mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
rsa.h
00001 /** 00002 * \file rsa.h 00003 * 00004 * \brief The RSA public-key cryptosystem 00005 * 00006 * Copyright (C) 2006-2014, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 #ifndef POLARSSL_RSA_H 00025 #define POLARSSL_RSA_H 00026 00027 #if !defined(POLARSSL_CONFIG_FILE) 00028 #include "config.h" 00029 #else 00030 #include POLARSSL_CONFIG_FILE 00031 #endif 00032 00033 #include "bignum.h" 00034 #include "md.h" 00035 00036 #if defined(POLARSSL_THREADING_C) 00037 #include "threading.h" 00038 #endif 00039 00040 /* 00041 * RSA Error codes 00042 */ 00043 #define POLARSSL_ERR_RSA_BAD_INPUT_DATA -0x4080 /**< Bad input parameters to function. */ 00044 #define POLARSSL_ERR_RSA_INVALID_PADDING -0x4100 /**< Input data contains invalid padding and is rejected. */ 00045 #define POLARSSL_ERR_RSA_KEY_GEN_FAILED -0x4180 /**< Something failed during generation of a key. */ 00046 #define POLARSSL_ERR_RSA_KEY_CHECK_FAILED -0x4200 /**< Key failed to pass the library's validity check. */ 00047 #define POLARSSL_ERR_RSA_PUBLIC_FAILED -0x4280 /**< The public key operation failed. */ 00048 #define POLARSSL_ERR_RSA_PRIVATE_FAILED -0x4300 /**< The private key operation failed. */ 00049 #define POLARSSL_ERR_RSA_VERIFY_FAILED -0x4380 /**< The PKCS#1 verification failed. */ 00050 #define POLARSSL_ERR_RSA_OUTPUT_TOO_LARGE -0x4400 /**< The output buffer for decryption is not large enough. */ 00051 #define POLARSSL_ERR_RSA_RNG_FAILED -0x4480 /**< The random generator failed to generate non-zeros. */ 00052 00053 /* 00054 * RSA constants 00055 */ 00056 #define RSA_PUBLIC 0 00057 #define RSA_PRIVATE 1 00058 00059 #define RSA_PKCS_V15 0 00060 #define RSA_PKCS_V21 1 00061 00062 #define RSA_SIGN 1 00063 #define RSA_CRYPT 2 00064 00065 #define RSA_SALT_LEN_ANY -1 00066 00067 /* 00068 * The above constants may be used even if the RSA module is compile out, 00069 * eg for alternative (PKCS#11) RSA implemenations in the PK layers. 00070 */ 00071 #if defined(POLARSSL_RSA_C) 00072 00073 #ifdef __cplusplus 00074 extern "C" { 00075 #endif 00076 00077 /** 00078 * \brief RSA context structure 00079 */ 00080 typedef struct 00081 { 00082 int ver ; /*!< always 0 */ 00083 size_t len ; /*!< size(N) in chars */ 00084 00085 mpi N ; /*!< public modulus */ 00086 mpi E ; /*!< public exponent */ 00087 00088 mpi D ; /*!< private exponent */ 00089 mpi P ; /*!< 1st prime factor */ 00090 mpi Q ; /*!< 2nd prime factor */ 00091 mpi DP ; /*!< D % (P - 1) */ 00092 mpi DQ ; /*!< D % (Q - 1) */ 00093 mpi QP ; /*!< 1 / (Q % P) */ 00094 00095 mpi RN ; /*!< cached R^2 mod N */ 00096 mpi RP ; /*!< cached R^2 mod P */ 00097 mpi RQ ; /*!< cached R^2 mod Q */ 00098 00099 mpi Vi ; /*!< cached blinding value */ 00100 mpi Vf ; /*!< cached un-blinding value */ 00101 00102 int padding; /*!< RSA_PKCS_V15 for 1.5 padding and 00103 RSA_PKCS_v21 for OAEP/PSS */ 00104 int hash_id; /*!< Hash identifier of md_type_t as 00105 specified in the md.h header file 00106 for the EME-OAEP and EMSA-PSS 00107 encoding */ 00108 #if defined(POLARSSL_THREADING_C) 00109 threading_mutex_t mutex ; /*!< Thread-safety mutex */ 00110 #endif 00111 } 00112 rsa_context; 00113 00114 /** 00115 * \brief Initialize an RSA context 00116 * 00117 * Note: Set padding to RSA_PKCS_V21 for the RSAES-OAEP 00118 * encryption scheme and the RSASSA-PSS signature scheme. 00119 * 00120 * \param ctx RSA context to be initialized 00121 * \param padding RSA_PKCS_V15 or RSA_PKCS_V21 00122 * \param hash_id RSA_PKCS_V21 hash identifier 00123 * 00124 * \note The hash_id parameter is actually ignored 00125 * when using RSA_PKCS_V15 padding. 00126 * 00127 * \note Choice of padding mode is strictly enforced for private key 00128 * operations, since there might be security concerns in 00129 * mixing padding modes. For public key operations it's merely 00130 * a default value, which can be overriden by calling specific 00131 * rsa_rsaes_xxx or rsa_rsassa_xxx functions. 00132 * 00133 * \note The chosen hash is always used for OEAP encryption. 00134 * For PSS signatures, it's always used for making signatures, 00135 * but can be overriden (and always is, if set to 00136 * POLARSSL_MD_NONE) for verifying them. 00137 */ 00138 void rsa_init( rsa_context *ctx, 00139 int padding, 00140 int hash_id); 00141 00142 /** 00143 * \brief Set padding for an already initialized RSA context 00144 * See \c rsa_init() for details. 00145 * 00146 * \param ctx RSA context to be set 00147 * \param padding RSA_PKCS_V15 or RSA_PKCS_V21 00148 * \param hash_id RSA_PKCS_V21 hash identifier 00149 */ 00150 void rsa_set_padding( rsa_context *ctx, int padding, int hash_id); 00151 00152 /** 00153 * \brief Generate an RSA keypair 00154 * 00155 * \param ctx RSA context that will hold the key 00156 * \param f_rng RNG function 00157 * \param p_rng RNG parameter 00158 * \param nbits size of the public key in bits 00159 * \param exponent public exponent (e.g., 65537) 00160 * 00161 * \note rsa_init() must be called beforehand to setup 00162 * the RSA context. 00163 * 00164 * \return 0 if successful, or an POLARSSL_ERR_RSA_XXX error code 00165 */ 00166 int rsa_gen_key( rsa_context *ctx, 00167 int (*f_rng)(void *, unsigned char *, size_t), 00168 void *p_rng, 00169 unsigned int nbits, int exponent ); 00170 00171 /** 00172 * \brief Check a public RSA key 00173 * 00174 * \param ctx RSA context to be checked 00175 * 00176 * \return 0 if successful, or an POLARSSL_ERR_RSA_XXX error code 00177 */ 00178 int rsa_check_pubkey( const rsa_context *ctx ); 00179 00180 /** 00181 * \brief Check a private RSA key 00182 * 00183 * \param ctx RSA context to be checked 00184 * 00185 * \return 0 if successful, or an POLARSSL_ERR_RSA_XXX error code 00186 */ 00187 int rsa_check_privkey( const rsa_context *ctx ); 00188 00189 /** 00190 * \brief Check a public-private RSA key pair. 00191 * Check each of the contexts, and make sure they match. 00192 * 00193 * \param pub RSA context holding the public key 00194 * \param prv RSA context holding the private key 00195 * 00196 * \return 0 if successful, or an POLARSSL_ERR_RSA_XXX error code 00197 */ 00198 int rsa_check_pub_priv( const rsa_context *pub, const rsa_context *prv ); 00199 00200 /** 00201 * \brief Do an RSA public key operation 00202 * 00203 * \param ctx RSA context 00204 * \param input input buffer 00205 * \param output output buffer 00206 * 00207 * \return 0 if successful, or an POLARSSL_ERR_RSA_XXX error code 00208 * 00209 * \note This function does NOT take care of message 00210 * padding. Also, be sure to set input[0] = 0 or assure that 00211 * input is smaller than N. 00212 * 00213 * \note The input and output buffers must be large 00214 * enough (eg. 128 bytes if RSA-1024 is used). 00215 */ 00216 int rsa_public( rsa_context *ctx, 00217 const unsigned char *input, 00218 unsigned char *output ); 00219 00220 /** 00221 * \brief Do an RSA private key operation 00222 * 00223 * \param ctx RSA context 00224 * \param f_rng RNG function (Needed for blinding) 00225 * \param p_rng RNG parameter 00226 * \param input input buffer 00227 * \param output output buffer 00228 * 00229 * \return 0 if successful, or an POLARSSL_ERR_RSA_XXX error code 00230 * 00231 * \note The input and output buffers must be large 00232 * enough (eg. 128 bytes if RSA-1024 is used). 00233 */ 00234 int rsa_private( rsa_context *ctx, 00235 int (*f_rng)(void *, unsigned char *, size_t), 00236 void *p_rng, 00237 const unsigned char *input, 00238 unsigned char *output ); 00239 00240 /** 00241 * \brief Generic wrapper to perform a PKCS#1 encryption using the 00242 * mode from the context. Add the message padding, then do an 00243 * RSA operation. 00244 * 00245 * \param ctx RSA context 00246 * \param f_rng RNG function (Needed for padding and PKCS#1 v2.1 encoding 00247 * and RSA_PRIVATE) 00248 * \param p_rng RNG parameter 00249 * \param mode RSA_PUBLIC or RSA_PRIVATE 00250 * \param ilen contains the plaintext length 00251 * \param input buffer holding the data to be encrypted 00252 * \param output buffer that will hold the ciphertext 00253 * 00254 * \return 0 if successful, or an POLARSSL_ERR_RSA_XXX error code 00255 * 00256 * \note The output buffer must be as large as the size 00257 * of ctx->N (eg. 128 bytes if RSA-1024 is used). 00258 */ 00259 int rsa_pkcs1_encrypt( rsa_context *ctx, 00260 int (*f_rng)(void *, unsigned char *, size_t), 00261 void *p_rng, 00262 int mode, size_t ilen, 00263 const unsigned char *input, 00264 unsigned char *output ); 00265 00266 /** 00267 * \brief Perform a PKCS#1 v1.5 encryption (RSAES-PKCS1-v1_5-ENCRYPT) 00268 * 00269 * \param ctx RSA context 00270 * \param f_rng RNG function (Needed for padding and RSA_PRIVATE) 00271 * \param p_rng RNG parameter 00272 * \param mode RSA_PUBLIC or RSA_PRIVATE 00273 * \param ilen contains the plaintext length 00274 * \param input buffer holding the data to be encrypted 00275 * \param output buffer that will hold the ciphertext 00276 * 00277 * \return 0 if successful, or an POLARSSL_ERR_RSA_XXX error code 00278 * 00279 * \note The output buffer must be as large as the size 00280 * of ctx->N (eg. 128 bytes if RSA-1024 is used). 00281 */ 00282 int rsa_rsaes_pkcs1_v15_encrypt( rsa_context *ctx, 00283 int (*f_rng)(void *, unsigned char *, size_t), 00284 void *p_rng, 00285 int mode, size_t ilen, 00286 const unsigned char *input, 00287 unsigned char *output ); 00288 00289 /** 00290 * \brief Perform a PKCS#1 v2.1 OAEP encryption (RSAES-OAEP-ENCRYPT) 00291 * 00292 * \param ctx RSA context 00293 * \param f_rng RNG function (Needed for padding and PKCS#1 v2.1 encoding 00294 * and RSA_PRIVATE) 00295 * \param p_rng RNG parameter 00296 * \param mode RSA_PUBLIC or RSA_PRIVATE 00297 * \param label buffer holding the custom label to use 00298 * \param label_len contains the label length 00299 * \param ilen contains the plaintext length 00300 * \param input buffer holding the data to be encrypted 00301 * \param output buffer that will hold the ciphertext 00302 * 00303 * \return 0 if successful, or an POLARSSL_ERR_RSA_XXX error code 00304 * 00305 * \note The output buffer must be as large as the size 00306 * of ctx->N (eg. 128 bytes if RSA-1024 is used). 00307 */ 00308 int rsa_rsaes_oaep_encrypt( rsa_context *ctx, 00309 int (*f_rng)(void *, unsigned char *, size_t), 00310 void *p_rng, 00311 int mode, 00312 const unsigned char *label, size_t label_len, 00313 size_t ilen, 00314 const unsigned char *input, 00315 unsigned char *output ); 00316 00317 /** 00318 * \brief Generic wrapper to perform a PKCS#1 decryption using the 00319 * mode from the context. Do an RSA operation, then remove 00320 * the message padding 00321 * 00322 * \param ctx RSA context 00323 * \param f_rng RNG function (Only needed for RSA_PRIVATE) 00324 * \param p_rng RNG parameter 00325 * \param mode RSA_PUBLIC or RSA_PRIVATE 00326 * \param olen will contain the plaintext length 00327 * \param input buffer holding the encrypted data 00328 * \param output buffer that will hold the plaintext 00329 * \param output_max_len maximum length of the output buffer 00330 * 00331 * \return 0 if successful, or an POLARSSL_ERR_RSA_XXX error code 00332 * 00333 * \note The output buffer must be as large as the size 00334 * of ctx->N (eg. 128 bytes if RSA-1024 is used) otherwise 00335 * an error is thrown. 00336 */ 00337 int rsa_pkcs1_decrypt( rsa_context *ctx, 00338 int (*f_rng)(void *, unsigned char *, size_t), 00339 void *p_rng, 00340 int mode, size_t *olen, 00341 const unsigned char *input, 00342 unsigned char *output, 00343 size_t output_max_len ); 00344 00345 /** 00346 * \brief Perform a PKCS#1 v1.5 decryption (RSAES-PKCS1-v1_5-DECRYPT) 00347 * 00348 * \param ctx RSA context 00349 * \param f_rng RNG function (Only needed for RSA_PRIVATE) 00350 * \param p_rng RNG parameter 00351 * \param mode RSA_PUBLIC or RSA_PRIVATE 00352 * \param olen will contain the plaintext length 00353 * \param input buffer holding the encrypted data 00354 * \param output buffer that will hold the plaintext 00355 * \param output_max_len maximum length of the output buffer 00356 * 00357 * \return 0 if successful, or an POLARSSL_ERR_RSA_XXX error code 00358 * 00359 * \note The output buffer must be as large as the size 00360 * of ctx->N (eg. 128 bytes if RSA-1024 is used) otherwise 00361 * an error is thrown. 00362 */ 00363 int rsa_rsaes_pkcs1_v15_decrypt( rsa_context *ctx, 00364 int (*f_rng)(void *, unsigned char *, size_t), 00365 void *p_rng, 00366 int mode, size_t *olen, 00367 const unsigned char *input, 00368 unsigned char *output, 00369 size_t output_max_len ); 00370 00371 /** 00372 * \brief Perform a PKCS#1 v2.1 OAEP decryption (RSAES-OAEP-DECRYPT) 00373 * 00374 * \param ctx RSA context 00375 * \param f_rng RNG function (Only needed for RSA_PRIVATE) 00376 * \param p_rng RNG parameter 00377 * \param mode RSA_PUBLIC or RSA_PRIVATE 00378 * \param label buffer holding the custom label to use 00379 * \param label_len contains the label length 00380 * \param olen will contain the plaintext length 00381 * \param input buffer holding the encrypted data 00382 * \param output buffer that will hold the plaintext 00383 * \param output_max_len maximum length of the output buffer 00384 * 00385 * \return 0 if successful, or an POLARSSL_ERR_RSA_XXX error code 00386 * 00387 * \note The output buffer must be as large as the size 00388 * of ctx->N (eg. 128 bytes if RSA-1024 is used) otherwise 00389 * an error is thrown. 00390 */ 00391 int rsa_rsaes_oaep_decrypt( rsa_context *ctx, 00392 int (*f_rng)(void *, unsigned char *, size_t), 00393 void *p_rng, 00394 int mode, 00395 const unsigned char *label, size_t label_len, 00396 size_t *olen, 00397 const unsigned char *input, 00398 unsigned char *output, 00399 size_t output_max_len ); 00400 00401 /** 00402 * \brief Generic wrapper to perform a PKCS#1 signature using the 00403 * mode from the context. Do a private RSA operation to sign 00404 * a message digest 00405 * 00406 * \param ctx RSA context 00407 * \param f_rng RNG function (Needed for PKCS#1 v2.1 encoding and for 00408 * RSA_PRIVATE) 00409 * \param p_rng RNG parameter 00410 * \param mode RSA_PUBLIC or RSA_PRIVATE 00411 * \param md_alg a POLARSSL_MD_* (use POLARSSL_MD_NONE for signing raw data) 00412 * \param hashlen message digest length (for POLARSSL_MD_NONE only) 00413 * \param hash buffer holding the message digest 00414 * \param sig buffer that will hold the ciphertext 00415 * 00416 * \return 0 if the signing operation was successful, 00417 * or an POLARSSL_ERR_RSA_XXX error code 00418 * 00419 * \note The "sig" buffer must be as large as the size 00420 * of ctx->N (eg. 128 bytes if RSA-1024 is used). 00421 * 00422 * \note In case of PKCS#1 v2.1 encoding, see comments on 00423 * \note \c rsa_rsassa_pss_sign() for details on md_alg and hash_id. 00424 */ 00425 int rsa_pkcs1_sign( rsa_context *ctx, 00426 int (*f_rng)(void *, unsigned char *, size_t), 00427 void *p_rng, 00428 int mode, 00429 md_type_t md_alg, 00430 unsigned int hashlen, 00431 const unsigned char *hash, 00432 unsigned char *sig ); 00433 00434 /** 00435 * \brief Perform a PKCS#1 v1.5 signature (RSASSA-PKCS1-v1_5-SIGN) 00436 * 00437 * \param ctx RSA context 00438 * \param f_rng RNG function (Only needed for RSA_PRIVATE) 00439 * \param p_rng RNG parameter 00440 * \param mode RSA_PUBLIC or RSA_PRIVATE 00441 * \param md_alg a POLARSSL_MD_* (use POLARSSL_MD_NONE for signing raw data) 00442 * \param hashlen message digest length (for POLARSSL_MD_NONE only) 00443 * \param hash buffer holding the message digest 00444 * \param sig buffer that will hold the ciphertext 00445 * 00446 * \return 0 if the signing operation was successful, 00447 * or an POLARSSL_ERR_RSA_XXX error code 00448 * 00449 * \note The "sig" buffer must be as large as the size 00450 * of ctx->N (eg. 128 bytes if RSA-1024 is used). 00451 */ 00452 int rsa_rsassa_pkcs1_v15_sign( rsa_context *ctx, 00453 int (*f_rng)(void *, unsigned char *, size_t), 00454 void *p_rng, 00455 int mode, 00456 md_type_t md_alg, 00457 unsigned int hashlen, 00458 const unsigned char *hash, 00459 unsigned char *sig ); 00460 00461 /** 00462 * \brief Perform a PKCS#1 v2.1 PSS signature (RSASSA-PSS-SIGN) 00463 * 00464 * \param ctx RSA context 00465 * \param f_rng RNG function (Needed for PKCS#1 v2.1 encoding and for 00466 * RSA_PRIVATE) 00467 * \param p_rng RNG parameter 00468 * \param mode RSA_PUBLIC or RSA_PRIVATE 00469 * \param md_alg a POLARSSL_MD_* (use POLARSSL_MD_NONE for signing raw data) 00470 * \param hashlen message digest length (for POLARSSL_MD_NONE only) 00471 * \param hash buffer holding the message digest 00472 * \param sig buffer that will hold the ciphertext 00473 * 00474 * \return 0 if the signing operation was successful, 00475 * or an POLARSSL_ERR_RSA_XXX error code 00476 * 00477 * \note The "sig" buffer must be as large as the size 00478 * of ctx->N (eg. 128 bytes if RSA-1024 is used). 00479 * 00480 * \note The hash_id in the RSA context is the one used for the 00481 * encoding. md_alg in the function call is the type of hash 00482 * that is encoded. According to RFC 3447 it is advised to 00483 * keep both hashes the same. 00484 */ 00485 int rsa_rsassa_pss_sign( rsa_context *ctx, 00486 int (*f_rng)(void *, unsigned char *, size_t), 00487 void *p_rng, 00488 int mode, 00489 md_type_t md_alg, 00490 unsigned int hashlen, 00491 const unsigned char *hash, 00492 unsigned char *sig ); 00493 00494 /** 00495 * \brief Generic wrapper to perform a PKCS#1 verification using the 00496 * mode from the context. Do a public RSA operation and check 00497 * the message digest 00498 * 00499 * \param ctx points to an RSA public key 00500 * \param f_rng RNG function (Only needed for RSA_PRIVATE) 00501 * \param p_rng RNG parameter 00502 * \param mode RSA_PUBLIC or RSA_PRIVATE 00503 * \param md_alg a POLARSSL_MD_* (use POLARSSL_MD_NONE for signing raw data) 00504 * \param hashlen message digest length (for POLARSSL_MD_NONE only) 00505 * \param hash buffer holding the message digest 00506 * \param sig buffer holding the ciphertext 00507 * 00508 * \return 0 if the verify operation was successful, 00509 * or an POLARSSL_ERR_RSA_XXX error code 00510 * 00511 * \note The "sig" buffer must be as large as the size 00512 * of ctx->N (eg. 128 bytes if RSA-1024 is used). 00513 * 00514 * \note In case of PKCS#1 v2.1 encoding, see comments on 00515 * \c rsa_rsassa_pss_verify() about md_alg and hash_id. 00516 */ 00517 int rsa_pkcs1_verify( rsa_context *ctx, 00518 int (*f_rng)(void *, unsigned char *, size_t), 00519 void *p_rng, 00520 int mode, 00521 md_type_t md_alg, 00522 unsigned int hashlen, 00523 const unsigned char *hash, 00524 const unsigned char *sig ); 00525 00526 /** 00527 * \brief Perform a PKCS#1 v1.5 verification (RSASSA-PKCS1-v1_5-VERIFY) 00528 * 00529 * \param ctx points to an RSA public key 00530 * \param f_rng RNG function (Only needed for RSA_PRIVATE) 00531 * \param p_rng RNG parameter 00532 * \param mode RSA_PUBLIC or RSA_PRIVATE 00533 * \param md_alg a POLARSSL_MD_* (use POLARSSL_MD_NONE for signing raw data) 00534 * \param hashlen message digest length (for POLARSSL_MD_NONE only) 00535 * \param hash buffer holding the message digest 00536 * \param sig buffer holding the ciphertext 00537 * 00538 * \return 0 if the verify operation was successful, 00539 * or an POLARSSL_ERR_RSA_XXX error code 00540 * 00541 * \note The "sig" buffer must be as large as the size 00542 * of ctx->N (eg. 128 bytes if RSA-1024 is used). 00543 */ 00544 int rsa_rsassa_pkcs1_v15_verify( rsa_context *ctx, 00545 int (*f_rng)(void *, unsigned char *, size_t), 00546 void *p_rng, 00547 int mode, 00548 md_type_t md_alg, 00549 unsigned int hashlen, 00550 const unsigned char *hash, 00551 const unsigned char *sig ); 00552 00553 /** 00554 * \brief Perform a PKCS#1 v2.1 PSS verification (RSASSA-PSS-VERIFY) 00555 * (This is the "simple" version.) 00556 * 00557 * \param ctx points to an RSA public key 00558 * \param f_rng RNG function (Only needed for RSA_PRIVATE) 00559 * \param p_rng RNG parameter 00560 * \param mode RSA_PUBLIC or RSA_PRIVATE 00561 * \param md_alg a POLARSSL_MD_* (use POLARSSL_MD_NONE for signing raw data) 00562 * \param hashlen message digest length (for POLARSSL_MD_NONE only) 00563 * \param hash buffer holding the message digest 00564 * \param sig buffer holding the ciphertext 00565 * 00566 * \return 0 if the verify operation was successful, 00567 * or an POLARSSL_ERR_RSA_XXX error code 00568 * 00569 * \note The "sig" buffer must be as large as the size 00570 * of ctx->N (eg. 128 bytes if RSA-1024 is used). 00571 * 00572 * \note The hash_id in the RSA context is the one used for the 00573 * verification. md_alg in the function call is the type of 00574 * hash that is verified. According to RFC 3447 it is advised to 00575 * keep both hashes the same. If hash_id in the RSA context is 00576 * unset, the md_alg from the function call is used. 00577 */ 00578 int rsa_rsassa_pss_verify( rsa_context *ctx, 00579 int (*f_rng)(void *, unsigned char *, size_t), 00580 void *p_rng, 00581 int mode, 00582 md_type_t md_alg, 00583 unsigned int hashlen, 00584 const unsigned char *hash, 00585 const unsigned char *sig ); 00586 00587 /** 00588 * \brief Perform a PKCS#1 v2.1 PSS verification (RSASSA-PSS-VERIFY) 00589 * (This is the version with "full" options.) 00590 * 00591 * \param ctx points to an RSA public key 00592 * \param f_rng RNG function (Only needed for RSA_PRIVATE) 00593 * \param p_rng RNG parameter 00594 * \param mode RSA_PUBLIC or RSA_PRIVATE 00595 * \param md_alg a POLARSSL_MD_* (use POLARSSL_MD_NONE for signing raw data) 00596 * \param hashlen message digest length (for POLARSSL_MD_NONE only) 00597 * \param hash buffer holding the message digest 00598 * \param mgf1_hash_id message digest used for mask generation 00599 * \param expected_salt_len Length of the salt used in padding, use 00600 * RSA_SALT_LEN_ANY to accept any salt length 00601 * \param sig buffer holding the ciphertext 00602 * 00603 * \return 0 if the verify operation was successful, 00604 * or an POLARSSL_ERR_RSA_XXX error code 00605 * 00606 * \note The "sig" buffer must be as large as the size 00607 * of ctx->N (eg. 128 bytes if RSA-1024 is used). 00608 * 00609 * \note The hash_id in the RSA context is ignored. 00610 */ 00611 int rsa_rsassa_pss_verify_ext( rsa_context *ctx, 00612 int (*f_rng)(void *, unsigned char *, size_t), 00613 void *p_rng, 00614 int mode, 00615 md_type_t md_alg, 00616 unsigned int hashlen, 00617 const unsigned char *hash, 00618 md_type_t mgf1_hash_id, 00619 int expected_salt_len, 00620 const unsigned char *sig ); 00621 00622 /** 00623 * \brief Copy the components of an RSA context 00624 * 00625 * \param dst Destination context 00626 * \param src Source context 00627 * 00628 * \return O on success, 00629 * POLARSSL_ERR_MPI_MALLOC_FAILED on memory allocation failure 00630 */ 00631 int rsa_copy( rsa_context *dst, const rsa_context *src ); 00632 00633 /** 00634 * \brief Free the components of an RSA key 00635 * 00636 * \param ctx RSA Context to free 00637 */ 00638 void rsa_free( rsa_context *ctx ); 00639 00640 /** 00641 * \brief Checkup routine 00642 * 00643 * \return 0 if successful, or 1 if the test failed 00644 */ 00645 int rsa_self_test( int verbose ); 00646 00647 #ifdef __cplusplus 00648 } 00649 #endif 00650 00651 #endif /* POLARSSL_RSA_C */ 00652 00653 #endif /* rsa.h */ 00654
Generated on Tue Jul 12 2022 13:50:38 by
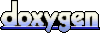