mbed TLS library
Dependents: HTTPClient-SSL WS_SERVER
pk.h
00001 /** 00002 * \file pk.h 00003 * 00004 * \brief Public Key abstraction layer 00005 * 00006 * Copyright (C) 2006-2013, ARM Limited, All Rights Reserved 00007 * 00008 * This file is part of mbed TLS (https://tls.mbed.org) 00009 * 00010 * This program is free software; you can redistribute it and/or modify 00011 * it under the terms of the GNU General Public License as published by 00012 * the Free Software Foundation; either version 2 of the License, or 00013 * (at your option) any later version. 00014 * 00015 * This program is distributed in the hope that it will be useful, 00016 * but WITHOUT ANY WARRANTY; without even the implied warranty of 00017 * MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the 00018 * GNU General Public License for more details. 00019 * 00020 * You should have received a copy of the GNU General Public License along 00021 * with this program; if not, write to the Free Software Foundation, Inc., 00022 * 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA. 00023 */ 00024 00025 #ifndef POLARSSL_PK_H 00026 #define POLARSSL_PK_H 00027 00028 #if !defined(POLARSSL_CONFIG_FILE) 00029 #include "config.h" 00030 #else 00031 #include POLARSSL_CONFIG_FILE 00032 #endif 00033 00034 #include "md.h" 00035 00036 #if defined(POLARSSL_RSA_C) 00037 #include "rsa.h" 00038 #endif 00039 00040 #if defined(POLARSSL_ECP_C) 00041 #include "ecp.h" 00042 #endif 00043 00044 #if defined(POLARSSL_ECDSA_C) 00045 #include "ecdsa.h" 00046 #endif 00047 00048 #define POLARSSL_ERR_PK_MALLOC_FAILED -0x2F80 /**< Memory alloation failed. */ 00049 #define POLARSSL_ERR_PK_TYPE_MISMATCH -0x2F00 /**< Type mismatch, eg attempt to encrypt with an ECDSA key */ 00050 #define POLARSSL_ERR_PK_BAD_INPUT_DATA -0x2E80 /**< Bad input parameters to function. */ 00051 #define POLARSSL_ERR_PK_FILE_IO_ERROR -0x2E00 /**< Read/write of file failed. */ 00052 #define POLARSSL_ERR_PK_KEY_INVALID_VERSION -0x2D80 /**< Unsupported key version */ 00053 #define POLARSSL_ERR_PK_KEY_INVALID_FORMAT -0x2D00 /**< Invalid key tag or value. */ 00054 #define POLARSSL_ERR_PK_UNKNOWN_PK_ALG -0x2C80 /**< Key algorithm is unsupported (only RSA and EC are supported). */ 00055 #define POLARSSL_ERR_PK_PASSWORD_REQUIRED -0x2C00 /**< Private key password can't be empty. */ 00056 #define POLARSSL_ERR_PK_PASSWORD_MISMATCH -0x2B80 /**< Given private key password does not allow for correct decryption. */ 00057 #define POLARSSL_ERR_PK_INVALID_PUBKEY -0x2B00 /**< The pubkey tag or value is invalid (only RSA and EC are supported). */ 00058 #define POLARSSL_ERR_PK_INVALID_ALG -0x2A80 /**< The algorithm tag or value is invalid. */ 00059 #define POLARSSL_ERR_PK_UNKNOWN_NAMED_CURVE -0x2A00 /**< Elliptic curve is unsupported (only NIST curves are supported). */ 00060 #define POLARSSL_ERR_PK_FEATURE_UNAVAILABLE -0x2980 /**< Unavailable feature, e.g. RSA disabled for RSA key. */ 00061 #define POLARSSL_ERR_PK_SIG_LEN_MISMATCH -0x2000 /**< The signature is valid but its length is less than expected. */ 00062 00063 00064 #if defined(POLARSSL_RSA_C) 00065 /** 00066 * Quick access to an RSA context inside a PK context. 00067 * 00068 * \warning You must make sure the PK context actually holds an RSA context 00069 * before using this macro! 00070 */ 00071 #define pk_rsa( pk ) ( (rsa_context *) (pk).pk_ctx ) 00072 #endif /* POLARSSL_RSA_C */ 00073 00074 #if defined(POLARSSL_ECP_C) 00075 /** 00076 * Quick access to an EC context inside a PK context. 00077 * 00078 * \warning You must make sure the PK context actually holds an EC context 00079 * before using this macro! 00080 */ 00081 #define pk_ec( pk ) ( (ecp_keypair *) (pk).pk_ctx ) 00082 #endif /* POLARSSL_ECP_C */ 00083 00084 00085 #ifdef __cplusplus 00086 extern "C" { 00087 #endif 00088 00089 /** 00090 * \brief Public key types 00091 */ 00092 typedef enum { 00093 POLARSSL_PK_NONE=0, 00094 POLARSSL_PK_RSA, 00095 POLARSSL_PK_ECKEY, 00096 POLARSSL_PK_ECKEY_DH, 00097 POLARSSL_PK_ECDSA, 00098 POLARSSL_PK_RSA_ALT, 00099 POLARSSL_PK_RSASSA_PSS, 00100 } pk_type_t; 00101 00102 /** 00103 * \brief Options for RSASSA-PSS signature verification. 00104 * See \c rsa_rsassa_pss_verify_ext() 00105 */ 00106 typedef struct 00107 { 00108 md_type_t mgf1_hash_id; 00109 int expected_salt_len; 00110 00111 } pk_rsassa_pss_options; 00112 00113 /** 00114 * \brief Types for interfacing with the debug module 00115 */ 00116 typedef enum 00117 { 00118 POLARSSL_PK_DEBUG_NONE = 0, 00119 POLARSSL_PK_DEBUG_MPI, 00120 POLARSSL_PK_DEBUG_ECP, 00121 } pk_debug_type; 00122 00123 /** 00124 * \brief Item to send to the debug module 00125 */ 00126 typedef struct 00127 { 00128 pk_debug_type type; 00129 const char *name; 00130 void *value; 00131 } pk_debug_item; 00132 00133 /** Maximum number of item send for debugging, plus 1 */ 00134 #define POLARSSL_PK_DEBUG_MAX_ITEMS 3 00135 00136 /** 00137 * \brief Public key information and operations 00138 */ 00139 typedef struct 00140 { 00141 /** Public key type */ 00142 pk_type_t type; 00143 00144 /** Type name */ 00145 const char *name; 00146 00147 /** Get key size in bits */ 00148 size_t (*get_size)( const void * ); 00149 00150 /** Tell if the context implements this type (e.g. ECKEY can do ECDSA) */ 00151 int (*can_do)( pk_type_t type ); 00152 00153 /** Verify signature */ 00154 int (*verify_func)( void *ctx, md_type_t md_alg, 00155 const unsigned char *hash, size_t hash_len, 00156 const unsigned char *sig, size_t sig_len ); 00157 00158 /** Make signature */ 00159 int (*sign_func)( void *ctx, md_type_t md_alg, 00160 const unsigned char *hash, size_t hash_len, 00161 unsigned char *sig, size_t *sig_len, 00162 int (*f_rng)(void *, unsigned char *, size_t), 00163 void *p_rng ); 00164 00165 /** Decrypt message */ 00166 int (*decrypt_func)( void *ctx, const unsigned char *input, size_t ilen, 00167 unsigned char *output, size_t *olen, size_t osize, 00168 int (*f_rng)(void *, unsigned char *, size_t), 00169 void *p_rng ); 00170 00171 /** Encrypt message */ 00172 int (*encrypt_func)( void *ctx, const unsigned char *input, size_t ilen, 00173 unsigned char *output, size_t *olen, size_t osize, 00174 int (*f_rng)(void *, unsigned char *, size_t), 00175 void *p_rng ); 00176 00177 /** Check public-private key pair */ 00178 int (*check_pair_func)( const void *pub, const void *prv ); 00179 00180 /** Allocate a new context */ 00181 void * (*ctx_alloc_func)( void ); 00182 00183 /** Free the given context */ 00184 void (*ctx_free_func)( void *ctx ); 00185 00186 /** Interface with the debug module */ 00187 void (*debug_func)( const void *ctx, pk_debug_item *items ); 00188 00189 } pk_info_t; 00190 00191 /** 00192 * \brief Public key container 00193 */ 00194 typedef struct 00195 { 00196 const pk_info_t * pk_info; /**< Public key informations */ 00197 void * pk_ctx; /**< Underlying public key context */ 00198 } pk_context; 00199 00200 /** 00201 * \brief Types for RSA-alt abstraction 00202 */ 00203 typedef int (*pk_rsa_alt_decrypt_func)( void *ctx, int mode, size_t *olen, 00204 const unsigned char *input, unsigned char *output, 00205 size_t output_max_len ); 00206 typedef int (*pk_rsa_alt_sign_func)( void *ctx, 00207 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng, 00208 int mode, md_type_t md_alg, unsigned int hashlen, 00209 const unsigned char *hash, unsigned char *sig ); 00210 typedef size_t (*pk_rsa_alt_key_len_func)( void *ctx ); 00211 00212 /** 00213 * \brief Return information associated with the given PK type 00214 * 00215 * \param pk_type PK type to search for. 00216 * 00217 * \return The PK info associated with the type or NULL if not found. 00218 */ 00219 const pk_info_t *pk_info_from_type( pk_type_t pk_type ); 00220 00221 /** 00222 * \brief Initialize a pk_context (as NONE) 00223 */ 00224 void pk_init( pk_context *ctx ); 00225 00226 /** 00227 * \brief Free a pk_context 00228 */ 00229 void pk_free( pk_context *ctx ); 00230 00231 /** 00232 * \brief Initialize a PK context with the information given 00233 * and allocates the type-specific PK subcontext. 00234 * 00235 * \param ctx Context to initialize. Must be empty (type NONE). 00236 * \param info Information to use 00237 * 00238 * \return 0 on success, 00239 * POLARSSL_ERR_PK_BAD_INPUT_DATA on invalid input, 00240 * POLARSSL_ERR_PK_MALLOC_FAILED on allocation failure. 00241 * 00242 * \note For contexts holding an RSA-alt key, use 00243 * \c pk_init_ctx_rsa_alt() instead. 00244 */ 00245 int pk_init_ctx( pk_context *ctx, const pk_info_t *info ); 00246 00247 /** 00248 * \brief Initialize an RSA-alt context 00249 * 00250 * \param ctx Context to initialize. Must be empty (type NONE). 00251 * \param key RSA key pointer 00252 * \param decrypt_func Decryption function 00253 * \param sign_func Signing function 00254 * \param key_len_func Function returning key length in bytes 00255 * 00256 * \return 0 on success, or POLARSSL_ERR_PK_BAD_INPUT_DATA if the 00257 * context wasn't already initialized as RSA_ALT. 00258 * 00259 * \note This function replaces \c pk_init_ctx() for RSA-alt. 00260 */ 00261 int pk_init_ctx_rsa_alt( pk_context *ctx, void * key, 00262 pk_rsa_alt_decrypt_func decrypt_func, 00263 pk_rsa_alt_sign_func sign_func, 00264 pk_rsa_alt_key_len_func key_len_func ); 00265 00266 /** 00267 * \brief Get the size in bits of the underlying key 00268 * 00269 * \param ctx Context to use 00270 * 00271 * \return Key size in bits, or 0 on error 00272 */ 00273 size_t pk_get_size( const pk_context *ctx ); 00274 00275 /** 00276 * \brief Get the length in bytes of the underlying key 00277 * \param ctx Context to use 00278 * 00279 * \return Key length in bytes, or 0 on error 00280 */ 00281 static inline size_t pk_get_len( const pk_context *ctx ) 00282 { 00283 return( ( pk_get_size( ctx ) + 7 ) / 8 ); 00284 } 00285 00286 /** 00287 * \brief Tell if a context can do the operation given by type 00288 * 00289 * \param ctx Context to test 00290 * \param type Target type 00291 * 00292 * \return 0 if context can't do the operations, 00293 * 1 otherwise. 00294 */ 00295 int pk_can_do( pk_context *ctx, pk_type_t type ); 00296 00297 /** 00298 * \brief Verify signature (including padding if relevant). 00299 * 00300 * \param ctx PK context to use 00301 * \param md_alg Hash algorithm used (see notes) 00302 * \param hash Hash of the message to sign 00303 * \param hash_len Hash length or 0 (see notes) 00304 * \param sig Signature to verify 00305 * \param sig_len Signature length 00306 * 00307 * \return 0 on success (signature is valid), 00308 * POLARSSL_ERR_PK_SIG_LEN_MISMATCH if the signature is 00309 * valid but its actual length is less than sig_len, 00310 * or a specific error code. 00311 * 00312 * \note For RSA keys, the default padding type is PKCS#1 v1.5. 00313 * Use \c pk_verify_ext( POLARSSL_PK_RSASSA_PSS, ... ) 00314 * to verify RSASSA_PSS signatures. 00315 * 00316 * \note If hash_len is 0, then the length associated with md_alg 00317 * is used instead, or an error returned if it is invalid. 00318 * 00319 * \note md_alg may be POLARSSL_MD_NONE, only if hash_len != 0 00320 */ 00321 int pk_verify( pk_context *ctx, md_type_t md_alg, 00322 const unsigned char *hash, size_t hash_len, 00323 const unsigned char *sig, size_t sig_len ); 00324 00325 /** 00326 * \brief Verify signature, with options. 00327 * (Includes verification of the padding depending on type.) 00328 * 00329 * \param type Signature type (inc. possible padding type) to verify 00330 * \param options Pointer to type-specific options, or NULL 00331 * \param ctx PK context to use 00332 * \param md_alg Hash algorithm used (see notes) 00333 * \param hash Hash of the message to sign 00334 * \param hash_len Hash length or 0 (see notes) 00335 * \param sig Signature to verify 00336 * \param sig_len Signature length 00337 * 00338 * \return 0 on success (signature is valid), 00339 * POLARSSL_ERR_PK_TYPE_MISMATCH if the PK context can't be 00340 * used for this type of signatures, 00341 * POLARSSL_ERR_PK_SIG_LEN_MISMATCH if the signature is 00342 * valid but its actual length is less than sig_len, 00343 * or a specific error code. 00344 * 00345 * \note If hash_len is 0, then the length associated with md_alg 00346 * is used instead, or an error returned if it is invalid. 00347 * 00348 * \note md_alg may be POLARSSL_MD_NONE, only if hash_len != 0 00349 * 00350 * \note If type is POLARSSL_PK_RSASSA_PSS, then options must point 00351 * to a pk_rsassa_pss_options structure, 00352 * otherwise it must be NULL. 00353 */ 00354 int pk_verify_ext( pk_type_t type, const void *options, 00355 pk_context *ctx, md_type_t md_alg, 00356 const unsigned char *hash, size_t hash_len, 00357 const unsigned char *sig, size_t sig_len ); 00358 00359 /** 00360 * \brief Make signature, including padding if relevant. 00361 * 00362 * \param ctx PK context to use 00363 * \param md_alg Hash algorithm used (see notes) 00364 * \param hash Hash of the message to sign 00365 * \param hash_len Hash length or 0 (see notes) 00366 * \param sig Place to write the signature 00367 * \param sig_len Number of bytes written 00368 * \param f_rng RNG function 00369 * \param p_rng RNG parameter 00370 * 00371 * \return 0 on success, or a specific error code. 00372 * 00373 * \note For RSA keys, the default padding type is PKCS#1 v1.5. 00374 * There is no interface in the PK module to make RSASSA-PSS 00375 * signatures yet. 00376 * 00377 * \note If hash_len is 0, then the length associated with md_alg 00378 * is used instead, or an error returned if it is invalid. 00379 * 00380 * \note md_alg may be POLARSSL_MD_NONE, only if hash_len != 0 00381 */ 00382 int pk_sign( pk_context *ctx, md_type_t md_alg, 00383 const unsigned char *hash, size_t hash_len, 00384 unsigned char *sig, size_t *sig_len, 00385 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ); 00386 00387 /** 00388 * \brief Decrypt message (including padding if relevant). 00389 * 00390 * \param ctx PK context to use 00391 * \param input Input to decrypt 00392 * \param ilen Input size 00393 * \param output Decrypted output 00394 * \param olen Decrypted message length 00395 * \param osize Size of the output buffer 00396 * \param f_rng RNG function 00397 * \param p_rng RNG parameter 00398 * 00399 * \note For RSA keys, the default padding type is PKCS#1 v1.5. 00400 * 00401 * \return 0 on success, or a specific error code. 00402 */ 00403 int pk_decrypt( pk_context *ctx, 00404 const unsigned char *input, size_t ilen, 00405 unsigned char *output, size_t *olen, size_t osize, 00406 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ); 00407 00408 /** 00409 * \brief Encrypt message (including padding if relevant). 00410 * 00411 * \param ctx PK context to use 00412 * \param input Message to encrypt 00413 * \param ilen Message size 00414 * \param output Encrypted output 00415 * \param olen Encrypted output length 00416 * \param osize Size of the output buffer 00417 * \param f_rng RNG function 00418 * \param p_rng RNG parameter 00419 * 00420 * \note For RSA keys, the default padding type is PKCS#1 v1.5. 00421 * 00422 * \return 0 on success, or a specific error code. 00423 */ 00424 int pk_encrypt( pk_context *ctx, 00425 const unsigned char *input, size_t ilen, 00426 unsigned char *output, size_t *olen, size_t osize, 00427 int (*f_rng)(void *, unsigned char *, size_t), void *p_rng ); 00428 00429 /** 00430 * \brief Check if a public-private pair of keys matches. 00431 * 00432 * \param pub Context holding a public key. 00433 * \param prv Context holding a private (and public) key. 00434 * 00435 * \return 0 on success or POLARSSL_ERR_PK_BAD_INPUT_DATA 00436 */ 00437 int pk_check_pair( const pk_context *pub, const pk_context *prv ); 00438 00439 /** 00440 * \brief Export debug information 00441 * 00442 * \param ctx Context to use 00443 * \param items Place to write debug items 00444 * 00445 * \return 0 on success or POLARSSL_ERR_PK_BAD_INPUT_DATA 00446 */ 00447 int pk_debug( const pk_context *ctx, pk_debug_item *items ); 00448 00449 /** 00450 * \brief Access the type name 00451 * 00452 * \param ctx Context to use 00453 * 00454 * \return Type name on success, or "invalid PK" 00455 */ 00456 const char * pk_get_name( const pk_context *ctx ); 00457 00458 /** 00459 * \brief Get the key type 00460 * 00461 * \param ctx Context to use 00462 * 00463 * \return Type on success, or POLARSSL_PK_NONE 00464 */ 00465 pk_type_t pk_get_type( const pk_context *ctx ); 00466 00467 #if defined(POLARSSL_PK_PARSE_C) 00468 /** \ingroup pk_module */ 00469 /** 00470 * \brief Parse a private key 00471 * 00472 * \param ctx key to be initialized 00473 * \param key input buffer 00474 * \param keylen size of the buffer 00475 * \param pwd password for decryption (optional) 00476 * \param pwdlen size of the password 00477 * 00478 * \note On entry, ctx must be empty, either freshly initialised 00479 * with pk_init() or reset with pk_free(). If you need a 00480 * specific key type, check the result with pk_can_do(). 00481 * 00482 * \note The key is also checked for correctness. 00483 * 00484 * \return 0 if successful, or a specific PK or PEM error code 00485 */ 00486 int pk_parse_key( pk_context *ctx, 00487 const unsigned char *key, size_t keylen, 00488 const unsigned char *pwd, size_t pwdlen ); 00489 00490 /** \ingroup pk_module */ 00491 /** 00492 * \brief Parse a public key 00493 * 00494 * \param ctx key to be initialized 00495 * \param key input buffer 00496 * \param keylen size of the buffer 00497 * 00498 * \note On entry, ctx must be empty, either freshly initialised 00499 * with pk_init() or reset with pk_free(). If you need a 00500 * specific key type, check the result with pk_can_do(). 00501 * 00502 * \note The key is also checked for correctness. 00503 * 00504 * \return 0 if successful, or a specific PK or PEM error code 00505 */ 00506 int pk_parse_public_key( pk_context *ctx, 00507 const unsigned char *key, size_t keylen ); 00508 00509 #if defined(POLARSSL_FS_IO) 00510 /** \ingroup pk_module */ 00511 /** 00512 * \brief Load and parse a private key 00513 * 00514 * \param ctx key to be initialized 00515 * \param path filename to read the private key from 00516 * \param password password to decrypt the file (can be NULL) 00517 * 00518 * \note On entry, ctx must be empty, either freshly initialised 00519 * with pk_init() or reset with pk_free(). If you need a 00520 * specific key type, check the result with pk_can_do(). 00521 * 00522 * \note The key is also checked for correctness. 00523 * 00524 * \return 0 if successful, or a specific PK or PEM error code 00525 */ 00526 int pk_parse_keyfile( pk_context *ctx, 00527 const char *path, const char *password ); 00528 00529 /** \ingroup pk_module */ 00530 /** 00531 * \brief Load and parse a public key 00532 * 00533 * \param ctx key to be initialized 00534 * \param path filename to read the private key from 00535 * 00536 * \note On entry, ctx must be empty, either freshly initialised 00537 * with pk_init() or reset with pk_free(). If you need a 00538 * specific key type, check the result with pk_can_do(). 00539 * 00540 * \note The key is also checked for correctness. 00541 * 00542 * \return 0 if successful, or a specific PK or PEM error code 00543 */ 00544 int pk_parse_public_keyfile( pk_context *ctx, const char *path ); 00545 #endif /* POLARSSL_FS_IO */ 00546 #endif /* POLARSSL_PK_PARSE_C */ 00547 00548 #if defined(POLARSSL_PK_WRITE_C) 00549 /** 00550 * \brief Write a private key to a PKCS#1 or SEC1 DER structure 00551 * Note: data is written at the end of the buffer! Use the 00552 * return value to determine where you should start 00553 * using the buffer 00554 * 00555 * \param ctx private to write away 00556 * \param buf buffer to write to 00557 * \param size size of the buffer 00558 * 00559 * \return length of data written if successful, or a specific 00560 * error code 00561 */ 00562 int pk_write_key_der( pk_context *ctx, unsigned char *buf, size_t size ); 00563 00564 /** 00565 * \brief Write a public key to a SubjectPublicKeyInfo DER structure 00566 * Note: data is written at the end of the buffer! Use the 00567 * return value to determine where you should start 00568 * using the buffer 00569 * 00570 * \param ctx public key to write away 00571 * \param buf buffer to write to 00572 * \param size size of the buffer 00573 * 00574 * \return length of data written if successful, or a specific 00575 * error code 00576 */ 00577 int pk_write_pubkey_der( pk_context *ctx, unsigned char *buf, size_t size ); 00578 00579 #if defined(POLARSSL_PEM_WRITE_C) 00580 /** 00581 * \brief Write a public key to a PEM string 00582 * 00583 * \param ctx public key to write away 00584 * \param buf buffer to write to 00585 * \param size size of the buffer 00586 * 00587 * \return 0 successful, or a specific error code 00588 */ 00589 int pk_write_pubkey_pem( pk_context *ctx, unsigned char *buf, size_t size ); 00590 00591 /** 00592 * \brief Write a private key to a PKCS#1 or SEC1 PEM string 00593 * 00594 * \param ctx private to write away 00595 * \param buf buffer to write to 00596 * \param size size of the buffer 00597 * 00598 * \return 0 successful, or a specific error code 00599 */ 00600 int pk_write_key_pem( pk_context *ctx, unsigned char *buf, size_t size ); 00601 #endif /* POLARSSL_PEM_WRITE_C */ 00602 #endif /* POLARSSL_PK_WRITE_C */ 00603 00604 /* 00605 * WARNING: Low-level functions. You probably do not want to use these unless 00606 * you are certain you do ;) 00607 */ 00608 00609 #if defined(POLARSSL_PK_PARSE_C) 00610 /** 00611 * \brief Parse a SubjectPublicKeyInfo DER structure 00612 * 00613 * \param p the position in the ASN.1 data 00614 * \param end end of the buffer 00615 * \param pk the key to fill 00616 * 00617 * \return 0 if successful, or a specific PK error code 00618 */ 00619 int pk_parse_subpubkey( unsigned char **p, const unsigned char *end, 00620 pk_context *pk ); 00621 #endif /* POLARSSL_PK_PARSE_C */ 00622 00623 #if defined(POLARSSL_PK_WRITE_C) 00624 /** 00625 * \brief Write a subjectPublicKey to ASN.1 data 00626 * Note: function works backwards in data buffer 00627 * 00628 * \param p reference to current position pointer 00629 * \param start start of the buffer (for bounds-checking) 00630 * \param key public key to write away 00631 * 00632 * \return the length written or a negative error code 00633 */ 00634 int pk_write_pubkey( unsigned char **p, unsigned char *start, 00635 const pk_context *key ); 00636 #endif /* POLARSSL_PK_WRITE_C */ 00637 00638 /* 00639 * Internal module functions. You probably do not want to use these unless you 00640 * know you do. 00641 */ 00642 #if defined(POLARSSL_FS_IO) 00643 int pk_load_file( const char *path, unsigned char **buf, size_t *n ); 00644 #endif 00645 00646 #ifdef __cplusplus 00647 } 00648 #endif 00649 00650 #endif /* POLARSSL_PK_H */ 00651
Generated on Tue Jul 12 2022 13:50:37 by
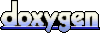