csr_free (x509_csr *csr) |
Unallocate all CSR data. |
Detailed Description
X.509 certificate signing request parsing and writing.
Copyright (C) 2006-2014, ARM Limited, All Rights Reserved
This file is part of mbed TLS (https://tls.mbed.org)
This program is free software; you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation; either version 2 of the License, or (at your option) any later version.
This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details.
You should have received a copy of the GNU General Public License along with this program; if not, write to the Free Software Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA.
Definition in file x509_csr.h.
Function Documentation
int x509write_csr_der | ( | x509write_csr * | ctx, |
unsigned char * | buf, | ||
size_t | size, | ||
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng | ||
) |
Write a CSR (Certificate Signing Request) to a DER structure Note: data is written at the end of the buffer! Use the return value to determine where you should start using the buffer.
- Parameters:
-
ctx CSR to write away buf buffer to write to size size of the buffer f_rng RNG function (for signature, see note) p_rng RNG parameter
- Returns:
- length of data written if successful, or a specific error code
- Note:
- f_rng may be NULL if RSA is used for signature and the signature is made offline (otherwise f_rng is desirable for countermeasures against timing attacks). ECDSA signatures always require a non-NULL f_rng.
Definition at line 130 of file x509write_csr.c.
void x509write_csr_free | ( | x509write_csr * | ctx ) |
Free the contents of a CSR context.
- Parameters:
-
ctx CSR context to free
Definition at line 57 of file x509write_csr.c.
void x509write_csr_init | ( | x509write_csr * | ctx ) |
Initialize a CSR context.
- Parameters:
-
ctx CSR context to initialize
Definition at line 52 of file x509write_csr.c.
int x509write_csr_pem | ( | x509write_csr * | ctx, |
unsigned char * | buf, | ||
size_t | size, | ||
int(*)(void *, unsigned char *, size_t) | f_rng, | ||
void * | p_rng | ||
) |
Write a CSR (Certificate Signing Request) to a PEM string.
- Parameters:
-
ctx CSR to write away buf buffer to write to size size of the buffer f_rng RNG function (for signature, see note) p_rng RNG parameter
- Returns:
- 0 successful, or a specific error code
- Note:
- f_rng may be NULL if RSA is used for signature and the signature is made offline (otherwise f_rng is desirable for couermeasures against timing attacks). ECDSA signatures always require a non-NULL f_rng.
Definition at line 232 of file x509write_csr.c.
int x509write_csr_set_extension | ( | x509write_csr * | ctx, |
const char * | oid, | ||
size_t | oid_len, | ||
const unsigned char * | val, | ||
size_t | val_len | ||
) |
Generic function to add to or replace an extension in the CSR.
- Parameters:
-
ctx CSR context to use oid OID of the extension oid_len length of the OID val value of the extension OCTET STRING val_len length of the value data
- Returns:
- 0 if successful, or a POLARSSL_ERR_X509WRITE_MALLOC_FAILED
Definition at line 81 of file x509write_csr.c.
void x509write_csr_set_key | ( | x509write_csr * | ctx, |
pk_context * | key | ||
) |
Set the key for a CSR (public key will be included, private key used to sign the CSR when writing it)
- Parameters:
-
ctx CSR context to use key Asymetric key to include
Definition at line 70 of file x509write_csr.c.
int x509write_csr_set_key_usage | ( | x509write_csr * | ctx, |
unsigned char | key_usage | ||
) |
Set the Key Usage Extension flags (e.g.
KU_DIGITAL_SIGNATURE | KU_KEY_CERT_SIGN)
- Parameters:
-
ctx CSR context to use key_usage key usage flags to set
- Returns:
- 0 if successful, or POLARSSL_ERR_X509WRITE_MALLOC_FAILED
Definition at line 89 of file x509write_csr.c.
void x509write_csr_set_md_alg | ( | x509write_csr * | ctx, |
md_type_t | md_alg | ||
) |
Set the MD algorithm to use for the signature (e.g.
POLARSSL_MD_SHA1)
- Parameters:
-
ctx CSR context to use md_alg MD algorithm to use
Definition at line 65 of file x509write_csr.c.
int x509write_csr_set_ns_cert_type | ( | x509write_csr * | ctx, |
unsigned char | ns_cert_type | ||
) |
Set the Netscape Cert Type flags (e.g.
NS_CERT_TYPE_SSL_CLIENT | NS_CERT_TYPE_EMAIL)
- Parameters:
-
ctx CSR context to use ns_cert_type Netscape Cert Type flags to set
- Returns:
- 0 if successful, or POLARSSL_ERR_X509WRITE_MALLOC_FAILED
Definition at line 109 of file x509write_csr.c.
int x509write_csr_set_subject_name | ( | x509write_csr * | ctx, |
const char * | subject_name | ||
) |
Set the subject name for a CSR Subject names should contain a comma-separated list of OID types and values: e.g.
"C=UK,O=ARM,CN=mbed TLS Server 1"
- Parameters:
-
ctx CSR context to use subject_name subject name to set
- Returns:
- 0 if subject name was parsed successfully, or a specific error code
Definition at line 75 of file x509write_csr.c.
Generated on Tue Jul 12 2022 13:50:40 by
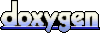