mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
pk_wrap.c
00001 /* 00002 * Public Key abstraction layer: wrapper functions 00003 * 00004 * Copyright (C) 2006-2014, ARM Limited, All Rights Reserved 00005 * 00006 * This file is part of mbed TLS (https://tls.mbed.org) 00007 * 00008 * This program is free software; you can redistribute it and/or modify 00009 * it under the terms of the GNU General Public License as published by 00010 * the Free Software Foundation; either version 2 of the License, or 00011 * (at your option) any later version. 00012 * 00013 * This program is distributed in the hope that it will be useful, 00014 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00015 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00016 * GNU General Public License for more details. 00017 * 00018 * You should have received a copy of the GNU General Public License along 00019 * with this program; if not, write to the Free Software Foundation, Inc., 00020 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00021 */ 00022 00023 #if !defined(POLARSSL_CONFIG_FILE) 00024 #include "polarssl/config.h" 00025 #else 00026 #include POLARSSL_CONFIG_FILE 00027 #endif 00028 00029 #if defined(POLARSSL_PK_C) 00030 #include "polarssl/pk_wrap.h" 00031 00032 /* Even if RSA not activated, for the sake of RSA-alt */ 00033 #include "polarssl/rsa.h" 00034 00035 #include <string.h> 00036 00037 #if defined(POLARSSL_ECP_C) 00038 #include "polarssl/ecp.h" 00039 #endif 00040 00041 #if defined(POLARSSL_ECDSA_C) 00042 #include "polarssl/ecdsa.h" 00043 #endif 00044 00045 #if defined(POLARSSL_PLATFORM_C) 00046 #include "polarssl/platform.h" 00047 #else 00048 #include <stdlib.h> 00049 #define polarssl_malloc malloc 00050 #define polarssl_free free 00051 #endif 00052 00053 /* Implementation that should never be optimized out by the compiler */ 00054 static void polarssl_zeroize( void *v, size_t n ) { 00055 volatile unsigned char *p = v; while( n-- ) *p++ = 0; 00056 } 00057 00058 #if defined(POLARSSL_RSA_C) 00059 static int rsa_can_do( pk_type_t type ) 00060 { 00061 return( type == POLARSSL_PK_RSA || 00062 type == POLARSSL_PK_RSASSA_PSS ); 00063 } 00064 00065 static size_t rsa_get_size( const void *ctx ) 00066 { 00067 return( 8 * ((const rsa_context *) ctx)->len ); 00068 } 00069 00070 static int rsa_verify_wrap( void *ctx, md_type_t md_alg, 00071 const unsigned char *hash, size_t hash_len, 00072 const unsigned char *sig, size_t sig_len ) 00073 { 00074 int ret; 00075 00076 if( sig_len < ((rsa_context *) ctx)->len ) 00077 return( POLARSSL_ERR_RSA_VERIFY_FAILED ); 00078 00079 if( ( ret = rsa_pkcs1_verify( (rsa_context *) ctx, NULL, NULL, 00080 RSA_PUBLIC, md_alg, 00081 (unsigned int) hash_len, hash, sig ) ) != 0 ) 00082 return( ret ); 00083 00084 if( sig_len > ((rsa_context *) ctx)->len ) 00085 return( POLARSSL_ERR_PK_SIG_LEN_MISMATCH ); 00086 00087 return( 0 ); 00088 } 00089 00090 static int rsa_sign_wrap( void *ctx, md_type_t md_alg, 00091 const unsigned char *hash, size_t hash_len, 00092 unsigned char *sig, size_t *sig_len, 00093 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ) 00094 { 00095 *sig_len = ((rsa_context *) ctx)->len; 00096 00097 return( rsa_pkcs1_sign( (rsa_context *) ctx, f_rng, p_rng, RSA_PRIVATE, 00098 md_alg, (unsigned int) hash_len, hash, sig ) ); 00099 } 00100 00101 static int rsa_decrypt_wrap( void *ctx, 00102 const unsigned char *input, size_t ilen, 00103 unsigned char *output, size_t *olen, size_t osize, 00104 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ) 00105 { 00106 if( ilen != ((rsa_context *) ctx)->len ) 00107 return( POLARSSL_ERR_RSA_BAD_INPUT_DATA ); 00108 00109 return( rsa_pkcs1_decrypt( (rsa_context *) ctx, f_rng, p_rng, 00110 RSA_PRIVATE, olen, input, output, osize ) ); 00111 } 00112 00113 static int rsa_encrypt_wrap( void *ctx, 00114 const unsigned char *input, size_t ilen, 00115 unsigned char *output, size_t *olen, size_t osize, 00116 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ) 00117 { 00118 *olen = ((rsa_context *) ctx)->len; 00119 00120 if( *olen > osize ) 00121 return( POLARSSL_ERR_RSA_OUTPUT_TOO_LARGE ); 00122 00123 return( rsa_pkcs1_encrypt( (rsa_context *) ctx, 00124 f_rng, p_rng, RSA_PUBLIC, ilen, input, output ) ); 00125 } 00126 00127 static int rsa_check_pair_wrap( const void *pub, const void *prv ) 00128 { 00129 return( rsa_check_pub_priv( (const rsa_context *) pub, 00130 (const rsa_context *) prv ) ); 00131 } 00132 00133 static void *rsa_alloc_wrap( void ) 00134 { 00135 void *ctx = polarssl_malloc( sizeof( rsa_context ) ); 00136 00137 if( ctx != NULL ) 00138 rsa_init( (rsa_context *) ctx, 0, 0 ); 00139 00140 return( ctx ); 00141 } 00142 00143 static void rsa_free_wrap( void *ctx ) 00144 { 00145 rsa_free( (rsa_context *) ctx ); 00146 polarssl_free( ctx ); 00147 } 00148 00149 static void rsa_debug( const void *ctx, pk_debug_item *items ) 00150 { 00151 items->type = POLARSSL_PK_DEBUG_MPI; 00152 items->name = "rsa.N"; 00153 items->value = &( ((rsa_context *) ctx)->N ); 00154 00155 items++; 00156 00157 items->type = POLARSSL_PK_DEBUG_MPI; 00158 items->name = "rsa.E"; 00159 items->value = &( ((rsa_context *) ctx)->E ); 00160 } 00161 00162 const pk_info_t rsa_info = { 00163 POLARSSL_PK_RSA, 00164 "RSA", 00165 rsa_get_size, 00166 rsa_can_do, 00167 rsa_verify_wrap, 00168 rsa_sign_wrap, 00169 rsa_decrypt_wrap, 00170 rsa_encrypt_wrap, 00171 rsa_check_pair_wrap, 00172 rsa_alloc_wrap, 00173 rsa_free_wrap, 00174 rsa_debug, 00175 }; 00176 #endif /* POLARSSL_RSA_C */ 00177 00178 #if defined(POLARSSL_ECP_C) 00179 /* 00180 * Generic EC key 00181 */ 00182 static int eckey_can_do( pk_type_t type ) 00183 { 00184 return( type == POLARSSL_PK_ECKEY || 00185 type == POLARSSL_PK_ECKEY_DH || 00186 type == POLARSSL_PK_ECDSA ); 00187 } 00188 00189 static size_t eckey_get_size( const void *ctx ) 00190 { 00191 return( ((ecp_keypair *) ctx)->grp.pbits ); 00192 } 00193 00194 #if defined(POLARSSL_ECDSA_C) 00195 /* Forward declarations */ 00196 static int ecdsa_verify_wrap( void *ctx, md_type_t md_alg, 00197 const unsigned char *hash, size_t hash_len, 00198 const unsigned char *sig, size_t sig_len ); 00199 00200 static int ecdsa_sign_wrap( void *ctx, md_type_t md_alg, 00201 const unsigned char *hash, size_t hash_len, 00202 unsigned char *sig, size_t *sig_len, 00203 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ); 00204 00205 static int eckey_verify_wrap( void *ctx, md_type_t md_alg, 00206 const unsigned char *hash, size_t hash_len, 00207 const unsigned char *sig, size_t sig_len ) 00208 { 00209 int ret; 00210 ecdsa_context ecdsa; 00211 00212 ecdsa_init( &ecdsa ); 00213 00214 if( ( ret = ecdsa_from_keypair( &ecdsa, ctx ) ) == 0 ) 00215 ret = ecdsa_verify_wrap( &ecdsa, md_alg, hash, hash_len, sig, sig_len ); 00216 00217 ecdsa_free( &ecdsa ); 00218 00219 return( ret ); 00220 } 00221 00222 static int eckey_sign_wrap( void *ctx, md_type_t md_alg, 00223 const unsigned char *hash, size_t hash_len, 00224 unsigned char *sig, size_t *sig_len, 00225 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ) 00226 { 00227 int ret; 00228 ecdsa_context ecdsa; 00229 00230 ecdsa_init( &ecdsa ); 00231 00232 if( ( ret = ecdsa_from_keypair( &ecdsa, ctx ) ) == 0 ) 00233 ret = ecdsa_sign_wrap( &ecdsa, md_alg, hash, hash_len, sig, sig_len, 00234 f_rng, p_rng ); 00235 00236 ecdsa_free( &ecdsa ); 00237 00238 return( ret ); 00239 } 00240 00241 #endif /* POLARSSL_ECDSA_C */ 00242 00243 static int eckey_check_pair( const void *pub, const void *prv ) 00244 { 00245 return( ecp_check_pub_priv( (const ecp_keypair *) pub, 00246 (const ecp_keypair *) prv ) ); 00247 } 00248 00249 static void *eckey_alloc_wrap( void ) 00250 { 00251 void *ctx = polarssl_malloc( sizeof( ecp_keypair ) ); 00252 00253 if( ctx != NULL ) 00254 ecp_keypair_init( ctx ); 00255 00256 return( ctx ); 00257 } 00258 00259 static void eckey_free_wrap( void *ctx ) 00260 { 00261 ecp_keypair_free( (ecp_keypair *) ctx ); 00262 polarssl_free( ctx ); 00263 } 00264 00265 static void eckey_debug( const void *ctx, pk_debug_item *items ) 00266 { 00267 items->type = POLARSSL_PK_DEBUG_ECP; 00268 items->name = "eckey.Q"; 00269 items->value = &( ((ecp_keypair *) ctx)->Q ); 00270 } 00271 00272 const pk_info_t eckey_info = { 00273 POLARSSL_PK_ECKEY, 00274 "EC", 00275 eckey_get_size, 00276 eckey_can_do, 00277 #if defined(POLARSSL_ECDSA_C) 00278 eckey_verify_wrap, 00279 eckey_sign_wrap, 00280 #else 00281 NULL, 00282 NULL, 00283 #endif 00284 NULL, 00285 NULL, 00286 eckey_check_pair, 00287 eckey_alloc_wrap, 00288 eckey_free_wrap, 00289 eckey_debug, 00290 }; 00291 00292 /* 00293 * EC key restricted to ECDH 00294 */ 00295 static int eckeydh_can_do( pk_type_t type ) 00296 { 00297 return( type == POLARSSL_PK_ECKEY || 00298 type == POLARSSL_PK_ECKEY_DH ); 00299 } 00300 00301 const pk_info_t eckeydh_info = { 00302 POLARSSL_PK_ECKEY_DH, 00303 "EC_DH", 00304 eckey_get_size, /* Same underlying key structure */ 00305 eckeydh_can_do, 00306 NULL, 00307 NULL, 00308 NULL, 00309 NULL, 00310 eckey_check_pair, 00311 eckey_alloc_wrap, /* Same underlying key structure */ 00312 eckey_free_wrap, /* Same underlying key structure */ 00313 eckey_debug, /* Same underlying key structure */ 00314 }; 00315 #endif /* POLARSSL_ECP_C */ 00316 00317 #if defined(POLARSSL_ECDSA_C) 00318 static int ecdsa_can_do( pk_type_t type ) 00319 { 00320 return( type == POLARSSL_PK_ECDSA ); 00321 } 00322 00323 static int ecdsa_verify_wrap( void *ctx, md_type_t md_alg, 00324 const unsigned char *hash, size_t hash_len, 00325 const unsigned char *sig, size_t sig_len ) 00326 { 00327 int ret; 00328 ((void) md_alg); 00329 00330 ret = ecdsa_read_signature( (ecdsa_context *) ctx, 00331 hash, hash_len, sig, sig_len ); 00332 00333 if( ret == POLARSSL_ERR_ECP_SIG_LEN_MISMATCH ) 00334 return( POLARSSL_ERR_PK_SIG_LEN_MISMATCH ); 00335 00336 return( ret ); 00337 } 00338 00339 static int ecdsa_sign_wrap( void *ctx, md_type_t md_alg, 00340 const unsigned char *hash, size_t hash_len, 00341 unsigned char *sig, size_t *sig_len, 00342 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ) 00343 { 00344 /* Use deterministic ECDSA by default if available */ 00345 #if defined(POLARSSL_ECDSA_DETERMINISTIC) 00346 ((void) f_rng); 00347 ((void) p_rng); 00348 00349 return( ecdsa_write_signature_det( (ecdsa_context *) ctx, 00350 hash, hash_len, sig, sig_len, md_alg ) ); 00351 #else 00352 ((void) md_alg); 00353 00354 return( ecdsa_write_signature( (ecdsa_context *) ctx, 00355 hash, hash_len, sig, sig_len, f_rng, p_rng ) ); 00356 #endif /* POLARSSL_ECDSA_DETERMINISTIC */ 00357 } 00358 00359 static void *ecdsa_alloc_wrap( void ) 00360 { 00361 void *ctx = polarssl_malloc( sizeof( ecdsa_context ) ); 00362 00363 if( ctx != NULL ) 00364 ecdsa_init( (ecdsa_context *) ctx ); 00365 00366 return( ctx ); 00367 } 00368 00369 static void ecdsa_free_wrap( void *ctx ) 00370 { 00371 ecdsa_free( (ecdsa_context *) ctx ); 00372 polarssl_free( ctx ); 00373 } 00374 00375 const pk_info_t ecdsa_info = { 00376 POLARSSL_PK_ECDSA, 00377 "ECDSA", 00378 eckey_get_size, /* Compatible key structures */ 00379 ecdsa_can_do, 00380 ecdsa_verify_wrap, 00381 ecdsa_sign_wrap, 00382 NULL, 00383 NULL, 00384 eckey_check_pair, /* Compatible key structures */ 00385 ecdsa_alloc_wrap, 00386 ecdsa_free_wrap, 00387 eckey_debug, /* Compatible key structures */ 00388 }; 00389 #endif /* POLARSSL_ECDSA_C */ 00390 00391 /* 00392 * Support for alternative RSA-private implementations 00393 */ 00394 00395 static int rsa_alt_can_do( pk_type_t type ) 00396 { 00397 return( type == POLARSSL_PK_RSA ); 00398 } 00399 00400 static size_t rsa_alt_get_size( const void *ctx ) 00401 { 00402 const rsa_alt_context *rsa_alt = (const rsa_alt_context *) ctx; 00403 00404 return( 8 * rsa_alt->key_len_func( rsa_alt->key ) ); 00405 } 00406 00407 static int rsa_alt_sign_wrap( void *ctx, md_type_t md_alg, 00408 const unsigned char *hash, size_t hash_len, 00409 unsigned char *sig, size_t *sig_len, 00410 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ) 00411 { 00412 rsa_alt_context *rsa_alt = (rsa_alt_context *) ctx; 00413 00414 *sig_len = rsa_alt->key_len_func( rsa_alt->key ); 00415 00416 return( rsa_alt->sign_func( rsa_alt->key, f_rng, p_rng, RSA_PRIVATE, 00417 md_alg, (unsigned int) hash_len, hash, sig ) ); 00418 } 00419 00420 static int rsa_alt_decrypt_wrap( void *ctx, 00421 const unsigned char *input, size_t ilen, 00422 unsigned char *output, size_t *olen, size_t osize, 00423 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ) 00424 { 00425 rsa_alt_context *rsa_alt = (rsa_alt_context *) ctx; 00426 00427 ((void) f_rng); 00428 ((void) p_rng); 00429 00430 if( ilen != rsa_alt->key_len_func( rsa_alt->key ) ) 00431 return( POLARSSL_ERR_RSA_BAD_INPUT_DATA ); 00432 00433 return( rsa_alt->decrypt_func( rsa_alt->key, 00434 RSA_PRIVATE, olen, input, output, osize ) ); 00435 } 00436 00437 #if defined(POLARSSL_RSA_C) 00438 static int rsa_alt_check_pair( const void *pub, const void *prv ) 00439 { 00440 unsigned char sig[POLARSSL_MPI_MAX_SIZE]; 00441 unsigned char hash[32]; 00442 size_t sig_len = 0; 00443 int ret; 00444 00445 if( rsa_alt_get_size( prv ) != rsa_get_size( pub ) ) 00446 return( POLARSSL_ERR_RSA_KEY_CHECK_FAILED ); 00447 00448 memset( hash, 0x2a, sizeof( hash ) ); 00449 00450 if( ( ret = rsa_alt_sign_wrap( (void *) prv, POLARSSL_MD_NONE, 00451 hash, sizeof( hash ), 00452 sig, &sig_len, NULL, NULL ) ) != 0 ) 00453 { 00454 return( ret ); 00455 } 00456 00457 if( rsa_verify_wrap( (void *) pub, POLARSSL_MD_NONE, 00458 hash, sizeof( hash ), sig, sig_len ) != 0 ) 00459 { 00460 return( POLARSSL_ERR_RSA_KEY_CHECK_FAILED ); 00461 } 00462 00463 return( 0 ); 00464 } 00465 #endif /* POLARSSL_RSA_C */ 00466 00467 static void *rsa_alt_alloc_wrap( void ) 00468 { 00469 void *ctx = polarssl_malloc( sizeof( rsa_alt_context ) ); 00470 00471 if( ctx != NULL ) 00472 memset( ctx, 0, sizeof( rsa_alt_context ) ); 00473 00474 return( ctx ); 00475 } 00476 00477 static void rsa_alt_free_wrap( void *ctx ) 00478 { 00479 polarssl_zeroize( ctx, sizeof( rsa_alt_context ) ); 00480 polarssl_free( ctx ); 00481 } 00482 00483 const pk_info_t rsa_alt_info = { 00484 POLARSSL_PK_RSA_ALT, 00485 "RSA-alt", 00486 rsa_alt_get_size, 00487 rsa_alt_can_do, 00488 NULL, 00489 rsa_alt_sign_wrap, 00490 rsa_alt_decrypt_wrap, 00491 NULL, 00492 #if defined(POLARSSL_RSA_C) 00493 rsa_alt_check_pair, 00494 #else 00495 NULL, 00496 #endif 00497 rsa_alt_alloc_wrap, 00498 rsa_alt_free_wrap, 00499 NULL, 00500 }; 00501 00502 #endif /* POLARSSL_PK_C */ 00503
Generated on Tue Jul 12 2022 13:50:37 by
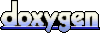